
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers
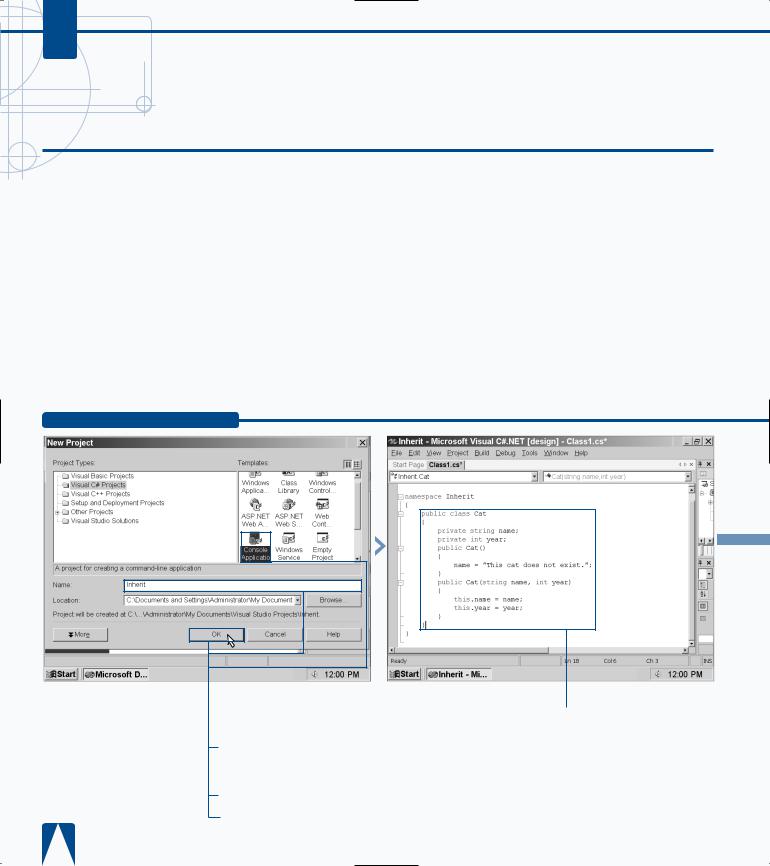
C#
EMPLOY CLASS INHERITANCE
ou can create classes with objects that more than one Yclass can refer to. Class inheritance lets you define
objects in a class once and then have other classes in your program refer to those objects.
Class inheritance speeds up the programming process by reusing code from a base class in other inheriting classes without adding extra code. You can also change objects in your base class that apply to all the inheriting classes.
Inheritance is not the best solution for all programming circumstances — interfaces can provide a better solution. See page 110 for more information on class inheritance and interfaces.
C# only gives you the ability to inherit classes from one base class. The base class is the very first class that all other classes inherit from. Like C++, you can have nested classes. Nested classes let you create classes within the class that you are programming in the MDE parent window. A nested class directly inherits from its parent class. Having nested classes makes it faster for you to create inheriting classes than if you created new classes one at a time.
You can override some base class information such as methods in your inheriting class, but for the most part inheriting classes observe the base class rules.
EMPLOY CLASS INHERITANCE
Console |
Applicatio |
New Project window
.
Click the Console
Application icon in the
Templates pane.
Type a name for the file.
Click OK.
■ The Class1.cs code appears in the parent window.
Á Delete all code after the namespace Inherit code.
‡ Type the code that establishes the variables and constructors.

PROGRAMMING C# BUILDING BLOCKS 4
The rule in C# is that there is only one base class. If you try to create classes with the same name that inherit from different base classes, C# will not let you do so.
Example:
abstract class A // Base class A
{
}
class B : A // Inherits from class A
{
}
class C: B // C inherits from B that inherits from A
{
}
abstract class D // new base class
{
}
class C: D // Error; you cannot inherit from two base classes at once
{
}
° Type the code that outputs the information
and the inheriting class that processes the information for output.
· Run the program by pressing the F5 key.
■ The output strings appear on the screen.
‚ Save the program as the filename.
Note: You may want to change the summary comment in the class to note to others that NewClass inherits from Class1.
73

C#
PROGRAM INSTANCE CONSTRUCTORS
class has two key parts: constructors and destructors. AA constructor is a declaration that tells the compiler
what type of class you have created, the features of your class, and how you will treat every instance of your class.
An instance is a variable of an object in the class. For example, two separate instances in the Cat class can be Mewsette and Tigger. Every member that belongs to your class has a status associated with it: static or instance.
A static member of a class belongs to the class itself, not any specific instance, and maintains a current value. This is
useful if you want to have a class of passwords with information that does not change. An instance lets you input different variables for your class. For example, if you have a Cat class, you can include various types of information such as name, weight, breed, and so on.
The instance constructor initializer is a piece of code that implements initialization of an instance of a class — in short, the piece of code that makes your class work. If you do not have a constructor in your class, C# adds one automatically — constructor initializers are that important. When you add a new class, C# adds the constructor initializer automatically.
PROGRAM INSTANCE CONSTRUCTORS
Console
Applicatio
Project in the ■ The New Project window appears.
‹ Click the Console Application icon in the Templates pane.
› Type a name for the file.
ˇ Click OK.

|
|
PROGRAMMING C# BUILDING BLOCKS |
4 |
|
|
||
|
|
|
|
|
|
|
|
|
You can add the this keyword so a class or struct can overload |
|
|
|
|
||
|
existing constructors and call one of its own constructors instead. |
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
TYPE THIS: |
|
RESULT: |
|
|
|
|
|
using System; |
|
2 |
|
|
|
|
|
class Class1 { |
|
4 |
|
|
|
|
|
public int a; |
|
|
|
|
|
|
|
public Class1() : this (2) //gives a the value of 2 { } |
|
|
|
|
|
|
|
public Class1(int b) { |
|
|
|
|
|
|
|
a = b //overloads the existing constructor } |
|
|
|
|
|
|
|
static void Main(string[] args) { |
|
|
|
|
|
|
|
Class1 x1 = new Class1(); |
|
|
|
|
|
|
|
Class1 x2 = new Class1(4); // 4 is the new value per |
|
|
|
|
|
|
|
the overloaded constructor |
|
|
|
|
|
|
|
Console.WriteLine(x1.a); |
|
|
|
|
|
|
|
Console.WriteLine(x2.a); |
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
■ The Class1.cs code appears in the parent window.
Á Delete all code after the namespace Construct code.
‡ Type the code that establishes the integer.
°Type the Amount constructor that sets the initial value.
CONTINUED
75
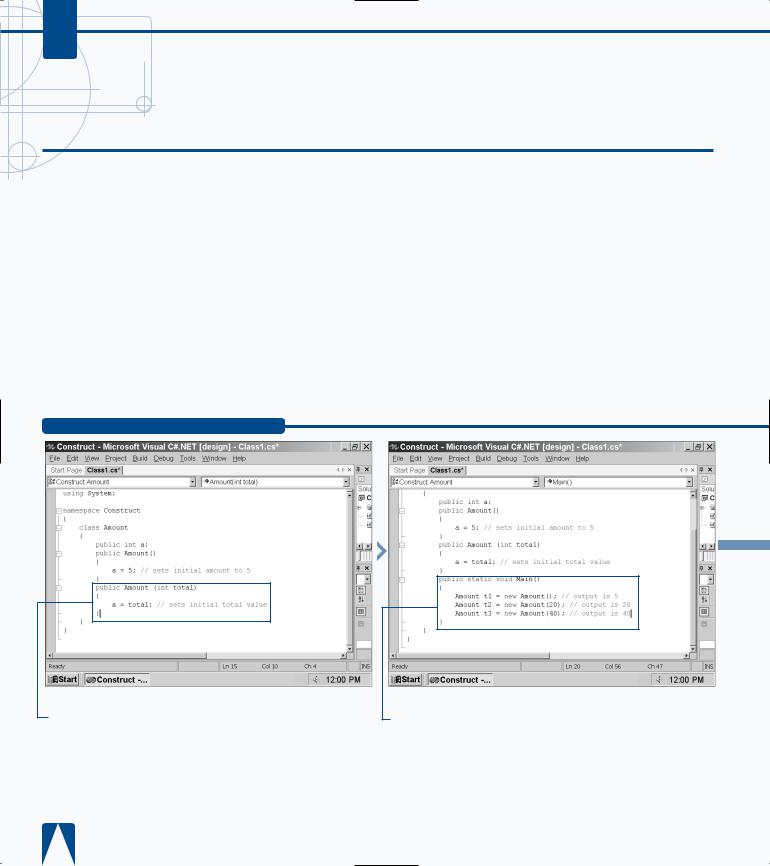
C#
PROGRAM INSTANCE CONSTRUCTORS
he constructor declaration can include a set of Tattributes that help define what it is the class is doing.
For example, if your class is creating a method, you can set attributes that determine how your class can be accessed. You can also add access modifiers to the class no matter what type of class you construct. These five access modifiers — public, protected internal, protected, internal, or private — determine your class accessibility.
The constructor initializer is only meant as the starting (and defining) point for the constructor itself. If you have a nested class, that nested class constructor initializer can access the parameters in the parent class constructor
initializer. For example, you can have a class with the constructor class One and the constructor of the second class can be class Two: One. The constructor initializer cannot, however, access any parameters in the constructor itself.
If you have an object in an inherited class, you can determine what class the object accesses — the base keyword tells the object to access class information from the base class, and the this keyword tells the object to access class information from the class in which it resides. If a constructor has no initializer, C# creates a base variable automatically.
PROGRAM INSTANCE CONSTRUCTORS
‚ Type the amount values in the Main method.
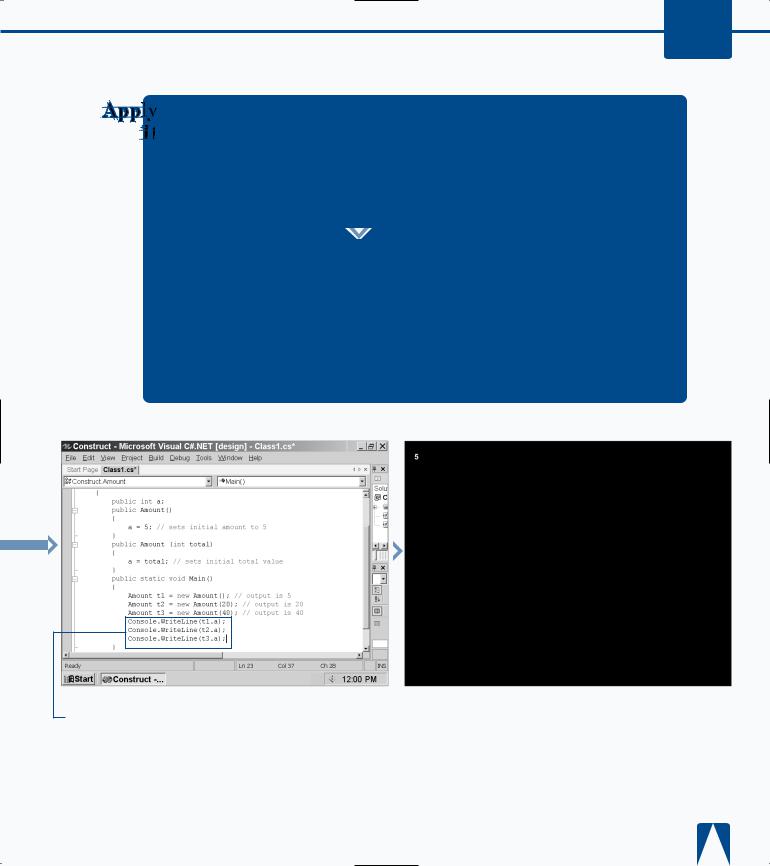
PROGRAMMING C# BUILDING BLOCKS 4
You can create two separate classes in one by creating an internal class and then accessing the internal class from another class.
|
TYPE THIS IN CLASS1: |
|
|
RESULT: |
|
|
|
||||
|
internal class Base { |
|
|
The MDE window |
|
|
public static int x = 0; |
|
|
|
|
|
|
|
reports an error |
|
|
|
} |
|
|
|
|
|
|
|
because your class Test |
|
|
|
|
|
|
|
|
|
|
|
|
is trying to create a |
|
|
|
|
|
new instance from the |
|
|
TYPE THIS IN CLASS2: |
|
abstract class Base. |
|
|
|
class Test |
|
|
|
|
|
{ |
|
|
|
|
|
public static void Main() |
|
|
|
|
|
{ |
|
|
|
|
|
Base thisBase = new Base(); |
|
|
|
|
|
} |
|
|
|
|
|
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
— Type the output code for all three values in the Main method.
± Run the program by |
¡ Save the program as the |
pressing the F5 key. |
filename. |
■ The value appears on the |
|
screen. |
|
77