
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers
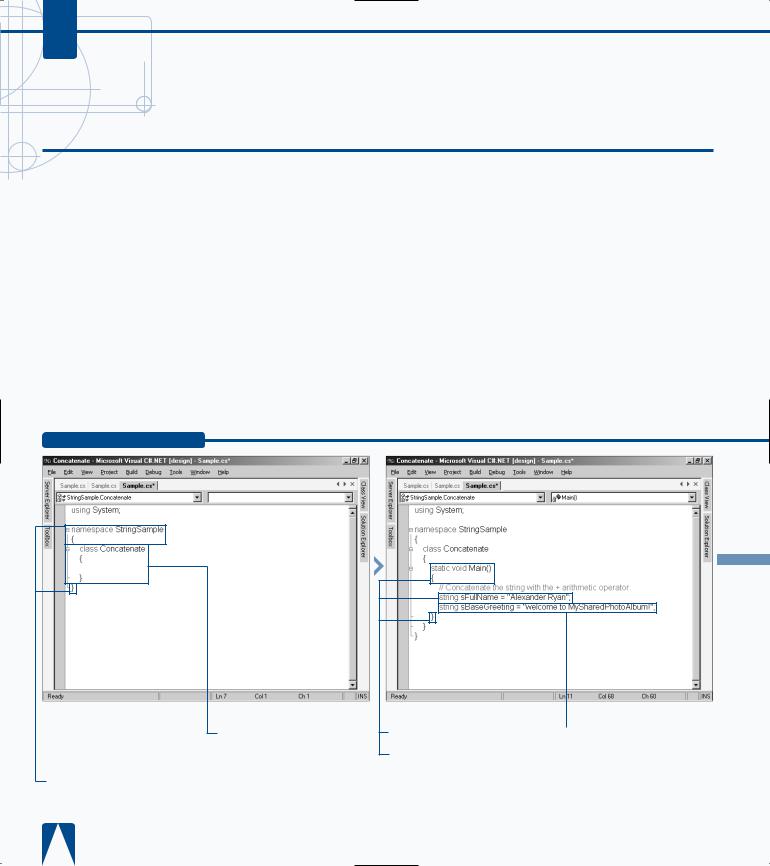
C#
CONCATENATE STRINGS
Concatenating, or joining, strings is a common task for building useful strings. Most of the time, you build strings from more than one source. Values for strings
can come from a combination of sources (database calls, constants, integer counters, and so on).
To build out a string from multiple sources, you concatenate these strings in a specified sequence. You can accomplish the concatenate of two or more string sources in several ways. You can use the arithmetic operator (+) or the (+=) assignment operator. Use the arithmetic operator (+) to combine strings in the order that they appear in the
expression, or use the assignment operator (+=) to append a string to an existing string. As you append your strings, you have to include the spacing inside the double-quotes of your string.
You can also use the Concat method on the String class to perform concatenation. With this method, you can concatenate one or more String classes together and get a new String returned to you. Another overloaded implementation of the String Class allows you to pass a string array, which is handy if you have many strings to concatenate into one representative string.
CONCATENATE STRINGS
‹ Change the class name to
Concatenate.
› Save the file.
ˇ Add the Main function.
Á Create a string variable and initialize the string with a name.
‡ Create another string variable and initialize the string with a greeting.
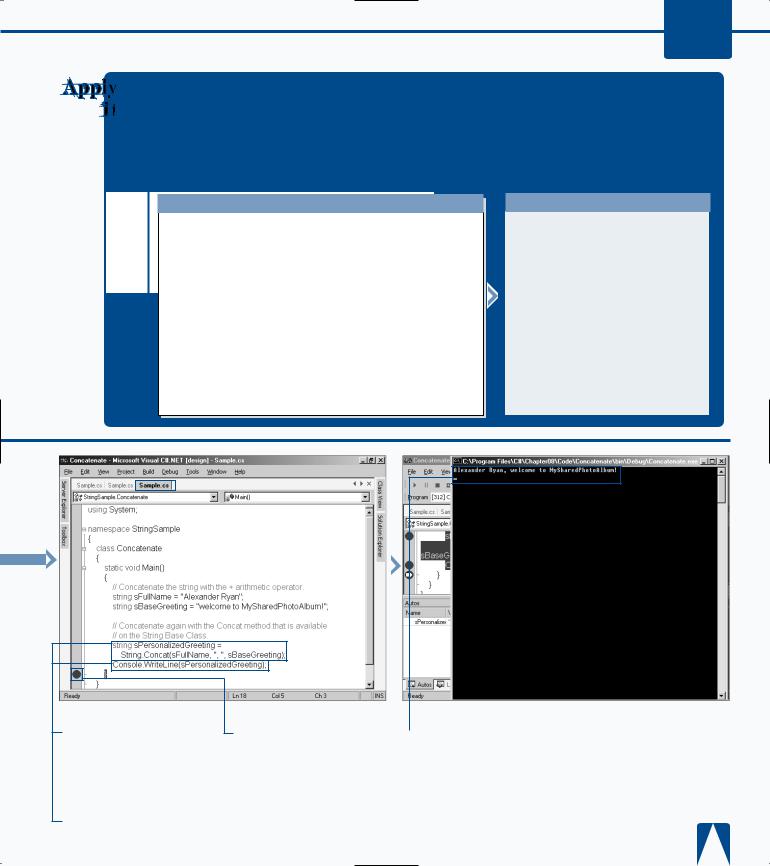
WORKING WITH STRINGS 8
Knowing that the String object is immutable and that it returns a new instance of a String object, you may want to explore the
System.Text.StringBuilder framework class. The
StringBuilder class lets you concatenate strings without having to create a new object each time you modify the string. The StringBuilder class also gives you more flexibility with concatenating, like appending versus inserting.
TYPE THIS:
using System; using System.Text;
namespace StringSample
{
class Concatenate
{
static void Main()
{
StringBuilder sbPersonalGreeting =
new StringBuilder("Hello, how are you today");
sbPersonalGreeting.Insert(0,"Danny - "); sbPersonalGreeting.Append("?");
Console.WriteLine(sbPersonalGreeting);
}
}
}
RESULT:
C:\> csc ConcatenateStrings_ai.cs
C:\> ConcatenateStrings_ai.exe
Danny – Hello, how are you today?
C:\>
° Create a new string variable and initialize the variable by using the
String.Concat function and the two strings previously created.
· Write the resulting string to the console.
‚ Set a debug stop.
— Press F5 to save, build, and run the console application.
■ A message appears showing the concatenated string.
163
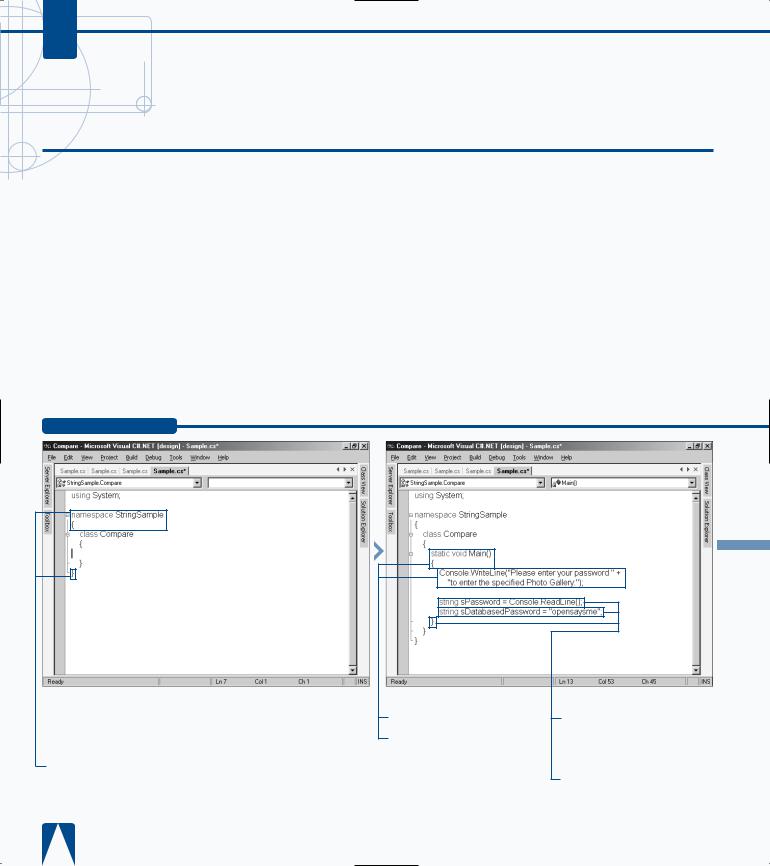
C#
COMPARE STRINGS
Comparing strings in code is useful when performing logical operations. String comparisons are useful in expressions that are used for an if or switch
statement. For example, you can use a string comparison when someone is logging onto your Web site. You can compare the password that the user entered to the password in the database.
There are several comparison methods for a string, the simplest being two equals signs (==), which is the equality operator. This operator checks to see if the two strings hold the same value (length, characters, and sequence of characters).
The String class contains some very useful comparison methods — Compare, CompareOrdinal, CompareTo, StartsWith, EndsWith, Equals, IndexOf, and
LastIndexOf. The method you choose depends on if you are looking for a binary response (for example, getting a true or false for the presence of a substring, or if both strings match based on the method’s criteria) or position of where a substring exists.
With the Compare method, the comparison is done in the expression of the if statement. Note that it returns an integer, which is used in a comparison to zero. If zero is returned, then a match is found.
COMPARE STRINGS
‹ Rename the class name to
Compare.
› Save the file.
to
ˇ Add the Main function.
Á Use the WriteLine method to prompt the user for the password.
‡ Create a string variable that is initialized with the value that is read from the console.
° Create a string variable for the password and set the password.

WORKING WITH STRINGS 8
Another way to approach string comparisons is to run the CompareTo method on the first string variable and give it the second string variable as the parameter to that method.
TYPE THIS:
using System;
namespace StringSample
{
class Compare
{
static void Main()
{
Console.WriteLine("Please enter your password " + "to enter the specified Photo Gallery:");
string sPassword = Console.ReadLine(); string sDatabasedPassword = "opensaysme";
if (sDatabasedPassword.CompareTo(sPassword)==0) Console.WriteLine("You can view the photos");
else
Console.WriteLine("You do not have permission" + " to view the photos");
}
}
}
RESULT:
C:\>csc CompareStrings.cs
C:\> CompareStrings.exe
Please enter your password to enter the specified Photo Gallery.
Opensaysme
You can view the photos.
c:\>
· Check the password using an if statement and write a message to the console if the password matches.
‚ Use an else statement if the password does not match and write another message to the console.
— Set a debug stop.
± Press F5 to save, build, and run the console application.
■ A message prompting for the password appears.
¡ Type in the password of opensaysme.
■ A message about being able to view the photos appears.
165