
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers
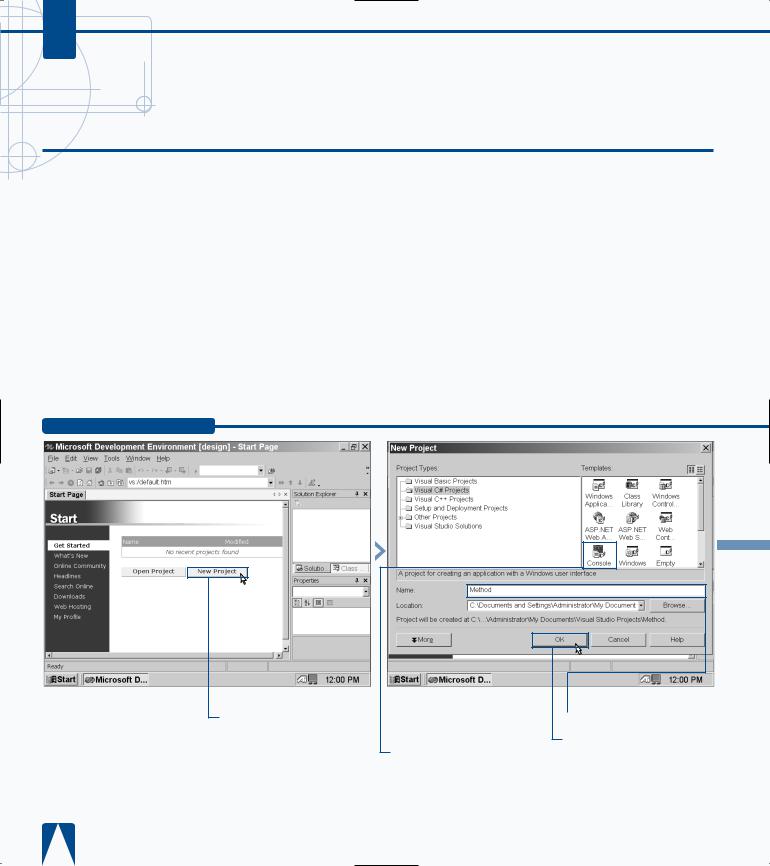
C#
VIEW THE MAIN METHOD
The MDE window automates some code generation so you can better use your time writing the meat of your code.
After you create a new C# program, the MDE window creates the basic structure of your program based on the program you want to create. For example, if you create a console application, then the MDE window will create one class with a Main method included so you can perform functions such as specify variables, perform calculations, and output results on the screen.
The Main method is the block of code where you perform many of your functions. Without a Main method your C#
program cannot run, so no matter what type of C# project you want to create, the MDE window will always include a skeleton Main method — a Main method that does not have any functional code within it.
The default state for the Main method is void — the method returns no values of its own. Instead, the method processes the code within it. For example, you can add two numbers and output the result.
Depending on the type of project you create, the Main method contains comments that tell you to replace the comments with the functional code.
VIEW THE MAIN METHOD
¤ Click New Project in the Start page.
■ The New Project window appears.
‹ Click the Console Application icon in the Templates pane.
› Type a name for your file.
ˇ Click OK.
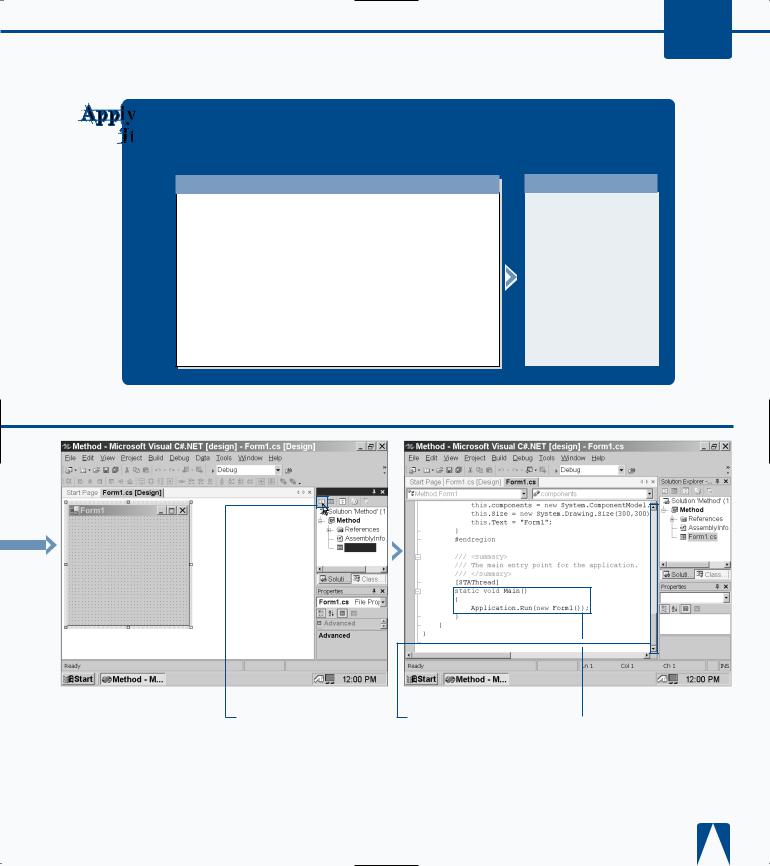
WORKING WITH VISUAL C# BASICS 3
The Length property lets you test the Main method that contains the string arguments to see if the method works as it should.
TYPE THIS:
using System; class Class1;
{
public static void Main(string[] args) if (args.Length == 0)
{
Console.Writeline("Please enter a numeric argument: ");
return 1;
}
}
RESULT:
Please enter a numeric argument: 1
The return statement is the last statement in the Main method and returns the number 1 as the output.
Solution Explorer...
Form1.cs
■ The form appears in the parent window.
Á Click the View Code button in the Solution Explorer window.
‡ Scroll down the code window until you reach the bottom.
■ The Main method appears that tells the application to run the form.
39
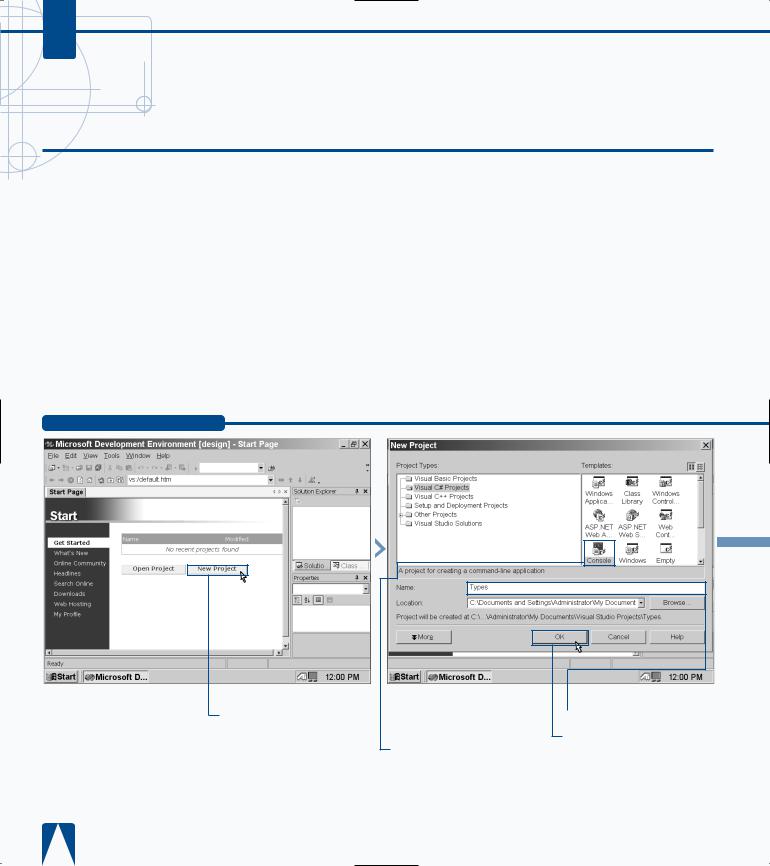
C#
COMBINE PROGRAM TYPES
C# categorizes the elements it uses, such as numbers and characters, into types. These types include predefined basic types, such as numeric and Boolean
types, and user-defined types that include structs and enumerated types. Basic types include numbers and the type the number belongs to identifies the kind of number it is. For example, a number that is an integer can only be a whole number in a range from –2,147,643,848 to 2,147,483,647. Integers cannot have decimal places; numbers with decimal places belong in the decimal type. You declare these types when you equate a number with a variable, such as declaring that the number 5 is an integer.
As with other programming languages, C# requires that you declare the correct type for its associated number.
Numeric types belong to the struct category that is one of the two large C# type categories. The other is the enumeration type. The enumeration type lets you specify a list of constants and then assigns numbers to those constants so you can select one of the constants for use in your program. For example, you can specify months of the year in an enumeration and then output a month on the screen by calling the enumeration number associated with that month.
COMBINE PROGRAM TYPES
Click New Project in the Start page.
■ The New Project window appears.
‹ Click the Console Application icon in the Templates pane.
› Type a name for your file.
ˇ Click OK.
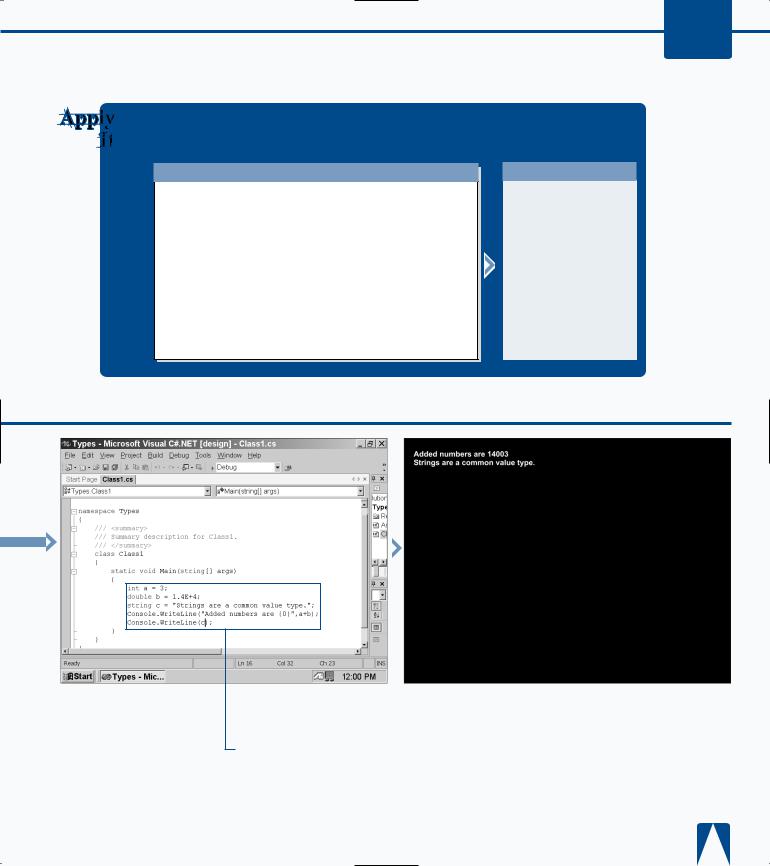
WORKING WITH VISUAL C# BASICS 3
You can determine the value of your constants by assigning constants to the first enumeration element.
TYPE THIS:
using System;
public EnumClass
{
enum WeekDays {Mon=1, Tues, Wed, Thurs, Fri, Sat, Sun}
public static void Main()
{
int x = (int) WeekDays.Wed;
Console.WriteLine("The Wednesday enum value is {0}", x);
}
}
RESULT:
The Wednesday enum value is 3.
■ The Class1.cs code appears in the parent window.
Note: You can make more room for your Start page by clicking and dragging the right edge of the Start page until you reach the maximum size for the Start page.
Á Delete the comments within the Main method.
‡ Type the code that defines some numeric and string types, adds the numeric types, and outputs the result.
° Run the program by |
· Save the program as the |
pressing the F5 key. |
filename. |
■ The combined numeric |
|
types and string types appear |
|
on the screen. |
|
41