
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers
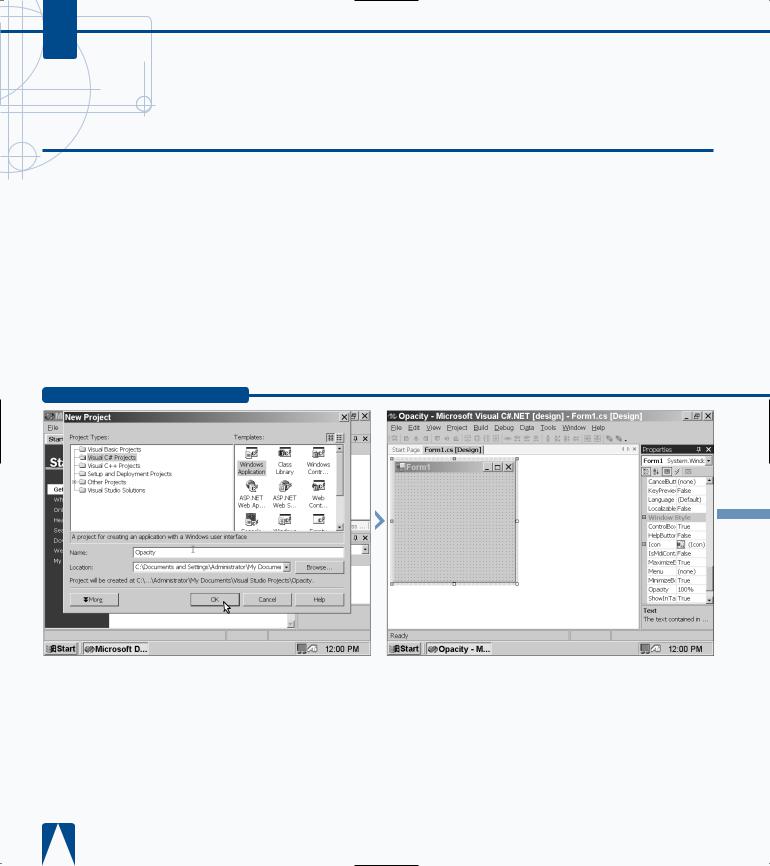
C#
CREATE A TRANSPARENT FORM
f you design a C# program for use with Windows 2000 IServer or Professional, then you can control the opacity of the form. Windows 2000 lets you determine how transparent or how solid the form appears on your screen.
A less opaque, or solid, form on your screen is very useful if you want to have a form that is not currently selected in the background so users will know that they cannot use that form. You may also want to keep a form completely transparent to the user so you can keep the form within that space so other elements do not infringe upon that space.
You set the form opacity level by setting the Opacity property in the Properties window. The opacity level ranges from 0% completely transparent to 100% completely opaque. The two digits after the decimal point represent the percentage of form opacity. After you set the opacity property, the form becomes more or less opaque depending on your setting. The default opacity setting is 100%.
If your program users do not use a version of Windows 2000, then the Opacity property will not apply, and the form will appear on the user’s screen as completely opaque.
CREATE A TRANSPARENT FORM
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Á Click the Properties |
|
‡ Scroll down to the |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
the Windows |
|
|
|
|||||||
|
|
|
|
|
|
|
|
|
||||||||
|
|
|
|
|
|
|
|
|||||||||
|
|
|
|
|
|
|
icon in the |
window. |
Opacity property in the list. |
|
||||||
|
|
|
|
|
|
|
pane. |
|
|
|
|
|
|
|||
|
|
|
|
|
|
a name for the file. |
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
|||||
|
|
|
|
|
|
OK. |
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
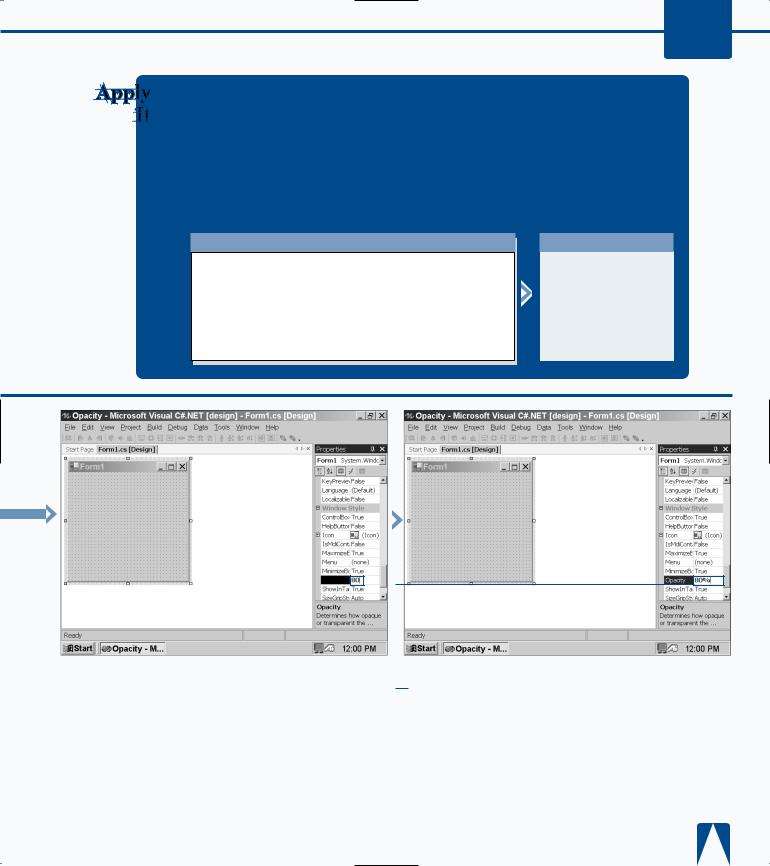
BUILDING FORMS 10
If your run you program on a computer running Windows 2000 or later, you can set the opacity of your form to make the form more transparent. Making your form less opaque can let the user know that the form is inactive, or you can hide the form from the user by making the form completely transparent. Type the following code with the public class
Form:System.Windows.Forms.Form.
TYPE THIS:
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container() this.Size = new System.Drawing.Size(100,100); this.Text = "MyForm";
this.Opacity = 0.8;
}
RESULT:
The form opacity is set to 80 percent — a 100 percent opacity level is 1 — which results in a somewhat faded form on the screen.
Opacity
|
° Type the Opacity |
|
|
■ The new Opacity |
|
|
|||
percentage and press Enter. |
|
percentage appears in |
||
|
|
|
|
bold type. |
■ If you run your program in Windows 2000, the form appears at 80 percent opacity.
· Save the program as the filename.
215

C#
AN INTRODUCTION TO WEB FORMS AND CONTROLS
The Net Platform
The .NET platform provides the ASP.NET Framework for building user interfaces for Web applications. Even though ASP.NET is a totally new framework, you may find ASP.NET applications easy to develop due to many of the transferable skills that come from development with ASP applications. ASP.NET runs on the same platform that ASP applications run on today, Windows 2000 and Internet Information Server (IIS) 5.0. ASP.NET applications uses Web Forms as the primary file type, which have an extension of .aspx. IIS processes this
file type through a special Internet Server Application Program Interface (ISAPI) filter that handles the requested Web Form. Web Forms are a close relative to ASP (Active Server Page) pages. The server-side processing in ASP.NET applications exposes to you a vast amount of information that ASP hides and makes available only if you program in C++ for ISAPI extensions and filters. Even though the information is exposed, you are able to use some of the shortcuts that are available with ASP applications.
Web Form Controls
When building Web Forms, you choose from two classifications of controls: The Web Server Controls, which resides in the System.Web.UI.WebControls namespace, and the HTML Controls, which are in the namespace System.Web.UI.HtmlControls. The
HTML Controls directly map to standard HTML tags, which all browsers support. For example, the HTMLButton class maps to a button html tag. The Web
Server Controls are more abstract classes whose object model does not necessarily reflect HTML syntax. They include most standard HTML tags and extend them with controls that implement the use of multiple HTML tags to render the control. For example, the DataGrid Class can generate table tags, anchor tags, and/or button tags, depending on how it is configured in design time. Using Web Server Controls requires you to use the asp namespace inside of the Web Form. For example, the Button Web server control has the following syntax inside of the Web Form: <ASP:BUTTON ID="cmdContinue" TEXT="Continue" onClick="Button_OnClick" RUNAT="Server"/>. Compare this to the definition
the equivalent HTML control has as well as to the equivalent standard HTML tag:
HTML Control: <input type=submit value="Enter"ID="cmd Continue OnServerClick="Submit_Click" runat=server>.
Standard HTML tag: <input type=submit value="Enter" ID="cmdContinue OnServerClick="Submit_Click">.
The main difference between the Web Server and HTML Controls is that the element on the Web Form has a runat="server" attribute. This attribute allows for capabilities that are present in server-side code. The main difference between the Web Server Controls and HTML Controls is the namespace provided for the Web Server Controls (asp:).
This chapter gives you a quick overview of ASP.NET programming. You can read the book ASP.NET: Your visual blueprint for creating Web applications on the
.NET framework (Hungry Minds, Inc., 2001), if you want to dig into further details of Web development with ASP.NET.
216
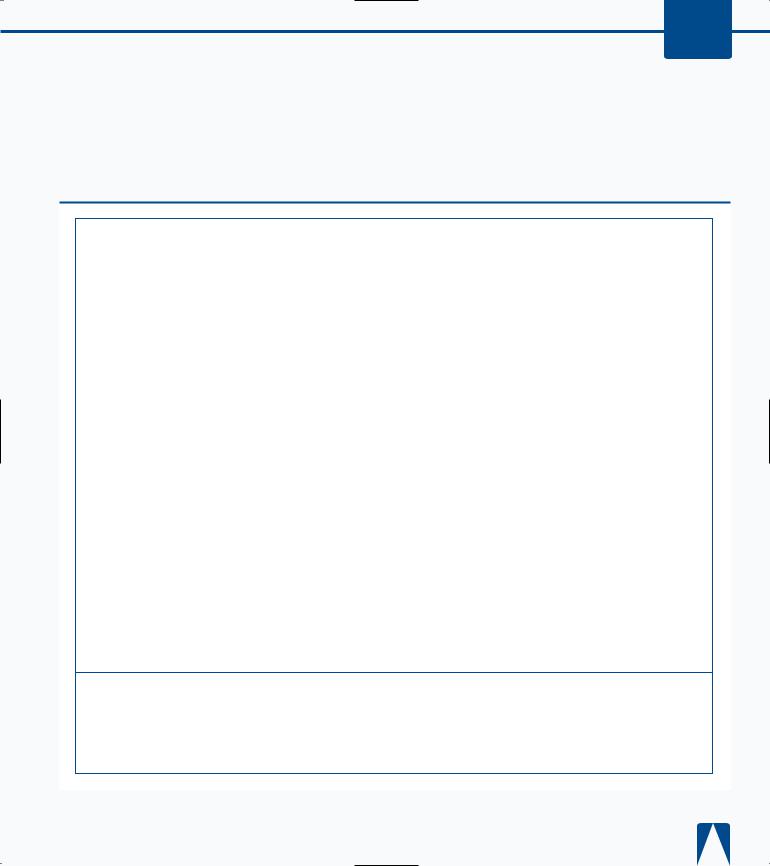
PROGRAMMING WEB APPLICATIONS 11
Separation of User Interface and User Services
ASP.NET applications give you the ability to separate user interface code and your user services code. The user interface code, which is your HTML tags, typically requires different skills than a developer that is responsible for user services code, the code that supports your user interface and runs on the Web server. This separation of code is a welcomed change to development of Web applications on the Microsoft platform; having this code seperation promotes more of the service-based model that Microsoft supports. This code separation also yields a programming style in ASP.NET applications that is better-structured code compared to the ASP style of programming.
The standard type of page that you develop on an ASP.NET application is a Web Form. Web Forms in ASP.NET applications consist of two files. One file holds the HTML, or presentation, and has the .aspx extension. The other file, which contains the user services code, is the code-behind page. If you program in C# for the code-behind page, your page has an extension of .cs
(but if you are developing in Visual Studio .NET, the extension is aspx.cs). This code-behind page holds the code that needs to run on the Web server or application server. The language that runs in the codebehind page needs to be a compliant .NET language, such as C#. The following page directive at the top of the .aspx page associates these two pages, where WebFormName is the name of the .aspx page and ApplicationName is the name of the virtual directory:
<%@ Page language="c#"
Codebehind=" WebFormName.aspx.cs"
AutoEventWireup="false"
Inherits="ApplicationName.WebFormName" %>
The code in a code-behind page follows objectoriented programming (OOP) concepts, where code is implemented within an event handler and the code within this handler accesses objects through their properties, fields, and methods. These objects can be elements on the Web Form or class libraries. In ASP code, programmers are responsible for all of this, except the event handling is not there. With the absence of event handling, the style of server-side ASP was procedural coding versus OOP.
In current ASP development, you are limited to VBScript and JScript for server-side code. Using these scripting languages for server-side code has its limitations (such as error handling, data types, and event handling). Having first-class languages such as VB and C#, as the server-side code for an .aspx page yields more programming power and better structured code. To interact with the .aspx page, you can inherite the Page class of the System.Web.UI namespace on the codebehind page. The Page class exposes some familiar objects that are common in ASP development today (such as Response, Request, Application,
Session, and Server) and implements some common events that VB programmers are accustomed to using (such as Page_Load, Page_Unload, and Page_Init).
Web Forms
Web Forms are the primary file type used in ASP.NET to create Web pages. Web Forms have an extension of .aspx. These pages are the next generation pages to ASP pages that are created in ASP applications. Web Forms are more
sophisticated than the earlier asp pages found in ASP applications. Web Forms offer new capabilities such as separation of code from presentaion, the availability of a vast array of controls that are provided by the framework, and the capability of creating your own controls.
217