
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers
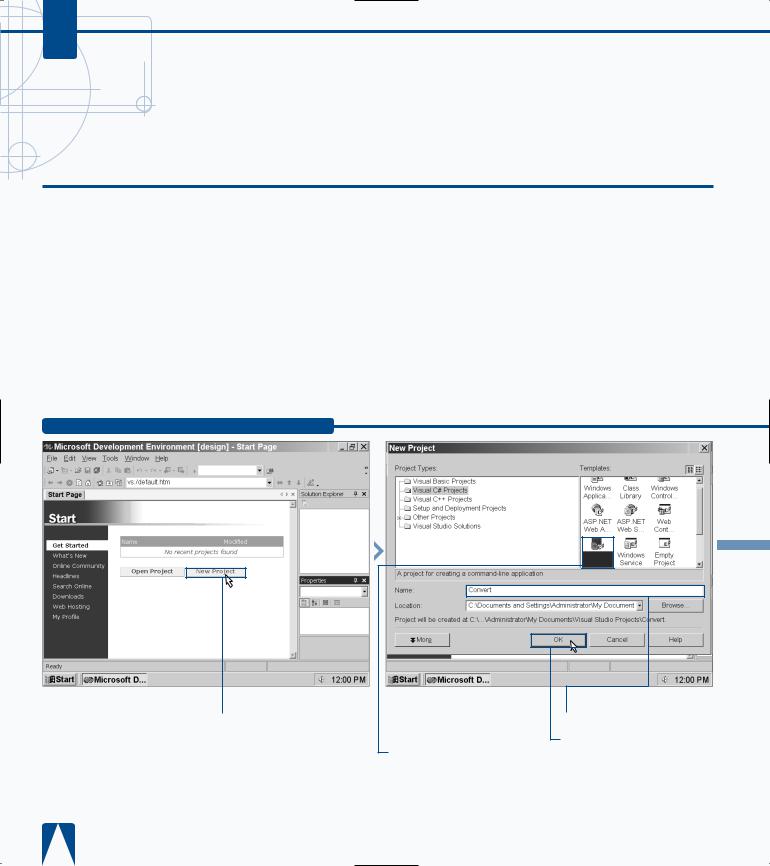
C#
CONVERT VALUE TYPES TO REFERENCE TYPES
isual C# enables you to convert value types to Vreference types and vice versa with a process called
boxing. Boxing refers to the value type to reference type conversion process. Unboxing is the reverse procedure that converts reference types to value types.
Visual C# boxes value types, including struct and built-in value types, by copying the value from the value type into the object. After you box the value type, you can change the value of that value type. Boxing is useful when you need to copy a value from one value type to one or more value types. For example, you can copy an integer value to one or
more integers by having those other integers reference the object you created when you boxed the integer value.
Unboxing lets you convert an object into a value type or an interface type into a value type that implements that interface. When Visual C# unboxes the object, it checks the object to see if it is the same value type as the one you specify in the unboxing argument. If Visual C# sees that this is true, it unboxes the object value and places it into the value type.
CONVERT VALUE TYPES TO REFERENCE TYPES
Console
Applicatio
■ The New Project window appears.
‹ Click the Console Application icon in the Templates pane.
› Type a name for the file.
ˇ Click OK.

WORKING WITH TYPES AND INTERFACES 5
You can unbox an object with a boxed object value. For example, if you see an object statement with a = 5, you want to move the number 5 from the object to an integer, or else the compiler will return an error. You can test whether an object value has been boxed correctly using the try and catch arguments.
TYPE THIS:
using System; public class Unbox
{
int a = 5;
object x = a // boxes a into object x try
{
int b = (int) x;
Console.WriteLine("The integer unboxed successfully.");
}
catch (InvalidCastException e) // If there is an error, the catch argument catches it.
{
Console.WriteLine("{0} Unboxing error!",e);
}
}
RESULT:
The integer unboxed successfully.
■
Á

C#
PROGRAM POINTER TYPES
hen you compile a project, the Visual Studio .NET Wgarbage collector manages all objects in your class
and ensures that all objects handle memory correctly and have legitimate references. However, there may be times when you need to have an object access a particular memory address that you do not want the garbage collector to touch. Visual Studio .NET gives you this control with unsafe mode and pointers.
When you enter the unsafe keyword in code, you tell the compiler and the Visual Studio .NET runtime environment (the Common Language Runtime) that the garbage collector should not manage those memory blocks that have been allocated in the unsafe argument. You point to the memory blocks to reserve by using the pointer type.
The key portion of your unsafe code block is the fixed pointer type. The fixed pointer type pins down the memory you want to reference so the garbage collector will not allocate that memory block at random to other objects in your program.
Note that if you try to create pointer types and do not explicitly create the unsafe context in your code, the pointers will be considered invalid. In that case the MDE window will alert you to this error, and if you try to compile your project, the compiler will return an error.
PROGRAM POINTER TYPES
Console
Applicatio
Properties
¤ Click New Project. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
■ The New Project window |
|
|
|
|
|
||||
|
|
|
|
|
|||||
› Type a name for the file. |
|||||||||
|
appears. |
|
ˇ Click OK. |
||||||
|
|
‹ Click the Console |
|
||||||
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
|
|||
|
Application icon in the |
|
|
|
|
|
|||
|
Templates pane. |
|
|
|
|
|
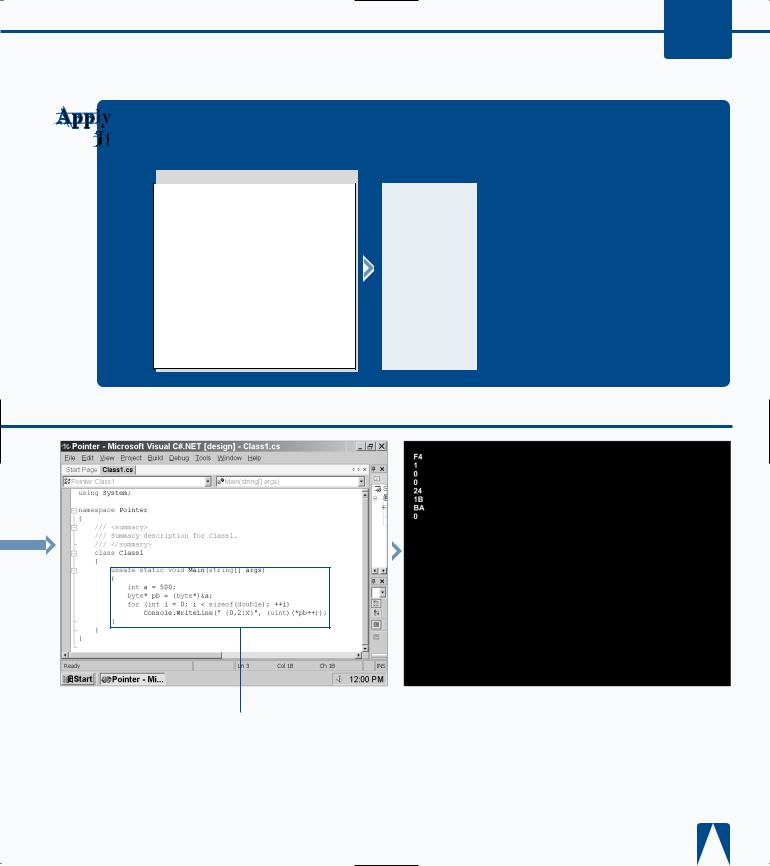
WORKING WITH TYPES AND INTERFACES 5
You can initialize pointers of different types by nesting fixed statements within each other. This approach saves time when you need to declare several different pointer types.
TYPE THIS: |
|
RESULT: |
using System; |
2 |
|
class Pointer |
4 |
|
{ |
6 |
|
int x, y; |
|
|
unsafe static void Main() |
|
|
{ |
|
|
Pointer test = new Pointer(); |
|
|
Fixed(int* p1 = &test.x) |
|
|
Fixed (int* p2 = &test.y) |
|
|
*p1 = 2; |
|
|
*p2 = 4; |
|
|
Console.WriteLine(test.x); |
|
|
Console.WriteLine(test.y); |
|
|
} |
|
|
If you receive an error running unsafe code you have not told the compiler to compile unsafe code. You can do so by selecting the project name in the Solution Explorer window and pressing Shift+F4 on your keyboard. When the Property Pages window appears, you can click the Configuration Properties file folder in the left-pane and then change the Allow unsafe code blocks setting to True.
}
■ The appears window
Á Delete within