
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers

C#
TRIM SPACES
When you have a string that contains unwanted white space in the beginning or end of a string, you can resolve this with using the trimming
capabilities built into the String class. Visual C# provides three options for creating a copy of your string and trimming the white spaces from them: String.Trim,
String.TrimEnd, and String.TrimStart.
The String.Trim, String.TrimEnd, and
String.TrimStart methods determine what white space is and what is not by using the Char.IsWhiteSpace method. The Char.IsWhiteSpace method indicates whether the specified Unicode character is categorized as white space.
The String.Trim method trims both ends of the string. If only the end or beginning white space characters need to be trimmed, you can use the String.TrimEnd and String.TrimStart methods respectively. These trim methods also have overloaded implementations that allow specified character(s) to be included as nondesirable characters to be trimmed off of the extremes of the string. To include additional characters, you would provide an array of characters in the parameter list of the desired trim method. This character list does not have to be specified in any order. It uses all members of the array as it trims characters off of the string.
TRIM SPACES
console |
‹ Rename the class name to |
the |
TrimSpaces. |
namespace to |
› Save the file. |
|
ˇ Add the Main function.
Á Create a string variable and initialize with a welcome message with a space at the beginning and end of the string.
‡ Create a string variable that is initialized with a name.
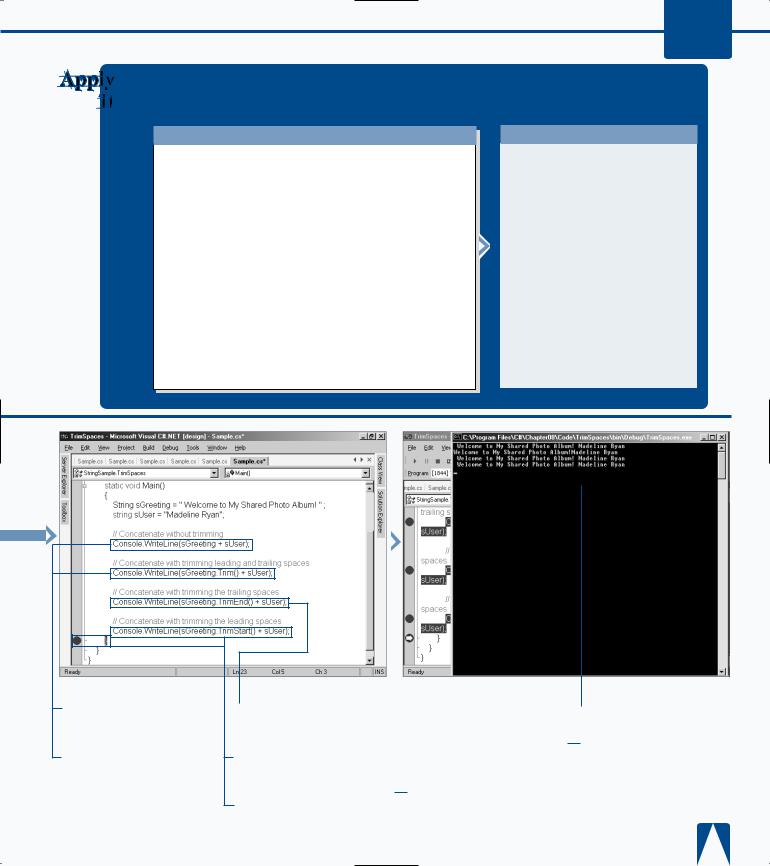
WORKING WITH STRINGS 8
Trimming strings for more than white space can enable you to strip any other unwanted characters like punctuation.
TYPE THIS:
using System;
namespace StringSample
{
///<summary>
///The Trim can accept an array of unicode chars that
///will be used to trim from either end of the string.
///Note that it does not matter what order the chars
///are set.
///</summary>
class TrimSpaces
{
static void Main()
{
String sGreeting =
" Welcome to My Shared Photo Album! " ;
Console.Write(sGreeting.Trim( char.Parse(" "), char.Parse("!")));
}
}
}
RESULT:
C:\>csc TrimSpaces_ai.cs
C:\> TrimSpaces_ai.exe
Welcome to My Shared Photo Album
C:\>
° Concatenate the message without trimming and output to the console.
· Trim the leading and trailing spaces, concatenate the message, and output to the console.
‚ Trim the trailing spaces, concatenate the message, and output to the console.
— Trim the leading spaces, concatenate the message, and output to the console.
± Set a debug stop.
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
¡ Press F5 to save, build, |
|
|
|
|
||
|
■ The message appears |
||||||
|
and run the console |
without a trailing space. |
|||||
|
application. |
■ The message appears |
|||||
|
|
|
|
||||
|
|
■ The message appears with |
without a leading space. |
||||
|
|
||||||
|
leading and trailing spaces. |
|
|
|
|
||
|
■ The message appears with |
|
|
|
|
||
|
|
|
|
|
|||
|
neither leading nor trailing |
|
|
|
|
||
|
spaces. |
|
|
|
|
175
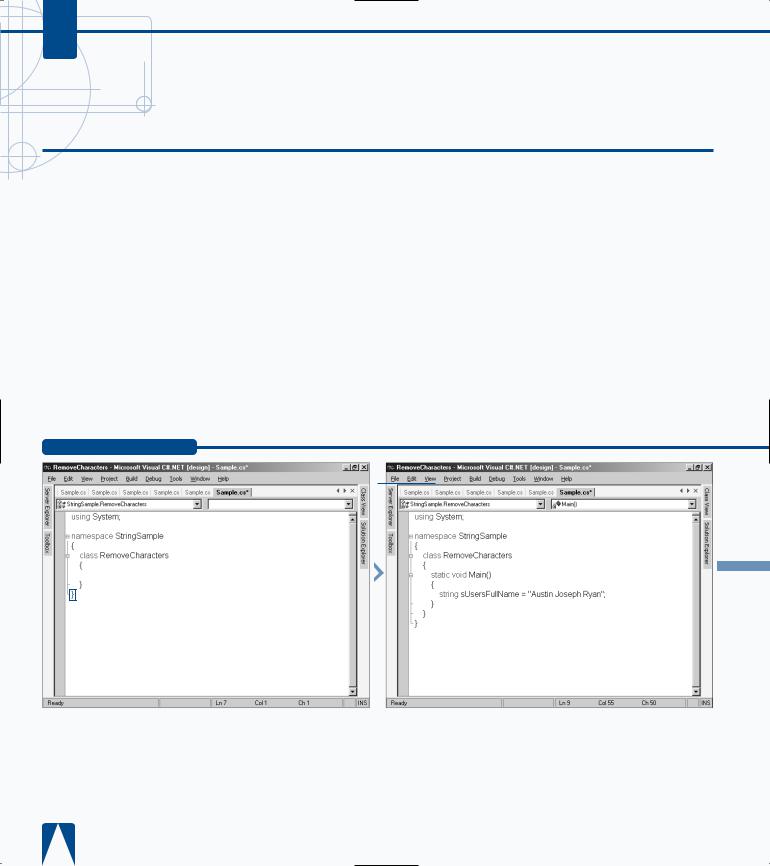
C#
REMOVE CHARACTERS
You can clean up your string of unwanted characters with members of the .NET Framework String class. The String class contains members that can remove
unwanted characters from the extremes of a string or throughout the string.
To trim characters from the extremes (start and end), you can refer to the “Trim Spaces” task in this chapter. To remove characters from anywhere in the string, you can use the String.Remove method. The String.Remove method requires two parameters for execution. The first parameter, startIndex, is an integer that indicates the
starting position for deleting characters. The second parameter, count, is an integer that indicates the number of characters to delete from the startIndex.
When using the Remove method, you will most likely determine the startIndex and count programmatically.
For example, you may know a list of characters that are in data file from a mainframe. You can search for the characters in the string for their position. You can use the
IndexOf and LastIndexOf methods of the String class to see what index position contains that character. This position can be used as the startIndex, and you can give a count of 1.
REMOVE CHARACTERS
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
‹ Rename the class name to |
|
ˇ Add the Main function. |
|
|
|
||||
|
|
|
|
|
|
Á Create a string variable |
||||||||||
|
|
|
|
|
|
|
|
|||||||||
|
|
|
|
|
|
RemoveCharacters. |
that is initialized with a full |
|||||||||
|
|
|
|
|
|
› Save the file. |
name including the first, |
|||||||||
|
|
|
|
|
|
middle, and last name. |
||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
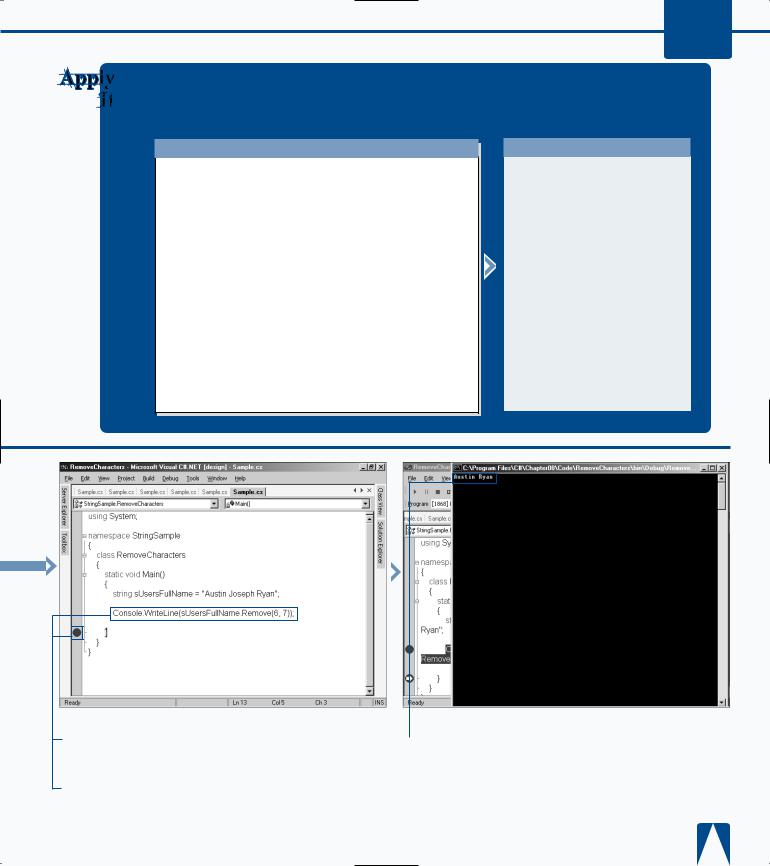
WORKING WITH STRINGS 8
This example takes this section’s example one step further and programitically determines the values for the startIndex and count parameters.
TYPE THIS:
using System;
namespace StringSample
{
class RemoveCharacters
{
static void Main(string[] args)
{
string sUsersFullName = "Austin Joseph Ryan";
string[] sNameParts = sUsersFullName.Split( char.Parse(" "));
if (sNameParts.GetUpperBound(0)==2)
{
string sShortName = sUsersFullName.Remove(sNameParts[0].Length,
sNameParts[1].Length + 1); Console.WriteLine(sShortName);
}
}
}
}
RESULT:
C:\>csc RemoveCharacters_ai.cs
C:\> RemoveCharacters_ai.exe
Austin Ryan
C:\>
‡ Use the Remove function to remove the middle name from the string.
° Set a debug stop.
· Press F5 to save, build, and run the console application.
■ A message appears with just the first and last name.
177