
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers

C#
SAVE XML TO A FILE
You will sometimes need to persist data as XML. In ADO.NET, the persistence mechanism for DataSets is XML. XML provides an excellent way to save and
retrieve data without a database server.
One of the fastest ways to write data is by using the
XMLTextWriter class that is part of the System.Xml namespace. This writer provides a fast, forward-only way of generating XML and helps you to build XML documents that conform to the W3C Extensible Markup Language (XML) 1.0 and the Namespaces in XML specifications. You can find the latest XML specification at www.w3c.org.
The XMLTextWriter is an implementation of the XMLWriter abstract class. You can write your own
implementation of this abstract class, but if the XMLTextWriter has what you need, you use this .NET Framework class. Typically, you use an XMLTextWriter if you need to quickly write XML to file, stream, or a TextWriter, and do not need to use the Document
Object Model (DOM).
The XMLTextWriter has formatting capabilities to assist in giving a file with nice indentions that are handy when reading the documents in a text viewer. When you construct your XML, you use one of several Write methods, depending on what part of the XML document you are constructing (element, attribute, or comment).
SAVE XML TO A FILE
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
‹ Rename the namespace |
|
Á Create the Main function. |
|||||||||||
|
|
|
|
|
|
|
|
|
||||||||||||||
|
|
|
|
|
|
|
|
|
to XMLSamples. |
|
‡ Create an |
|||||||||||
|
|
|
|
|
|
|
|
|
› Rename the class name |
|
||||||||||||
|
|
|
|
|
|
|
|
|
XmlTextWriter variable |
|||||||||||||
|
|
|
|
|
|
|
|
|
||||||||||||||
|
|
|
|
|
|
|
|
|
to WriteXML. |
and initialize the variable |
||||||||||||
|
|
|
|
|
|
|
|
|
||||||||||||||
|
|
|
|
|
|
|
|
|
|
ˇ Save the file. |
to null. |
|||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
° Set the XmlTextWriter variable equal to a new
XmlTextWriter, using the location of the XML file.
· Begin the XML document using the
WriteStartDocument method.

USING THE XML FRAMEWORK CLASS 13
You can use verbatim strings to handcraft XML and set the indention in your code. Remember that you will have to double up your quotes inside of the string.
TYPE THIS:
using System; using System.IO; using System.Xml; public class Sample
{public static void Main()
{ XmlDocument doc = new XmlDocument();
string sXML =
@""<?xml version=""1.0"" standalone=""no""?> <!—This file represents a list of favorite photos—> <photofavorites owner=""Frank Ryan"">
<photo cat=""vacation"" date=""2000""> <title>Maddie with Minnie</title>
</photo>
</photofavorites>"; // end of string doc.LoadXml(sXML); doc.Save("data.xml");
}}
RESULT:
XML document created in the internals of the class and echoed out to the console.
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
‚ Begin an element using |
|
|
¡ End the XML document |
|||||
|
|
|
|||||||
|
the WriteStartElement |
by adding the Flush and |
|||||||
|
method. |
Close methods. |
|||||||
|
— Add an attribute to |
™ Press F5 to save, build, |
|||||||
|
|||||||||
|
the element. |
and run the console |
|||||||
|
|
± End the element using the |
application. |
||||||
|
|
|
|
|
|
|
|||
|
WriteEndElement |
|
|
|
|
|
|||
|
method. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
£ Open the XML file that |
|
■ The XML file has the |
|
|
|
|||
|
was created, which is located |
|
elements and |
|
|
in the bin\Debug directory. |
|
attributes created. |
247

C#
QUERY XML WITH XPATH
XML is great for portable data. If you want a quick way to query XML documents for pieces of data relevant to your application, XPath is a high-performance
mechanism to get this done. XPath is specified by W3C and is a general query language specification for extracting information from XML documents. XPath functionality has its own namespace in the .NET Framework. The System.Xml.XPath namespace has four classes that work together to provide efficient XML data searches.
The classes provided by System.Xml.XPath are:
XPathDocument, XPathExpression, XPathNavigator, and XPathNodeIterator. XPathDocument is used to cache your XML document in a high-performance oriented cache for XSLT processing. To query this cache, you will
need an XPath expression. This can be done with just a string that contains an XPath expression or you can use the XPathExpression class. If you want performance, you will use the XPathExpression class because it
compiles once and can be rerun without requiring subsequent compiles. The XPath expression is provided to a select method on the XPathNavigator class. The XPathNavigator object will return an XPathNodeIterator object from executing the Select method. After calling this method, the XPathNodeIterator returned represents the set
of selected nodes. You can use MoveNext on the XPathNodeIterator to walk the selected node set.
QUERY XML WITH XPATH
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
‹ Rename the namespace |
|
Á Create the Main function. |
||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|||||||||
|
|
|
|
|
|
|
|
|
to XMLSamples. |
‡ Create a new |
||||||||||
|
|
|
|
|
|
|
|
|
|
|
› Rename the class name |
|||||||||
|
|
|
|
|
|
|
|
|
|
XPathDocument using |
||||||||||
|
|
|
|
|
|
|
|
|
|
|||||||||||
|
|
|
|
|
|
|
|
|
to XMLwithXPath. |
the location of the |
||||||||||
|
|
|
|
|
|
|
|
|
||||||||||||
|
|
|
|
|
|
|
|
|
|
ˇ Save the file. |
XML document. |
|||||||||
|
|
|
|
|
|
|
|
|
|
|
° Create a new |
|||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
||||||||
|
|
|
|
|
|
|
|
|
|
|
|
XPathNavigator using the |
||||||||
|
|
|
|
|
|
|
|
|
|
|
|
XPathDocument created. |
· Create an
XPathNodeIterator variable that will contain the result of running an XPath query that returns all of the photo/title elements.
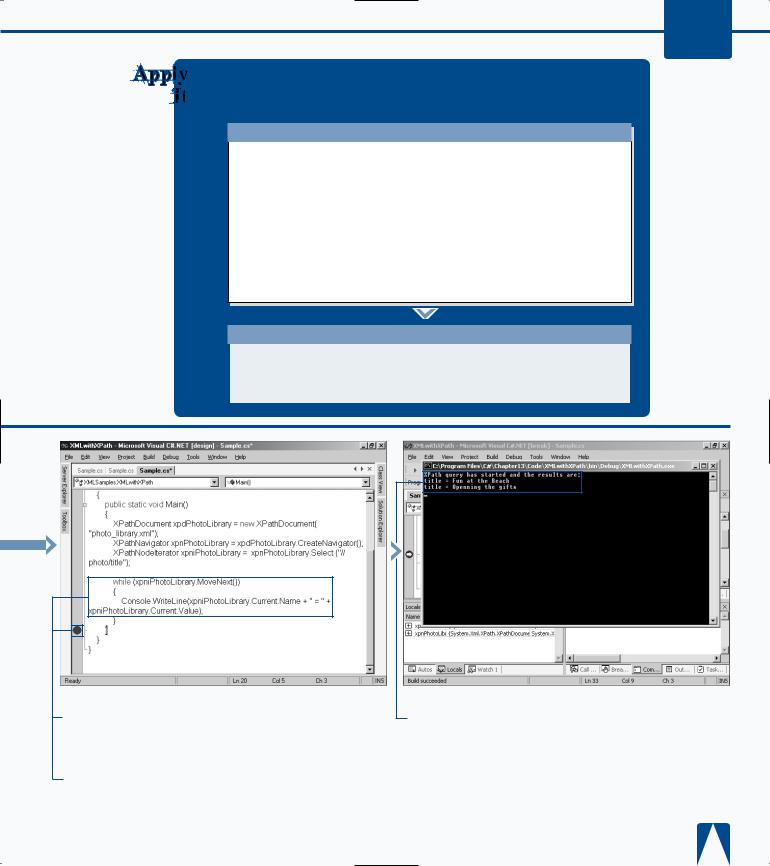
USING THE XML FRAMEWORK CLASS 13
You can use the recursive decent operator to search for an element at any depth. Make sure that the source XML document, photo_library.xml, is in the working directory of the EXE file.
TYPE THIS:
using System; using System.IO; using System.Xml; using System.Xml.XPath; namespace XMLSamples {
public class XMLwithXPath {
private const String sXMLDocument = "photo_library.xml"; public static void Main() {
Console.WriteLine ("XPath query results are:");
XPathDocument xpdPhotoLibrary = new XPathDocument(sXMLDocument); XPathNavigator xpnPhotoLibrary = xpdPhotoLibrary.CreateNavigator(); XPathNodeIterator xpniPhotoLibrary =
xpnPhotoLibrary.Select ("//photo/title"); while (xpniPhotoLibrary.MoveNext())
Console.WriteLine(xpniPhotoLibrary.Current.Name + " = " + xpniPhotoLibrary.Current.Value);
}}}
RESULT:
XPath query results are:
title = Fun at the Beach
title = Opening the gifts
‚ Add a while loop to output the name and the value of the node to
the console.
— Set a debug stop.
± Press F5 to save, build, and run the console application.
■ A message appears that shows the name and the value for the two elements that match the XPath query.
249