
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers

C#
SEARCH FOR SUBSTRINGS
When working with filenames that are embedded in a fully qualified file path, it is helpful to have substring searching capabilities. Different parts of
that fully qualified path can be useful to your program. For example, you may want to check for the file extension or maybe for a certain directory path. The String class provides several methods that assist you in this process.
One way to search for substrings is to use comparision methods. Comparison methods that work with substrings are StartsWith and EndsWith, which essentially do what the method name indicates (that is, find substrings that start a string and finish off a string). These methods yield a Boolean response that indicates if the substring was found.
If you are just looking for a specific character, you can use the IndexOf and LastIndexOf method of the String class to see what index position contains that character.
Another useful way to find substrings is to use regular expressions. This is a more sophisticated way to determine if a substring exists. With regular expressions, you can go further than substrings and look for patterns that exist in the string.
Another handy string-comparison method is the EndsWith method. You can use EndsWith to identify the extension of a file and determine if code should run or not.
SEARCH FOR SUBSTRINGS
|
|
|
|
|
|
|
‹ Rename the class name to |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
ˇ Add the Main function. Á Create a string array of |
|||||||
|
|
|
|
|
|
|
|
|||||||
|
|
|
|
|
|
Search. |
|
|
|
|
size 3 and initialize the first |
|||
|
|
|
|
|
|
› Save the file. |
|
|
|
|
element in the array with a |
|||
|
|
|
|
|
|
|
|
|
|
filename and the second two |
||||
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
|
|
|
|
|
|
|
|
|
|
elements with image |
||
|
|
|
|
|
|
|
|
|
|
|
|
filenames. |

WORKING WITH STRINGS 8
Regular expressions are a very powerful way to find substrings or patterns in a string. If you are trying to accomplish a complicated search of a string, use regular expressions.
TYPE THIS:
using System;
using System.Text.RegularExpressions; namespace StringSample
{
class Search
{
static void Main()
{
string[] sFileNames = new string[3] { "allphotos.aspx", "lri_familyreunion_jan2001_001.jpg", "hri_familyreunion_jan2001_001.jpg"};
Regex rePictureFile = new Regex(".jpg");
foreach (string sFileName in sFileNames)
{
if (rePictureFile.Match(sFileName).Success) Console.WriteLine("{0} is a photo file.",
sFileName);
}
}
}
}
RESULT:
C:\>csc SearchSubStrings_ai.cs
C:\> SearchSubStrings_ai.exe
lri_familyreunion_jan2001_001.jpg is a photo file.
hri_familyreunion_jan2001_001.jpg is a photo file.
C:\>
‡ Use a foreach statement to loop through all the elements in the array.
° In the foreach loop, use the EndsWith function to check the element for a .jpg extension and write the filename to the console if it is a JPEG file.
· Set a debug stop.
‚ Press F5 to save, build, and run the console application.
■ A message appears displaying the two JPEG filenames.
167
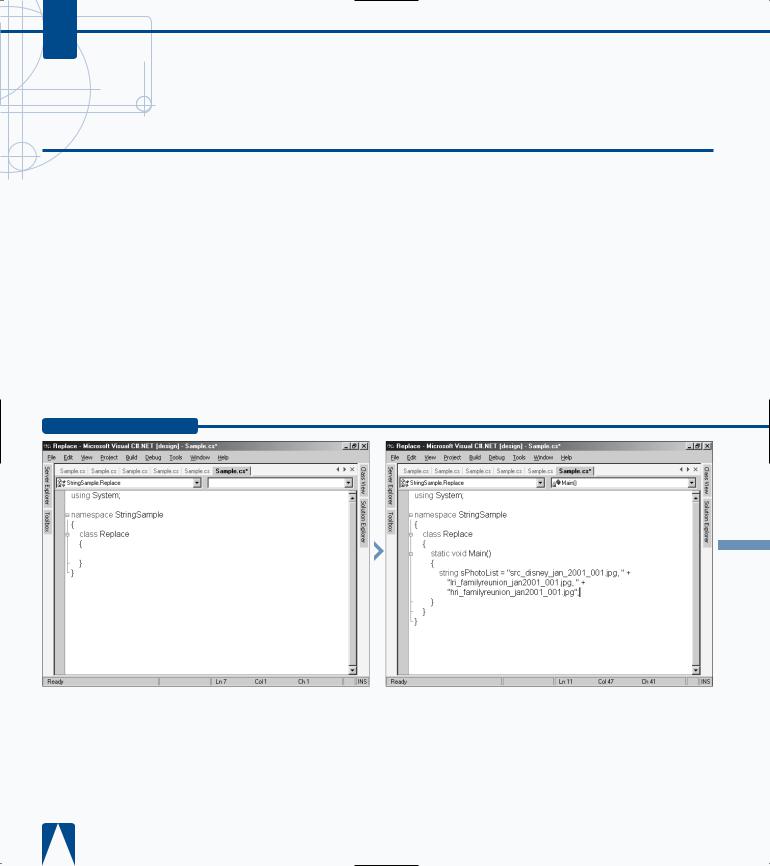
C#
REPLACE CHARACTERS
If you need to create a string from replacing characters in an existing string, you can use either the String or StringBuilder classes to perform this operation. For example, you may want to take a comma-separated file and
turn it into a tab-separated file.
On the String class, the Replace method lets you replace a character in one string with another character. When you use the String.Replace method, it will search for all instances of the character in the affected string and replace it with the character you specify. If you do not intend to remove more than one of the same character from your string, your best course of action is to replace your original
string using the StringBuilder class discussed earlier in this chapter.
The StringBuilder.Replace method is much more flexible with replacing characters within a string. The
StringBuilder.Replace method gives you four methods for replacing characters: replacing a character string with a new one, replacing all instances of a specified character with another one (just like the String.Replace method), replacing all instances of a string within a specified range, and replacing all instances of a character in a specified range with a new character.
REPLACE CHARACTERS
|
|
|
|
|
|
|
|
‹ Rename the class name to |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
ˇ Add the Main function. Á Create a string and |
||||||||
|
|
|
|
|
|
|
|
||||||||
|
|
|
|
|
|
|
Replace. |
|
|
|
|
initialize the string with three |
|||
|
|
|
|
|
|
|
› Save the file. |
|
|
|
|
image filenames separated by |
|||
|
|
|
|
|
|
|
|
|
|
|
commas. |
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
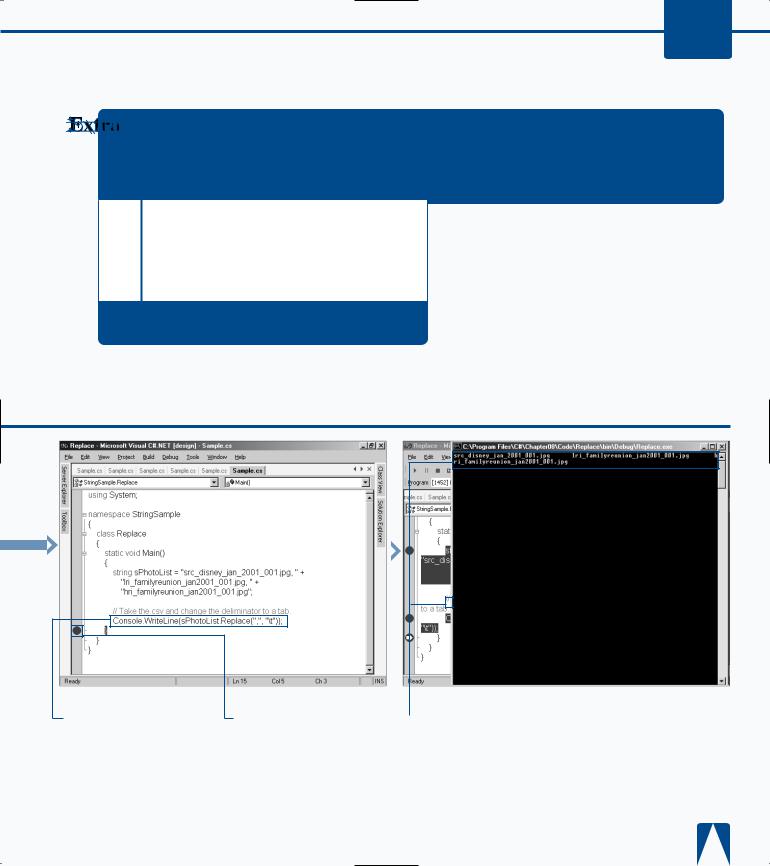
WORKING WITH STRINGS 8
The String.Replace method is rather straightforward, but it is also quite limited just like the String class it references. If you have a StringBuilder class, then you can use the
StringBuilder.Replace method for changing your original String class.
The String.Replace and StringBuilder. Replace methods are both case sensitive, so if you try to replace a character with the lowercase letter t, then Replace function will leave all uppercase T characters alone. If you want to search for all uppercase T characters, then you have to include another String.Replace or String.Builder.Replace method that searches for an uppercase T.
Visual C# returns an ArgumentNullException exception if the character that you are trying to replace is a null value — the character you are trying to find does not exist or if the string has no characters in it at all.
‡ Use the Replace function to replace the commas with tabs and write the result to the console.
° Set a debug stop.
· Press F5 to save, build, and run the console application.
■ A message appears with tabs separating the image filenames.
169