
- •maranGraphics
- •CREDITS
- •ACKNOWLEDGMENTS
- •ABOUT THE AUTHORS
- •AUTHORS’ ACKNOWLEDGMENTS
- •TABLE OF CONTENTS
- •HOW TO USE THIS BOOK
- •INTRODUCTION TO C#
- •START VISUAL STUDIO .NET
- •OPEN A NEW C# PROJECT
- •OPEN A C# WEB PROJECT
- •SET JSCRIPT .NET AS THE DEFAULT SCRIPT LANGUAGE
- •EXPLORE THE CLASS VIEW WINDOW
- •VIEW THE CONTENTS WINDOW
- •GET HELP USING THE INDEX WINDOW
- •SEARCH FOR HELP
- •ADD COMPONENTS FROM THE TOOLBOX
- •ADD A TASK TO THE TASK LIST
- •CHANGE FORM PROPERTIES IN THE PROPERTIES WINDOW
- •ADD A CUSTOM TOOLBAR
- •DELETE A TOOLBAR
- •CHANGE THE VISUAL STUDIO ENVIRONMENT
- •MANAGE OPEN WINDOWS
- •OPEN A PROJECT
- •VIEW THE MAIN METHOD
- •COMBINE PROGRAM TYPES
- •ADD REFERENCE TYPES
- •ADD OPERATORS
- •INSERT ATTRIBUTES
- •ENTER CLASSES
- •ADD COMMENTS TO CODE
- •WRITE YOUR FIRST PROGRAM
- •ENTER XML DOCUMENTATION
- •ACCESS DOCUMENTATION
- •LOG A BUG REPORT
- •VIEW INFORMATION ABOUT C# BUILDING BLOCKS
- •PROGRAM CLASSES
- •ADD A CLASS
- •EMPLOY CLASS INHERITANCE
- •PROGRAM INSTANCE CONSTRUCTORS
- •INSERT DESTRUCTORS
- •PROGRAM STRUCTS
- •DISPLAY HEAP AND STACK INFORMATION
- •FIND TYPE INFORMATION
- •PROGRAM CONSTANT EXPRESSIONS
- •SPECIFY VALUE TYPES
- •PROGRAM NUMERIC TYPES
- •PROGRAM THE BOOLEAN TYPE
- •DECLARE REFERENCE TYPES
- •ENTER REFERENCE TYPE DECLARATIONS
- •CONVERT VALUE TYPES TO REFERENCE TYPES
- •PROGRAM POINTER TYPES
- •INSERT THE VOID TYPE
- •ADD INTERFACE PROPERTIES
- •ADD AN INTERFACE INDEX
- •VIEW INFORMATION ABOUT METHODS
- •ADD A METHOD
- •ADD STATIC METHODS
- •INCLUDE NON-STATIC METHODS
- •ENTER DELEGATES
- •PROGRAM EVENTS
- •ADD AN EVENT-HANDLING METHOD
- •VIEW INFORMATION ABOUT ARRAYS
- •ENTER SINGLE-DIMENSIONAL ARRAYS
- •ADD MULTIDIMENSIONAL ARRAYS
- •PROGRAM ARRAY-OF-ARRAYS
- •ITERATE THROUGH ARRAY ELEMENTS
- •SORT ARRAYS
- •SEARCH ARRAYS
- •IMPLEMENT A COLLECTIONS CLASS
- •PROGRAM STRUCTS
- •ADD AN INDEXER
- •INCLUDE ENUMERATIONS
- •CREATE STRING LITERALS AND VARIABLES
- •ASSIGN VALUES TO STRINGS
- •CONCATENATE STRINGS
- •COMPARE STRINGS
- •SEARCH FOR SUBSTRINGS
- •REPLACE CHARACTERS
- •EXTRACT SUBSTRINGS
- •CHANGE THE CHARACTER CASE
- •TRIM SPACES
- •REMOVE CHARACTERS
- •SPLIT A STRING
- •JOIN STRINGS
- •PAD STRINGS
- •VIEW INFORMATION ABOUT PROPERTIES
- •COMPARE PROPERTIES AND INDEXERS
- •PROGRAM PROPERTY ACCESSORS
- •DECLARE ABSTRACT PROPERTIES
- •INCLUDE PROPERTIES ON INTERFACES
- •VIEW INFORMATION ABOUT WINDOWS FORMS
- •ADD A WINDOWS FORM IN THE WINDOWS FORM DESIGNER
- •SET THE FORM TYPE
- •CHOOSE THE STARTUP WINDOWS FORM
- •CREATE A MODAL FORM
- •LAYOUT A FORM
- •SET A FORM LOCATION
- •CHANGE FORM PROPERTIES
- •CREATE A TRANSPARENT FORM
- •AN INTRODUCTION TO WEB FORMS AND CONTROLS
- •CREATE AN ASP.NET WEB SITE
- •CREATE A WEB FORM
- •ADD SERVER CONTROLS TO A WEB FORM
- •READ AND CHANGE PROPERTIES FROM OBJECTS ON A WEB FORM
- •USING SERVER-SIDE COMPONENTS ON WEB FORMS
- •INTRODUCING DATA ACCESS WITH ADO.NET
- •DISPLAY DATA WITH THE DATAGRID CONTROL
- •CONFIGURE THE DATAGRID CONTROL
- •INSERT DATA INTO A SQL DATABASE
- •UPDATE DATA FROM A SQL DATABASE
- •DELETE DATA FROM A SQL DATABASE
- •EXECUTE A STORED PROCEDURE IN A SQL DATABASE
- •READ XML FROM A FILE
- •SAVE XML TO A FILE
- •QUERY XML WITH XPATH
- •APPLY XSL TO XML
- •INTRODUCTION TO DISTRIBUTED APPLICATIONS
- •CREATE AN APPLICATION WITH PRIVATE ASSEMBLIES
- •CREATE AN APPLICATION WITH SHARED ASSEMBLIES
- •VERSION A SHARED ASSEMBLY
- •CONFIGURE A CLIENT FOR A VERSIONED ASSEMBLY
- •CREATE A WEB SERVICE
- •USING A WEB SERVICE
- •INTRODUCTION TO EXCEPTION HANDLING
- •THROWING AN EXCEPTION
- •HANDLING EXCEPTIONS WITH THE CATCH BLOCK
- •USING THE FINALLY BLOCK
- •WRITE ERRORS TO THE APPLICATION LOG
- •BASIC EXAMPLES
- •WHAT’S ON THE CD-ROM
- •USING THE E-VERSION OF THIS BOOK
- •INDEX
- •Symbols & Numbers

C#
INTRODUCING DATA ACCESS WITH ADO.NET
ost production-grade applications need some form Mof data access. Data access in the .NET Framework
is simplified for you through the ADO.NET Framework classes. These classes are found in System.Data namespace, which has two major namespaces: one for SQL Server data stores and another for data stores that can be accessed through OLE DB.
The SQL Server .NET Data Provider classes come from the System.Data.SqlClient namespace. The SQL Server
.NET Data Provider uses its own protocol to communicate with SQL Server. The provider is lightweight and performs well, accessing a SQL Server data source directly without adding an OLE DB or Open Database Connectivity (ODBC) layer. When you need to work with other database besides Microsoft SQL Server, you should use the OLE DB .NET Data Provider, which you can find in the
System.Data.OleDb namespace.
If you are familiar with ADO, you may notice some similaries when accessing data in C# with ADO.NET. The Connection and Command objects, for example, have almost identical properties and methods. The brand new part in ADO.NET is in the area of reading and persisting records of data. In the days of ADO, Recordsets transported returned data from a SQL database; however, in ADO.NET, the Recordset is gone, replaced by things like the DataSet,
DataReader, DataTables, and DataViews.
To orient you to ADO.NET’s new object model, these pages outline a few key members of the ADO.NET classes (System.Data namespace). Because both the
System.Data.SqlClient and System.Data.OleDb implement most of the same base classes, the examples reflect the perspective of only one of the providers,
SqlClient.
CONNECTION
Connections are the starting point to your data access and determine how you connect to the data store. You need to set properties, like ConnectionString, to establish communications to your data store.
SQLCONNECTION KEY PROPERTIES AND METHODS
PROPERTY |
DESCRIPTION |
|
|
ConnectionString |
(read/write) string used to open a SQL Server database |
|
|
ConnectionTimeout |
(read) maximum time allowed for a connection attempt |
|
|
Database |
(read) name of the current (or soon to be) connected database |
|
|
DataSource |
(read) name of SQL Server instance to connect to |
|
|
ServerVersion |
(read) string that identifies version of the connected SQL Server instance |
|
|
State |
(read) current state of the connection |
METHOD |
DESCRIPTION |
|
|
BeginTransaction |
(overloaded) begins a database transaction |
|
|
ChangeDatabase |
changes the current database for an open SqlConnection |
|
|
Close |
closes the connection to the database |
|
|
CreateCommand |
creates and returns a SqlCommand object associated with the |
|
SqlConnection |
|
|
Open |
opens a database connection with the property settings specified by the |
|
ConnectionString |
230

ACCESSING DATA WITH C# AND ADO.NET 12
COMMAND
ADO.NET commands are important for stored procedures and running SQL Statements.
SQLCOMMAND KEY PROPERTIES AND METHODS
PROPERTY |
DESCRIPTION |
|
|
CommandText |
(read/write) the T-SQL statement or stored procedure to execute at the data source |
|
|
CommandTimeout |
(read/write) maximum time allowed for a command execution attempt |
|
|
CommandType |
(read/write) a value indicating how the CommandText property is to be interpreted |
|
|
Connection |
(read/write) the SqlConnection used by this instance of the SqlCommand |
|
|
Parameters |
(read) the SqlParameterCollection |
|
|
Transaction |
(read/write) the transaction in which the SqlCommand executes |
METHOD |
DESCRIPTION |
|
|
Cancel |
cancels the execution of a SqlCommand |
|
|
CreateParameter |
creates a new instance of a SqlParameter object |
|
|
ExecuteNonQuery |
executes a T-SQL statement against the connection and returns the number of |
|
rows affected |
|
|
ExecuteReader |
(overloaded) sends the CommandText to the connection and builds a SqlDataReader |
|
|
ExecuteScalar |
executes the query, and returns the first column of the first row in the resultset |
|
returned by the query |
|
|
ExecuteXmlReader |
sends the CommandText to the connection and builds an XmlReader object |
|
|
Prepare |
creates a prepared version of the command on an instance of SQL Server |
DATA ADAPTER
A DataAdapter is the object that bridges between the source data and the DataSet object so retrieve and updates can occur.
DATAADAPTER KEY PROPERTIES AND METHODS
PROPERTY |
DESCRIPTION |
|
|
AcceptChangesDuringFill |
(read/write) a value indicating whether AcceptChanges is called on a DataRow |
|
after it is added to the DataTable |
|
|
TableMappings |
(read) a collection that provides the master mapping between a source table and a |
|
DataTable |
METHOD |
DESCRIPTION |
|
|
Fill |
adds or refreshes rows in the DataSet to match those in the data source using |
|
the DataSet name, and creates a DataTable named "Table" |
|
|
FillSchema |
adds a DataTable named "Table" to the specified DataSet and configures the |
|
schema to match that in the data source based on the specified SchemaType |
|
|
GetFillParameters |
retrieves the parameters set by the user when executing a SQL select statement |
|
|
Update |
Calls the respective insert, update, or delete statements for respective action |
|
in the specified DataSet from a DataTable named "Table" |
231

C#
DISPLAY DATA WITH THE DATAGRID CONTROL
You can use the DataGrid Web Server Control to build tables containing data. One of the advantages of using the DataGrid Web Server Control is not having
to manually construct the table. Because you will bind DataGrid control to data, you do not have to programmatically loop through DataSets and other data structure types, nor write out table tags, formatting, and data field values as you hit each record in the data storage.
The process of binding to a DataGrid is quite simple. First you must retrieve a data source. Then you assign that data
source to the DataSource property of the DataGrid control. Lastly, you call the DataBind method of the
DataGrid control.
The data source for the DataGrid control will most likely be a database, but the control is not restricted to binding to only traditional database stores. For example, ADO.NET data structures can be built from other providers like Exchange, WebDav, and Active Directory. Also, any lists derived from ICollection can also be used as a data source.
DISPLAY DATA WITH THE DATAGRID CONTROL
more ■ The form's code-behind server page appears with the
Page_Load event handler.
page. › Add a SqlConnection variable and initialize with a valid connection string to your database.
ˇ Add a SqlDataAdapter variable and initialize with a valid select statement.
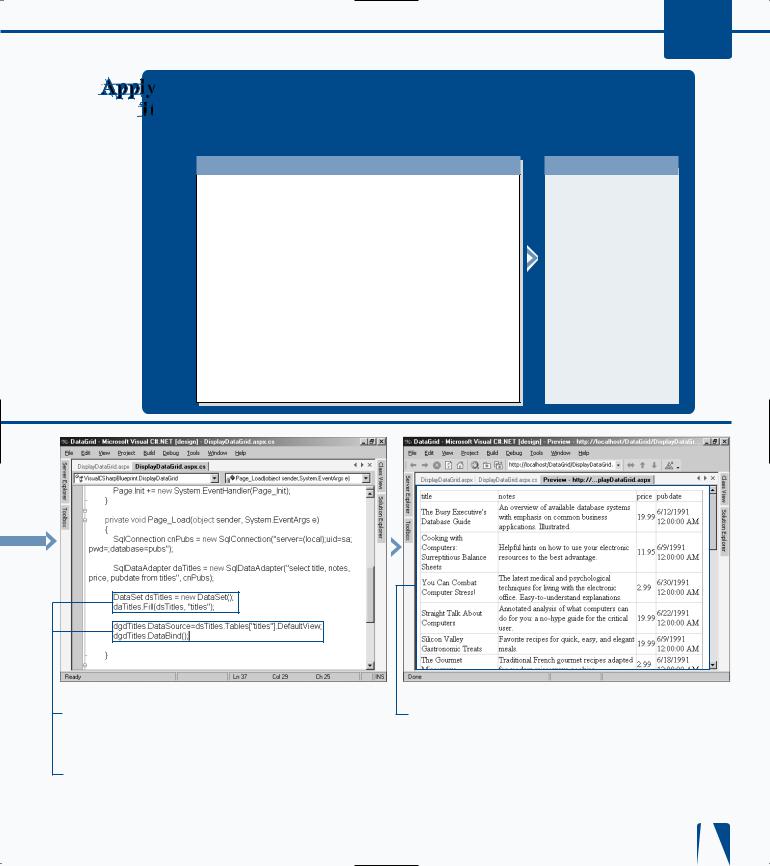
ACCESSING DATA WITH C# AND ADO.NET 12
The DataGrid Web Server Control has paging capabilities that are used to display a result into multiple navigable pages. When the page index changes, the CurrentPageIndex attribute on the DataGrid needs to be set.
TYPE THIS:
<SCRIPT language="C#" runat="server">
void Page_Load(object sender, System.EventArgs e){ if (!IsPostBack)
BindData(); }
void Grid_Change(Object sender, DataGridPageChangedEventArgs e){ dgdTitles.CurrentPageIndex = e.NewPageIndex; BindData(); }
void BindData() {
SqlConnection cnPubs = new SqlConnection( "server=(local);uid=sa;pwd=;database=pubs");
SqlDataAdapter daTitles = new SqlDataAdapter( "select title, notes, price, pubdate "
+ "from titles", cnPubs); DataSet dsTitles = new DataSet(); daTitles.Fill(dsTitles, "titles"); dgdTitles.DataSource=
dsTitles.Tables["titles"].DefaultView; dgdTitles.DataBind(); }
</SCRIPT>
RESULT:
An HTML page with an HTML table containing all rows in the titles table for the specified columns.
Á Add a DataSet variable and use the Fill method of the DataAdapter to populate the DataSet.
‡ Set the DataSource property for the data grid to the DataSet created and use the DataBind method to bind the DataGrid.
° Build and browse the Web page.
Note: See page 220 for more information on building and browsing a Web page.
■ The data returned from the select statement is displayed in the DataGrid.
233