
- •Introduction
- •Who should read this book
- •How This Book Is Organized
- •How to Use This Book
- •Where to Find the LISP Programs
- •CHAPTER 1: Introducing AutoLISP
- •Understanding the Interpreter and Evaluation
- •The Components of an Expression
- •Using Arguments and Expressions
- •Using Variables
- •Understanding Data Types
- •Integers and Real Numbers
- •Strings
- •Lists
- •File Descriptors
- •Object Names
- •Selection Sets
- •Symbols
- •Subrs
- •Atoms
- •Assigning Values to Variables with Setq
- •Preventing Evaluation of Arguments
- •Applying Variables
- •Functions for Assigning Values to Variables
- •Adding Prompts
- •CHAPTER 2: Storing and Running Programs
- •Creating an AutoLISP Program
- •What you Need
- •Creating an AutoLISP File
- •Loading an AutoLISP file
- •Running a Loaded Program
- •Understanding How a Program Works
- •Using AutoCAD Commands in AutoLISP
- •How to Create a Program
- •Local and Global Variables
- •Automatic Loading of Programs
- •Managing Large Acad.lsp files
- •Using AutoLISP in a Menu
- •Using Script Files
- •CHAPTER 3: Organizing a Program
- •Looking at a Programs Design
- •Outlining Your Programming Project
- •Using Functions
- •Adding a Function
- •Reusing Functions
- •Creating an 3D Box program
- •Creating a 3D Wedge Program
- •Making Your Code More Readable
- •Using Prettyprint
- •Using Comments
- •Using Capitals and Lower Case Letters
- •Dynamic Scoping
- •CHAPTER 4: Interacting with the Drawing Editor
- •A Sample Program Using Getdist
- •How to Get Angle Values
- •Using Getangle and Getorient
- •How to Get Text Input
- •Using Getstring
- •Using Getkword
- •How to Get Numeric Values
- •Using Getreal and Getint
- •How to Control User Input
- •Using Initget
- •Prompting for Dissimilar Variable Types
- •Using Multiple Keywords
- •How to Select Groups of Objects
- •Using Ssget
- •A Sample Program Using Ssget
- •CHAPTER 5: Making Decisions with AutoLISP
- •Making Decisions
- •How to Test for Conditions
- •Using the If function
- •How to Make Several Expressions Act like One
- •How to Test Multiple Conditions
- •Using the Cond function
- •How to Repeat parts of a Program
- •Using the While Function
- •Using the Repeat Function
- •Using Test Expressions
- •CHAPTER 6: Working With Geometry
- •How to find Angles and Distances
- •Understanding the Angle, Distance, and Polar Functions
- •Using Trigonometry to Solve a Problem
- •Gathering Information
- •Finding Points Using Trigonometry
- •Functions Useful in Geometric Transformations
- •Trans
- •Atan
- •Inters
- •CHAPTER 7: Working with Text
- •Working With String Data Types
- •Searching for Strings
- •Converting a Number to a String
- •How to read ASCII text files
- •Using a File Import Program
- •Writing ASCII Files to Disk
- •Using a Text Export Program
- •CHAPTER 8: Interacting with AutoLISP
- •Reading and Writing to the Screen
- •Reading the Cursor Dynamically
- •Writing Text to the Status and Menu Areas
- •Calling Menus from AutoLISP
- •Drawing Temporary Images on the Drawing Area
- •Using Defaults in a Program
- •Adding Default Responses to your Program
- •Dealing with Aborted Functions
- •Using the *error* Function
- •Organizing Code to Reduce Errors
- •Debugging Programs
- •Common Programming Errors
- •Using Variables as Debugging Tools
- •CHAPTER 9: Using Lists to store data
- •Getting Data from a List
- •Using Simple Lists for Data Storage
- •Evaluating Data from an Entire List at Once
- •Using Complex Lists to Store Data
- •Using Lists for Comparisons
- •Locating Elements in a List
- •Searching Through Lists
- •Finding the Properties of AutoCAD Objects
- •Using Selection Sets and Object Names
- •Understanding the structure of Property Lists
- •Changing the properties of AutoCAD objects
- •Getting an Object Name and Coordinate Together
- •CHAPTER 10: Editing AutoCAD objects
- •Editing Multiple objects
- •Improving Processing Speed
- •Using Cmdecho to Speed up Your Program
- •Improving Speed Through Direct Database Access
- •Filtering Objects for Specific Properties
- •Filtering a Selection Set
- •Selecting Objects Based on Properties
- •Accessing AutoCAD's System Tables
- •CHAPTER 11: Accessing Complex Objects
- •Accessing Polyline Vertices
- •Defining a New Polyline
- •Drawing the new Polyline
- •Testing for Polyline Types
- •How Arcs are Described in Polylines
- •Accessing Object Handles and Block Attributes
- •Using Object Handles
- •Using Object Handles
- •Extracting Attribute Data
- •Appendix A: Menu Primer
- •Appendix B: Error Messages
- •Appendix C: Group Codes
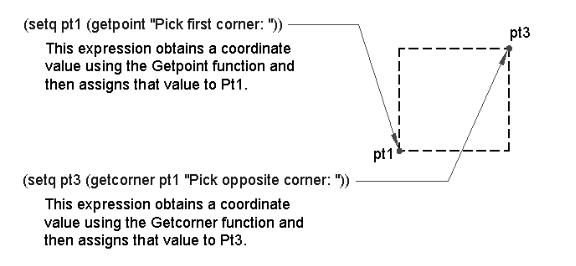
The ABC’s of AutoLISP by George Omura
Understanding How a Program Works
Up until now, you have been dealing with very simple AutoLISP expressions that perform simple tasks such as adding or multiplying numbers or setting system variables. Now that you know how to save AutoLISP code in a file, you can begin to create larger programs. The box program is really nothing more than a collection of expressions that are designed to work together to obtain specific results. In this section, we will examine the Box program to see how it works.
The Box program draws the box by first obtaining a corner point using Getpoint:
(setq pt1 (getpoint pt1 "Pick first corner: ''))
The user will see only the prompt portion of this expression:
Pick first corner:
Next, the opposite corner point is obtained using Getcorner (see Figure 2.3).
(setq pt3 (getcorner pt1 "Pick opposite corner: ''))
Again, the user only sees the prompt string:
Pick opposite corner:
Figure 2.3: The workings of the box program
You may recall that Getcorner will display a window as the user move the cursor. In this box program, this window allows the user to visually see the shape of the box before the opposite corner is selected (see figure 2.2). Once the
33
Copyright © 2001 George Omura,,World rights reserved
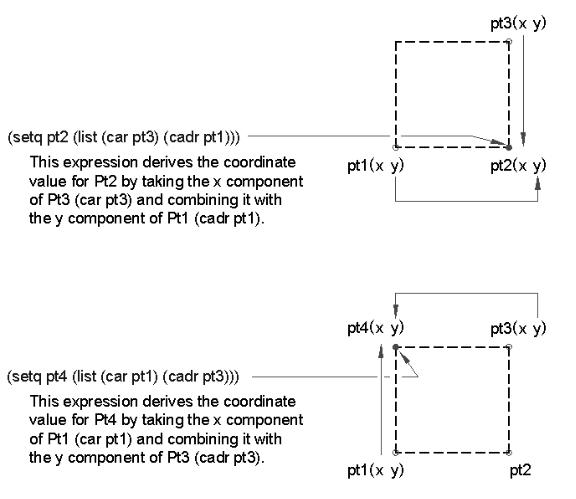
The ABC’s of AutoLISP by George Omura
second point is selected, the Box program uses the point coordinates of the first and opposite corners to derive the other two corners of the box. This is done by manipulating the known coordinates using Car, Cadr, and List (see Figure 2.4).
pt2 (list (car pt3) (cadr pt1)))
pt4 (list (car pt1) (cadr pt3)))
Figure 2.4: Using Car, Cadr, and List to derive the remaining box corners
Pt2 is derived by combining the X component of Pt3 with the Y component of Pt1. Pt 4 is derived from combining the X component of Pt1 with the Y component of Pt3 (see figure 2.5).
34
Copyright © 2001 George Omura,,World rights reserved
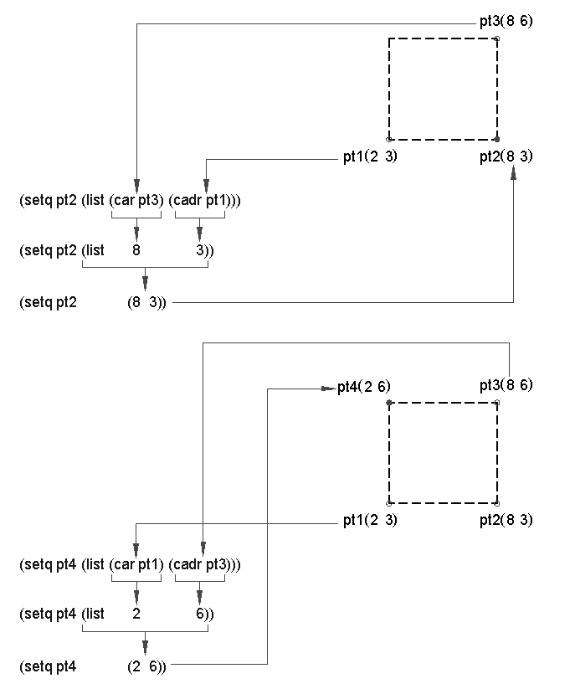
The ABC’s of AutoLISP by George Omura
Figure 2.5: Using Car and Cadr to derive Pt2 and Pt4
35
Copyright © 2001 George Omura,,World rights reserved
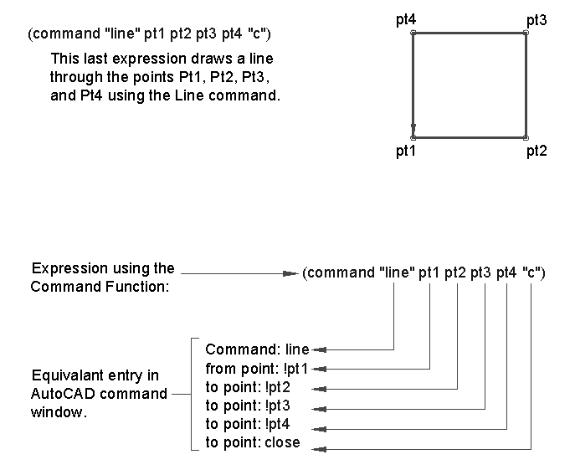
The ABC’s of AutoLISP by George Omura
Using AutoCAD Commands in AutoLISP
The last line in the box program:
(command "line'' pt1 pt2 pt3 pt4 "c'')
shows you how AutoCAD commands are used in an AutoLISP expression (see figure 2.6). Command is an AutoLISP function that calls standard AutoCAD commands. The command to be called following the Command function is enclosed in quotation marks. Anything in quotation marks after the Command function is treated as keyboard input. Variables follow, but unlike accessing variables from the command prompt, they do not have to be preceded by an exclamation point. The C enclosed in quotation marks at the end of the expression indicates a Close option for the Line command (see Figure 2.7.
Figure 2.6: Using AutoCAD commands in a function.
Figure 2.7: Variables help move information from one expression to another.
36
Copyright © 2001 George Omura,,World rights reserved