
- •Introduction
- •Who should read this book
- •How This Book Is Organized
- •How to Use This Book
- •Where to Find the LISP Programs
- •CHAPTER 1: Introducing AutoLISP
- •Understanding the Interpreter and Evaluation
- •The Components of an Expression
- •Using Arguments and Expressions
- •Using Variables
- •Understanding Data Types
- •Integers and Real Numbers
- •Strings
- •Lists
- •File Descriptors
- •Object Names
- •Selection Sets
- •Symbols
- •Subrs
- •Atoms
- •Assigning Values to Variables with Setq
- •Preventing Evaluation of Arguments
- •Applying Variables
- •Functions for Assigning Values to Variables
- •Adding Prompts
- •CHAPTER 2: Storing and Running Programs
- •Creating an AutoLISP Program
- •What you Need
- •Creating an AutoLISP File
- •Loading an AutoLISP file
- •Running a Loaded Program
- •Understanding How a Program Works
- •Using AutoCAD Commands in AutoLISP
- •How to Create a Program
- •Local and Global Variables
- •Automatic Loading of Programs
- •Managing Large Acad.lsp files
- •Using AutoLISP in a Menu
- •Using Script Files
- •CHAPTER 3: Organizing a Program
- •Looking at a Programs Design
- •Outlining Your Programming Project
- •Using Functions
- •Adding a Function
- •Reusing Functions
- •Creating an 3D Box program
- •Creating a 3D Wedge Program
- •Making Your Code More Readable
- •Using Prettyprint
- •Using Comments
- •Using Capitals and Lower Case Letters
- •Dynamic Scoping
- •CHAPTER 4: Interacting with the Drawing Editor
- •A Sample Program Using Getdist
- •How to Get Angle Values
- •Using Getangle and Getorient
- •How to Get Text Input
- •Using Getstring
- •Using Getkword
- •How to Get Numeric Values
- •Using Getreal and Getint
- •How to Control User Input
- •Using Initget
- •Prompting for Dissimilar Variable Types
- •Using Multiple Keywords
- •How to Select Groups of Objects
- •Using Ssget
- •A Sample Program Using Ssget
- •CHAPTER 5: Making Decisions with AutoLISP
- •Making Decisions
- •How to Test for Conditions
- •Using the If function
- •How to Make Several Expressions Act like One
- •How to Test Multiple Conditions
- •Using the Cond function
- •How to Repeat parts of a Program
- •Using the While Function
- •Using the Repeat Function
- •Using Test Expressions
- •CHAPTER 6: Working With Geometry
- •How to find Angles and Distances
- •Understanding the Angle, Distance, and Polar Functions
- •Using Trigonometry to Solve a Problem
- •Gathering Information
- •Finding Points Using Trigonometry
- •Functions Useful in Geometric Transformations
- •Trans
- •Atan
- •Inters
- •CHAPTER 7: Working with Text
- •Working With String Data Types
- •Searching for Strings
- •Converting a Number to a String
- •How to read ASCII text files
- •Using a File Import Program
- •Writing ASCII Files to Disk
- •Using a Text Export Program
- •CHAPTER 8: Interacting with AutoLISP
- •Reading and Writing to the Screen
- •Reading the Cursor Dynamically
- •Writing Text to the Status and Menu Areas
- •Calling Menus from AutoLISP
- •Drawing Temporary Images on the Drawing Area
- •Using Defaults in a Program
- •Adding Default Responses to your Program
- •Dealing with Aborted Functions
- •Using the *error* Function
- •Organizing Code to Reduce Errors
- •Debugging Programs
- •Common Programming Errors
- •Using Variables as Debugging Tools
- •CHAPTER 9: Using Lists to store data
- •Getting Data from a List
- •Using Simple Lists for Data Storage
- •Evaluating Data from an Entire List at Once
- •Using Complex Lists to Store Data
- •Using Lists for Comparisons
- •Locating Elements in a List
- •Searching Through Lists
- •Finding the Properties of AutoCAD Objects
- •Using Selection Sets and Object Names
- •Understanding the structure of Property Lists
- •Changing the properties of AutoCAD objects
- •Getting an Object Name and Coordinate Together
- •CHAPTER 10: Editing AutoCAD objects
- •Editing Multiple objects
- •Improving Processing Speed
- •Using Cmdecho to Speed up Your Program
- •Improving Speed Through Direct Database Access
- •Filtering Objects for Specific Properties
- •Filtering a Selection Set
- •Selecting Objects Based on Properties
- •Accessing AutoCAD's System Tables
- •CHAPTER 11: Accessing Complex Objects
- •Accessing Polyline Vertices
- •Defining a New Polyline
- •Drawing the new Polyline
- •Testing for Polyline Types
- •How Arcs are Described in Polylines
- •Accessing Object Handles and Block Attributes
- •Using Object Handles
- •Using Object Handles
- •Extracting Attribute Data
- •Appendix A: Menu Primer
- •Appendix B: Error Messages
- •Appendix C: Group Codes
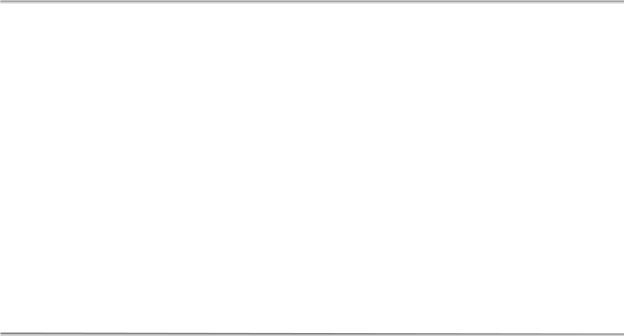
The ABC’s of AutoLISP by George Omura
Once the or function returns a T, the second argument is evaluated which means that a 3dbox is drawn. If or returns nil, then the third argument is evaluated drawing a 2 dimensional box. The and function works in a similar way to or but and requires that all its arguments return non-nil before it returns T. Both or and will accept more that two arguments.
How to Make Several Expressions Act like One
There will be times when you will want several expressions to be evaluated depending on the results of a predicate. As an example, lets assume that you have decided to make the 2 dimensional and 3 dimensional box programs part of the C:MAINBOX program to save memory. You could place the code for these functions directly in the C:MAINBOX program and have a file that looks like figure 5.5. When this program is run, it acts exactly the same way as in the previous exercise. But in figure 5.4, the functions BOX1 and 3DBOX are incorporated into the if expression as arguments.
(defun |
C:MAINBOX (/ pt1 |
pt2 pt3 pt4 h choose) |
(setq choose (getstring |
"\nDo you want a 3D box <Y=yes/Return=no>? ")) |
|
(if |
(or (equal choose "y")(equal choose "Y")) |
|
(progn |
;if choose = Y or y then draw a 3D |
|
box |
|
|
(getinfo)
(setq h (getreal "Enter height of box: ")) (procinfo)
(output)
(command "change" "Last" "" "Properties" "thickness" h "" "3dface" pt1 pt2 pt3 pt4 ""
"3dface" ".xy" pt1 h ".xy" pt2 h ".xy" pt3 h ".xy" pt4 h ""
);end command );end progn
(progn ;if choose /= Y or y then draw a 2D box (getinfo)
(procinfo)
(output) );end progn
);end if );end MAINBOX
Figure 5.4: The C;Mainbox program incorporating the code from Box1 and 3dbox
98
Copyright © 2001 George Omura,,World rights reserved
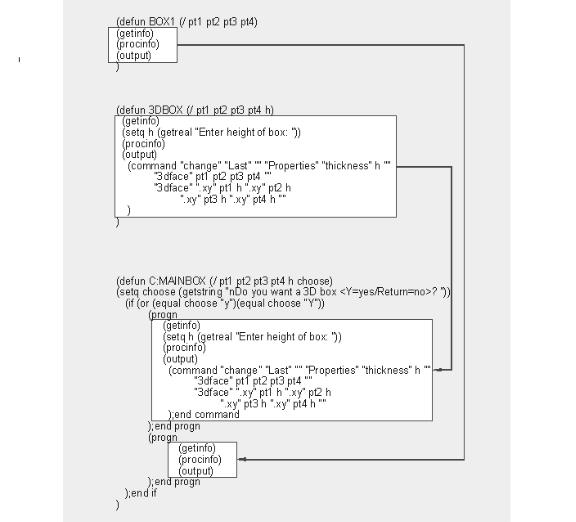
The ABC’s of AutoLISP by George Omura
Figure 5.5: Using the progn function
We are able to do this using the progn function. Progn allows you to have several expression where only one is expected. Its syntax is as follows:
(progn
(expression1) (expression2) (expression3) . . .
)
99
Copyright © 2001 George Omura,,World rights reserved
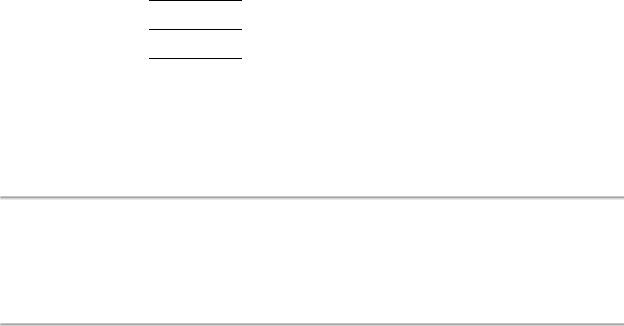
The ABC’s of AutoLISP by George Omura
Figure 5.5 shows how we arrived at the program in figure 5.4. The calls to functions BOX1 and 3DBOX were replaced by the actual expressions used in those functions.
How to Test Multiple Conditions
There is also another function that acts very much like the if function called Cond. Arguments to cond consists of one or more expressions that have as their first element a test expression followed by an object that is to be evaluated if the test returns T. Cond evaluates each test until it comes to one that returns T. It then evaluates the expression or atom associated with that test. If other test expressions follow, they are ignored.
Using the Cond function
The syntax for cond is:
(cond
((test expression)[expression/atom][expression/atom]...) ((test expression)[expression/atom][expression/atom]...) ((test expression)[expression/atom][expression/atom]...) ((test expression)[expression/atom][expression/atom]...)
)
Figure 5.6 shows the cond function used in place of the if function. Cond's syntax also allows for more than one expression for each test expression. This means that you don't have to use the Progn function with cond if you want several expressions evaluated as a result of a test.
(defun C:MAINBOX (/ choose)
(setq choose (getstring "\nDo you want a 3D box <Y=yes/Return=no>? ")) (cond
( (or (equal choose "y") (equal choose "Y")) (3dbox)) ( (or (/= choose "y") (/= choose "Y")) (box1))
)
)
Figure 5.6: Using cond in place of if
100
Copyright © 2001 George Omura,,World rights reserved
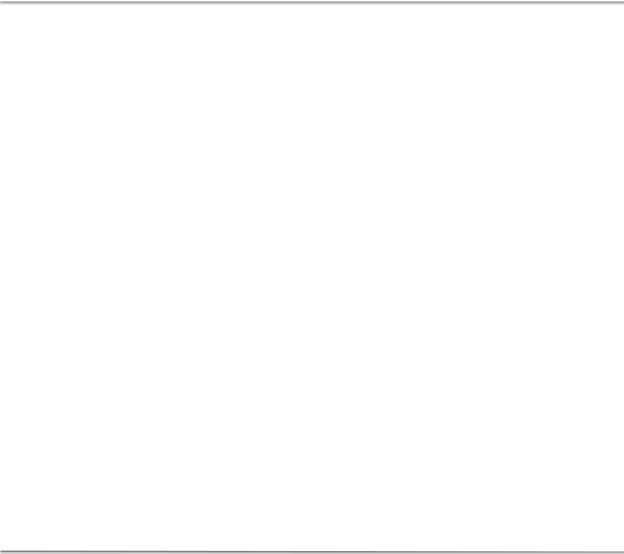
The ABC’s of AutoLISP by George Omura
Figure 5.7 shows another program called Chaos that uses the Cond function. Chaos is an AutoLISP version of a mathematical game used to demonstrate the creation of fractals through an iterated function. The game works by following the steps shown in Figure 5.8.
;function to find the midpoint between two points (defun mid (a b)
(list (/ (+ (car a)(car b)) 2) (/ (+ (cadr a)(cadr b)) 2)
)
)
;function to generate random number (defun rand (pt / rns rleng lastrn)
(setq rns (rtos (* (car pt)(cadr pt)(getvar "tdusrtimer")))) (setq rnleng (strlen rns))
(setq lastrn (substr rns rnleng 1)) (setq rn (* 0.6 (atof lastrn))) (fix rn)
)
;The Chaos game
(defun C:CHAOS (/ pta ptb ptc rn count lastpt randn key) (setq pta '( 2.0000 1 )) ;define point a
(setq ptb '( 7.1962 10)) ;define point b (setq ptc '(12.3923 1 )) ;define point c
(setq lastpt (getpoint "Pick a start point:")) ;pick a point to start (while (/= key 3) ;while pick button not pushed
(setq randn (rand lastpt)) ;get random number (cond ;find midpoint to a b or c
( (= randn 0)(setq lastpt (mid lastpt pta)) ) ;use corner a if 0 ( (= randn 1)(setq lastpt (mid lastpt pta)) ) ;use corner a if 1 ( (= randn 2)(setq lastpt (mid lastpt ptb)) ) ;use corner b if 2 ( (= randn 3)(setq lastpt (mid lastpt ptb)) ) ;use corner b if 3 ( (= randn 4)(setq lastpt (mid lastpt ptc)) ) ;use corner c if 4 ( (= randn 5)(setq lastpt (mid lastpt ptc)) ) ;use corner c if 5
);end cond
(grdraw lastpt lastpt 5) ;draw midpoint (setq key (car (grread T))) ;test for pick
);end while );end Chaos
Figure 5.7: The Chaos game program
101
Copyright © 2001 George Omura,,World rights reserved
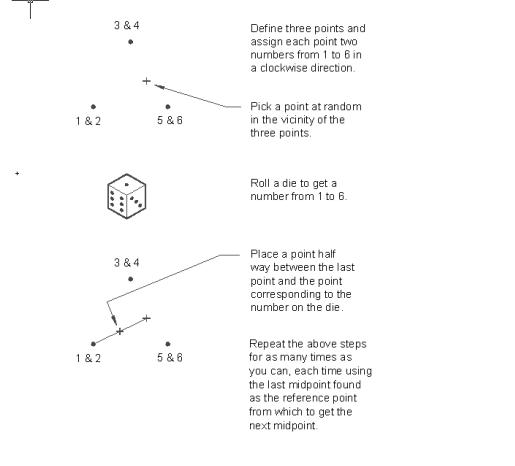
The ABC’s of AutoLISP by George Omura
Cond can be used anywhere you would use if. For example:
(if (not C:BOX) (load "box") (princ "Box is already loaded. "))
can be written:
(cond
((not C:BOX) (load "box"))
((not (not C:BOX)) (princ "Box is already loaded. "))
)
Figure 5.8: How to play the Chaos game
102
Copyright © 2001 George Omura,,World rights reserved