
- •Introduction
- •Who should read this book
- •How This Book Is Organized
- •How to Use This Book
- •Where to Find the LISP Programs
- •CHAPTER 1: Introducing AutoLISP
- •Understanding the Interpreter and Evaluation
- •The Components of an Expression
- •Using Arguments and Expressions
- •Using Variables
- •Understanding Data Types
- •Integers and Real Numbers
- •Strings
- •Lists
- •File Descriptors
- •Object Names
- •Selection Sets
- •Symbols
- •Subrs
- •Atoms
- •Assigning Values to Variables with Setq
- •Preventing Evaluation of Arguments
- •Applying Variables
- •Functions for Assigning Values to Variables
- •Adding Prompts
- •CHAPTER 2: Storing and Running Programs
- •Creating an AutoLISP Program
- •What you Need
- •Creating an AutoLISP File
- •Loading an AutoLISP file
- •Running a Loaded Program
- •Understanding How a Program Works
- •Using AutoCAD Commands in AutoLISP
- •How to Create a Program
- •Local and Global Variables
- •Automatic Loading of Programs
- •Managing Large Acad.lsp files
- •Using AutoLISP in a Menu
- •Using Script Files
- •CHAPTER 3: Organizing a Program
- •Looking at a Programs Design
- •Outlining Your Programming Project
- •Using Functions
- •Adding a Function
- •Reusing Functions
- •Creating an 3D Box program
- •Creating a 3D Wedge Program
- •Making Your Code More Readable
- •Using Prettyprint
- •Using Comments
- •Using Capitals and Lower Case Letters
- •Dynamic Scoping
- •CHAPTER 4: Interacting with the Drawing Editor
- •A Sample Program Using Getdist
- •How to Get Angle Values
- •Using Getangle and Getorient
- •How to Get Text Input
- •Using Getstring
- •Using Getkword
- •How to Get Numeric Values
- •Using Getreal and Getint
- •How to Control User Input
- •Using Initget
- •Prompting for Dissimilar Variable Types
- •Using Multiple Keywords
- •How to Select Groups of Objects
- •Using Ssget
- •A Sample Program Using Ssget
- •CHAPTER 5: Making Decisions with AutoLISP
- •Making Decisions
- •How to Test for Conditions
- •Using the If function
- •How to Make Several Expressions Act like One
- •How to Test Multiple Conditions
- •Using the Cond function
- •How to Repeat parts of a Program
- •Using the While Function
- •Using the Repeat Function
- •Using Test Expressions
- •CHAPTER 6: Working With Geometry
- •How to find Angles and Distances
- •Understanding the Angle, Distance, and Polar Functions
- •Using Trigonometry to Solve a Problem
- •Gathering Information
- •Finding Points Using Trigonometry
- •Functions Useful in Geometric Transformations
- •Trans
- •Atan
- •Inters
- •CHAPTER 7: Working with Text
- •Working With String Data Types
- •Searching for Strings
- •Converting a Number to a String
- •How to read ASCII text files
- •Using a File Import Program
- •Writing ASCII Files to Disk
- •Using a Text Export Program
- •CHAPTER 8: Interacting with AutoLISP
- •Reading and Writing to the Screen
- •Reading the Cursor Dynamically
- •Writing Text to the Status and Menu Areas
- •Calling Menus from AutoLISP
- •Drawing Temporary Images on the Drawing Area
- •Using Defaults in a Program
- •Adding Default Responses to your Program
- •Dealing with Aborted Functions
- •Using the *error* Function
- •Organizing Code to Reduce Errors
- •Debugging Programs
- •Common Programming Errors
- •Using Variables as Debugging Tools
- •CHAPTER 9: Using Lists to store data
- •Getting Data from a List
- •Using Simple Lists for Data Storage
- •Evaluating Data from an Entire List at Once
- •Using Complex Lists to Store Data
- •Using Lists for Comparisons
- •Locating Elements in a List
- •Searching Through Lists
- •Finding the Properties of AutoCAD Objects
- •Using Selection Sets and Object Names
- •Understanding the structure of Property Lists
- •Changing the properties of AutoCAD objects
- •Getting an Object Name and Coordinate Together
- •CHAPTER 10: Editing AutoCAD objects
- •Editing Multiple objects
- •Improving Processing Speed
- •Using Cmdecho to Speed up Your Program
- •Improving Speed Through Direct Database Access
- •Filtering Objects for Specific Properties
- •Filtering a Selection Set
- •Selecting Objects Based on Properties
- •Accessing AutoCAD's System Tables
- •CHAPTER 11: Accessing Complex Objects
- •Accessing Polyline Vertices
- •Defining a New Polyline
- •Drawing the new Polyline
- •Testing for Polyline Types
- •How Arcs are Described in Polylines
- •Accessing Object Handles and Block Attributes
- •Using Object Handles
- •Using Object Handles
- •Extracting Attribute Data
- •Appendix A: Menu Primer
- •Appendix B: Error Messages
- •Appendix C: Group Codes
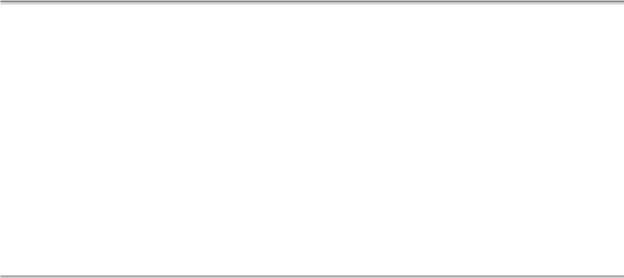
The ABC’s of AutoLISP by George Omura
Writing ASCII Files to Disk
AutoLISP lets you create ASCII files. You can use this capability to store different types of information ranging from the current drawing status to general notes that appear in a drawing. The program in figure 7.13 demonstrates this capability.
;Program to export text from AutoCAD -- Exprt.lsp (Defun C:EXPRT (/ fname txt selset count nme oldtx)
(setq fname (getstring "\nEnter name of file to be saved: "))
(setq txt (open fname "w")) |
;open file, assign symbol |
(setq selset (ssget)) |
;get selection set |
(setq count 0) |
;set count to zero |
(if (/= selset nil) |
|
(while (< count (sslength selset)) |
;while count < # of lines |
(setq nme (ssname selset count)) |
;extract text string |
(setq oldtx (cdr (assoc 1 (entget nme)))) |
|
(write-line oldtx txt) |
;write string to file |
(setq count (1+ count)) |
;go to next line |
);end while |
|
);end if |
|
(close txt) |
;close file |
);end C:EXPRT |
|
Figure 7.13: A text export program
Using a Text Export Program
Exit AutoCAD then create a file called Exprt.lsp containing the program shown in figure 7.16. Return to AutoCAD, load the file and proceed with the following:
1.Erase the text you imported previously.
2.Use the dtext command and starting the text at the coordinate 2,8, write the following lines:
For want of a nail, the shoe was lost; For want of a shoe, the horse was lost; For want of a horse, the rider was lost;
3.Enter exprt to start the text export program.
156
Copyright © 2001 George Omura,,World rights reserved
The ABC’s of AutoLISP by George Omura
4. At the prompt:
Enter name of file to save:
Enter test.txt.
5. At the prompt:
Select objects:
Pick the lines of text you just entered picking each one individually from top to bottom. Press return when you are done.
The file test.txt is created containing the text you had just entered using AutoCAD's Dtext command. To make sure the file exists, enter the following at the command prompt:
type test.txt
The AutoCAD type command is the same as the DOS type command. It displays the contents of a text file. AutoCAD will switch to text mode and the contents of test.txt will be displayed on the screen.
The Exprt program starts by prompting the user to enter a name for the file to be saved to:
(Defun c:exprt (/ ts n dir)
(setq fname (getstring "\nEnter name of file to save: "))
Then, just as with the imprt program, the open function opens the file:
(setq txt (open n "w"))
In this case, the "w" code is used with the open functions since this file is to be written to. The next line obtains a group of objects for editing using the ssget function:
(setq selset (ssget))
This selection set is saved as the variable selset. Next, a variable count is give the value 0 in preparation for the while expression that follows:
(setq count 0)
The next if expression checks to see that the user has indeed picks objects for editing.
(if (/= selset nil)
If the variable selset does not return nil, then the while expression is evaluated:
(while (< count (sslength selset))
(setq entnme (ssname selset count))
157
Copyright © 2001 George Omura,,World rights reserved

The ABC’s of AutoLISP by George Omura
(setq oldtx (cdr (assoc 1 (entget entnme))))
(write-line oldtx txt)
(setq count (1+ count))
)
This while expression uses the count variable to determine the number of times it must evaluate its set of expressions:
(while (< count (sslength selset))
The sslength function returns the number of objects contained in a selection set. In this case, it returns the number of objects recorded in the variable selset. This value is compared with the variable count to determine whether or not to evaluate the expressions found under the while expression.
The next two expressions extract the text string value from the first of the objects selected:
(setq entnme (ssname selset count))
(setq oldtx (cdr (assoc 1 (entget entnme))))
This extraction process involves several new functions which are discussed in chapter . For now, just accept that the end result is the assignment of the text value of the selected object to the variable oldtx.
Now the actual writing to the file occurs:
(write-line oldtx txt)
Here the write-line functions reads the string held by oldtx and writes it to the file represented by the variable txt.
Write-line's syntax is:
(write-line [string][file descriptor])
The first argument is the string to be written to file while the second argument is a variable assigned to the open file.
The next line increases the value of count by one:
(setq count (1+ count))
This expression counts the number of times a string of text, and therefore an object, has been processed. Since the while test expression checks to see if the value of count is less than the number of objects selected, once count reaches a value equivalent to the number of objects selected and processed, the while expression stops processing.
158
Copyright © 2001 George Omura,,World rights reserved
The ABC’s of AutoLISP by George Omura
Finally, the all important close expression appears:
)
(close txt)
)
Just as with the C:IMPRT program, close must be used to properly close the file under DOS, otherwise its contents may be in-accessible.
Read-line and write-line are two of several file read and write functions available in AutoLISP. Table shows several other functions along with a brief description.
Function
(prin1 symbol/expression)
(princ symbol/expression)
print symbol/expression)
(read-char file_descriptor)
read-line file_descriptor)
write-char integer file_descriptor)
Description
Prints any expression to the screen prompt. If a file descriptor is included as an argument, the expression is written to the file as well.
The same as prin1 but execute's control characters. Also, String quotation marks are dropped.
The Same as prin1 but a new line is printed before its expression and a space is printed after.
Reads a single character from the keyboard. If a file descriptor is included as an argument, it reads a character from the file. The value returned is in the form of an ASCII character code.
Reads a string from the keyboard or a line of text from an open file.
Writes a single character to the screen prompt or if a file descriptor is provided, to an open file. The character argument is a number representing an ASCII character code.
write-line string file_descriptor)Writesfile. a string to the screen prompt or if a file descriptor is provided, to an open
The three functions prin1, princ, and print are nearly identical with some slight differences. All three use the same syntax as shown in the following:
(princ [string or string variable][optional file descriptor])
The file descriptor is a symbol that has been assigned an open file.
159
Copyright © 2001 George Omura,,World rights reserved
The ABC’s of AutoLISP by George Omura
The main difference between these three functions is in what they produce as values. The following shows an expression using prin1 followed by the resulting value:
(prin1 "\nFor want of a nail...")
"For want of a nail...""\nFor want of a nail..."
Notice that the string argument to prin1 is printed twice to the prompt line. This is because both prin1 and AutoLISP will print something to the prompt. Prin1 prints its a literal version of its string argument. AutoLISPs read-evaluate- print loop also prints the value of the last object evaluated. The net result it the appearance of the string twice on the same line.
Princ differs from prin1 in that instead of printing a literal version of its string argument, it will act on any control characters included in the string:
(princ "\nFor want of a nail...")
For want of a nail..."\nFor want of a nail..."
In the prin1 example, the \n control character is printed without acting on it. In the princ example above, the \n character causes the AutoCAD prompt to advance one line. Also, the string is printed without the quotation marks. Again, the AutoLISP interpreter prints the value of the string directly to the prompt line after princ does it's work. Table Shows the other control characters and what they do.
Character |
Use |
\e |
Escape |
\n |
New line |
\r |
Return |
\t |
Tab |
\nnn |
Character whose octal code is nnn |
The print function differs from the prin1 function in that it advances the prompt one line before printing the string then it adds a space at the end of the string:
(print "\nFor want of a nail...")
"\nFor want of a nail..." "\nFor want of a nail..."
Just as with prin1, print prints a literal version of its string argument.
160
Copyright © 2001 George Omura,,World rights reserved
The ABC’s of AutoLISP by George Omura
Conclusion
While a good deal of your effort using AutoLISP will concentrate on graphics and numeric computation, the ability to manipulate string data will be an important part of your work. You have been introduced to those functions that enable you to work with strings.
You have also seen how these functions work together to perform some simple tasks like reading and writing text files. But you don't have to limit yourself to using these functions for text editing. Since any kind of information can be stored as a string, you may find ways to further enhance your use of AutoCAD through the manipulation of string data. One of the more obvious uses that comes to mind is the importation of numeric data for graphing, charting or other types of data analysis. As long as data is stored in an ASCII format, AutoLISP can read it. And with AutoLISP's data conversion functions, numeric data can be easily translated from ASCII files.
161
Copyright © 2001 George Omura,,World rights reserved