
- •Introduction
- •Who should read this book
- •How This Book Is Organized
- •How to Use This Book
- •Where to Find the LISP Programs
- •CHAPTER 1: Introducing AutoLISP
- •Understanding the Interpreter and Evaluation
- •The Components of an Expression
- •Using Arguments and Expressions
- •Using Variables
- •Understanding Data Types
- •Integers and Real Numbers
- •Strings
- •Lists
- •File Descriptors
- •Object Names
- •Selection Sets
- •Symbols
- •Subrs
- •Atoms
- •Assigning Values to Variables with Setq
- •Preventing Evaluation of Arguments
- •Applying Variables
- •Functions for Assigning Values to Variables
- •Adding Prompts
- •CHAPTER 2: Storing and Running Programs
- •Creating an AutoLISP Program
- •What you Need
- •Creating an AutoLISP File
- •Loading an AutoLISP file
- •Running a Loaded Program
- •Understanding How a Program Works
- •Using AutoCAD Commands in AutoLISP
- •How to Create a Program
- •Local and Global Variables
- •Automatic Loading of Programs
- •Managing Large Acad.lsp files
- •Using AutoLISP in a Menu
- •Using Script Files
- •CHAPTER 3: Organizing a Program
- •Looking at a Programs Design
- •Outlining Your Programming Project
- •Using Functions
- •Adding a Function
- •Reusing Functions
- •Creating an 3D Box program
- •Creating a 3D Wedge Program
- •Making Your Code More Readable
- •Using Prettyprint
- •Using Comments
- •Using Capitals and Lower Case Letters
- •Dynamic Scoping
- •CHAPTER 4: Interacting with the Drawing Editor
- •A Sample Program Using Getdist
- •How to Get Angle Values
- •Using Getangle and Getorient
- •How to Get Text Input
- •Using Getstring
- •Using Getkword
- •How to Get Numeric Values
- •Using Getreal and Getint
- •How to Control User Input
- •Using Initget
- •Prompting for Dissimilar Variable Types
- •Using Multiple Keywords
- •How to Select Groups of Objects
- •Using Ssget
- •A Sample Program Using Ssget
- •CHAPTER 5: Making Decisions with AutoLISP
- •Making Decisions
- •How to Test for Conditions
- •Using the If function
- •How to Make Several Expressions Act like One
- •How to Test Multiple Conditions
- •Using the Cond function
- •How to Repeat parts of a Program
- •Using the While Function
- •Using the Repeat Function
- •Using Test Expressions
- •CHAPTER 6: Working With Geometry
- •How to find Angles and Distances
- •Understanding the Angle, Distance, and Polar Functions
- •Using Trigonometry to Solve a Problem
- •Gathering Information
- •Finding Points Using Trigonometry
- •Functions Useful in Geometric Transformations
- •Trans
- •Atan
- •Inters
- •CHAPTER 7: Working with Text
- •Working With String Data Types
- •Searching for Strings
- •Converting a Number to a String
- •How to read ASCII text files
- •Using a File Import Program
- •Writing ASCII Files to Disk
- •Using a Text Export Program
- •CHAPTER 8: Interacting with AutoLISP
- •Reading and Writing to the Screen
- •Reading the Cursor Dynamically
- •Writing Text to the Status and Menu Areas
- •Calling Menus from AutoLISP
- •Drawing Temporary Images on the Drawing Area
- •Using Defaults in a Program
- •Adding Default Responses to your Program
- •Dealing with Aborted Functions
- •Using the *error* Function
- •Organizing Code to Reduce Errors
- •Debugging Programs
- •Common Programming Errors
- •Using Variables as Debugging Tools
- •CHAPTER 9: Using Lists to store data
- •Getting Data from a List
- •Using Simple Lists for Data Storage
- •Evaluating Data from an Entire List at Once
- •Using Complex Lists to Store Data
- •Using Lists for Comparisons
- •Locating Elements in a List
- •Searching Through Lists
- •Finding the Properties of AutoCAD Objects
- •Using Selection Sets and Object Names
- •Understanding the structure of Property Lists
- •Changing the properties of AutoCAD objects
- •Getting an Object Name and Coordinate Together
- •CHAPTER 10: Editing AutoCAD objects
- •Editing Multiple objects
- •Improving Processing Speed
- •Using Cmdecho to Speed up Your Program
- •Improving Speed Through Direct Database Access
- •Filtering Objects for Specific Properties
- •Filtering a Selection Set
- •Selecting Objects Based on Properties
- •Accessing AutoCAD's System Tables
- •CHAPTER 11: Accessing Complex Objects
- •Accessing Polyline Vertices
- •Defining a New Polyline
- •Drawing the new Polyline
- •Testing for Polyline Types
- •How Arcs are Described in Polylines
- •Accessing Object Handles and Block Attributes
- •Using Object Handles
- •Using Object Handles
- •Extracting Attribute Data
- •Appendix A: Menu Primer
- •Appendix B: Error Messages
- •Appendix C: Group Codes
The ABC’s of AutoLISP by George Omura
Filtering Objects for Specific Properties
There are a number of other functions available that allow you to manipulate selection sets Table lists them and gives a brief description of what they do:
Function |
Description |
(ssadd [ent. name][s. set]) |
Creates a selection set. If used with no arguments, a selection set is created |
|
with no objects. If only an object name given as an argument, then a |
|
selection set is created that contains that object. If an object name and a |
|
selection set name is given, then the object is added to the selection set. |
(ssdel [ent. name][s. set]) |
Deletes an object from a selection set. ssdel then returns the name of the |
|
selection set. IF the object is not a member of the selection set, then ssdel |
|
returns nil. |
(sslength [s. set]) |
Returns the number of objects in a selection set. |
(ssmemb [ent. name][s. set]) |
Checks to see if an object is a member of a selection set. If it is, then ssmemb |
|
returns the name of the selection set. if not, then ssmemb returns nil. |
(ssname [s. set][nth object]) |
Returns the object name of a single object in a selection set. The second |
|
argument to ssname corresponds to the object's number within the selection |
|
set. The object numbers begin with zero and go to one minus the total |
|
number of objects in the selection set. |
You have already seen two of these functions, sslength and ssname, used in previous examples. Lets see how we can use ssadd to filter out object selections.
Filtering a Selection Set
Figure 10-8 shows a function that is intended to filter out objects in a selection set based on layers. This function is useful where a group of objects are so close together that they are difficult to select, or in situations where several objects of different layers lie on top of each other and you want to select just the object on a specific layer. It returns a selection or object name depending on whether the filtered selection set contains only one item.
226
Copyright © 2001 George Omura,,World rights reserved

The ABC’s of AutoLISP by George Omura
;function to filter entities by layer -- Lfilter.lsp--------------------------
(defun LFILTER (/ lay sset count ent newent)
(setq lay (cons 8 (strcase (getstring "\nEnter layer name: "))))
(setq sset (ssget)) |
;get entities |
|
(setq count 0) |
;set counter to zero |
|
(while (< count (sslength sset)) |
;while still select. set |
|
(setq |
lay2 (assoc 8 (entget(setq ent(ssname sset count))))) ;get layer |
|
|
(if (equal lay lay2) |
;if layer matches entity |
|
(if (not newent) |
;if new not select. set |
|
(setq newent (ssadd ent)) |
;make new select. set |
|
(setq newent (ssadd ent newent)) |
;else add to select. set |
|
);end if |
|
|
);end if |
|
(setq |
count (1+ count)) |
|
);end while |
|
|
(if (= 1 |
(sslength newent))(ssname newent 0) newent) ;return select. set or |
|
);end defun |
|
entity name |
Figure 10.8: The layer filtering program
Let's see first hand how this function works.
1.Open a file call Lfilter.lsp and copy the Lfilter program in figure 10.8. Save and exit the file.
2.Return to the AutoCAD Chapt10 drawing and erase any objects in the file.
3.Create the layers listed in table 10.1. Be sure to assign the line types indicated for each layer.
4.Draw the lines shown in figure 10.9. and assign each line to the layer shown directly to the right of each line. Use the coordinate and spacing information indicated in the drawing to place the lines.
5.Load the Lfilter program, then issue the erase command.
6.At the Select object prompt, enter:
(lfilter)
You will get the prompt:
Enter layer name:
227
Copyright © 2001 George Omura,,World rights reserved
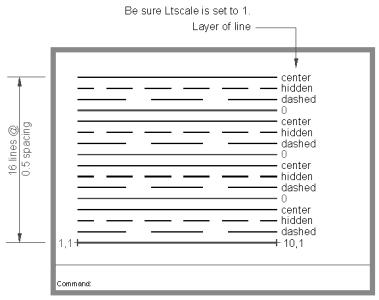
The ABC’s of AutoLISP by George Omura
8.Enter hidden. You will get the next prompt:
Select objects:
9.Enter C to use a crossing window and pick the points 2,1.25 for the lower left corner of the window and coordinate 8,8.25 for the upper right. The lines that pass through the crossing window will ghost. Press return after picking points. The lines will un-ghost then the prompt will return the number of objects found. The objects in the selection you just made with the crossing window that are on the layer hidden will ghost.
10.Press return. You have just erased only the items in your crossing window that were on the layer hidden.
11.Enter the Oops command in preparation for the next section.
Layer Name |
Linetype |
hidden |
hidden |
center |
center |
dashed |
dashed |
Table 10.1: Linetypes for drawing in figure 10.8
Figure 10.9: Test drawing for Lfilter.lsp
228
Copyright © 2001 George Omura,,World rights reserved
The ABC’s of AutoLISP by George Omura
Now that you know what Lfilter does, let's look at how it works. First, it creates a dotted pair representing the layer sublist in an object property list:
(defun LFILTER (/ lay sset count ent newent)
(setq lay (cons 8
(strcase (getstring "\nEnter layer name: "))))
When you are prompted for a layer, the name you enter is first converted to all upper case using the Strcase function. This is done because the value associated with the layer group code in a property list is always in upper case.
In order to make a comparison of data, we must make sure that the values we use in the comparison are in the same format. Since AutoLISP is case sensitive when it comes to string values, we must make sure that the value the user enters matches the case of the layer name in the property list. The strcase function will convert a string to either all upper case or lower case depending on whether a third argument is present and is not nil.
Next, the cons function creates a dotted pair using 8 as the first element. 8 is the group code for layer names. The dotted pair looks like this:
(8 . "Hidden")
Finally, the newly created dotted pair is assigned to the variable lay which will later be used as a filtering value.
The next line obtains the selection set to be filtered:
(setq sset (ssget))
Here, ssget is used without any argument thus allowing the user to select objects using any of the usual AutoCAD selection options. This selection set is then assigned to the variable sset.
Next we come to the while expression.
(setq count 0)
(while (< count (sslength sset)) (setq lay2 (assoc 8
(entget(setq ent(ssname sset count))))) (if (equal lay lay2)
(if (not newent)
(setq newent (ssadd ent))
(setq newent (ssadd ent newent))
229
Copyright © 2001 George Omura,,World rights reserved
The ABC’s of AutoLISP by George Omura
);end if
);end if
(setq count (1+ count))
);end while
This while expression compares the 8 group code sublist of each object in the selection against the variable lay. If it finds a match, the object is added to a new selection set newent. Let's look at this while expression in detail.
First, the counter is set to zero and the conditional test is set up:
(setq count 0)
(while (< count (sslength sset))
Then the 8 group code sublist from the first object in the selection set is extracted and assigned to the variable lay2:
(setq lay2 (assoc 8
(entget(setq ent(ssname sset count)))))
Next, the variables lay lay2 are compared:
(if (equal lay lay2)
If there is a match signifying that the object is on layer "HIDDEN", then the program checks to see if the selection set newent exists:
(if (not newent)
(setq newent (ssadd ent))
(setq newent (ssadd ent newent))
If newent does not exist, then ssadd creates a new selection set containing the object whose layer group code sublist matches (8 . "HIDDEN") then assigns that selection set to the variable newent. If newent does exist, ssadd adds the object to the selection set newent and redefines newent. This last conditional if is required since ssadd must be given different arguments depending on whether it is to create a new selection set or just add an object to an existing selection set.
Finally, the counter in increased by one and the while conditional loop repeats itself:
);end if
);end if
(setq count (1+ count))
);end while
230
Copyright © 2001 George Omura,,World rights reserved