
- •Contents
- •Preface
- •Introduction to Computers, the Internet and the Web
- •1.3 Computer Organization
- •Languages
- •1.9 Java Class Libraries
- •1.12 The Internet and the World Wide Web
- •1.14 General Notes about Java and This Book
- •Sections
- •Introduction to Java Applications
- •2.4 Displaying Text in a Dialog Box
- •2.5 Another Java Application: Adding Integers
- •2.8 Decision Making: Equality and Relational Operators
- •Introduction to Java Applets
- •3.2 Sample Applets from the Java 2 Software Development Kit
- •3.3 A Simple Java Applet: Drawing a String
- •3.4 Two More Simple Applets: Drawing Strings and Lines
- •3.6 Viewing Applets in a Web Browser
- •3.7 Java Applet Internet and World Wide Web Resources
- •Repetition)
- •Class Attributes
- •5.8 Labeled break and continue Statements
- •5.9 Logical Operators
- •Methods
- •6.2 Program Modules in Java
- •6.7 Java API Packages
- •6.13 Example Using Recursion: The Fibonacci Series
- •6.16 Methods of Class JApplet
- •Class Operations
- •Arrays
- •7.6 Passing Arrays to Methods
- •7.8 Searching Arrays: Linear Search and Binary Search
- •Collaboration Among Objects
- •8.2 Implementing a Time Abstract Data Type with a Class
- •8.3 Class Scope
- •8.4 Controlling Access to Members
- •8.5 Creating Packages
- •8.7 Using Overloaded Constructors
- •8.9 Software Reusability
- •8.10 Final Instance Variables
- •Classes
- •8.16 Data Abstraction and Encapsulation
- •9.2 Superclasses and Subclasses
- •9.5 Constructors and Finalizers in Subclasses
- •Conversion
- •9.11 Type Fields and switch Statements
- •9.14 Abstract Superclasses and Concrete Classes
- •9.17 New Classes and Dynamic Binding
- •9.18 Case Study: Inheriting Interface and Implementation
- •9.19 Case Study: Creating and Using Interfaces
- •9.21 Notes on Inner Class Definitions
- •Strings and Characters
- •10.2 Fundamentals of Characters and Strings
- •10.21 Card Shuffling and Dealing Simulation
- •Handling
- •Graphics and Java2D
- •11.2 Graphics Contexts and Graphics Objects
- •11.5 Drawing Lines, Rectangles and Ovals
- •11.9 Java2D Shapes
- •12.12 Adapter Classes
- •Cases
- •13.3 Creating a Customized Subclass of JPanel
- •Applications
- •Controller
- •Exception Handling
- •14.6 Throwing an Exception
- •14.7 Catching an Exception
- •Multithreading
- •15.3 Thread States: Life Cycle of a Thread
- •15.4 Thread Priorities and Thread Scheduling
- •15.5 Thread Synchronization
- •15.9 Daemon Threads
- •Multithreading
- •Design Patterns
- •Files and Streams
- •16.2 Data Hierarchy
- •16.3 Files and Streams
- •Networking
- •17.2 Manipulating URIs
- •17.3 Reading a File on a Web Server
- •17.4 Establishing a Simple Server Using Stream Sockets
- •17.5 Establishing a Simple Client Using Stream Sockets
- •17.9 Security and the Network
- •18.2 Loading, Displaying and Scaling Images
- •18.3 Animating a Series of Images
- •18.5 Image Maps
- •18.6 Loading and Playing Audio Clips
- •18.7 Internet and World Wide Web Resources
- •Data Structures
- •19.4 Linked Lists
- •20.8 Bit Manipulation and the Bitwise Operators
- •Collections
- •21.8 Maps
- •21.9 Synchronization Wrappers
- •21.10 Unmodifiable Wrappers
- •22.2 Playing Media
- •22.3 Formatting and Saving Captured Media
- •22.5 Java Sound
- •22.8 Internet and World Wide Web Resources
- •Hexadecimal Numbers
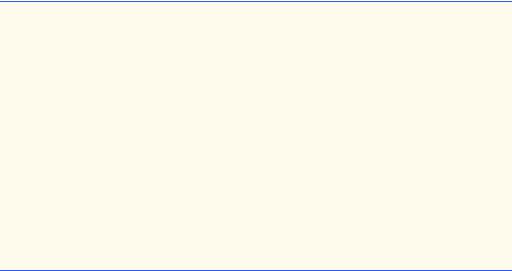
Chapter 18 |
Multimedia: Images, Animation, Audio and Video |
1081 |
Class LogoApplet (Fig. 18.4) defines an init method in which the three HTML parameters are read with Applet method getParameter (lines 31, 37 and 40). After the applet reads these parameters and the two integer parameters are converted to int values, the if/else structure at lines 48 through 51 creates a LogoAnimator2 and calls its three-argument constructor. If the imageName is null or totalImages is 0, the applet calls the default LogoAnimator2 constructor and uses the default animation. Otherwise, the applet passes totalImages, animationDelay and imageName to the three-argument LogoAnimator2 constructor, and the constructor uses those arguments to customize the animation. LogoAnimator2’s three-argument constructor invokes LogoAnimator’s initalizeAnimation method to load the images and determine the width and height of the animation.
18.5 Image Maps
Image maps are a common technique used to create interactive Web pages. An image map is an image that has hot areas that the user can click to accomplish a task, such as loading a different Web page into a browser. When the user positions the mouse pointer over a hot area, normally a descriptive message appears in the status area of the browser or in a popup window.
Figure 18.5 loads an image containing several of the common tip icons used throughout this book. The program allows the user to position the mouse pointer over an icon and display a descriptive message for the icon. Event handler mouseMoved (lines 42–46) takes the mouse coordinates and passes them to method translateLocation (lines 63–76). Method translateLocation tests the coordinates to determine the icon over which the mouse was positioned when the mouseMoved event occurred. Method translateLocation then returns a message indicating what the icon represents. This message is displayed in the appletviewer’s (or browser’s) status bar.
1// Fig. 18.5: ImageMap.java
2 // Demonstrating an image map.
3
4 // Java core packages
5import java.awt.*;
6 import java.awt.event.*;
7
8 // Java extension packages
9 import javax.swing.*;
10
11public class ImageMap extends JApplet {
12private ImageIcon mapImage;
13
14private String captions[] = { "Common Programming Error",
15"Good Programming Practice",
16"Graphical User Interface Tip", "Performance Tip",
17"Portability Tip", "Software Engineering Observation",
18"Testing and Debugging Tip" };
19
Fig. 18.5 Demonstrating an image map (part 1 of 4).
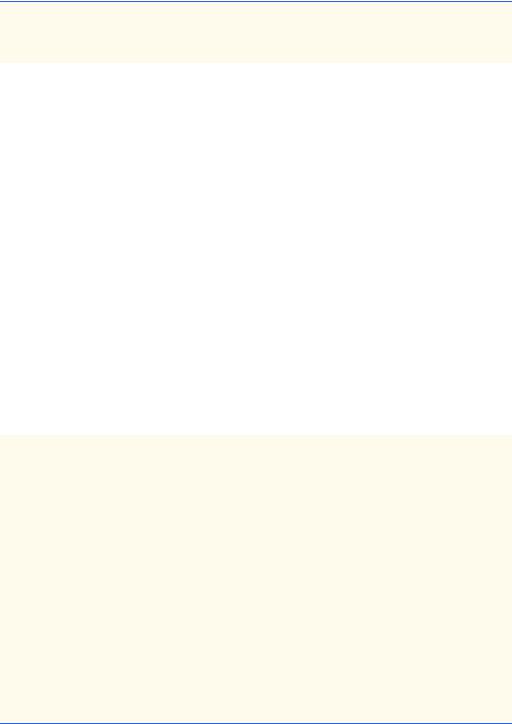
1082 |
Multimedia: Images, Animation, Audio and Video |
Chapter 18 |
20// set up mouse listeners
21public void init()
22{
23addMouseListener(
24 |
|
|
25 |
new MouseAdapter() { |
|
26 |
|
|
27 |
|
// indicate when mouse pointer exits applet area |
28 |
|
public void mouseExited( MouseEvent event ) |
29 |
|
{ |
30 |
|
showStatus( "Pointer outside applet" ); |
31 |
|
} |
32 |
|
|
33 |
} |
// end anonymous inner class |
34 |
|
|
35 |
); // end addMouseListener method call |
|
36 |
|
|
37 |
addMouseMotionListener( |
|
38 |
|
|
39 |
new MouseMotionAdapter() { |
|
40 |
|
|
41 |
|
// determine icon over which mouse appears |
42 |
|
public void mouseMoved( MouseEvent event ) |
43 |
|
{ |
44 |
|
showStatus( translateLocation( |
45 |
|
event.getX(), event.getY() ) ); |
46 |
|
} |
47 |
|
|
48 |
} |
// end anonymous inner class |
49 |
|
|
50 |
); // end addMouseMotionListener method call |
|
51 |
|
|
52 |
mapImage = new ImageIcon( "icons.png" ); |
|
53 |
|
|
54 |
} // end method init |
|
55 |
|
|
56// display mapImage
57public void paint( Graphics g )
58{
59mapImage.paintIcon( this, g, 0, 0 );
60}
61
62// return tip caption based on mouse coordinates
63public String translateLocation( int x, int y )
64{
65// if coordinates outside image, return immediately
66if ( x >= mapImage.getIconWidth() ||
67 |
y >= |
mapImage.getIconHeight() ) |
68 |
return |
""; |
69 |
|
|
70// determine icon number (0 - 6)
71int iconWidth = mapImage.getIconWidth() / 7;
72int iconNumber = x / iconWidth;
Fig. 18.5 Demonstrating an image map (part 2 of 4).

Chapter 18 |
Multimedia: Images, Animation, Audio and Video |
1083 |
73
74// return appropriate icon caption
75return captions[ iconNumber ];
76}
77
78 } // end class ImageMap
Fig. 18.5 Demonstrating an image map (part 3 of 4).

1084 |
Multimedia: Images, Animation, Audio and Video |
Chapter 18 |
|
|
|
|
|
|
Fig. 18.5 Demonstrating an image map (part 4 of 4).
Clicking in this applet will not cause any action. In Chapter 17, Networking, we discussed the techniques required to load another Web page into a browser via URLs and the AppletContext interface. Using those techniques, this applet could associate each icon with a URL that the browser would display when the user clicks the icon.
18.6 Loading and Playing Audio Clips
Java programs can manipulate and play audio clips. It is easy for users to capture their own audio clips, and there are many clips available in software products and over the Internet. Your system needs to be equipped with audio hardware (speakers and a sound board) to be able to play the audio clips.
Java provides several mechanisms for playing sounds in an applet. The two simplest methods are the Applet’s play method and the play method from the AudioClip interface. Additional audio capabilities are discussed in Chapter 22. If you would like to play a sound once in a program, the Applet method play loads the sound and plays it once; the sound is marked for garbage collection after it plays. The Applet method play has two forms:
public void play( URL location, String soundFileName ); public void play( URL soundURL );
The first version loads the audio clip stored in file soundFileName from location and plays the sound. The first argument is normally a call to the applet’s getDocument-
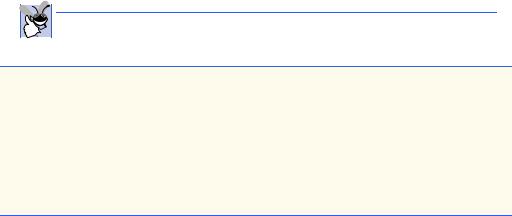
Chapter 18 |
Multimedia: Images, Animation, Audio and Video |
1085 |
Base or getCodeBase method. Method getDocumentBase indicates the location of the HTML file that loaded the applet (if the applet is in a package, this indicates the location of the package or JAR file containing the package). Method getCodeBase indicates the location of the applet’s .class file. The second version of method play takes a URL that contains the location and the file name of the audio clip. The statement
play( getDocumentBase(), "hi.au" );
loads the audio clip in file hi.au and plays it once.
The sound engine that plays the audio clips supports several audio file formats, including Sun Audio file format (.au extension), Windows Wave file format (.wav extension), Macintosh AIFF file format (.aif or .aiff extension) and Musical Instrument Digital Interface (MIDI) file format (.mid or .rmi extensions). The Java Media Framework (JMF) and Java Sound APIs support additional formats.
The program of Fig. 18.6 demonstrates loading and playing an AudioClip (package java.applet). This technique is more flexible than Applet method play. An applet can use an AudioClip to store audio for repeated use throughout the program’s execution. Applet method getAudioClip has two forms that take the same arguments as method play described previously. Method getAudioClip returns a reference to an AudioClip. An AudioClip has three methods—play, loop and stop. Method play plays the audio once. Method loop continuously loops the audio clip in the background. Method stop terminates an audio clip that is currently playing. In the program, each of these methods is associated with a button on the applet.
Lines 62–63 in the applet’s init method use getAudioClip to load two audio files—a Windows Wave file (welcome.wav) and a Sun Audio file (hi.au). The user can select which audio clip to play from JComboBox chooseSound. Notice that the applet’s stop method is overridden at lines 69–72. When the user switches Web pages, the applet container calls the applet’s stop method. This enables the applet to stop playing the audio clip. Otherwise, the audio clip continues to play in the background—even if the applet is not displayed in the browser. This is not really a problem, but it can be annoying to the user if the audio clip is looping. The stop method is provided here as a convenience to the user.
Good Programming Practice 18.1
When playing audio clips in an applet or application, provide a mechanism for the user to disable the audio.
1 // Fig. 18.6: LoadAudioAndPlay.java
2 // Load an audio clip and play it.
3
4 // Java core packages
5 import java.applet.*;
6import java.awt.*;
7 import java.awt.event.*;
8
9 // Java extension packages
10 import javax.swing.*;
Fig. 18.6 Loading and playing an AudioClip (part 1 of 3).
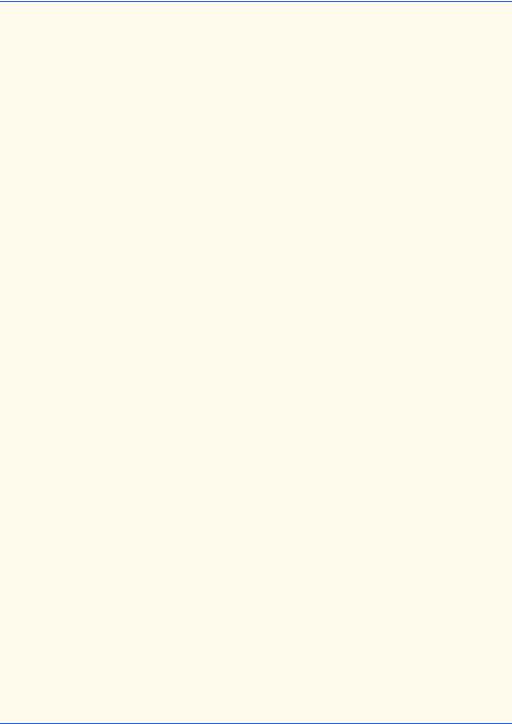
1086 |
Multimedia: Images, Animation, Audio and Video |
Chapter 18 |
11
12public class LoadAudioAndPlay extends JApplet {
13private AudioClip sound1, sound2, currentSound;
14private JButton playSound, loopSound, stopSound;
15private JComboBox chooseSound;
16
17// load the image when the applet begins executing
18public void init()
19{
20Container container = getContentPane();
21container.setLayout( new FlowLayout() );
22
23String choices[] = { "Welcome", "Hi" };
24chooseSound = new JComboBox( choices );
26 |
chooseSound.addItemListener( |
27 |
|
28 |
new ItemListener() { |
29 |
|
30 |
// stop sound and change to sound to user's selection |
31 |
public void itemStateChanged( ItemEvent e ) |
32 |
{ |
33 |
currentSound.stop(); |
34 |
|
35 |
currentSound = |
36 |
chooseSound.getSelectedIndex() == 0 ? |
37 |
sound1 : sound2; |
38 |
} |
39 |
|
40 |
} // end anonymous inner class |
41 |
|
42 |
); // end addItemListener method call |
43 |
|
44 |
container.add( chooseSound ); |
45 |
|
46// set up button event handler and buttons
47ButtonHandler handler = new ButtonHandler();
49playSound = new JButton( "Play" );
50playSound.addActionListener( handler );
51container.add( playSound );
52
53loopSound = new JButton( "Loop" );
54loopSound.addActionListener( handler );
55container.add( loopSound );
56
57stopSound = new JButton( "Stop" );
58stopSound.addActionListener( handler );
59container.add( stopSound );
60
61// load sounds and set currentSound
62sound1 = getAudioClip( getDocumentBase(), "welcome.wav" );
63sound2 = getAudioClip( getDocumentBase(), "hi.au" );
Fig. 18.6 Loading and playing an AudioClip (part 2 of 3).