
- •Contents
- •Preface
- •Introduction to Computers, the Internet and the Web
- •1.3 Computer Organization
- •Languages
- •1.9 Java Class Libraries
- •1.12 The Internet and the World Wide Web
- •1.14 General Notes about Java and This Book
- •Sections
- •Introduction to Java Applications
- •2.4 Displaying Text in a Dialog Box
- •2.5 Another Java Application: Adding Integers
- •2.8 Decision Making: Equality and Relational Operators
- •Introduction to Java Applets
- •3.2 Sample Applets from the Java 2 Software Development Kit
- •3.3 A Simple Java Applet: Drawing a String
- •3.4 Two More Simple Applets: Drawing Strings and Lines
- •3.6 Viewing Applets in a Web Browser
- •3.7 Java Applet Internet and World Wide Web Resources
- •Repetition)
- •Class Attributes
- •5.8 Labeled break and continue Statements
- •5.9 Logical Operators
- •Methods
- •6.2 Program Modules in Java
- •6.7 Java API Packages
- •6.13 Example Using Recursion: The Fibonacci Series
- •6.16 Methods of Class JApplet
- •Class Operations
- •Arrays
- •7.6 Passing Arrays to Methods
- •7.8 Searching Arrays: Linear Search and Binary Search
- •Collaboration Among Objects
- •8.2 Implementing a Time Abstract Data Type with a Class
- •8.3 Class Scope
- •8.4 Controlling Access to Members
- •8.5 Creating Packages
- •8.7 Using Overloaded Constructors
- •8.9 Software Reusability
- •8.10 Final Instance Variables
- •Classes
- •8.16 Data Abstraction and Encapsulation
- •9.2 Superclasses and Subclasses
- •9.5 Constructors and Finalizers in Subclasses
- •Conversion
- •9.11 Type Fields and switch Statements
- •9.14 Abstract Superclasses and Concrete Classes
- •9.17 New Classes and Dynamic Binding
- •9.18 Case Study: Inheriting Interface and Implementation
- •9.19 Case Study: Creating and Using Interfaces
- •9.21 Notes on Inner Class Definitions
- •Strings and Characters
- •10.2 Fundamentals of Characters and Strings
- •10.21 Card Shuffling and Dealing Simulation
- •Handling
- •Graphics and Java2D
- •11.2 Graphics Contexts and Graphics Objects
- •11.5 Drawing Lines, Rectangles and Ovals
- •11.9 Java2D Shapes
- •12.12 Adapter Classes
- •Cases
- •13.3 Creating a Customized Subclass of JPanel
- •Applications
- •Controller
- •Exception Handling
- •14.6 Throwing an Exception
- •14.7 Catching an Exception
- •Multithreading
- •15.3 Thread States: Life Cycle of a Thread
- •15.4 Thread Priorities and Thread Scheduling
- •15.5 Thread Synchronization
- •15.9 Daemon Threads
- •Multithreading
- •Design Patterns
- •Files and Streams
- •16.2 Data Hierarchy
- •16.3 Files and Streams
- •Networking
- •17.2 Manipulating URIs
- •17.3 Reading a File on a Web Server
- •17.4 Establishing a Simple Server Using Stream Sockets
- •17.5 Establishing a Simple Client Using Stream Sockets
- •17.9 Security and the Network
- •18.2 Loading, Displaying and Scaling Images
- •18.3 Animating a Series of Images
- •18.5 Image Maps
- •18.6 Loading and Playing Audio Clips
- •18.7 Internet and World Wide Web Resources
- •Data Structures
- •19.4 Linked Lists
- •20.8 Bit Manipulation and the Bitwise Operators
- •Collections
- •21.8 Maps
- •21.9 Synchronization Wrappers
- •21.10 Unmodifiable Wrappers
- •22.2 Playing Media
- •22.3 Formatting and Saving Captured Media
- •22.5 Java Sound
- •22.8 Internet and World Wide Web Resources
- •Hexadecimal Numbers
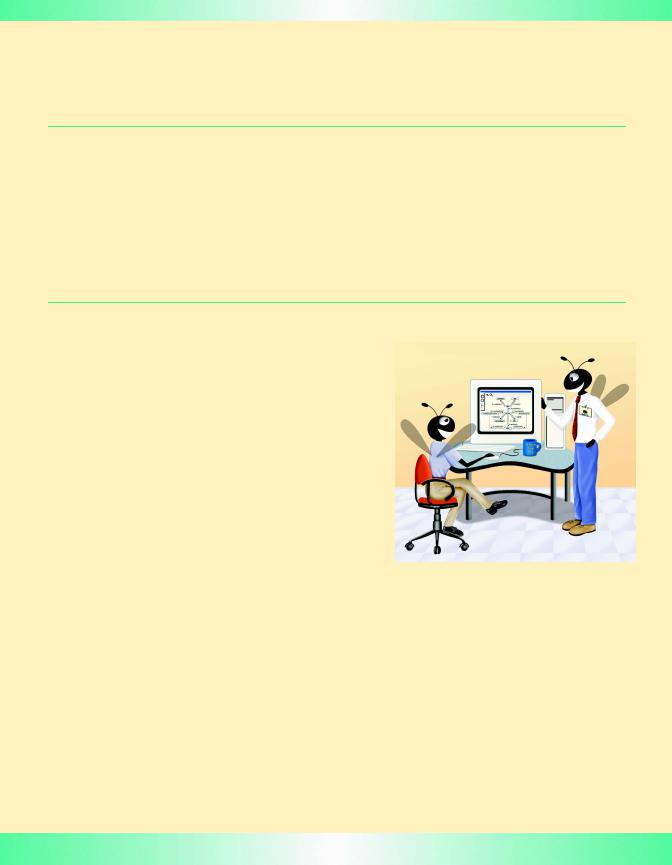
17
Networking
Objectives
•To understand Java networking with URIs, sockets and datagrams.
•To implement Java networking applications by using sockets and datagrams.
•To understand how to implement Java clients and servers that communicate with one another.
•To understand how to implement network-based collaborative applications.
•To construct a multithreaded server.
If the presence of electricity can be made visible in any part of a circuit, I see no reason why intelligence may not be transmitted instantaneously by electricity.
Samuel F. B. Morse
Mr. Watson, come here, I want you.
Alexander Graham Bell
What networks of railroads, highways and canals were in another age, the networks of telecommunications, information and computerization … are today.
Bruno Kreisky, Austrian Chancellor
Science may never come up with a better officecommunication system than the coffee break.
Earl Wilson
It’s currently a problem of access to gigabits through punybaud.
J. C. R. Licklider
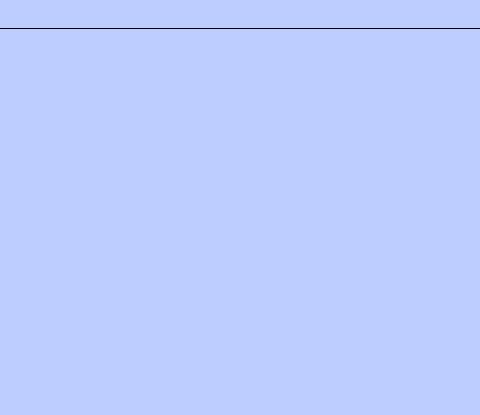
Chapter 17 |
Networking |
979 |
Outline
17.1Introduction
17.2Manipulating URIs
17.3Reading a File on a Web Server
17.4Establishing a Simple Server Using Stream Sockets
17.5Establishing a Simple Client Using Stream Sockets
17.6Client/Server Interaction with Stream Socket Connections
17.7Connectionless Client/Server Interaction with Datagrams
17.8Client/Server Tic-Tac-Toe Using a Multithreaded Server
17.9Security and the Network
17.10DeitelMessenger Chat Server and Client
17.10.1DeitelMessengerServer and Supporting Classes
17.10.2DeitelMessenger Client and Supporting Classes
17.11(Optional) Discovering Design Patterns: Design Patterns Used in Packages java.io and java.net
17.11.1Creational Design Patterns
17.11.2Structural Design Patterns
17.11.3Architectural Patterns
17.11.4Conclusion
Summary • Terminology • Self-Review Exercises • Answers to Self-Review Exercises • Exercises
17.1 Introduction
There is much excitement over the Internet and the World Wide Web. The Internet ties the “information world” together. The World Wide Web makes the Internet easy to use and gives it the flair and sizzle of multimedia. Organizations see the Internet and the Web as crucial to their information-systems strategies. Java provides a number of built-in networking capabilities that make it easy to develop Internet-based and Web-based applications. Not only can Java specify parallelism through multithreading, but it can enable programs to search the world for information and to collaborate with programs running on other computers internationally, nationally or just within an organization. Java can enable applets and applications to communicate with one another (subject to security constraints).
Networking is a massive and complex topic. Computer science and computer engineering students will typically take a full-semester, upper level course in computer networking and continue with further study at the graduate level. Java provides a rich complement of networking capabilities and will likely be used as an implementation vehicle in computer networking courses. In Java How to Program, Fourth Edition, we introduce a portion of Java’s networking concepts and capabilities. For more advanced networking capabilities, refer to our book Advanced Java 2 Platform How to Program.
Java’s networking capabilities are grouped into several packages. The fundamental networking capabilities are defined by classes and interfaces of package java.net,
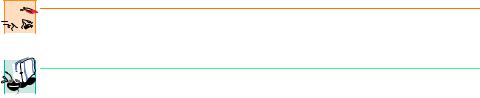
980 |
Networking |
Chapter 17 |
through which Java offers socket-based communications that enable applications to view networking as streams of data. The classes and interfaces of package java.net also offer packet-based communications that enable individual packets of information to be trans- mitted—this is commonly used to transmit audio and video over the Internet. In this chapter, we show how to create and manipulate sockets and how to communicate with packets of data.
Our discussion of networking focuses on both sides of a client-server relationship. The client requests that some action be performed, and the server performs the action and responds to the client. A common implementation of the request-response model is between World Wide Web browsers and World Wide Web servers. When a user selects a Web site to browse through a browser (the client application), a request is sent to the appropriate Web server (the server application). The server normally responds to the client by sending an appropriate HTML Web page.
We demonstrate the Swing GUI component JEditorPane and its ability to render an HTML document downloaded from the World Wide Web. We also introduce Java’s a socket-based communications, which enable applications to view networking as if it were file I/O—a program can read from a socket or write to a socket as simply as reading from a file or writing to a file. We show how to create and manipulate sockets.
Java provides stream sockets and datagram sockets. With stream sockets, a process establishes a connection to another process. While the connection is in place, data flows between the processes in continuous streams. Stream sockets are said to provide a connec- tion-oriented service. The protocol used for transmission is the popular TCP (Transmission Control Protocol).
With datagram sockets, individual packets of information are transmitted. This is not the right protocol for everyday users, because, unlike TCP, the protocol used—UDP, the User Datagram Protocol—is a connectionless service, and it does not guarantee that packets arrive in any particular order. In fact, packets can be lost, can be duplicated and can even arrive out of sequence. So, with UDP, significant extra programming is required on the user’s part to deal with these problems (if the user chooses to do so). UDP is most appropriate for network applications that do not require the error checking and reliability of TCP. Stream sockets and the TCP protocol will be the most desirable for the vast majority of Java programmers.
Performance Tip 17.1
Connectionless services generally offer greater performance, but less reliability than con-
nection-oriented services.
Portability Tip 17.1
The TCP protocol and its related set of protocols enable a great variety of heterogeneous
computer systems (i.e., computer systems with different processors and different operating systems) to intercommunicate.
The chapter ends with a case study in which we implement a client/server chat application similar to the instant-messaging services popular on the Web today. The program incorporates many networking techniques introduced in this chapter. The program also introduces multicasting, in which a server can publish information and clients can subscribe to that information. Each time the server publishes more information, all subscribers receive that information. Throughout the examples of this chapter, we will see that many of the networking details are handled by the Java classes we use.
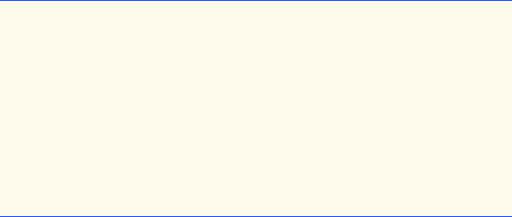
Chapter 17 |
Networking |
981 |
17.2 Manipulating URIs
The Internet offers many protocols. The HyperText Transfer Protocol (HTTP) that forms the basis of the World Wide Web uses URIs (Uniform Resource Identifiers) to locate data on the Internet. URIs frequently are called URLs (Uniform Resource Locators). Actually, a URL is a type of URI. Common URIs refer to files or directories and can reference objects that perform complex tasks, such as database lookups and Internet searches. If you know the URI of publicly available HTML files anywhere on the World Wide Web, you can access that data through HTTP. Java makes it easy to manipulate URIs. Using a URI that refers to the exact location of a resource (such as a Web page) as an argument to the showDocument method of interface AppletContext causes the browser in which the applet is executing to display the resource at the specified URI. The applet of Fig. 17.1 and Fig. 17.2 enables the user to select a Web page from a JList and causes the browser to display the corresponding page.
This applet takes advantage of applet parameters specified in the HTML document that invokes the applet. When browsing the World Wide Web, often you will come across applets that are in the public domain—you can use them free of charge on your own Web pages (normally in exchange for crediting the applet’s creator). One common feature of such applets is the ability to customize the applet via parameters that are supplied from the HTML file that invokes the applet. For example, Fig. 17.1 contains the HTML that invokes the applet SiteSelector in Fig. 17.2. The HTML document contains eight parameters specified with the param element—these lines must appear between the starting and ending applet tags. The applet can read these values and use them to customize itself. Any number of param tags can appear between the starting and ending applet tags. Each parameter has a name and a value. Applet method getParameter retrieves the value associated with a specific parameter and returns the value as a String. The argument passed to getParameter is a String containing the name of the parameter in the param tag. In this example, parameters represent the title of each Web site the user can select and the location of each site. Any number of parameters can be specified. However, these parameters must be named title#, where the value of # starts at 0 and increments by one for each new title. Each title should have a corresponding location parameter of the form location#, where the value of # starts at 0 and increments by one for each new location. The statement
1<html>
2<title>Site Selector</title>
3<body>
4<applet code = "SiteSelector.class" width = "300" height = "75">
5<param name = "title0" value = "Java Home Page">
6 <param name = "location0" value = "http://java.sun.com/">
7<param name = "title1" value = "Deitel">
8 <param name = "location1" value = "http://www.deitel.com/">
9 <param name = "title2" value = "JGuru">
10<param name = "location2" value = "http://www.jGuru.com/">
11<param name = "title3" value = "JavaWorld">
12<param name = "location3" value = "http://www.javaworld.com/">
13</applet>
14</body>
15</html>
Fig. 17.1 HTML document to load SiteSelector applet.

982 |
Networking |
Chapter 17 |
String title = getParameter( "title0" );
gets the value associated with the "title0" parameter and assigns it to String reference title. If there is not a param tag containing the specified parameter, getParameter returns null.
The applet (Fig. 17.2) obtains from the HTML document (Fig. 17.1) the choices that will be displayed in the applet’s JList. Class SiteSelector uses a Hashtable (package java.util) to store the World Wide Web site names and URIs. A Hashtable stores key/ value pairs. The program uses the key to store and retrieve the associated value in the Hashtable. In this example, the key is the String in the JList that represents the Web site name, and the value is a URL object that stores the URI of the Web site to display in the browser. Class Hashtable provides two methods of importance in this example—put and get. Method put takes two arguments—a key and its associated value—and places the value in the Hashtable at a location determined by the key. Method get takes one argu- ment—a key—and retrieves the value (as an Object reference) associated with the key. Class SiteSelector also contains a Vector (package java.util) in which the site names are placed so they can be used to initialize the JList (one version of the JList constructor receives a Vector object). A Vector is a dynamically resizable array of Objects. Class Vector provides method add to add a new element to the end of the Vector. Classes Hashtable and Vector are discussed in detail in Chapter 20.
Lines 23–24 in method init (lines 20–63) create the Hashtable and Vector objects. Line 27 calls our utility method getSitesFromHTMLParameters (lines 66– 108) to obtain the HTML parameters from the HTML document that invoked the applet.
In method getSitesFromHTMLParameters, line 75 uses Applet method getParameter to obtain a Web site title. If the title is not null, the loop at lines 78– 106 begins executing. Line 81 uses Applet method getParameter to obtain the corresponding location. Line 87 uses the location as the initial value of a new URL object. The URL constructor determines whether the String passed as an argument represents a valid Uniform Resource Identifier. If not, the URL constructor throws a MalformedURLException. Notice that the URL constructor must be called in a try block. If the
URL constructor generates a MalformedURLException, the call to printStackTrace (line 98) causes the program to display a stack trace. Then the program attempts to obtain the next Web site title. The program does not add the site for the invalid URI to the Hashtable, so the title will not be displayed in the JList.
Common Programming Error 17.1
A MalformedURLException is thrown when a String that is not in proper URI format
is passed to a URL constructor.
For a proper URL, line 90 places the title and URL into the Hashtable, and line 93 adds the title to the Vector. Line 104 gets the next title from the HTML document. When the call to getParameter at line 104 returns null, the loop terminates.
When method getSitesFromHTMLParameters returns to init, lines 30–61 construct the applet’s GUI. Lines 31–32 add the JLabel “Choose a site to browse” to the NORTH of the content pane’s BorderLayout. Lines 36–58 register an instance of an anonymous inner class that implements ListSelectionListener to handle the siteChooser’s events. Lines 60–61 add siteChooser to the CENTER of the content pane’s BorderLayout.
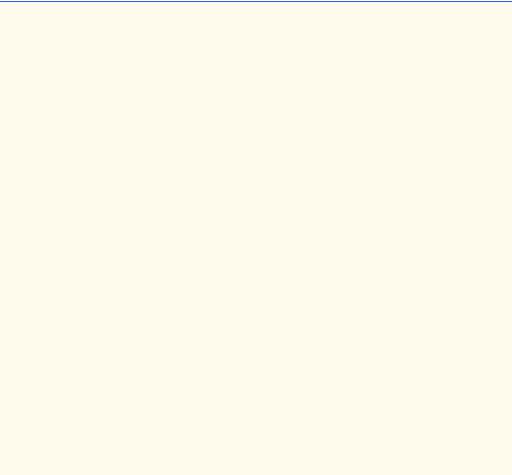
Chapter 17 |
Networking |
983 |
1// Fig. 17.2: SiteSelector.java
2 // This program uses a button to load a document from a URL.
3
4 // Java core packages
5 import java.net.*;
6 import java.util.*;
7import java.awt.*;
8 import java.applet.AppletContext;
9
10// Java extension packages
11import javax.swing.*;
12import javax.swing.event.*;
14 |
public class SiteSelector extends |
JApplet { |
|||
15 |
private Hashtable sites; |
// |
site |
names and URLs |
|
16 |
private |
Vector siteNames; |
// |
site |
names |
17 |
private |
JList siteChooser; |
// list |
of sites to choose from |
|
18 |
|
|
|
|
|
19// read HTML parameters and set up GUI
20public void init()
21{
22// create Hashtable and Vector
23sites = new Hashtable();
24siteNames = new Vector();
25
26// obtain parameters from HTML document
27getSitesFromHTMLParameters();
28
29// create GUI components and layout interface
30Container container = getContentPane();
31container.add( new JLabel( "Choose a site to browse" ),
32 |
BorderLayout.NORTH ); |
33 |
|
34 |
siteChooser = new JList( siteNames ); |
35 |
|
36 |
siteChooser.addListSelectionListener( |
37 |
|
38 |
new ListSelectionListener() { |
39 |
|
40 |
// go to site user selected |
41 |
public void valueChanged( ListSelectionEvent event ) |
42 |
{ |
43 |
// get selected site name |
44 |
Object object = siteChooser.getSelectedValue(); |
45 |
|
46 |
// use site name to locate corresponding URL |
47 |
URL newDocument = ( URL ) sites.get( object ); |
48 |
|
49 |
// get reference to applet container |
50 |
AppletContext browser = getAppletContext(); |
51 |
|
52 |
// tell applet container to change pages |
53 |
browser.showDocument( newDocument ); |
|
|
Fig. 17.2 Loading a document from a URL into a browser (part 1 of 3).
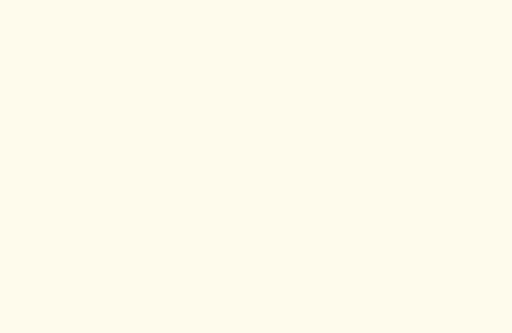
984 |
Networking |
Chapter 17 |
|
|
|
54 |
} |
// end method valueChanged |
55 |
|
|
56 |
} // end anonymous inner class |
|
57 |
|
|
58 |
); // end call to addListSelectionListener |
|
59 |
|
|
60 |
container.add( new JScrollPane( siteChooser ), |
|
61 |
BorderLayout.CENTER ); |
|
62 |
|
|
63 |
} // end method init |
|
64 |
|
|
65// obtain parameters from HTML document
66private void getSitesFromHTMLParameters()
67{
68// look for applet parameters in the HTML document
69// and add sites to Hashtable
70String title, location;
71URL url;
72int counter = 0;
73
74// obtain first site title
75title = getParameter( "title" + counter );
77// loop until no more parameters in HTML document
78while ( title != null ) {
79 |
|
80 |
// obtain site location |
81 |
location = getParameter( "location" + counter ); |
82 |
|
83 |
// place title/URL in Hashtable and title in Vector |
84 |
try { |
85 |
|
86 |
// convert location to URL |
87 |
url = new URL( location ); |
88 |
|
89 |
// put title/URL in Hashtable |
90 |
sites.put( title, url ); |
91 |
|
92 |
// put title in Vector |
93 |
siteNames.add( title ); |
94 |
} |
95 |
|
96 |
// process invalid URL format |
97 |
catch ( MalformedURLException urlException ) { |
98 |
urlException.printStackTrace(); |
99 |
} |
100 |
|
101 |
++counter; |
102 |
|
103 |
// obtain next site title |
104 |
title = getParameter( "title" + counter ); |
105 |
|
106 |
} // end while |
|
|
Fig. 17.2 Loading a document from a URL into a browser (part 2 of 3).
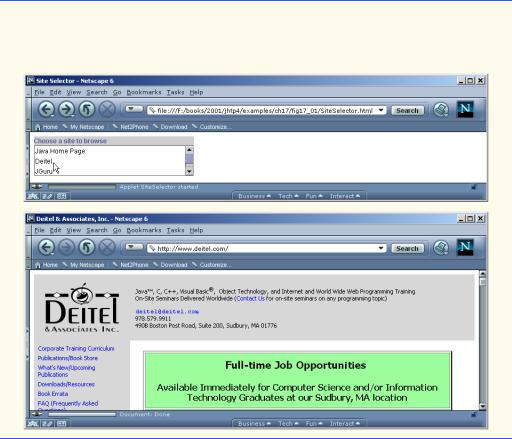
Chapter 17 |
Networking |
985 |
107
108 } // end method getSitesFromHTMLParameters
109
110 } // end class SiteSelector
Fig. 17.2 Loading a document from a URL into a browser (part 3 of 3).
When the user selects one of the Web sites in siteChooser, the program calls method valueChanged (lines 41–54). Line 44 obtains the selected site name from the JList. Line 47 passes the selected site name (the key) to Hashtable method get, which locates and returns an Object reference to the corresponding URL object (the value). The URL cast operator converts the reference to a URL that can be assigned to reference newDocument.
Line 50 uses Applet method getAppletContext to get a reference to an AppletContext object that represents the applet container. Line 53 uses the AppletContext reference browser to invoke AppletContext method showDocument, which receives a URL object as an argument and passes it to the AppletContext (i.e., the browser). The browser displays in the current browser window the World Wide Web resource associated with that URL. In this example, all the resources are HTML documents.
For programmers familiar with HTML frames, there is a second version of AppletContext method showDocument that enables an applet to specify the so-called target frame in which to display the World Wide Web resource. The second version of showDocument takes two arguments—a URL object specifying the resource to display and a String representing the target frame. There are some special target frames that can be used as the second argument. The target frame _blank results in a new Web browser