
- •Contents
- •Preface
- •Introduction to Computers, the Internet and the Web
- •1.3 Computer Organization
- •Languages
- •1.9 Java Class Libraries
- •1.12 The Internet and the World Wide Web
- •1.14 General Notes about Java and This Book
- •Sections
- •Introduction to Java Applications
- •2.4 Displaying Text in a Dialog Box
- •2.5 Another Java Application: Adding Integers
- •2.8 Decision Making: Equality and Relational Operators
- •Introduction to Java Applets
- •3.2 Sample Applets from the Java 2 Software Development Kit
- •3.3 A Simple Java Applet: Drawing a String
- •3.4 Two More Simple Applets: Drawing Strings and Lines
- •3.6 Viewing Applets in a Web Browser
- •3.7 Java Applet Internet and World Wide Web Resources
- •Repetition)
- •Class Attributes
- •5.8 Labeled break and continue Statements
- •5.9 Logical Operators
- •Methods
- •6.2 Program Modules in Java
- •6.7 Java API Packages
- •6.13 Example Using Recursion: The Fibonacci Series
- •6.16 Methods of Class JApplet
- •Class Operations
- •Arrays
- •7.6 Passing Arrays to Methods
- •7.8 Searching Arrays: Linear Search and Binary Search
- •Collaboration Among Objects
- •8.2 Implementing a Time Abstract Data Type with a Class
- •8.3 Class Scope
- •8.4 Controlling Access to Members
- •8.5 Creating Packages
- •8.7 Using Overloaded Constructors
- •8.9 Software Reusability
- •8.10 Final Instance Variables
- •Classes
- •8.16 Data Abstraction and Encapsulation
- •9.2 Superclasses and Subclasses
- •9.5 Constructors and Finalizers in Subclasses
- •Conversion
- •9.11 Type Fields and switch Statements
- •9.14 Abstract Superclasses and Concrete Classes
- •9.17 New Classes and Dynamic Binding
- •9.18 Case Study: Inheriting Interface and Implementation
- •9.19 Case Study: Creating and Using Interfaces
- •9.21 Notes on Inner Class Definitions
- •Strings and Characters
- •10.2 Fundamentals of Characters and Strings
- •10.21 Card Shuffling and Dealing Simulation
- •Handling
- •Graphics and Java2D
- •11.2 Graphics Contexts and Graphics Objects
- •11.5 Drawing Lines, Rectangles and Ovals
- •11.9 Java2D Shapes
- •12.12 Adapter Classes
- •Cases
- •13.3 Creating a Customized Subclass of JPanel
- •Applications
- •Controller
- •Exception Handling
- •14.6 Throwing an Exception
- •14.7 Catching an Exception
- •Multithreading
- •15.3 Thread States: Life Cycle of a Thread
- •15.4 Thread Priorities and Thread Scheduling
- •15.5 Thread Synchronization
- •15.9 Daemon Threads
- •Multithreading
- •Design Patterns
- •Files and Streams
- •16.2 Data Hierarchy
- •16.3 Files and Streams
- •Networking
- •17.2 Manipulating URIs
- •17.3 Reading a File on a Web Server
- •17.4 Establishing a Simple Server Using Stream Sockets
- •17.5 Establishing a Simple Client Using Stream Sockets
- •17.9 Security and the Network
- •18.2 Loading, Displaying and Scaling Images
- •18.3 Animating a Series of Images
- •18.5 Image Maps
- •18.6 Loading and Playing Audio Clips
- •18.7 Internet and World Wide Web Resources
- •Data Structures
- •19.4 Linked Lists
- •20.8 Bit Manipulation and the Bitwise Operators
- •Collections
- •21.8 Maps
- •21.9 Synchronization Wrappers
- •21.10 Unmodifiable Wrappers
- •22.2 Playing Media
- •22.3 Formatting and Saving Captured Media
- •22.5 Java Sound
- •22.8 Internet and World Wide Web Resources
- •Hexadecimal Numbers
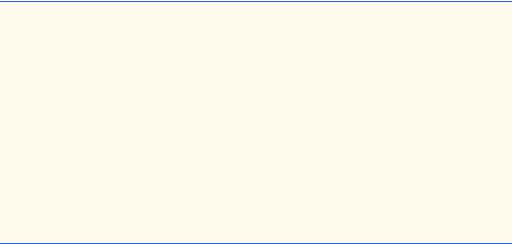
986 |
Networking |
Chapter 17 |
window to display the content from the specified URI. The target frame _self specifies that the content from the specified URI should be displayed in the same frame as the applet (the applet’s HTML page is replaced in this case). The target frame _top specifies that the browser should remove the current frames in the browser window, then display the content from the specified URI in the current window. For more information on HTML and frames, see the World Wide Web Consortium (W3C) Web site
http://www.w3.org
[Note: This applet must be run from a World Wide Web browser, such as Netscape Navigator or Microsoft Internet Explorer, to see the results of displaying another Web page. The appletviewer is capable only of executing applets—it ignores all other HTML tags. If the Web sites in the program contained Java applets, only those applets would appear in the appletviewer when the user selects a Web site. Each applet would execute in a separate appletviewer window. Of the browsers mentioned here, only Netscape Navigator 6 currently supports the features of Java 2. You will need to use the Java Plug-in (discussed in Chapter 3) to execute this applet in Microsoft Internet Explorer or older versions of Netscape Navigator.]
17.3 Reading a File on a Web Server
The application of Fig. 17.3 uses Swing GUI component JEditorPane (from package javax.swing) to display the contents of a file on a Web server. The user inputs the URI in the JTextField at the top of the window, and the program displays the corresponding document (if it exists) in the JEditorPane. Class JEditorPane is able to render both plain text and HTML-formatted text, so this application acts as a simple Web browser. The application also demonstrates how to process HyperlinkEvents when the user clicks a hyperlink in the HTML document. The screen captures in Fig. 17.3 illustrate that the JEditorPane can display both simple text (the first screen) and HTML text (the second screen). The techniques shown in this example also can be used in applets. However, applets are allowed to read files only on the server from which the applet was downloaded.
1// Fig. 17.3: ReadServerFile.java
2 // This program uses a JEditorPane to display the 3 // contents of a file on a Web server.
4
5 // Java core packages
6import java.awt.*;
7 import java.awt.event.*;
8 import java.net.*;
9 import java.io.*;
10
11// Java extension packages
12import javax.swing.*;
13import javax.swing.event.*;
15public class ReadServerFile extends JFrame {
16private JTextField enterField;
17private JEditorPane contentsArea;
Fig. 17.3 Reading a file by opening a connection through a URL (part 1 of 4)
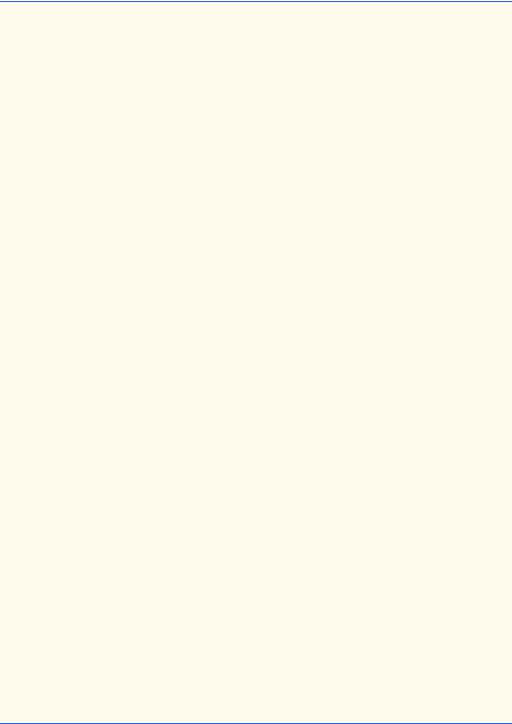
Chapter 17 |
Networking |
987 |
18
19// set up GUI
20public ReadServerFile()
21{
22super( "Simple Web Browser" );
24 Container container = getContentPane();
25
26// create enterField and register its listener
27enterField = new JTextField( "Enter file URL here" );
29 |
enterField.addActionListener( |
30 |
|
31 |
new ActionListener() { |
32 |
|
33 |
// get document specified by user |
34 |
public void actionPerformed( ActionEvent event ) |
35 |
{ |
36 |
getThePage( event.getActionCommand() ); |
37 |
} |
38 |
|
39 |
} // end anonymous inner class |
40 |
|
41 |
); // end call to addActionListener |
42 |
|
43 |
container.add( enterField, BorderLayout.NORTH ); |
44 |
|
45// create contentsArea and register HyperlinkEvent listener
46contentsArea = new JEditorPane();
47contentsArea.setEditable( false );
48 |
|
49 |
contentsArea.addHyperlinkListener( |
50 |
|
51 |
new HyperlinkListener() { |
52 |
|
53 |
// if user clicked hyperlink, go to specified page |
54 |
public void hyperlinkUpdate( HyperlinkEvent event ) |
55 |
{ |
56 |
if ( event.getEventType() == |
57 |
HyperlinkEvent.EventType.ACTIVATED ) |
58 |
getThePage( event.getURL().toString() ); |
59 |
} |
60 |
|
61 |
} // end anonymous inner class |
62 |
|
63 |
); // end call to addHyperlinkListener |
64 |
|
65 |
container.add( new JScrollPane( contentsArea ), |
66 |
BorderLayout.CENTER ); |
67 |
|
68setSize( 400, 300 );
69setVisible( true );
70}
Fig. 17.3 Reading a file by opening a connection through a URL (part 2 of 4)
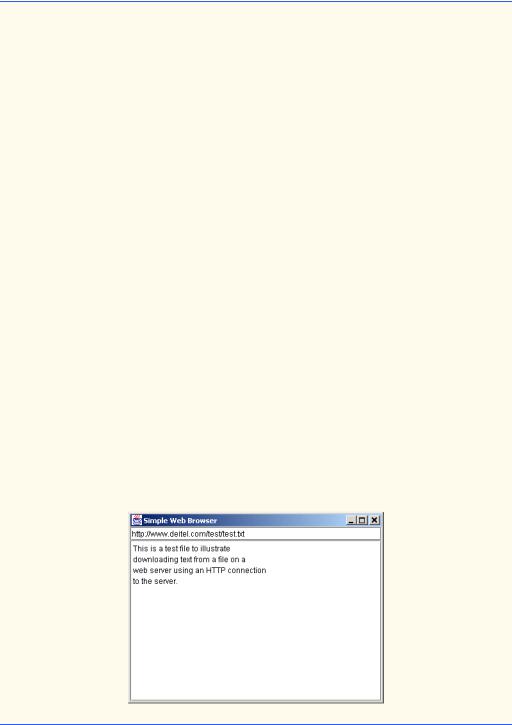
988 |
Networking |
Chapter 17 |
71
72// load document; change mouse cursor to indicate status
73private void getThePage( String location )
74{
75// change mouse cursor to WAIT_CURSOR
76setCursor( Cursor.getPredefinedCursor(
77 |
Cursor.WAIT_CURSOR ) ); |
78 |
|
79// load document into contentsArea and display location in
80// enterField
81try {
82 |
contentsArea.setPage( location ); |
83enterField.setText( location );
84}
85
86// process problems loading document
87catch ( IOException ioException ) {
88 |
JOptionPane.showMessageDialog( this, |
89 |
"Error retrieving specified URL", |
90 |
"Bad URL", JOptionPane.ERROR_MESSAGE ); |
91 |
} |
92 |
|
93 |
setCursor( Cursor.getPredefinedCursor( |
94Cursor.DEFAULT_CURSOR ) );
95}
96
97// begin application execution
98public static void main( String args[] )
99{
100 ReadServerFile application = new ReadServerFile();
101
102 application.setDefaultCloseOperation(
103JFrame.EXIT_ON_CLOSE );
104}
105
106 } // end class ReadServerFile
Fig. 17.3 Reading a file by opening a connection through a URL (part 3 of 4)
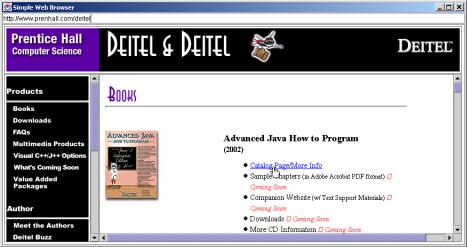
Chapter 17 |
Networking |
989 |
|
|
|
|
|
|
Fig. 17.3 Reading a file by opening a connection through a URL (part 4 of 4)
The application class ReadServerFile contains JTextField enterField, in which the user enters the URI of the file to read and JEditorPane contentsArea to display the contents of the file. When the user presses the Enter key in the JTextField, the program calls method actionPerformed (lines 34–37). Line 36 uses ActionEvent method getActionCommand to get the String the user input in the JTextField and passes that string to utility method getThePage (lines 73–95).
Lines 76–77 in method getThePage use method setCursor (inherited into class JFrame from class Component) to change the mouse cursor to the wait cursor (normally, an hourglass or a watch). If the file being downloaded is large, the wait cursor indicates to the user that the program is performing a task and that the user should wait for the task to complete. The static Cursor method getPredefinedCursor receives an integer indicating the cursor type (Cursor.WAIT_CURSOR in this case). See the API documentation for class Cursor for a complete list of cursors.
Line 82 uses JEditorPane method setPage to download the document specified by location and display it in the JEditorPane contents. If there is an error downloading the document, method setPage throws an IOException. Also, if an invalid URL is specified, a MalformedURLException (a sublcass of IOException) occurs. If the document loads successfully, line 83 displays the current location in enterField.
Lines 93–94 sets the Cursor back to Cursor.DEFAULT_CURSOR (the default Cursor) to indicate that the document download is complete.
Typically, an HTML document contains hyperlinks—text, images or GUI components which, when clicked, provide quick access to another document on the Web. If a JEditorPane contains an HTML document and the user clicks a hyperlink, the JEditorPane generates a HyperlinkEvent (package javax.swing.event) and notifies all registered HyperlinkListeners (package javax.swing.event) of that event. Lines 49–63 register a HyperlinkListener to handle HyperlinkEvents. When a HyperlinkEvent occurs, the program calls method hyperlinkUpdate (lines 54–