
- •Contents
- •Send Us Your Comments
- •Preface
- •What's New in PL/SQL?
- •1 Overview of PL/SQL
- •Advantages of PL/SQL
- •Tight Integration with SQL
- •Support for SQL
- •Better Performance
- •Higher Productivity
- •Full Portability
- •Tight Security
- •Support for Object-Oriented Programming
- •Understanding the Main Features of PL/SQL
- •Block Structure
- •Variables and Constants
- •Processing Queries with PL/SQL
- •Declaring PL/SQL Variables
- •Control Structures
- •Writing Reusable PL/SQL Code
- •Data Abstraction
- •Error Handling
- •PL/SQL Architecture
- •In the Oracle Database Server
- •In Oracle Tools
- •2 Fundamentals of the PL/SQL Language
- •Character Set
- •Lexical Units
- •Delimiters
- •Literals
- •Comments
- •Declarations
- •Using DEFAULT
- •Using NOT NULL
- •Using the %TYPE Attribute
- •Using the %ROWTYPE Attribute
- •Restrictions on Declarations
- •PL/SQL Naming Conventions
- •Scope and Visibility of PL/SQL Identifiers
- •Assigning Values to Variables
- •Assigning Boolean Values
- •Assigning a SQL Query Result to a PL/SQL Variable
- •PL/SQL Expressions and Comparisons
- •Logical Operators
- •Boolean Expressions
- •CASE Expressions
- •Handling Null Values in Comparisons and Conditional Statements
- •Summary of PL/SQL Built-In Functions
- •3 PL/SQL Datatypes
- •PL/SQL Number Types
- •PL/SQL Character and String Types
- •PL/SQL National Character Types
- •PL/SQL LOB Types
- •PL/SQL Boolean Types
- •PL/SQL Date, Time, and Interval Types
- •Datetime and Interval Arithmetic
- •Avoiding Truncation Problems Using Date and Time Subtypes
- •Overview of PL/SQL Subtypes
- •Using Subtypes
- •Converting PL/SQL Datatypes
- •Explicit Conversion
- •Implicit Conversion
- •Choosing Between Implicit and Explicit Conversion
- •DATE Values
- •RAW and LONG RAW Values
- •4 Using PL/SQL Control Structures
- •Overview of PL/SQL Control Structures
- •Testing Conditions: IF and CASE Statements
- •Using the IF-THEN Statement
- •Using the IF-THEN-ELSE Statement
- •Using the IF-THEN-ELSIF Statement
- •Using the CASE Statement
- •Guidelines for PL/SQL Conditional Statements
- •Controlling Loop Iterations: LOOP and EXIT Statements
- •Using the LOOP Statement
- •Using the EXIT Statement
- •Using the EXIT-WHEN Statement
- •Labeling a PL/SQL Loop
- •Using the WHILE-LOOP Statement
- •Using the FOR-LOOP Statement
- •Sequential Control: GOTO and NULL Statements
- •Using the GOTO Statement
- •Using the NULL Statement
- •5 Using PL/SQL Collections and Records
- •What Is a Collection?
- •Understanding Nested Tables
- •Understanding Varrays
- •Understanding Associative Arrays (Index-By Tables)
- •How Globalization Settings Affect VARCHAR2 Keys for Associative Arrays
- •Choosing Which PL/SQL Collection Types to Use
- •Choosing Between Nested Tables and Associative Arrays
- •Choosing Between Nested Tables and Varrays
- •Defining Collection Types
- •Declaring PL/SQL Collection Variables
- •Initializing and Referencing Collections
- •Referencing Collection Elements
- •Assigning Collections
- •Comparing Collections
- •Using PL/SQL Collections with SQL Statements
- •Using PL/SQL Varrays with INSERT, UPDATE, and SELECT Statements
- •Manipulating Individual Collection Elements with SQL
- •Using Multilevel Collections
- •Using Collection Methods
- •Checking If a Collection Element Exists (EXISTS Method)
- •Counting the Elements in a Collection (COUNT Method)
- •Checking the Maximum Size of a Collection (LIMIT Method)
- •Finding the First or Last Collection Element (FIRST and LAST Methods)
- •Looping Through Collection Elements (PRIOR and NEXT Methods)
- •Increasing the Size of a Collection (EXTEND Method)
- •Decreasing the Size of a Collection (TRIM Method)
- •Deleting Collection Elements (DELETE Method)
- •Applying Methods to Collection Parameters
- •Avoiding Collection Exceptions
- •What Is a PL/SQL Record?
- •Using Records as Procedure Parameters and Function Return Values
- •Assigning Values to Records
- •Comparing Records
- •Inserting PL/SQL Records into the Database
- •Updating the Database with PL/SQL Record Values
- •Restrictions on Record Inserts/Updates
- •Querying Data into Collections of Records
- •6 Performing SQL Operations from PL/SQL
- •Overview of SQL Support in PL/SQL
- •Data Manipulation
- •Transaction Control
- •SQL Functions
- •SQL Pseudocolumns
- •SQL Operators
- •Performing DML Operations from PL/SQL (INSERT, UPDATE, and DELETE)
- •Overview of Implicit Cursor Attributes
- •Using PL/SQL Records in SQL INSERT and UPDATE Statements
- •Issuing Queries from PL/SQL
- •Selecting At Most One Row: SELECT INTO Statement
- •Selecting Multiple Rows: BULK COLLECT Clause
- •Looping Through Multiple Rows: Cursor FOR Loop
- •Performing Complicated Query Processing: Explicit Cursors
- •Querying Data with PL/SQL
- •Querying Data with PL/SQL: Implicit Cursor FOR Loop
- •Querying Data with PL/SQL: Explicit Cursor FOR Loops
- •Overview of Explicit Cursors
- •Using Subqueries
- •Using Correlated Subqueries
- •Writing Maintainable PL/SQL Queries
- •Using Cursor Attributes
- •Overview of Explicit Cursor Attributes
- •Using Cursor Variables (REF CURSORs)
- •What Are Cursor Variables (REF CURSORs)?
- •Why Use Cursor Variables?
- •Declaring REF CURSOR Types and Cursor Variables
- •Controlling Cursor Variables: OPEN-FOR, FETCH, and CLOSE
- •Avoiding Errors with Cursor Variables
- •Restrictions on Cursor Variables
- •Using Cursor Expressions
- •Restrictions on Cursor Expressions
- •Example of Cursor Expressions
- •Constructing REF CURSORs with Cursor Subqueries
- •Overview of Transaction Processing in PL/SQL
- •Using COMMIT, SAVEPOINT, and ROLLBACK in PL/SQL
- •How Oracle Does Implicit Rollbacks
- •Ending Transactions
- •Setting Transaction Properties with SET TRANSACTION
- •Overriding Default Locking
- •Doing Independent Units of Work with Autonomous Transactions
- •Advantages of Autonomous Transactions
- •Controlling Autonomous Transactions
- •Using Autonomous Triggers
- •Calling Autonomous Functions from SQL
- •7 Performing SQL Operations with Native Dynamic SQL
- •What Is Dynamic SQL?
- •Why Use Dynamic SQL?
- •Using the EXECUTE IMMEDIATE Statement
- •Specifying Parameter Modes for Bind Variables in Dynamic SQL Strings
- •Building a Dynamic Query with Dynamic SQL
- •Examples of Dynamic SQL for Records, Objects, and Collections
- •Using Bulk Dynamic SQL
- •Using Dynamic SQL with Bulk SQL
- •Examples of Dynamic Bulk Binds
- •Guidelines for Dynamic SQL
- •When to Use or Omit the Semicolon with Dynamic SQL
- •Improving Performance of Dynamic SQL with Bind Variables
- •Passing Schema Object Names As Parameters
- •Using Duplicate Placeholders with Dynamic SQL
- •Using Cursor Attributes with Dynamic SQL
- •Passing Nulls to Dynamic SQL
- •Using Database Links with Dynamic SQL
- •Using Invoker Rights with Dynamic SQL
- •Using Pragma RESTRICT_REFERENCES with Dynamic SQL
- •Avoiding Deadlocks with Dynamic SQL
- •Backward Compatibility of the USING Clause
- •8 Using PL/SQL Subprograms
- •What Are Subprograms?
- •Advantages of PL/SQL Subprograms
- •Understanding PL/SQL Procedures
- •Understanding PL/SQL Functions
- •Using the RETURN Statement
- •Declaring Nested PL/SQL Subprograms
- •Passing Parameters to PL/SQL Subprograms
- •Actual Versus Formal Subprogram Parameters
- •Using Positional, Named, or Mixed Notation for Subprogram Parameters
- •Specifying Subprogram Parameter Modes
- •Using Default Values for Subprogram Parameters
- •Overloading Subprogram Names
- •Guidelines for Overloading with Numeric Types
- •Restrictions on Overloading
- •How Subprogram Calls Are Resolved
- •How Overloading Works with Inheritance
- •Using Invoker's Rights Versus Definer's Rights (AUTHID Clause)
- •Advantages of Invoker's Rights
- •Specifying the Privileges for a Subprogram with the AUTHID Clause
- •Who Is the Current User During Subprogram Execution?
- •How External References Are Resolved in Invoker's Rights Subprograms
- •Overriding Default Name Resolution in Invoker's Rights Subprograms
- •Granting Privileges on Invoker's Rights Subprograms
- •Using Roles with Invoker's Rights Subprograms
- •Using Views and Database Triggers with Invoker's Rights Subprograms
- •Using Database Links with Invoker's Rights Subprograms
- •Using Object Types with Invoker's Rights Subprograms
- •Using Recursion with PL/SQL
- •What Is a Recursive Subprogram?
- •Calling External Subprograms
- •Creating Dynamic Web Pages with PL/SQL Server Pages
- •Controlling Side Effects of PL/SQL Subprograms
- •Understanding Subprogram Parameter Aliasing
- •9 Using PL/SQL Packages
- •What Is a PL/SQL Package?
- •What Goes In a PL/SQL Package?
- •Example of a PL/SQL Package
- •Advantages of PL/SQL Packages
- •Understanding The Package Specification
- •Referencing Package Contents
- •Understanding The Package Body
- •Some Examples of Package Features
- •Private Versus Public Items in Packages
- •Overloading Packaged Subprograms
- •How Package STANDARD Defines the PL/SQL Environment
- •About the DBMS_ALERT Package
- •About the DBMS_OUTPUT Package
- •About the DBMS_PIPE Package
- •About the UTL_FILE Package
- •About the UTL_HTTP Package
- •Guidelines for Writing Packages
- •Separating Cursor Specs and Bodies with Packages
- •10 Handling PL/SQL Errors
- •Overview of PL/SQL Runtime Error Handling
- •Guidelines for Avoiding and Handling PL/SQL Errors and Exceptions
- •Advantages of PL/SQL Exceptions
- •Summary of Predefined PL/SQL Exceptions
- •Defining Your Own PL/SQL Exceptions
- •Declaring PL/SQL Exceptions
- •Scope Rules for PL/SQL Exceptions
- •Associating a PL/SQL Exception with a Number: Pragma EXCEPTION_INIT
- •How PL/SQL Exceptions Are Raised
- •Raising Exceptions with the RAISE Statement
- •How PL/SQL Exceptions Propagate
- •Reraising a PL/SQL Exception
- •Handling Raised PL/SQL Exceptions
- •Handling Exceptions Raised in Declarations
- •Handling Exceptions Raised in Handlers
- •Branching to or from an Exception Handler
- •Retrieving the Error Code and Error Message: SQLCODE and SQLERRM
- •Catching Unhandled Exceptions
- •Tips for Handling PL/SQL Errors
- •Continuing after an Exception Is Raised
- •Retrying a Transaction
- •Using Locator Variables to Identify Exception Locations
- •Overview of PL/SQL Compile-Time Warnings
- •PL/SQL Warning Categories
- •Controlling PL/SQL Warning Messages
- •Using the DBMS_WARNING Package
- •11 Tuning PL/SQL Applications for Performance
- •How PL/SQL Optimizes Your Programs
- •When to Tune PL/SQL Code
- •Guidelines for Avoiding PL/SQL Performance Problems
- •Avoiding CPU Overhead in PL/SQL Code
- •Avoiding Memory Overhead in PL/SQL Code
- •Profiling and Tracing PL/SQL Programs
- •Using The Trace API: Package DBMS_TRACE
- •Reducing Loop Overhead for DML Statements and Queries (FORALL, BULK COLLECT)
- •Using the FORALL Statement
- •Retrieving Query Results into Collections with the BULK COLLECT Clause
- •Writing Computation-Intensive Programs in PL/SQL
- •Tuning Dynamic SQL with EXECUTE IMMEDIATE and Cursor Variables
- •Tuning PL/SQL Procedure Calls with the NOCOPY Compiler Hint
- •Restrictions on NOCOPY
- •Compiling PL/SQL Code for Native Execution
- •Setting Up Transformation Pipelines with Table Functions
- •Overview of Table Functions
- •Using Pipelined Table Functions for Transformations
- •Writing a Pipelined Table Function
- •Returning Results from Table Functions
- •Pipelining Data Between PL/SQL Table Functions
- •Querying Table Functions
- •Optimizing Multiple Calls to Table Functions
- •Fetching from the Results of Table Functions
- •Passing Data with Cursor Variables
- •Performing DML Operations Inside Table Functions
- •Performing DML Operations on Table Functions
- •Handling Exceptions in Table Functions
- •12 Using PL/SQL Object Types
- •Overview of PL/SQL Object Types
- •What Is an Object Type?
- •Why Use Object Types?
- •Structure of an Object Type
- •Components of an Object Type
- •What Languages can I Use for Methods of Object Types?
- •How Object Types Handle the SELF Parameter
- •Overloading
- •Changing Attributes and Methods of an Existing Object Type (Type Evolution)
- •Defining Object Types
- •Overview of PL/SQL Type Inheritance
- •Declaring and Initializing Objects
- •Declaring Objects
- •Initializing Objects
- •How PL/SQL Treats Uninitialized Objects
- •Accessing Object Attributes
- •Defining Object Constructors
- •Calling Object Constructors
- •Calling Object Methods
- •Sharing Objects through the REF Modifier
- •Manipulating Objects through SQL
- •Selecting Objects
- •Inserting Objects
- •Updating Objects
- •Deleting Objects
- •13 PL/SQL Language Elements
- •Assignment Statement
- •AUTONOMOUS_TRANSACTION Pragma
- •Blocks
- •CASE Statement
- •CLOSE Statement
- •Collection Methods
- •Collections
- •Comments
- •COMMIT Statement
- •Constants and Variables
- •Cursor Attributes
- •Cursor Variables
- •Cursors
- •DELETE Statement
- •EXCEPTION_INIT Pragma
- •Exceptions
- •EXECUTE IMMEDIATE Statement
- •EXIT Statement
- •Expressions
- •FETCH Statement
- •FORALL Statement
- •Functions
- •GOTO Statement
- •IF Statement
- •INSERT Statement
- •Literals
- •LOCK TABLE Statement
- •LOOP Statements
- •MERGE Statement
- •NULL Statement
- •Object Types
- •OPEN Statement
- •OPEN-FOR Statement
- •OPEN-FOR-USING Statement
- •Packages
- •Procedures
- •RAISE Statement
- •Records
- •RESTRICT_REFERENCES Pragma
- •RETURN Statement
- •ROLLBACK Statement
- •%ROWTYPE Attribute
- •SAVEPOINT Statement
- •SCN_TO_TIMESTAMP Function
- •SELECT INTO Statement
- •SERIALLY_REUSABLE Pragma
- •SET TRANSACTION Statement
- •SQL Cursor
- •SQLCODE Function
- •SQLERRM Function
- •TIMESTAMP_TO_SCN Function
- •%TYPE Attribute
- •UPDATE Statement
- •Where to Find PL/SQL Sample Programs
- •Exercises for the Reader
- •Assigning Character Values
- •Comparing Character Values
- •Inserting Character Values
- •Selecting Character Values
- •Advantages of Wrapping PL/SQL Procedures
- •Running the PL/SQL Wrap Utility
- •Input and Output Files for the PL/SQL Wrap Utility
- •Limitations of the PL/SQL Wrap Utility
- •What Is Name Resolution?
- •Examples of Qualified Names and Dot Notation
- •Differences in Name Resolution Between SQL and PL/SQL
- •Understanding Capture
- •Inner Capture
- •Same-Scope Capture
- •Outer Capture
- •Avoiding Inner Capture in DML Statements
- •Qualifying References to Object Attributes and Methods
- •Calling Parameterless Subprograms and Methods
- •Name Resolution for SQL Versus PL/SQL
- •When Should I Use Bind Variables with PL/SQL?
- •When Do I Use or Omit the Semicolon with Dynamic SQL?
- •How Can I Use Regular Expressions with PL/SQL?
- •How Do I Continue After a PL/SQL Exception?
- •How Do I Pass a Result Set from PL/SQL to Java or Visual Basic (VB)?
- •How Do I Specify Different Kinds of Names with PL/SQL's Dot Notation?
- •What Can I Do with Objects and Object Types in PL/SQL?
- •How Do I Create a PL/SQL Procedure?
- •How Do I Input or Output Data with PL/SQL?
- •How Do I Perform a Case-Insensitive Query?
- •Index
- •Symbols
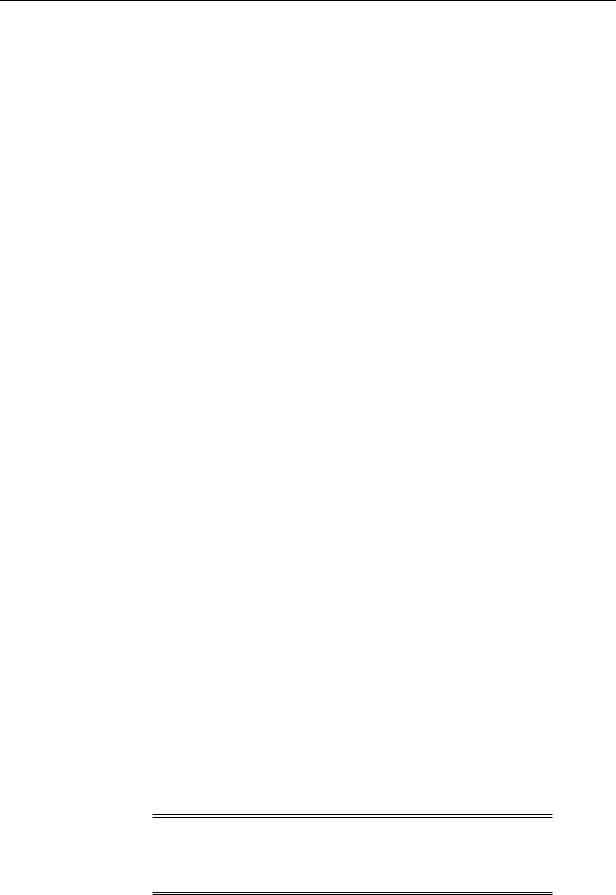
Setting Up Transformation Pipelines with Table Functions
In the example, the PIPE ROW(out_rec) statement pipelines data out of the PL/SQL table function. out_rec is a record, and its type matches the type of an element of the output collection.
The PIPE ROW statement may be used only in the body of pipelined table functions; an error is raised if it is used anywhere else. The PIPE ROW statement can be omitted for a pipelined table function that returns no rows.
A pipelined table function must have a RETURN statement that does not return a value. The RETURN statement transfers the control back to the consumer and ensures that the next fetch gets a NO_DATA_FOUND exception.
Because table functions pass control back and forth to a calling routine as rows areproduced, there is a restriction on combining table functions and PRAGMA AUTONOMOUS_TRANSACTION. If a table function is part of an autonomous transaction, it must COMMIT or ROLLBACK before each PIPE ROW statement, to avoid an error in the calling subprogram.
Oracle has three special SQL datatypes that enable you to dynamically encapsulate and access type descriptions, data instances, and sets of data instances of any other SQL type, including object and collection types. You can also use these three special types to create anonymous (that is, unnamed) types, including anonymous collection types. The types are SYS.ANYTYPE, SYS.ANYDATA, and SYS.ANYDATASET. The
SYS.ANYDATA type can be useful in some situations as a return value from table functions.
See Also: PL/SQL Packages and Types Reference for information about the interfaces to the ANYTYPE, ANYDATA, and ANYDATASET types and about the DBMS_TYPES package for use with these types.
Pipelining Data Between PL/SQL Table Functions
With serial execution, results are pipelined from one PL/SQL table function to another using an approach similar to co-routine execution. For example, the following statement pipelines results from function g to function f:
SELECT * FROM TABLE(f(CURSOR(SELECT * FROM TABLE(g()))));
Parallel execution works similarly except that each function executes in a different process (or set of processes).
Querying Table Functions
Pipelined table functions are used in the FROM clause of SELECT statements. The result rows are retrieved by Oracle iteratively from the table function implementation. For example:
SELECT x.Ticker, x.Price
FROM TABLE(StockPivot( CURSOR(SELECT * FROM StockTable))) x
WHERE x.PriceType='C';
Note: A table function returns a collection. In some cases, such as when the top-level query uses SELECT * and the query refers to a PL/SQL variable or a bind variable, you may need a CAST operator around the table function to specify the exact return type.
11-32 PL/SQL User's Guide and Reference

Setting Up Transformation Pipelines with Table Functions
Optimizing Multiple Calls to Table Functions
Multiple invocations of a table function, either within the same query or in separate queries result in multiple executions of the underlying implementation. By default, there is no buffering or reuse of rows.
For example,
SELECT * FROM TABLE(f(...)) t1, TABLE(f(...)) t2
WHERE t1.id = t2.id;
SELECT * FROM TABLE(f());
SELECT * FROM TABLE(f());
If the function always produces the same result value for each combination of values passed in, you can declare the function DETERMINISTIC, and Oracle automatically buffers rows for it. If the function is not really deterministic, results are unpredictable.
Fetching from the Results of Table Functions
PL/SQL cursors and ref cursors can be defined for queries over table functions. For example:
OPEN c FOR SELECT * FROM TABLE(f(...));
Cursors over table functions have the same fetch semantics as ordinary cursors. REF CURSOR assignments based on table functions do not have any special semantics.
However, the SQL optimizer will not optimize across PL/SQL statements. For example:
DECLARE
r SYS_REFCURSOR; BEGIN
OPEN r FOR SELECT * FROM TABLE(f(CURSOR(SELECT * FROM tab))); SELECT * BULK COLLECT INTO rec_tab FROM TABLE(g(r));
END;
/
does not execute as well as:
SELECT * FROM TABLE(g(CURSOR(SELECT * FROM
TABLE(f(CURSOR(SELECT * FROM tab))))));
This is so even ignoring the overhead associated with executing two SQL statements and assuming that the results can be pipelined between the two statements.
Passing Data with Cursor Variables
You can pass a set of rows to a PL/SQL function in a REF CURSOR parameter. For example, this function is declared to accept an argument of the predefined weakly typed REF CURSOR type SYS_REFCURSOR:
FUNCTION f(p1 IN SYS_REFCURSOR) RETURN ... ;
Results of a subquery can be passed to a function directly:
SELECT * FROM TABLE(f(CURSOR(SELECT empno FROM tab)));
In the example above, the CURSOR keyword is required to indicate that the results of a subquery should be passed as a REF CURSOR parameter.
Tuning PL/SQL Applications for Performance 11-33

Setting Up Transformation Pipelines with Table Functions
A predefined weak REF CURSOR type SYS_REFCURSOR is also supported. With SYS_REFCURSOR, you do not need to first create a REF CURSOR type in a package before you can use it.
To use a strong REF CURSOR type, you still must create a PL/SQL package and declare a strong REF CURSOR type in it. Also, if you are using a strong REF CURSOR type as an argument to a table function, then the actual type of the REF CURSOR argument must match the column type, or an error is generated. Weak REF CURSOR arguments to table functions can only be partitioned using the PARTITION BY ANY clause. You cannot use range or hash partitioning for weak REF CURSOR arguments.
Example 11–11 Example: Using Multiple REF CURSOR Input Variables
PL/SQL functions can accept multiple REF CURSOR input variables:
CREATE FUNCTION g(p1 pkg.refcur_t1, p2 pkg.refcur_t2) RETURN...
PIPELINED ... ;
/
Function g can be invoked as follows:
SELECT * FROM TABLE(g(CURSOR(SELECT employee_id FROM tab),
CURSOR(SELECT * FROM employees));
You can pass table function return values to other table functions by creating a REF CURSOR that iterates over the returned data:
SELECT * FROM TABLE(f(CURSOR(SELECT * FROM TABLE(g(...)))));
Example 11–12 Example: Explicitly Opening a REF CURSOR for a Query
You can explicitly open a REF CURSOR for a query and pass it as a parameter to a table function:
DECLARE
r SYS_REFCURSOR; rec ...;
BEGIN
OPEN r FOR SELECT * FROM TABLE(f(...)); -- Must return a single row result set. SELECT * INTO rec FROM TABLE(g(r));
END;
/
In this case, the table function closes the cursor when it completes, so your program should not explicitly try to close the cursor.
Example 11–13 Example: Using a Pipelined Table Function as an Aggregate Function
A table function can compute aggregate results using the input ref cursor. The following example computes a weighted average by iterating over a set of input rows.
DROP TABLE gradereport;
CREATE TABLE gradereport (student VARCHAR2(30), subject VARCHAR2(30), weight NUMBER, grade NUMBER);
INSERT INTO gradereport VALUES('Mark', 'Physics', 4, 4);
INSERT INTO gradereport VALUES('Mark','Chemistry', 4,3);
INSERT INTO gradereport VALUES('Mark','Maths', 3,3);
INSERT INTO gradereport VALUES('Mark','Economics', 3,4);
11-34 PL/SQL User's Guide and Reference

Setting Up Transformation Pipelines with Table Functions
CREATE OR replace TYPE gpa AS TABLE OF NUMBER;
/
CREATE OR replace FUNCTION weighted_average(input_values sys_refcursor)
RETURN gpa PIPELINED IS grade NUMBER;
total NUMBER := 0; total_weight NUMBER := 0; weight NUMBER := 0;
BEGIN
--The function accepts a ref cursor and loops through all the input rows. LOOP
FETCH input_values INTO weight, grade; EXIT WHEN input_values%NOTFOUND;
--Accumulate the weighted average.
total_weight := total_weight + weight; total := total + grade*weight;
END LOOP;
PIPE ROW (total / total_weight);
-- The function returns a single result. RETURN;
END;
/
show errors;
--The result comes back as a nested table with a single row.
--COLUMN_VALUE is a keyword that returns the contents of a nested table. select weighted_result.column_value from
table( weighted_average( cursor(select weight,grade from gradereport) ) ) weighted_result;
Performing DML Operations Inside Table Functions
To execute DML statements, declare a table function with the AUTONOMOUS_TRANSACTION pragma, which causes the function to execute in a new transaction not shared by other processes:
CREATE FUNCTION f(p SYS_REFCURSOR) return CollType PIPELINED IS PRAGMA AUTONOMOUS_TRANSACTION;
BEGIN NULL; END;
/
During parallel execution, each instance of the table function creates an independent transaction.
Performing DML Operations on Table Functions
Table functions cannot be the target table in UPDATE, INSERT, or DELETE statements. For example, the following statements will raise an error:
UPDATE F(CURSOR(SELECT * FROM tab)) SET col = value;
INSERT INTO f(...) VALUES ('any', 'thing');
However, you can create a view over a table function and use INSTEAD OF triggers to update it. For example:
CREATE VIEW BookTable AS
SELECT x.Name, x.Author
Tuning PL/SQL Applications for Performance 11-35