
- •Contents
- •Send Us Your Comments
- •Preface
- •What's New in PL/SQL?
- •1 Overview of PL/SQL
- •Advantages of PL/SQL
- •Tight Integration with SQL
- •Support for SQL
- •Better Performance
- •Higher Productivity
- •Full Portability
- •Tight Security
- •Support for Object-Oriented Programming
- •Understanding the Main Features of PL/SQL
- •Block Structure
- •Variables and Constants
- •Processing Queries with PL/SQL
- •Declaring PL/SQL Variables
- •Control Structures
- •Writing Reusable PL/SQL Code
- •Data Abstraction
- •Error Handling
- •PL/SQL Architecture
- •In the Oracle Database Server
- •In Oracle Tools
- •2 Fundamentals of the PL/SQL Language
- •Character Set
- •Lexical Units
- •Delimiters
- •Literals
- •Comments
- •Declarations
- •Using DEFAULT
- •Using NOT NULL
- •Using the %TYPE Attribute
- •Using the %ROWTYPE Attribute
- •Restrictions on Declarations
- •PL/SQL Naming Conventions
- •Scope and Visibility of PL/SQL Identifiers
- •Assigning Values to Variables
- •Assigning Boolean Values
- •Assigning a SQL Query Result to a PL/SQL Variable
- •PL/SQL Expressions and Comparisons
- •Logical Operators
- •Boolean Expressions
- •CASE Expressions
- •Handling Null Values in Comparisons and Conditional Statements
- •Summary of PL/SQL Built-In Functions
- •3 PL/SQL Datatypes
- •PL/SQL Number Types
- •PL/SQL Character and String Types
- •PL/SQL National Character Types
- •PL/SQL LOB Types
- •PL/SQL Boolean Types
- •PL/SQL Date, Time, and Interval Types
- •Datetime and Interval Arithmetic
- •Avoiding Truncation Problems Using Date and Time Subtypes
- •Overview of PL/SQL Subtypes
- •Using Subtypes
- •Converting PL/SQL Datatypes
- •Explicit Conversion
- •Implicit Conversion
- •Choosing Between Implicit and Explicit Conversion
- •DATE Values
- •RAW and LONG RAW Values
- •4 Using PL/SQL Control Structures
- •Overview of PL/SQL Control Structures
- •Testing Conditions: IF and CASE Statements
- •Using the IF-THEN Statement
- •Using the IF-THEN-ELSE Statement
- •Using the IF-THEN-ELSIF Statement
- •Using the CASE Statement
- •Guidelines for PL/SQL Conditional Statements
- •Controlling Loop Iterations: LOOP and EXIT Statements
- •Using the LOOP Statement
- •Using the EXIT Statement
- •Using the EXIT-WHEN Statement
- •Labeling a PL/SQL Loop
- •Using the WHILE-LOOP Statement
- •Using the FOR-LOOP Statement
- •Sequential Control: GOTO and NULL Statements
- •Using the GOTO Statement
- •Using the NULL Statement
- •5 Using PL/SQL Collections and Records
- •What Is a Collection?
- •Understanding Nested Tables
- •Understanding Varrays
- •Understanding Associative Arrays (Index-By Tables)
- •How Globalization Settings Affect VARCHAR2 Keys for Associative Arrays
- •Choosing Which PL/SQL Collection Types to Use
- •Choosing Between Nested Tables and Associative Arrays
- •Choosing Between Nested Tables and Varrays
- •Defining Collection Types
- •Declaring PL/SQL Collection Variables
- •Initializing and Referencing Collections
- •Referencing Collection Elements
- •Assigning Collections
- •Comparing Collections
- •Using PL/SQL Collections with SQL Statements
- •Using PL/SQL Varrays with INSERT, UPDATE, and SELECT Statements
- •Manipulating Individual Collection Elements with SQL
- •Using Multilevel Collections
- •Using Collection Methods
- •Checking If a Collection Element Exists (EXISTS Method)
- •Counting the Elements in a Collection (COUNT Method)
- •Checking the Maximum Size of a Collection (LIMIT Method)
- •Finding the First or Last Collection Element (FIRST and LAST Methods)
- •Looping Through Collection Elements (PRIOR and NEXT Methods)
- •Increasing the Size of a Collection (EXTEND Method)
- •Decreasing the Size of a Collection (TRIM Method)
- •Deleting Collection Elements (DELETE Method)
- •Applying Methods to Collection Parameters
- •Avoiding Collection Exceptions
- •What Is a PL/SQL Record?
- •Using Records as Procedure Parameters and Function Return Values
- •Assigning Values to Records
- •Comparing Records
- •Inserting PL/SQL Records into the Database
- •Updating the Database with PL/SQL Record Values
- •Restrictions on Record Inserts/Updates
- •Querying Data into Collections of Records
- •6 Performing SQL Operations from PL/SQL
- •Overview of SQL Support in PL/SQL
- •Data Manipulation
- •Transaction Control
- •SQL Functions
- •SQL Pseudocolumns
- •SQL Operators
- •Performing DML Operations from PL/SQL (INSERT, UPDATE, and DELETE)
- •Overview of Implicit Cursor Attributes
- •Using PL/SQL Records in SQL INSERT and UPDATE Statements
- •Issuing Queries from PL/SQL
- •Selecting At Most One Row: SELECT INTO Statement
- •Selecting Multiple Rows: BULK COLLECT Clause
- •Looping Through Multiple Rows: Cursor FOR Loop
- •Performing Complicated Query Processing: Explicit Cursors
- •Querying Data with PL/SQL
- •Querying Data with PL/SQL: Implicit Cursor FOR Loop
- •Querying Data with PL/SQL: Explicit Cursor FOR Loops
- •Overview of Explicit Cursors
- •Using Subqueries
- •Using Correlated Subqueries
- •Writing Maintainable PL/SQL Queries
- •Using Cursor Attributes
- •Overview of Explicit Cursor Attributes
- •Using Cursor Variables (REF CURSORs)
- •What Are Cursor Variables (REF CURSORs)?
- •Why Use Cursor Variables?
- •Declaring REF CURSOR Types and Cursor Variables
- •Controlling Cursor Variables: OPEN-FOR, FETCH, and CLOSE
- •Avoiding Errors with Cursor Variables
- •Restrictions on Cursor Variables
- •Using Cursor Expressions
- •Restrictions on Cursor Expressions
- •Example of Cursor Expressions
- •Constructing REF CURSORs with Cursor Subqueries
- •Overview of Transaction Processing in PL/SQL
- •Using COMMIT, SAVEPOINT, and ROLLBACK in PL/SQL
- •How Oracle Does Implicit Rollbacks
- •Ending Transactions
- •Setting Transaction Properties with SET TRANSACTION
- •Overriding Default Locking
- •Doing Independent Units of Work with Autonomous Transactions
- •Advantages of Autonomous Transactions
- •Controlling Autonomous Transactions
- •Using Autonomous Triggers
- •Calling Autonomous Functions from SQL
- •7 Performing SQL Operations with Native Dynamic SQL
- •What Is Dynamic SQL?
- •Why Use Dynamic SQL?
- •Using the EXECUTE IMMEDIATE Statement
- •Specifying Parameter Modes for Bind Variables in Dynamic SQL Strings
- •Building a Dynamic Query with Dynamic SQL
- •Examples of Dynamic SQL for Records, Objects, and Collections
- •Using Bulk Dynamic SQL
- •Using Dynamic SQL with Bulk SQL
- •Examples of Dynamic Bulk Binds
- •Guidelines for Dynamic SQL
- •When to Use or Omit the Semicolon with Dynamic SQL
- •Improving Performance of Dynamic SQL with Bind Variables
- •Passing Schema Object Names As Parameters
- •Using Duplicate Placeholders with Dynamic SQL
- •Using Cursor Attributes with Dynamic SQL
- •Passing Nulls to Dynamic SQL
- •Using Database Links with Dynamic SQL
- •Using Invoker Rights with Dynamic SQL
- •Using Pragma RESTRICT_REFERENCES with Dynamic SQL
- •Avoiding Deadlocks with Dynamic SQL
- •Backward Compatibility of the USING Clause
- •8 Using PL/SQL Subprograms
- •What Are Subprograms?
- •Advantages of PL/SQL Subprograms
- •Understanding PL/SQL Procedures
- •Understanding PL/SQL Functions
- •Using the RETURN Statement
- •Declaring Nested PL/SQL Subprograms
- •Passing Parameters to PL/SQL Subprograms
- •Actual Versus Formal Subprogram Parameters
- •Using Positional, Named, or Mixed Notation for Subprogram Parameters
- •Specifying Subprogram Parameter Modes
- •Using Default Values for Subprogram Parameters
- •Overloading Subprogram Names
- •Guidelines for Overloading with Numeric Types
- •Restrictions on Overloading
- •How Subprogram Calls Are Resolved
- •How Overloading Works with Inheritance
- •Using Invoker's Rights Versus Definer's Rights (AUTHID Clause)
- •Advantages of Invoker's Rights
- •Specifying the Privileges for a Subprogram with the AUTHID Clause
- •Who Is the Current User During Subprogram Execution?
- •How External References Are Resolved in Invoker's Rights Subprograms
- •Overriding Default Name Resolution in Invoker's Rights Subprograms
- •Granting Privileges on Invoker's Rights Subprograms
- •Using Roles with Invoker's Rights Subprograms
- •Using Views and Database Triggers with Invoker's Rights Subprograms
- •Using Database Links with Invoker's Rights Subprograms
- •Using Object Types with Invoker's Rights Subprograms
- •Using Recursion with PL/SQL
- •What Is a Recursive Subprogram?
- •Calling External Subprograms
- •Creating Dynamic Web Pages with PL/SQL Server Pages
- •Controlling Side Effects of PL/SQL Subprograms
- •Understanding Subprogram Parameter Aliasing
- •9 Using PL/SQL Packages
- •What Is a PL/SQL Package?
- •What Goes In a PL/SQL Package?
- •Example of a PL/SQL Package
- •Advantages of PL/SQL Packages
- •Understanding The Package Specification
- •Referencing Package Contents
- •Understanding The Package Body
- •Some Examples of Package Features
- •Private Versus Public Items in Packages
- •Overloading Packaged Subprograms
- •How Package STANDARD Defines the PL/SQL Environment
- •About the DBMS_ALERT Package
- •About the DBMS_OUTPUT Package
- •About the DBMS_PIPE Package
- •About the UTL_FILE Package
- •About the UTL_HTTP Package
- •Guidelines for Writing Packages
- •Separating Cursor Specs and Bodies with Packages
- •10 Handling PL/SQL Errors
- •Overview of PL/SQL Runtime Error Handling
- •Guidelines for Avoiding and Handling PL/SQL Errors and Exceptions
- •Advantages of PL/SQL Exceptions
- •Summary of Predefined PL/SQL Exceptions
- •Defining Your Own PL/SQL Exceptions
- •Declaring PL/SQL Exceptions
- •Scope Rules for PL/SQL Exceptions
- •Associating a PL/SQL Exception with a Number: Pragma EXCEPTION_INIT
- •How PL/SQL Exceptions Are Raised
- •Raising Exceptions with the RAISE Statement
- •How PL/SQL Exceptions Propagate
- •Reraising a PL/SQL Exception
- •Handling Raised PL/SQL Exceptions
- •Handling Exceptions Raised in Declarations
- •Handling Exceptions Raised in Handlers
- •Branching to or from an Exception Handler
- •Retrieving the Error Code and Error Message: SQLCODE and SQLERRM
- •Catching Unhandled Exceptions
- •Tips for Handling PL/SQL Errors
- •Continuing after an Exception Is Raised
- •Retrying a Transaction
- •Using Locator Variables to Identify Exception Locations
- •Overview of PL/SQL Compile-Time Warnings
- •PL/SQL Warning Categories
- •Controlling PL/SQL Warning Messages
- •Using the DBMS_WARNING Package
- •11 Tuning PL/SQL Applications for Performance
- •How PL/SQL Optimizes Your Programs
- •When to Tune PL/SQL Code
- •Guidelines for Avoiding PL/SQL Performance Problems
- •Avoiding CPU Overhead in PL/SQL Code
- •Avoiding Memory Overhead in PL/SQL Code
- •Profiling and Tracing PL/SQL Programs
- •Using The Trace API: Package DBMS_TRACE
- •Reducing Loop Overhead for DML Statements and Queries (FORALL, BULK COLLECT)
- •Using the FORALL Statement
- •Retrieving Query Results into Collections with the BULK COLLECT Clause
- •Writing Computation-Intensive Programs in PL/SQL
- •Tuning Dynamic SQL with EXECUTE IMMEDIATE and Cursor Variables
- •Tuning PL/SQL Procedure Calls with the NOCOPY Compiler Hint
- •Restrictions on NOCOPY
- •Compiling PL/SQL Code for Native Execution
- •Setting Up Transformation Pipelines with Table Functions
- •Overview of Table Functions
- •Using Pipelined Table Functions for Transformations
- •Writing a Pipelined Table Function
- •Returning Results from Table Functions
- •Pipelining Data Between PL/SQL Table Functions
- •Querying Table Functions
- •Optimizing Multiple Calls to Table Functions
- •Fetching from the Results of Table Functions
- •Passing Data with Cursor Variables
- •Performing DML Operations Inside Table Functions
- •Performing DML Operations on Table Functions
- •Handling Exceptions in Table Functions
- •12 Using PL/SQL Object Types
- •Overview of PL/SQL Object Types
- •What Is an Object Type?
- •Why Use Object Types?
- •Structure of an Object Type
- •Components of an Object Type
- •What Languages can I Use for Methods of Object Types?
- •How Object Types Handle the SELF Parameter
- •Overloading
- •Changing Attributes and Methods of an Existing Object Type (Type Evolution)
- •Defining Object Types
- •Overview of PL/SQL Type Inheritance
- •Declaring and Initializing Objects
- •Declaring Objects
- •Initializing Objects
- •How PL/SQL Treats Uninitialized Objects
- •Accessing Object Attributes
- •Defining Object Constructors
- •Calling Object Constructors
- •Calling Object Methods
- •Sharing Objects through the REF Modifier
- •Manipulating Objects through SQL
- •Selecting Objects
- •Inserting Objects
- •Updating Objects
- •Deleting Objects
- •13 PL/SQL Language Elements
- •Assignment Statement
- •AUTONOMOUS_TRANSACTION Pragma
- •Blocks
- •CASE Statement
- •CLOSE Statement
- •Collection Methods
- •Collections
- •Comments
- •COMMIT Statement
- •Constants and Variables
- •Cursor Attributes
- •Cursor Variables
- •Cursors
- •DELETE Statement
- •EXCEPTION_INIT Pragma
- •Exceptions
- •EXECUTE IMMEDIATE Statement
- •EXIT Statement
- •Expressions
- •FETCH Statement
- •FORALL Statement
- •Functions
- •GOTO Statement
- •IF Statement
- •INSERT Statement
- •Literals
- •LOCK TABLE Statement
- •LOOP Statements
- •MERGE Statement
- •NULL Statement
- •Object Types
- •OPEN Statement
- •OPEN-FOR Statement
- •OPEN-FOR-USING Statement
- •Packages
- •Procedures
- •RAISE Statement
- •Records
- •RESTRICT_REFERENCES Pragma
- •RETURN Statement
- •ROLLBACK Statement
- •%ROWTYPE Attribute
- •SAVEPOINT Statement
- •SCN_TO_TIMESTAMP Function
- •SELECT INTO Statement
- •SERIALLY_REUSABLE Pragma
- •SET TRANSACTION Statement
- •SQL Cursor
- •SQLCODE Function
- •SQLERRM Function
- •TIMESTAMP_TO_SCN Function
- •%TYPE Attribute
- •UPDATE Statement
- •Where to Find PL/SQL Sample Programs
- •Exercises for the Reader
- •Assigning Character Values
- •Comparing Character Values
- •Inserting Character Values
- •Selecting Character Values
- •Advantages of Wrapping PL/SQL Procedures
- •Running the PL/SQL Wrap Utility
- •Input and Output Files for the PL/SQL Wrap Utility
- •Limitations of the PL/SQL Wrap Utility
- •What Is Name Resolution?
- •Examples of Qualified Names and Dot Notation
- •Differences in Name Resolution Between SQL and PL/SQL
- •Understanding Capture
- •Inner Capture
- •Same-Scope Capture
- •Outer Capture
- •Avoiding Inner Capture in DML Statements
- •Qualifying References to Object Attributes and Methods
- •Calling Parameterless Subprograms and Methods
- •Name Resolution for SQL Versus PL/SQL
- •When Should I Use Bind Variables with PL/SQL?
- •When Do I Use or Omit the Semicolon with Dynamic SQL?
- •How Can I Use Regular Expressions with PL/SQL?
- •How Do I Continue After a PL/SQL Exception?
- •How Do I Pass a Result Set from PL/SQL to Java or Visual Basic (VB)?
- •How Do I Specify Different Kinds of Names with PL/SQL's Dot Notation?
- •What Can I Do with Objects and Object Types in PL/SQL?
- •How Do I Create a PL/SQL Procedure?
- •How Do I Input or Output Data with PL/SQL?
- •How Do I Perform a Case-Insensitive Query?
- •Index
- •Symbols

PL/SQL Expressions and Comparisons
Assigning Boolean Values
Only the values TRUE, FALSE, and NULL can be assigned to a Boolean variable. You can assign these literal values, or expressions such as comparisons using relational operators.
DECLARE
done BOOLEAN; -- DONE is initially NULL counter NUMBER := 0;
BEGIN
done := FALSE; -- Assign a literal value
WHILE done != TRUE -- Compare to a literal value LOOP
counter := counter + 1;
done := (counter > 500); -- If counter > 500, DONE = TRUE END LOOP;
END;
/
Assigning a SQL Query Result to a PL/SQL Variable
You can use the SELECT statement to have Oracle assign values to a variable. For each item in the select list, there must be a corresponding, type-compatible variable in the INTO list. For example:
DECLARE |
|
emp_id |
employees.employee_id%TYPE := 100; |
emp_name |
employees.last_name%TYPE; |
wages |
NUMBER(7,2); |
BEGIN
SELECT last_name, salary + (salary * nvl(commission_pct,0)) INTO emp_name, wages FROM employees
WHERE employee_id = emp_id;
dbms_output.put_line('Employee ' || emp_name || ' might make ' || wages); END;
/
Because SQL does not have a Boolean type, you cannot select column values into a Boolean variable.
PL/SQL Expressions and Comparisons
Expressions are constructed using operands and operators. An operand is a variable, constant, literal, or function call that contributes a value to an expression. An example of a simple arithmetic expression follows:
-X / 2 + 3
Unary operators such as the negation operator (-) operate on one operand; binary operators such as the division operator (/) operate on two operands. PL/SQL has no ternary operators.
The simplest expressions consist of a single variable, which yields a value directly. PL/SQL evaluates an expression by combining the values of the operands in ways specified by the operators. An expression always returns a single value. PL/SQL determines the datatype of this value by examining the expression and the context in which it appears.
Fundamentals of the PL/SQL Language 2-17
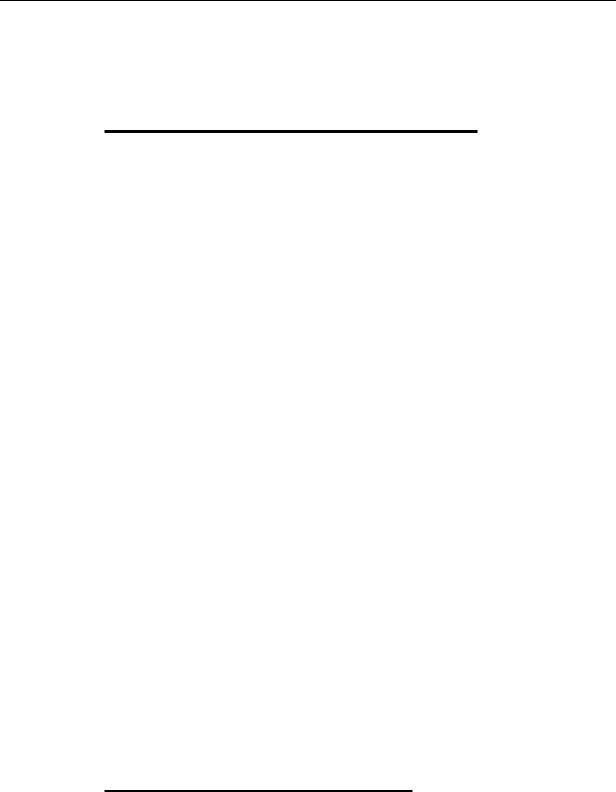
PL/SQL Expressions and Comparisons
Operator Precedence
The operations within an expression are done in a particular order depending on their precedence (priority). Table 2–1 shows the default order of operations from first to last (top to bottom).
Table 2–1 Order of Operations
Operator |
Operation |
|
|
** |
exponentiation |
+, - |
identity, negation |
*, / |
multiplication, division |
+, -, || |
addition, subtraction, concatenation |
=, <, >, <=, >=, <>, !=, ~=, ^=, comparison |
|
IS NULL, LIKE, BETWEEN, IN |
|
NOT |
logical negation |
AND |
conjunction |
OR |
inclusion |
|
|
Operators with higher precedence are applied first. In the example below, both expressions yield 8 because division has a higher precedence than addition. Operators with the same precedence are applied in no particular order.
5 + 12 / 4
12 / 4 + 5
You can use parentheses to control the order of evaluation. For example, the following expression yields 7, not 11, because parentheses override the default operator precedence:
(8 + 6) / 2
In the next example, the subtraction is done before the division because the most deeply nested subexpression is always evaluated first:
100 + (20 / 5 + (7 - 3))
The following example shows that you can always use parentheses to improve readability, even when they are not needed:
(salary * 0.05) + (commission * 0.25)
Logical Operators
The logical operators AND, OR, and NOT follow the tri-state logic shown in Table 2–2. AND and OR are binary operators; NOT is a unary operator.
Table 2–2 Logic Truth Table
x |
y |
x AND y |
x OR y |
NOT x |
|
|
|
|
|
TRUE |
TRUE |
TRUE |
TRUE |
FALSE |
TRUE |
FALSE |
FALSE |
TRUE |
FALSE |
TRUE |
NULL |
NULL |
TRUE |
FALSE |
FALSE |
TRUE |
FALSE |
TRUE |
TRUE |
2-18 PL/SQL User's Guide and Reference

PL/SQL Expressions and Comparisons
Table 2–2 |
(Cont.) Logic Truth Table |
|
||
|
|
|
|
|
x |
y |
x AND y |
x OR y |
NOT x |
|
|
|
|
|
FALSE |
FALSE |
FALSE |
FALSE |
TRUE |
FALSE |
NULL |
FALSE |
NULL |
TRUE |
NULL |
TRUE |
NULL |
TRUE |
NULL |
NULL |
FALSE |
FALSE |
NULL |
NULL |
NULL |
NULL |
NULL |
NULL |
NULL |
|
|
|
|
|
As the truth table shows, AND returns TRUE only if both its operands are true. On the other hand, OR returns TRUE if either of its operands is true. NOT returns the opposite value (logical negation) of its operand. For example, NOT TRUE returns FALSE.
NOT NULL returns NULL, because nulls are indeterminate. Be careful to avoid unexpected results in expressions involving nulls; see "Handling Null Values in Comparisons and Conditional Statements" on page 2-25.
Order of Evaluation
When you do not use parentheses to specify the order of evaluation, operator precedence determines the order. Compare the following expressions:
NOT (valid AND done) |
| |
NOT valid AND done |
If the Boolean variables valid and done have the value FALSE, the first expression yields TRUE. However, the second expression yields FALSE because NOT has a higher precedence than AND. Therefore, the second expression is equivalent to:
(NOT valid) AND done
In the following example, notice that when valid has the value FALSE, the whole expression yields FALSE regardless of the value of done:
valid AND done
Likewise, in the next example, when valid has the value TRUE, the whole expression yields TRUE regardless of the value of done:
valid OR done
Short-Circuit Evaluation
When evaluating a logical expression, PL/SQL uses short-circuit evaluation. That is, PL/SQL stops evaluating the expression as soon as the result can be determined. This lets you write expressions that might otherwise cause an error. Consider the following OR expression:
DECLARE
on_hand INTEGER := 0; on_order INTEGER := 100;
BEGIN
--Does not cause divide-by-zero error; evaluation stops after 1st expr. IF (on_hand = 0) OR ((on_order / on_hand) < 5) THEN
dbms_output.put_line('There are no more widgets left!'); END IF;
END;
/
Fundamentals of the PL/SQL Language 2-19
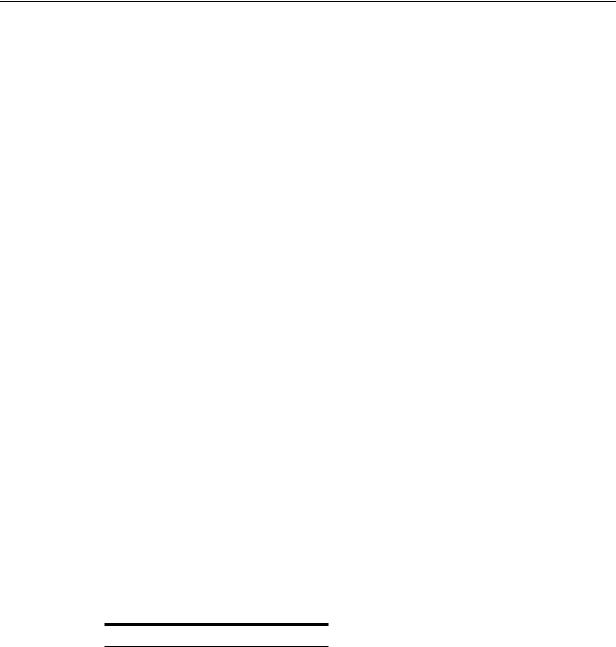
PL/SQL Expressions and Comparisons
When the value of on_hand is zero, the left operand yields TRUE, so PL/SQL does not evaluate the right operand. If PL/SQL evaluated both operands before applying the OR operator, the right operand would cause a division by zero error.
Comparison Operators
Comparison operators compare one expression to another. The result is always true, false, or null. Typically, you use comparison operators in conditional control statements and in the WHERE clause of SQL data manipulation statements. Here are some examples of comparisons for different types:
DECLARE
PROCEDURE assert(assertion VARCHAR2, truth BOOLEAN) IS
BEGIN
IF truth IS NULL THEN
dbms_output.put_line('Assertion ' || assertion || ' is unknown (NULL)'); ELSIF truth = TRUE THEN
dbms_output.put_line('Assertion ' || assertion || ' is TRUE'); ELSE
dbms_output.put_line('Assertion ' || assertion || ' is FALSE'); END IF;
END; BEGIN
assert('2 + 2 = 4', 2 + 2 = 4); assert('10 > 1', 10 > 1); assert('10 <= 1', 10 <= 1);
assert('5 BETWEEN 1 AND 10', 5 BETWEEN 1 AND 10); assert('NULL != 0', NULL != 0);
assert('3 IN (1,3,5)', 3 IN (1,3,5)); assert('''A'' < ''Z''', 'A' < 'Z');
assert('''baseball'' LIKE ''%all%''', 'baseball' LIKE '%all%'); assert('''suit'' || ''case'' = ''suitcase''', 'suit' || 'case' = 'suitcase');
END;
/
Relational Operators
Operator Meaning
=equal to
<>, !=, ~=, ^= not equal to
<less than
>greater than
<= |
less than or equal to |
>= |
greater than or equal to |
|
|
IS NULL Operator
The IS NULL operator returns the Boolean value TRUE if its operand is null or FALSE if it is not null. Comparisons involving nulls always yield NULL. Test whether a value is null as follows:
IF variable IS NULL THEN ...
2-20 PL/SQL User's Guide and Reference