
- •Contents
- •Send Us Your Comments
- •Preface
- •What's New in PL/SQL?
- •1 Overview of PL/SQL
- •Advantages of PL/SQL
- •Tight Integration with SQL
- •Support for SQL
- •Better Performance
- •Higher Productivity
- •Full Portability
- •Tight Security
- •Support for Object-Oriented Programming
- •Understanding the Main Features of PL/SQL
- •Block Structure
- •Variables and Constants
- •Processing Queries with PL/SQL
- •Declaring PL/SQL Variables
- •Control Structures
- •Writing Reusable PL/SQL Code
- •Data Abstraction
- •Error Handling
- •PL/SQL Architecture
- •In the Oracle Database Server
- •In Oracle Tools
- •2 Fundamentals of the PL/SQL Language
- •Character Set
- •Lexical Units
- •Delimiters
- •Literals
- •Comments
- •Declarations
- •Using DEFAULT
- •Using NOT NULL
- •Using the %TYPE Attribute
- •Using the %ROWTYPE Attribute
- •Restrictions on Declarations
- •PL/SQL Naming Conventions
- •Scope and Visibility of PL/SQL Identifiers
- •Assigning Values to Variables
- •Assigning Boolean Values
- •Assigning a SQL Query Result to a PL/SQL Variable
- •PL/SQL Expressions and Comparisons
- •Logical Operators
- •Boolean Expressions
- •CASE Expressions
- •Handling Null Values in Comparisons and Conditional Statements
- •Summary of PL/SQL Built-In Functions
- •3 PL/SQL Datatypes
- •PL/SQL Number Types
- •PL/SQL Character and String Types
- •PL/SQL National Character Types
- •PL/SQL LOB Types
- •PL/SQL Boolean Types
- •PL/SQL Date, Time, and Interval Types
- •Datetime and Interval Arithmetic
- •Avoiding Truncation Problems Using Date and Time Subtypes
- •Overview of PL/SQL Subtypes
- •Using Subtypes
- •Converting PL/SQL Datatypes
- •Explicit Conversion
- •Implicit Conversion
- •Choosing Between Implicit and Explicit Conversion
- •DATE Values
- •RAW and LONG RAW Values
- •4 Using PL/SQL Control Structures
- •Overview of PL/SQL Control Structures
- •Testing Conditions: IF and CASE Statements
- •Using the IF-THEN Statement
- •Using the IF-THEN-ELSE Statement
- •Using the IF-THEN-ELSIF Statement
- •Using the CASE Statement
- •Guidelines for PL/SQL Conditional Statements
- •Controlling Loop Iterations: LOOP and EXIT Statements
- •Using the LOOP Statement
- •Using the EXIT Statement
- •Using the EXIT-WHEN Statement
- •Labeling a PL/SQL Loop
- •Using the WHILE-LOOP Statement
- •Using the FOR-LOOP Statement
- •Sequential Control: GOTO and NULL Statements
- •Using the GOTO Statement
- •Using the NULL Statement
- •5 Using PL/SQL Collections and Records
- •What Is a Collection?
- •Understanding Nested Tables
- •Understanding Varrays
- •Understanding Associative Arrays (Index-By Tables)
- •How Globalization Settings Affect VARCHAR2 Keys for Associative Arrays
- •Choosing Which PL/SQL Collection Types to Use
- •Choosing Between Nested Tables and Associative Arrays
- •Choosing Between Nested Tables and Varrays
- •Defining Collection Types
- •Declaring PL/SQL Collection Variables
- •Initializing and Referencing Collections
- •Referencing Collection Elements
- •Assigning Collections
- •Comparing Collections
- •Using PL/SQL Collections with SQL Statements
- •Using PL/SQL Varrays with INSERT, UPDATE, and SELECT Statements
- •Manipulating Individual Collection Elements with SQL
- •Using Multilevel Collections
- •Using Collection Methods
- •Checking If a Collection Element Exists (EXISTS Method)
- •Counting the Elements in a Collection (COUNT Method)
- •Checking the Maximum Size of a Collection (LIMIT Method)
- •Finding the First or Last Collection Element (FIRST and LAST Methods)
- •Looping Through Collection Elements (PRIOR and NEXT Methods)
- •Increasing the Size of a Collection (EXTEND Method)
- •Decreasing the Size of a Collection (TRIM Method)
- •Deleting Collection Elements (DELETE Method)
- •Applying Methods to Collection Parameters
- •Avoiding Collection Exceptions
- •What Is a PL/SQL Record?
- •Using Records as Procedure Parameters and Function Return Values
- •Assigning Values to Records
- •Comparing Records
- •Inserting PL/SQL Records into the Database
- •Updating the Database with PL/SQL Record Values
- •Restrictions on Record Inserts/Updates
- •Querying Data into Collections of Records
- •6 Performing SQL Operations from PL/SQL
- •Overview of SQL Support in PL/SQL
- •Data Manipulation
- •Transaction Control
- •SQL Functions
- •SQL Pseudocolumns
- •SQL Operators
- •Performing DML Operations from PL/SQL (INSERT, UPDATE, and DELETE)
- •Overview of Implicit Cursor Attributes
- •Using PL/SQL Records in SQL INSERT and UPDATE Statements
- •Issuing Queries from PL/SQL
- •Selecting At Most One Row: SELECT INTO Statement
- •Selecting Multiple Rows: BULK COLLECT Clause
- •Looping Through Multiple Rows: Cursor FOR Loop
- •Performing Complicated Query Processing: Explicit Cursors
- •Querying Data with PL/SQL
- •Querying Data with PL/SQL: Implicit Cursor FOR Loop
- •Querying Data with PL/SQL: Explicit Cursor FOR Loops
- •Overview of Explicit Cursors
- •Using Subqueries
- •Using Correlated Subqueries
- •Writing Maintainable PL/SQL Queries
- •Using Cursor Attributes
- •Overview of Explicit Cursor Attributes
- •Using Cursor Variables (REF CURSORs)
- •What Are Cursor Variables (REF CURSORs)?
- •Why Use Cursor Variables?
- •Declaring REF CURSOR Types and Cursor Variables
- •Controlling Cursor Variables: OPEN-FOR, FETCH, and CLOSE
- •Avoiding Errors with Cursor Variables
- •Restrictions on Cursor Variables
- •Using Cursor Expressions
- •Restrictions on Cursor Expressions
- •Example of Cursor Expressions
- •Constructing REF CURSORs with Cursor Subqueries
- •Overview of Transaction Processing in PL/SQL
- •Using COMMIT, SAVEPOINT, and ROLLBACK in PL/SQL
- •How Oracle Does Implicit Rollbacks
- •Ending Transactions
- •Setting Transaction Properties with SET TRANSACTION
- •Overriding Default Locking
- •Doing Independent Units of Work with Autonomous Transactions
- •Advantages of Autonomous Transactions
- •Controlling Autonomous Transactions
- •Using Autonomous Triggers
- •Calling Autonomous Functions from SQL
- •7 Performing SQL Operations with Native Dynamic SQL
- •What Is Dynamic SQL?
- •Why Use Dynamic SQL?
- •Using the EXECUTE IMMEDIATE Statement
- •Specifying Parameter Modes for Bind Variables in Dynamic SQL Strings
- •Building a Dynamic Query with Dynamic SQL
- •Examples of Dynamic SQL for Records, Objects, and Collections
- •Using Bulk Dynamic SQL
- •Using Dynamic SQL with Bulk SQL
- •Examples of Dynamic Bulk Binds
- •Guidelines for Dynamic SQL
- •When to Use or Omit the Semicolon with Dynamic SQL
- •Improving Performance of Dynamic SQL with Bind Variables
- •Passing Schema Object Names As Parameters
- •Using Duplicate Placeholders with Dynamic SQL
- •Using Cursor Attributes with Dynamic SQL
- •Passing Nulls to Dynamic SQL
- •Using Database Links with Dynamic SQL
- •Using Invoker Rights with Dynamic SQL
- •Using Pragma RESTRICT_REFERENCES with Dynamic SQL
- •Avoiding Deadlocks with Dynamic SQL
- •Backward Compatibility of the USING Clause
- •8 Using PL/SQL Subprograms
- •What Are Subprograms?
- •Advantages of PL/SQL Subprograms
- •Understanding PL/SQL Procedures
- •Understanding PL/SQL Functions
- •Using the RETURN Statement
- •Declaring Nested PL/SQL Subprograms
- •Passing Parameters to PL/SQL Subprograms
- •Actual Versus Formal Subprogram Parameters
- •Using Positional, Named, or Mixed Notation for Subprogram Parameters
- •Specifying Subprogram Parameter Modes
- •Using Default Values for Subprogram Parameters
- •Overloading Subprogram Names
- •Guidelines for Overloading with Numeric Types
- •Restrictions on Overloading
- •How Subprogram Calls Are Resolved
- •How Overloading Works with Inheritance
- •Using Invoker's Rights Versus Definer's Rights (AUTHID Clause)
- •Advantages of Invoker's Rights
- •Specifying the Privileges for a Subprogram with the AUTHID Clause
- •Who Is the Current User During Subprogram Execution?
- •How External References Are Resolved in Invoker's Rights Subprograms
- •Overriding Default Name Resolution in Invoker's Rights Subprograms
- •Granting Privileges on Invoker's Rights Subprograms
- •Using Roles with Invoker's Rights Subprograms
- •Using Views and Database Triggers with Invoker's Rights Subprograms
- •Using Database Links with Invoker's Rights Subprograms
- •Using Object Types with Invoker's Rights Subprograms
- •Using Recursion with PL/SQL
- •What Is a Recursive Subprogram?
- •Calling External Subprograms
- •Creating Dynamic Web Pages with PL/SQL Server Pages
- •Controlling Side Effects of PL/SQL Subprograms
- •Understanding Subprogram Parameter Aliasing
- •9 Using PL/SQL Packages
- •What Is a PL/SQL Package?
- •What Goes In a PL/SQL Package?
- •Example of a PL/SQL Package
- •Advantages of PL/SQL Packages
- •Understanding The Package Specification
- •Referencing Package Contents
- •Understanding The Package Body
- •Some Examples of Package Features
- •Private Versus Public Items in Packages
- •Overloading Packaged Subprograms
- •How Package STANDARD Defines the PL/SQL Environment
- •About the DBMS_ALERT Package
- •About the DBMS_OUTPUT Package
- •About the DBMS_PIPE Package
- •About the UTL_FILE Package
- •About the UTL_HTTP Package
- •Guidelines for Writing Packages
- •Separating Cursor Specs and Bodies with Packages
- •10 Handling PL/SQL Errors
- •Overview of PL/SQL Runtime Error Handling
- •Guidelines for Avoiding and Handling PL/SQL Errors and Exceptions
- •Advantages of PL/SQL Exceptions
- •Summary of Predefined PL/SQL Exceptions
- •Defining Your Own PL/SQL Exceptions
- •Declaring PL/SQL Exceptions
- •Scope Rules for PL/SQL Exceptions
- •Associating a PL/SQL Exception with a Number: Pragma EXCEPTION_INIT
- •How PL/SQL Exceptions Are Raised
- •Raising Exceptions with the RAISE Statement
- •How PL/SQL Exceptions Propagate
- •Reraising a PL/SQL Exception
- •Handling Raised PL/SQL Exceptions
- •Handling Exceptions Raised in Declarations
- •Handling Exceptions Raised in Handlers
- •Branching to or from an Exception Handler
- •Retrieving the Error Code and Error Message: SQLCODE and SQLERRM
- •Catching Unhandled Exceptions
- •Tips for Handling PL/SQL Errors
- •Continuing after an Exception Is Raised
- •Retrying a Transaction
- •Using Locator Variables to Identify Exception Locations
- •Overview of PL/SQL Compile-Time Warnings
- •PL/SQL Warning Categories
- •Controlling PL/SQL Warning Messages
- •Using the DBMS_WARNING Package
- •11 Tuning PL/SQL Applications for Performance
- •How PL/SQL Optimizes Your Programs
- •When to Tune PL/SQL Code
- •Guidelines for Avoiding PL/SQL Performance Problems
- •Avoiding CPU Overhead in PL/SQL Code
- •Avoiding Memory Overhead in PL/SQL Code
- •Profiling and Tracing PL/SQL Programs
- •Using The Trace API: Package DBMS_TRACE
- •Reducing Loop Overhead for DML Statements and Queries (FORALL, BULK COLLECT)
- •Using the FORALL Statement
- •Retrieving Query Results into Collections with the BULK COLLECT Clause
- •Writing Computation-Intensive Programs in PL/SQL
- •Tuning Dynamic SQL with EXECUTE IMMEDIATE and Cursor Variables
- •Tuning PL/SQL Procedure Calls with the NOCOPY Compiler Hint
- •Restrictions on NOCOPY
- •Compiling PL/SQL Code for Native Execution
- •Setting Up Transformation Pipelines with Table Functions
- •Overview of Table Functions
- •Using Pipelined Table Functions for Transformations
- •Writing a Pipelined Table Function
- •Returning Results from Table Functions
- •Pipelining Data Between PL/SQL Table Functions
- •Querying Table Functions
- •Optimizing Multiple Calls to Table Functions
- •Fetching from the Results of Table Functions
- •Passing Data with Cursor Variables
- •Performing DML Operations Inside Table Functions
- •Performing DML Operations on Table Functions
- •Handling Exceptions in Table Functions
- •12 Using PL/SQL Object Types
- •Overview of PL/SQL Object Types
- •What Is an Object Type?
- •Why Use Object Types?
- •Structure of an Object Type
- •Components of an Object Type
- •What Languages can I Use for Methods of Object Types?
- •How Object Types Handle the SELF Parameter
- •Overloading
- •Changing Attributes and Methods of an Existing Object Type (Type Evolution)
- •Defining Object Types
- •Overview of PL/SQL Type Inheritance
- •Declaring and Initializing Objects
- •Declaring Objects
- •Initializing Objects
- •How PL/SQL Treats Uninitialized Objects
- •Accessing Object Attributes
- •Defining Object Constructors
- •Calling Object Constructors
- •Calling Object Methods
- •Sharing Objects through the REF Modifier
- •Manipulating Objects through SQL
- •Selecting Objects
- •Inserting Objects
- •Updating Objects
- •Deleting Objects
- •13 PL/SQL Language Elements
- •Assignment Statement
- •AUTONOMOUS_TRANSACTION Pragma
- •Blocks
- •CASE Statement
- •CLOSE Statement
- •Collection Methods
- •Collections
- •Comments
- •COMMIT Statement
- •Constants and Variables
- •Cursor Attributes
- •Cursor Variables
- •Cursors
- •DELETE Statement
- •EXCEPTION_INIT Pragma
- •Exceptions
- •EXECUTE IMMEDIATE Statement
- •EXIT Statement
- •Expressions
- •FETCH Statement
- •FORALL Statement
- •Functions
- •GOTO Statement
- •IF Statement
- •INSERT Statement
- •Literals
- •LOCK TABLE Statement
- •LOOP Statements
- •MERGE Statement
- •NULL Statement
- •Object Types
- •OPEN Statement
- •OPEN-FOR Statement
- •OPEN-FOR-USING Statement
- •Packages
- •Procedures
- •RAISE Statement
- •Records
- •RESTRICT_REFERENCES Pragma
- •RETURN Statement
- •ROLLBACK Statement
- •%ROWTYPE Attribute
- •SAVEPOINT Statement
- •SCN_TO_TIMESTAMP Function
- •SELECT INTO Statement
- •SERIALLY_REUSABLE Pragma
- •SET TRANSACTION Statement
- •SQL Cursor
- •SQLCODE Function
- •SQLERRM Function
- •TIMESTAMP_TO_SCN Function
- •%TYPE Attribute
- •UPDATE Statement
- •Where to Find PL/SQL Sample Programs
- •Exercises for the Reader
- •Assigning Character Values
- •Comparing Character Values
- •Inserting Character Values
- •Selecting Character Values
- •Advantages of Wrapping PL/SQL Procedures
- •Running the PL/SQL Wrap Utility
- •Input and Output Files for the PL/SQL Wrap Utility
- •Limitations of the PL/SQL Wrap Utility
- •What Is Name Resolution?
- •Examples of Qualified Names and Dot Notation
- •Differences in Name Resolution Between SQL and PL/SQL
- •Understanding Capture
- •Inner Capture
- •Same-Scope Capture
- •Outer Capture
- •Avoiding Inner Capture in DML Statements
- •Qualifying References to Object Attributes and Methods
- •Calling Parameterless Subprograms and Methods
- •Name Resolution for SQL Versus PL/SQL
- •When Should I Use Bind Variables with PL/SQL?
- •When Do I Use or Omit the Semicolon with Dynamic SQL?
- •How Can I Use Regular Expressions with PL/SQL?
- •How Do I Continue After a PL/SQL Exception?
- •How Do I Pass a Result Set from PL/SQL to Java or Visual Basic (VB)?
- •How Do I Specify Different Kinds of Names with PL/SQL's Dot Notation?
- •What Can I Do with Objects and Object Types in PL/SQL?
- •How Do I Create a PL/SQL Procedure?
- •How Do I Input or Output Data with PL/SQL?
- •How Do I Perform a Case-Insensitive Query?
- •Index
- •Symbols
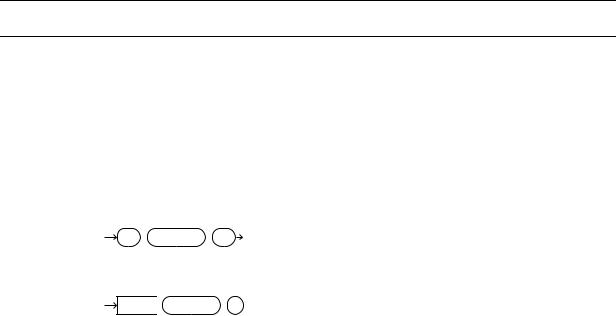
GOTO Statement
GOTO Statement
The GOTO statement branches unconditionally to a statement label or block label. The label must be unique within its scope and must precede an executable statement or a PL/SQL block. The GOTO statement transfers control to the labelled statement or block. For more information, see "Using the GOTO Statement" on page 4-12.
Syntax
label_declaration
<< label_name
>>
goto_statement
GOTO label_name
;
Keyword and Parameter Description
label_name
A label that you assigned to an executable statement or a PL/SQL block. A GOTO statement transfers control to the statement or block following <<label_name>>.
Usage Notes
Some possible destinations of a GOTO statement are not allowed. In particular, a GOTO statement cannot branch into an IF statement, LOOP statement, or sub-block.
From the current block, a GOTO statement can branch to another place in the block or into an enclosing block, but not into an exception handler. From an exception handler, a GOTO statement can branch into an enclosing block, but not into the current block.
If you use the GOTO statement to exit a cursor FOR loop prematurely, the cursor is closed automatically. The cursor is also closed automatically if an exception is raised inside the loop.
A given label can appear only once in a block. However, the label can appear in other blocks including enclosing blocks and sub-blocks. If a GOTO statement cannot find its target label in the current block, it branches to the first enclosing block in which the label appears.
Examples
A GOTO label cannot precede just any keyword. It must precede an executable statement or a PL/SQL block. To branch to a place that does not have an executable statement, add the NULL statement:
FOR ctr IN 1..50 LOOP DELETE FROM emp WHERE ...
IF SQL%FOUND THEN GOTO end_loop;
END IF;
...
<<end_loop>>
NULL; -- an executable statement that specifies inaction END LOOP;
PL/SQL Language Elements 13-71
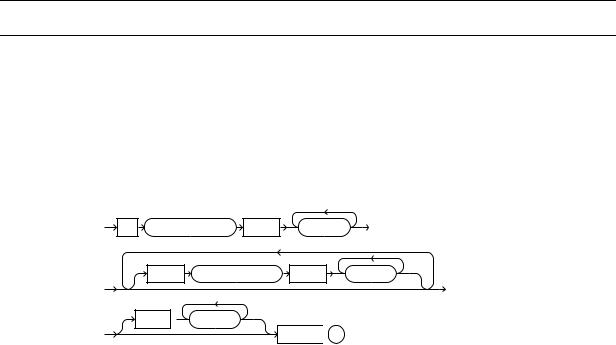
IF Statement
IF Statement
The IF statement executes or skips a sequence of statements, depending on the value of a Boolean expression. For more information, see "Testing Conditions: IF and CASE Statements" on page 4-2.
Syntax
if_statement |
|
|
|
|
|
IF |
boolean_expression |
THEN |
statement |
|
|
|
ELSIF |
boolean_expression |
THEN |
statement |
ELSE statement
END IF ;
Keyword and Parameter Description
boolean_expression
An expression that returns the Boolean value TRUE, FALSE, or NULL. Examples are comparisons for equality, greater-than, or less-than. The sequence following the THEN keyword is executed only if the expression returns TRUE.
ELSE
If control reaches this keyword, the sequence of statements that follows it is executed. This occurs when none of the previous conditional tests returned TRUE.
ELSIF
Introduces a Boolean expression that is evaluated if none of the preceding conditions returned TRUE.
THEN
If the expression returns TRUE, the statements after the THEN keyword are executed.
Usage Notes
There are three forms of IF statements: IF-THEN, IF-THEN-ELSE, and IF-THEN-ELSIF. The simplest form of IF statement associates a Boolean expression with a sequence of statements enclosed by the keywords THEN and END IF. The sequence of statements is executed only if the expression returns TRUE. If the expression returns FALSE or NULL, the IF statement does nothing. In either case, control passes to the next statement.
The second form of IF statement adds the keyword ELSE followed by an alternative sequence of statements. The sequence of statements in the ELSE clause is executed only if the Boolean expression returns FALSE or NULL. Thus, the ELSE clause ensures that a sequence of statements is executed.
The third form of IF statement uses the keyword ELSIF to introduce additional Boolean expressions. If the first expression returns FALSE or NULL, the ELSIF clause
13-72 PL/SQL User's Guide and Reference

IF Statement
evaluates another expression. An IF statement can have any number of ELSIF clauses; the final ELSE clause is optional. Boolean expressions are evaluated one by one from top to bottom. If any expression returns TRUE, its associated sequence of statements is executed and control passes to the next statement. If all expressions return FALSE or NULL, the sequence in the ELSE clause is executed.
An IF statement never executes more than one sequence of statements because processing is complete after any sequence of statements is executed. However, the THEN and ELSE clauses can include more IF statements. That is, IF statements can be nested.
Examples
In the example below, if shoe_count has a value of 10, both the first and second Boolean expressions return TRUE. Nevertheless, order_quantity is assigned the proper value of 50 because processing of an IF statement stops after an expression returns TRUE and its associated sequence of statements is executed. The expression associated with ELSIF is never evaluated and control passes to the INSERT statement.
IF shoe_count < 20 THEN order_quantity := 50;
ELSIF shoe_count < 30 THEN order_quantity := 20;
ELSE
order_quantity := 10; END IF;
INSERT INTO purchase_order VALUES (shoe_type, order_quantity);
In the following example, depending on the value of score, one of two status messages is inserted into the grades table:
IF score < 70 THEN fail := fail + 1;
INSERT INTO grades VALUES (student_id, 'Failed'); ELSE
pass := pass + 1;
INSERT INTO grades VALUES (student_id, 'Passed'); END IF;
Related Topics
CASE Statement, Expressions
PL/SQL Language Elements 13-73
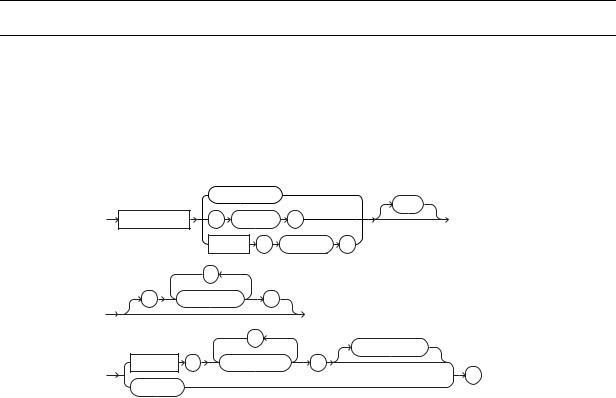
INSERT Statement
INSERT Statement
The INSERT statement adds one or more new rows of data to a database table. For a full description of the INSERT statement, see Oracle Database SQL Reference.
Syntax
insert_statement |
|
|
|
|
|
|
table_reference |
|
alias |
||
|
|
|
|
|
|
INSERT INTO |
( |
subquery |
) |
|
|
|
TABLE |
( |
subquery2 |
) |
|
|
, |
|
|
|
|
( |
column_name |
) |
|
|
|
|
|
|
, |
|
returning_clause |
|
|
|
|
|
|
VALUES |
( |
sql_expression |
) |
|
;
subquery3
Keyword and Parameter Description
alias
Another (usually short) name for the referenced table or view.
column_name[, column_name]...
A list of columns in a database table or view. The columns can be listed in any order, as long as the expressions in the VALUES clause are listed in the same order. Each column name can only be listed once. If the list does not include all the columns in a table, each missing columns is set to NULL or to a default value specified in the CREATE TABLE statement.
returning_clause
Returns values from inserted rows, eliminating the need to SELECT the rows afterward. You can retrieve the column values into variables or into collections. You cannot use the RETURNING clause for remote or parallel inserts. If the statement does not affect any rows, the values of the variables specified in the RETURNING clause are undefined. For the syntax of returning_clause, see "DELETE Statement" on
page 13-41.
sql_expression
Any expression valid in SQL. For example, it could be a literal, a PL/SQL variable, or a SQL query that returns a single value. For more information, see Oracle Database SQL Reference. PL/SQL also lets you use a record variable here.
subquery
A SELECT statement that provides a set of rows for processing. Its syntax is like that of select_into_statement without the INTO clause. See "SELECT INTO Statement" on page 13-123.
13-74 PL/SQL User's Guide and Reference

INSERT Statement
subquery3
A SELECT statement that returns a set of rows. Each row returned by the select statement is inserted into the table. The subquery must return a value for every column in the column list, or for every column in the table if there is no column list.
table_reference
A table or view that must be accessible when you execute the INSERT statement, and for which you must have INSERT privileges. For the syntax of table_reference, see "DELETE Statement" on page 13-41.
TABLE (subquery2)
The operand of TABLE is a SELECT statement that returns a single column value representing a nested table. This operator specifies that the value is a collection, not a scalar value.
VALUES (...)
Assigns the values of expressions to corresponding columns in the column list. If there is no column list, the first value is inserted into the first column defined by the CREATE TABLE statement, the second value is inserted into the second column, and so on.
There must be one value for each column in the column list. The datatypes of the values being inserted must be compatible with the datatypes of corresponding columns in the column list.
Usage Notes
Character and date literals in the VALUES list must be enclosed by single quotes ('). Numeric literals are not enclosed by quotes.
The implicit cursor SQL and the cursor attributes %NOTFOUND, %FOUND, %ROWCOUNT, and %ISOPEN let you access useful information about the execution of an INSERT statement.
Examples
The following examples show various forms of INSERT statement:
INSERT INTO bonus SELECT ename, job, sal, comm FROM emp WHERE comm > sal * 0.25;
...
INSERT INTO emp (empno, ename, job, sal, comm, deptno)
VALUES (4160, 'STURDEVIN', 'SECURITY GUARD', 2045, NULL, 30);
...
INSERT INTO dept
VALUES (my_deptno, UPPER(my_dname), 'CHICAGO');
Related Topics
DELETE Statement, SELECT INTO Statement, UPDATE Statement
PL/SQL Language Elements 13-75