
- •Contents
- •Send Us Your Comments
- •Preface
- •What's New in PL/SQL?
- •1 Overview of PL/SQL
- •Advantages of PL/SQL
- •Tight Integration with SQL
- •Support for SQL
- •Better Performance
- •Higher Productivity
- •Full Portability
- •Tight Security
- •Support for Object-Oriented Programming
- •Understanding the Main Features of PL/SQL
- •Block Structure
- •Variables and Constants
- •Processing Queries with PL/SQL
- •Declaring PL/SQL Variables
- •Control Structures
- •Writing Reusable PL/SQL Code
- •Data Abstraction
- •Error Handling
- •PL/SQL Architecture
- •In the Oracle Database Server
- •In Oracle Tools
- •2 Fundamentals of the PL/SQL Language
- •Character Set
- •Lexical Units
- •Delimiters
- •Literals
- •Comments
- •Declarations
- •Using DEFAULT
- •Using NOT NULL
- •Using the %TYPE Attribute
- •Using the %ROWTYPE Attribute
- •Restrictions on Declarations
- •PL/SQL Naming Conventions
- •Scope and Visibility of PL/SQL Identifiers
- •Assigning Values to Variables
- •Assigning Boolean Values
- •Assigning a SQL Query Result to a PL/SQL Variable
- •PL/SQL Expressions and Comparisons
- •Logical Operators
- •Boolean Expressions
- •CASE Expressions
- •Handling Null Values in Comparisons and Conditional Statements
- •Summary of PL/SQL Built-In Functions
- •3 PL/SQL Datatypes
- •PL/SQL Number Types
- •PL/SQL Character and String Types
- •PL/SQL National Character Types
- •PL/SQL LOB Types
- •PL/SQL Boolean Types
- •PL/SQL Date, Time, and Interval Types
- •Datetime and Interval Arithmetic
- •Avoiding Truncation Problems Using Date and Time Subtypes
- •Overview of PL/SQL Subtypes
- •Using Subtypes
- •Converting PL/SQL Datatypes
- •Explicit Conversion
- •Implicit Conversion
- •Choosing Between Implicit and Explicit Conversion
- •DATE Values
- •RAW and LONG RAW Values
- •4 Using PL/SQL Control Structures
- •Overview of PL/SQL Control Structures
- •Testing Conditions: IF and CASE Statements
- •Using the IF-THEN Statement
- •Using the IF-THEN-ELSE Statement
- •Using the IF-THEN-ELSIF Statement
- •Using the CASE Statement
- •Guidelines for PL/SQL Conditional Statements
- •Controlling Loop Iterations: LOOP and EXIT Statements
- •Using the LOOP Statement
- •Using the EXIT Statement
- •Using the EXIT-WHEN Statement
- •Labeling a PL/SQL Loop
- •Using the WHILE-LOOP Statement
- •Using the FOR-LOOP Statement
- •Sequential Control: GOTO and NULL Statements
- •Using the GOTO Statement
- •Using the NULL Statement
- •5 Using PL/SQL Collections and Records
- •What Is a Collection?
- •Understanding Nested Tables
- •Understanding Varrays
- •Understanding Associative Arrays (Index-By Tables)
- •How Globalization Settings Affect VARCHAR2 Keys for Associative Arrays
- •Choosing Which PL/SQL Collection Types to Use
- •Choosing Between Nested Tables and Associative Arrays
- •Choosing Between Nested Tables and Varrays
- •Defining Collection Types
- •Declaring PL/SQL Collection Variables
- •Initializing and Referencing Collections
- •Referencing Collection Elements
- •Assigning Collections
- •Comparing Collections
- •Using PL/SQL Collections with SQL Statements
- •Using PL/SQL Varrays with INSERT, UPDATE, and SELECT Statements
- •Manipulating Individual Collection Elements with SQL
- •Using Multilevel Collections
- •Using Collection Methods
- •Checking If a Collection Element Exists (EXISTS Method)
- •Counting the Elements in a Collection (COUNT Method)
- •Checking the Maximum Size of a Collection (LIMIT Method)
- •Finding the First or Last Collection Element (FIRST and LAST Methods)
- •Looping Through Collection Elements (PRIOR and NEXT Methods)
- •Increasing the Size of a Collection (EXTEND Method)
- •Decreasing the Size of a Collection (TRIM Method)
- •Deleting Collection Elements (DELETE Method)
- •Applying Methods to Collection Parameters
- •Avoiding Collection Exceptions
- •What Is a PL/SQL Record?
- •Using Records as Procedure Parameters and Function Return Values
- •Assigning Values to Records
- •Comparing Records
- •Inserting PL/SQL Records into the Database
- •Updating the Database with PL/SQL Record Values
- •Restrictions on Record Inserts/Updates
- •Querying Data into Collections of Records
- •6 Performing SQL Operations from PL/SQL
- •Overview of SQL Support in PL/SQL
- •Data Manipulation
- •Transaction Control
- •SQL Functions
- •SQL Pseudocolumns
- •SQL Operators
- •Performing DML Operations from PL/SQL (INSERT, UPDATE, and DELETE)
- •Overview of Implicit Cursor Attributes
- •Using PL/SQL Records in SQL INSERT and UPDATE Statements
- •Issuing Queries from PL/SQL
- •Selecting At Most One Row: SELECT INTO Statement
- •Selecting Multiple Rows: BULK COLLECT Clause
- •Looping Through Multiple Rows: Cursor FOR Loop
- •Performing Complicated Query Processing: Explicit Cursors
- •Querying Data with PL/SQL
- •Querying Data with PL/SQL: Implicit Cursor FOR Loop
- •Querying Data with PL/SQL: Explicit Cursor FOR Loops
- •Overview of Explicit Cursors
- •Using Subqueries
- •Using Correlated Subqueries
- •Writing Maintainable PL/SQL Queries
- •Using Cursor Attributes
- •Overview of Explicit Cursor Attributes
- •Using Cursor Variables (REF CURSORs)
- •What Are Cursor Variables (REF CURSORs)?
- •Why Use Cursor Variables?
- •Declaring REF CURSOR Types and Cursor Variables
- •Controlling Cursor Variables: OPEN-FOR, FETCH, and CLOSE
- •Avoiding Errors with Cursor Variables
- •Restrictions on Cursor Variables
- •Using Cursor Expressions
- •Restrictions on Cursor Expressions
- •Example of Cursor Expressions
- •Constructing REF CURSORs with Cursor Subqueries
- •Overview of Transaction Processing in PL/SQL
- •Using COMMIT, SAVEPOINT, and ROLLBACK in PL/SQL
- •How Oracle Does Implicit Rollbacks
- •Ending Transactions
- •Setting Transaction Properties with SET TRANSACTION
- •Overriding Default Locking
- •Doing Independent Units of Work with Autonomous Transactions
- •Advantages of Autonomous Transactions
- •Controlling Autonomous Transactions
- •Using Autonomous Triggers
- •Calling Autonomous Functions from SQL
- •7 Performing SQL Operations with Native Dynamic SQL
- •What Is Dynamic SQL?
- •Why Use Dynamic SQL?
- •Using the EXECUTE IMMEDIATE Statement
- •Specifying Parameter Modes for Bind Variables in Dynamic SQL Strings
- •Building a Dynamic Query with Dynamic SQL
- •Examples of Dynamic SQL for Records, Objects, and Collections
- •Using Bulk Dynamic SQL
- •Using Dynamic SQL with Bulk SQL
- •Examples of Dynamic Bulk Binds
- •Guidelines for Dynamic SQL
- •When to Use or Omit the Semicolon with Dynamic SQL
- •Improving Performance of Dynamic SQL with Bind Variables
- •Passing Schema Object Names As Parameters
- •Using Duplicate Placeholders with Dynamic SQL
- •Using Cursor Attributes with Dynamic SQL
- •Passing Nulls to Dynamic SQL
- •Using Database Links with Dynamic SQL
- •Using Invoker Rights with Dynamic SQL
- •Using Pragma RESTRICT_REFERENCES with Dynamic SQL
- •Avoiding Deadlocks with Dynamic SQL
- •Backward Compatibility of the USING Clause
- •8 Using PL/SQL Subprograms
- •What Are Subprograms?
- •Advantages of PL/SQL Subprograms
- •Understanding PL/SQL Procedures
- •Understanding PL/SQL Functions
- •Using the RETURN Statement
- •Declaring Nested PL/SQL Subprograms
- •Passing Parameters to PL/SQL Subprograms
- •Actual Versus Formal Subprogram Parameters
- •Using Positional, Named, or Mixed Notation for Subprogram Parameters
- •Specifying Subprogram Parameter Modes
- •Using Default Values for Subprogram Parameters
- •Overloading Subprogram Names
- •Guidelines for Overloading with Numeric Types
- •Restrictions on Overloading
- •How Subprogram Calls Are Resolved
- •How Overloading Works with Inheritance
- •Using Invoker's Rights Versus Definer's Rights (AUTHID Clause)
- •Advantages of Invoker's Rights
- •Specifying the Privileges for a Subprogram with the AUTHID Clause
- •Who Is the Current User During Subprogram Execution?
- •How External References Are Resolved in Invoker's Rights Subprograms
- •Overriding Default Name Resolution in Invoker's Rights Subprograms
- •Granting Privileges on Invoker's Rights Subprograms
- •Using Roles with Invoker's Rights Subprograms
- •Using Views and Database Triggers with Invoker's Rights Subprograms
- •Using Database Links with Invoker's Rights Subprograms
- •Using Object Types with Invoker's Rights Subprograms
- •Using Recursion with PL/SQL
- •What Is a Recursive Subprogram?
- •Calling External Subprograms
- •Creating Dynamic Web Pages with PL/SQL Server Pages
- •Controlling Side Effects of PL/SQL Subprograms
- •Understanding Subprogram Parameter Aliasing
- •9 Using PL/SQL Packages
- •What Is a PL/SQL Package?
- •What Goes In a PL/SQL Package?
- •Example of a PL/SQL Package
- •Advantages of PL/SQL Packages
- •Understanding The Package Specification
- •Referencing Package Contents
- •Understanding The Package Body
- •Some Examples of Package Features
- •Private Versus Public Items in Packages
- •Overloading Packaged Subprograms
- •How Package STANDARD Defines the PL/SQL Environment
- •About the DBMS_ALERT Package
- •About the DBMS_OUTPUT Package
- •About the DBMS_PIPE Package
- •About the UTL_FILE Package
- •About the UTL_HTTP Package
- •Guidelines for Writing Packages
- •Separating Cursor Specs and Bodies with Packages
- •10 Handling PL/SQL Errors
- •Overview of PL/SQL Runtime Error Handling
- •Guidelines for Avoiding and Handling PL/SQL Errors and Exceptions
- •Advantages of PL/SQL Exceptions
- •Summary of Predefined PL/SQL Exceptions
- •Defining Your Own PL/SQL Exceptions
- •Declaring PL/SQL Exceptions
- •Scope Rules for PL/SQL Exceptions
- •Associating a PL/SQL Exception with a Number: Pragma EXCEPTION_INIT
- •How PL/SQL Exceptions Are Raised
- •Raising Exceptions with the RAISE Statement
- •How PL/SQL Exceptions Propagate
- •Reraising a PL/SQL Exception
- •Handling Raised PL/SQL Exceptions
- •Handling Exceptions Raised in Declarations
- •Handling Exceptions Raised in Handlers
- •Branching to or from an Exception Handler
- •Retrieving the Error Code and Error Message: SQLCODE and SQLERRM
- •Catching Unhandled Exceptions
- •Tips for Handling PL/SQL Errors
- •Continuing after an Exception Is Raised
- •Retrying a Transaction
- •Using Locator Variables to Identify Exception Locations
- •Overview of PL/SQL Compile-Time Warnings
- •PL/SQL Warning Categories
- •Controlling PL/SQL Warning Messages
- •Using the DBMS_WARNING Package
- •11 Tuning PL/SQL Applications for Performance
- •How PL/SQL Optimizes Your Programs
- •When to Tune PL/SQL Code
- •Guidelines for Avoiding PL/SQL Performance Problems
- •Avoiding CPU Overhead in PL/SQL Code
- •Avoiding Memory Overhead in PL/SQL Code
- •Profiling and Tracing PL/SQL Programs
- •Using The Trace API: Package DBMS_TRACE
- •Reducing Loop Overhead for DML Statements and Queries (FORALL, BULK COLLECT)
- •Using the FORALL Statement
- •Retrieving Query Results into Collections with the BULK COLLECT Clause
- •Writing Computation-Intensive Programs in PL/SQL
- •Tuning Dynamic SQL with EXECUTE IMMEDIATE and Cursor Variables
- •Tuning PL/SQL Procedure Calls with the NOCOPY Compiler Hint
- •Restrictions on NOCOPY
- •Compiling PL/SQL Code for Native Execution
- •Setting Up Transformation Pipelines with Table Functions
- •Overview of Table Functions
- •Using Pipelined Table Functions for Transformations
- •Writing a Pipelined Table Function
- •Returning Results from Table Functions
- •Pipelining Data Between PL/SQL Table Functions
- •Querying Table Functions
- •Optimizing Multiple Calls to Table Functions
- •Fetching from the Results of Table Functions
- •Passing Data with Cursor Variables
- •Performing DML Operations Inside Table Functions
- •Performing DML Operations on Table Functions
- •Handling Exceptions in Table Functions
- •12 Using PL/SQL Object Types
- •Overview of PL/SQL Object Types
- •What Is an Object Type?
- •Why Use Object Types?
- •Structure of an Object Type
- •Components of an Object Type
- •What Languages can I Use for Methods of Object Types?
- •How Object Types Handle the SELF Parameter
- •Overloading
- •Changing Attributes and Methods of an Existing Object Type (Type Evolution)
- •Defining Object Types
- •Overview of PL/SQL Type Inheritance
- •Declaring and Initializing Objects
- •Declaring Objects
- •Initializing Objects
- •How PL/SQL Treats Uninitialized Objects
- •Accessing Object Attributes
- •Defining Object Constructors
- •Calling Object Constructors
- •Calling Object Methods
- •Sharing Objects through the REF Modifier
- •Manipulating Objects through SQL
- •Selecting Objects
- •Inserting Objects
- •Updating Objects
- •Deleting Objects
- •13 PL/SQL Language Elements
- •Assignment Statement
- •AUTONOMOUS_TRANSACTION Pragma
- •Blocks
- •CASE Statement
- •CLOSE Statement
- •Collection Methods
- •Collections
- •Comments
- •COMMIT Statement
- •Constants and Variables
- •Cursor Attributes
- •Cursor Variables
- •Cursors
- •DELETE Statement
- •EXCEPTION_INIT Pragma
- •Exceptions
- •EXECUTE IMMEDIATE Statement
- •EXIT Statement
- •Expressions
- •FETCH Statement
- •FORALL Statement
- •Functions
- •GOTO Statement
- •IF Statement
- •INSERT Statement
- •Literals
- •LOCK TABLE Statement
- •LOOP Statements
- •MERGE Statement
- •NULL Statement
- •Object Types
- •OPEN Statement
- •OPEN-FOR Statement
- •OPEN-FOR-USING Statement
- •Packages
- •Procedures
- •RAISE Statement
- •Records
- •RESTRICT_REFERENCES Pragma
- •RETURN Statement
- •ROLLBACK Statement
- •%ROWTYPE Attribute
- •SAVEPOINT Statement
- •SCN_TO_TIMESTAMP Function
- •SELECT INTO Statement
- •SERIALLY_REUSABLE Pragma
- •SET TRANSACTION Statement
- •SQL Cursor
- •SQLCODE Function
- •SQLERRM Function
- •TIMESTAMP_TO_SCN Function
- •%TYPE Attribute
- •UPDATE Statement
- •Where to Find PL/SQL Sample Programs
- •Exercises for the Reader
- •Assigning Character Values
- •Comparing Character Values
- •Inserting Character Values
- •Selecting Character Values
- •Advantages of Wrapping PL/SQL Procedures
- •Running the PL/SQL Wrap Utility
- •Input and Output Files for the PL/SQL Wrap Utility
- •Limitations of the PL/SQL Wrap Utility
- •What Is Name Resolution?
- •Examples of Qualified Names and Dot Notation
- •Differences in Name Resolution Between SQL and PL/SQL
- •Understanding Capture
- •Inner Capture
- •Same-Scope Capture
- •Outer Capture
- •Avoiding Inner Capture in DML Statements
- •Qualifying References to Object Attributes and Methods
- •Calling Parameterless Subprograms and Methods
- •Name Resolution for SQL Versus PL/SQL
- •When Should I Use Bind Variables with PL/SQL?
- •When Do I Use or Omit the Semicolon with Dynamic SQL?
- •How Can I Use Regular Expressions with PL/SQL?
- •How Do I Continue After a PL/SQL Exception?
- •How Do I Pass a Result Set from PL/SQL to Java or Visual Basic (VB)?
- •How Do I Specify Different Kinds of Names with PL/SQL's Dot Notation?
- •What Can I Do with Objects and Object Types in PL/SQL?
- •How Do I Create a PL/SQL Procedure?
- •How Do I Input or Output Data with PL/SQL?
- •How Do I Perform a Case-Insensitive Query?
- •Index
- •Symbols
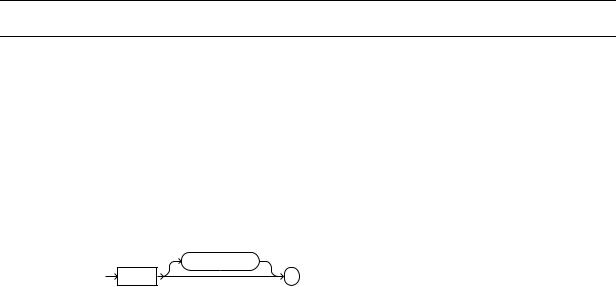
RAISE Statement
RAISE Statement
The RAISE statement stops normal execution of a PL/SQL block or subprogram and transfers control to an exception handler.
RAISE statements can raise predefined exceptions, such as ZERO_DIVIDE or NO_DATA_FOUND, or user-defined exceptions whose names you decide. For more information, see "Defining Your Own PL/SQL Exceptions" on page 10-6.
Syntax
raise_statement
exception_name
RAISE |
; |
Keyword and Parameter Description
exception_name
A predefined or user-defined exception. For a list of the predefined exceptions, see "Summary of Predefined PL/SQL Exceptions" on page 10-4.
Usage Notes
PL/SQL blocks and subprograms should RAISE an exception only when an error makes it impractical to continue processing. You can code a RAISE statement for a given exception anywhere within the scope of that exception.
When an exception is raised, if PL/SQL cannot find a handler for it in the current block, the exception propagates to successive enclosing blocks, until a handler is found or there are no more blocks to search. If no handler is found, PL/SQL returns an unhandled exception error to the host environment.
In an exception handler, you can omit the exception name in a RAISE statement, which raises the current exception again. This technique allows you to take some initial corrective action (perhaps just logging the problem), then pass control to another handler that does more extensive correction. When an exception is reraised, the first block searched is the enclosing block, not the current block.
Example
The following example raises an exception when an inventoried part is out of stock, or when a divide-by-zero situation is about to occur:
DECLARE
out_of_stock EXCEPTION; quantity_on_hand NUMBER := 0; denominator NUMBER := 0;
BEGIN
IF quantity_on_hand = 0 THEN RAISE out_of_stock;
END IF;
IF denominator = 0 THEN raise ZERO_DIVIDE;
END IF;
13-108 PL/SQL User's Guide and Reference

RAISE Statement
EXCEPTION
WHEN out_of_stock THEN
dbms_output.put_line('No more parts in stock.'); WHEN ZERO_DIVIDE THEN
dbms_output.put_line('Attempt to divide by zero.'); WHEN OTHERS THEN
dbms_output.put_line('Some other kind of problem...');
END;
/
Related Topics
Exceptions
PL/SQL Language Elements 13-109
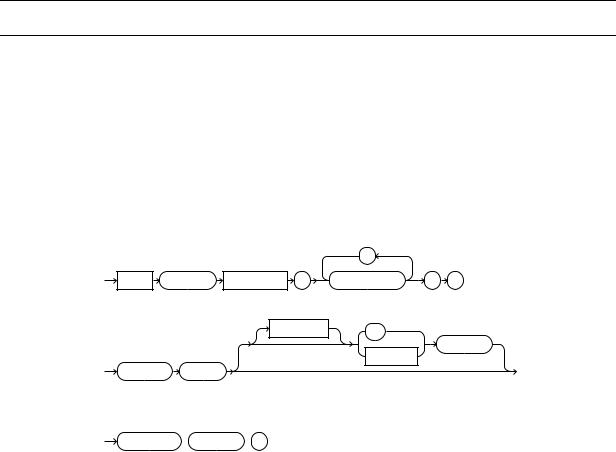
Records
Records
Records are composite variables that can store data values of different types, similar to a struct type in C, C++, or Java. For more information, see "What Is a PL/SQL Record?" on page 5-32.
In PL/SQL records are useful for holding data from table rows, or certain columns from table rows. For ease of maintenance, you can declare variables as table%ROWTYPE or cursor%ROWTYPE instead of creating new record types.
Syntax
record_type_definition |
|
|
, |
|
|
|
|
|
|
|
|
|
|
TYPE |
type_name |
IS RECORD |
( |
field_declaration |
) |
; |
field_declaration |
NOT NULL |
:= |
|
|
||
|
|
|
|
expression |
||
|
|
|
|
DEFAULT |
|
|
field_name |
datatype |
|
|
|
|
|
record_declaration
record_name type_name
;
Keyword and Parameter Description
datatype
A datatype specifier. For the syntax of datatype, see "Constants and Variables" on page 13-28.
expression
A combination of variables, constants, literals, operators, and function calls. The simplest expression consists of a single variable. For the syntax of expression, see "Expressions" on page 13-52. When the declaration is elaborated, the value of expression is assigned to the field. The value and the field must have compatible datatypes.
field_name
A field in a user-defined record.
NOT NULL
At run time, trying to assign a null to a field defined as NOT NULL raises the predefined exception VALUE_ERROR. The constraint NOT NULL must be followed by an initialization clause.
record_name
A user-defined record.
13-110 PL/SQL User's Guide and Reference

Records
type_name
A user-defined record type that was defined using the datatype specifier RECORD.
:= | DEFAULT
Initializes fields to default values.
Usage Notes
You can define RECORD types and declare user-defined records in the declarative part of any block, subprogram, or package.
A record can be initialized in its declaration:
DECLARE
TYPE TimeTyp IS RECORD ( seconds SMALLINT := 0, minutes SMALLINT := 0, hours SMALLINT := 0 );
You can use the %TYPE attribute to specify the datatype of a field. You can add the NOT NULL constraint to any field declaration to prevent the assigning of nulls to that field. Fields declared as NOT NULL must be initialized.
DECLARE
TYPE DeptRecTyp IS RECORD
(
deptno |
NUMBER(2) NOT NULL := 99, |
dname |
departments.department_name%TYPE, |
loc |
departments.location_id%TYPE, |
region |
regions%ROWTYPE |
);
dept_rec DeptRecTyp; BEGIN
dept_rec.dname := 'PURCHASING'; END;
/
To reference individual fields in a record, you use dot notation. For example, you might assign a value to the field dname in the record dept_rec as follows:
dept_rec.dname := 'PURCHASING';
Instead of assigning values separately to each field in a record, you can assign values to all fields at once:
■You can assign one user-defined record to another if they have the same datatype. (Having fields that match exactly is not enough.) You can assign a %ROWTYPE record to a user-defined record if their fields match in number and order, and corresponding fields have compatible datatypes.
■You can use the SELECT or FETCH statement to fetch column values into a record. The columns in the select-list must appear in the same order as the fields in your record.
You can declare and reference nested records. That is, a record can be the component of another record:
DECLARE |
|
|
|
TYPE TimeTyp IS RECORD ( minutes SMALLINT, hours |
SMALLINT ); |
||
TYPE MeetingTyp IS RECORD ( |
|
|
|
day |
DATE, |
|
|
time_of TimeTyp, |
-- nested record |
|
|
dept |
departments%ROWTYPE, -- nested record |
representing a table row |
PL/SQL Language Elements 13-111

Records
place VARCHAR2(20), purpose VARCHAR2(50) );
meeting MeetingTyp; seminar MeetingTyp;
BEGIN
seminar.time_of := meeting.time_of; END;
/
You can assign one nested record to another if they have the same datatype:
seminar.time_of := meeting.time_of;
Such assignments are allowed even if the containing records have different datatypes.
User-defined records follow the usual scoping and instantiation rules. In a package, they are instantiated when you first reference the package and cease to exist when you end the database session. In a block or subprogram, they are instantiated when you enter the block or subprogram and cease to exist when you exit the block or subprogram.
Like scalar variables, user-defined records can be declared as the formal parameters of procedures and functions. The restrictions that apply to scalar parameters also apply to user-defined records.
You can specify a RECORD type in the RETURN clause of a function spec. That allows the function to return a user-defined record of the same type. When calling a function that returns a user-defined record, use the following syntax to reference fields in the record:
function_name(parameter_list).field_name
To reference nested fields, use this syntax:
function_name(parameter_list).field_name.nested_field_name
If the function takes no parameters, code an empty parameter list. The syntax follows:
function_name().field_name
Example
The following example defines a RECORD type named DeptRecTyp, declares a record named dept_rec, then selects a row of values into the record:
DECLARE
TYPE DeptRecTyp IS RECORD (
deptno departments.department_id%TYPE,
dname |
departments.department_name%TYPE, |
loc |
departments.location_id%TYPE ); |
dept_rec DeptRecTyp; BEGIN
SELECT department_id, department_name, location_id INTO dept_rec FROM departments WHERE department_id = 20;
END;
/
Related Topics
Collections, Functions, Packages, Procedures
13-112 PL/SQL User's Guide and Reference