
- •Contents
- •Send Us Your Comments
- •Preface
- •What's New in PL/SQL?
- •1 Overview of PL/SQL
- •Advantages of PL/SQL
- •Tight Integration with SQL
- •Support for SQL
- •Better Performance
- •Higher Productivity
- •Full Portability
- •Tight Security
- •Support for Object-Oriented Programming
- •Understanding the Main Features of PL/SQL
- •Block Structure
- •Variables and Constants
- •Processing Queries with PL/SQL
- •Declaring PL/SQL Variables
- •Control Structures
- •Writing Reusable PL/SQL Code
- •Data Abstraction
- •Error Handling
- •PL/SQL Architecture
- •In the Oracle Database Server
- •In Oracle Tools
- •2 Fundamentals of the PL/SQL Language
- •Character Set
- •Lexical Units
- •Delimiters
- •Literals
- •Comments
- •Declarations
- •Using DEFAULT
- •Using NOT NULL
- •Using the %TYPE Attribute
- •Using the %ROWTYPE Attribute
- •Restrictions on Declarations
- •PL/SQL Naming Conventions
- •Scope and Visibility of PL/SQL Identifiers
- •Assigning Values to Variables
- •Assigning Boolean Values
- •Assigning a SQL Query Result to a PL/SQL Variable
- •PL/SQL Expressions and Comparisons
- •Logical Operators
- •Boolean Expressions
- •CASE Expressions
- •Handling Null Values in Comparisons and Conditional Statements
- •Summary of PL/SQL Built-In Functions
- •3 PL/SQL Datatypes
- •PL/SQL Number Types
- •PL/SQL Character and String Types
- •PL/SQL National Character Types
- •PL/SQL LOB Types
- •PL/SQL Boolean Types
- •PL/SQL Date, Time, and Interval Types
- •Datetime and Interval Arithmetic
- •Avoiding Truncation Problems Using Date and Time Subtypes
- •Overview of PL/SQL Subtypes
- •Using Subtypes
- •Converting PL/SQL Datatypes
- •Explicit Conversion
- •Implicit Conversion
- •Choosing Between Implicit and Explicit Conversion
- •DATE Values
- •RAW and LONG RAW Values
- •4 Using PL/SQL Control Structures
- •Overview of PL/SQL Control Structures
- •Testing Conditions: IF and CASE Statements
- •Using the IF-THEN Statement
- •Using the IF-THEN-ELSE Statement
- •Using the IF-THEN-ELSIF Statement
- •Using the CASE Statement
- •Guidelines for PL/SQL Conditional Statements
- •Controlling Loop Iterations: LOOP and EXIT Statements
- •Using the LOOP Statement
- •Using the EXIT Statement
- •Using the EXIT-WHEN Statement
- •Labeling a PL/SQL Loop
- •Using the WHILE-LOOP Statement
- •Using the FOR-LOOP Statement
- •Sequential Control: GOTO and NULL Statements
- •Using the GOTO Statement
- •Using the NULL Statement
- •5 Using PL/SQL Collections and Records
- •What Is a Collection?
- •Understanding Nested Tables
- •Understanding Varrays
- •Understanding Associative Arrays (Index-By Tables)
- •How Globalization Settings Affect VARCHAR2 Keys for Associative Arrays
- •Choosing Which PL/SQL Collection Types to Use
- •Choosing Between Nested Tables and Associative Arrays
- •Choosing Between Nested Tables and Varrays
- •Defining Collection Types
- •Declaring PL/SQL Collection Variables
- •Initializing and Referencing Collections
- •Referencing Collection Elements
- •Assigning Collections
- •Comparing Collections
- •Using PL/SQL Collections with SQL Statements
- •Using PL/SQL Varrays with INSERT, UPDATE, and SELECT Statements
- •Manipulating Individual Collection Elements with SQL
- •Using Multilevel Collections
- •Using Collection Methods
- •Checking If a Collection Element Exists (EXISTS Method)
- •Counting the Elements in a Collection (COUNT Method)
- •Checking the Maximum Size of a Collection (LIMIT Method)
- •Finding the First or Last Collection Element (FIRST and LAST Methods)
- •Looping Through Collection Elements (PRIOR and NEXT Methods)
- •Increasing the Size of a Collection (EXTEND Method)
- •Decreasing the Size of a Collection (TRIM Method)
- •Deleting Collection Elements (DELETE Method)
- •Applying Methods to Collection Parameters
- •Avoiding Collection Exceptions
- •What Is a PL/SQL Record?
- •Using Records as Procedure Parameters and Function Return Values
- •Assigning Values to Records
- •Comparing Records
- •Inserting PL/SQL Records into the Database
- •Updating the Database with PL/SQL Record Values
- •Restrictions on Record Inserts/Updates
- •Querying Data into Collections of Records
- •6 Performing SQL Operations from PL/SQL
- •Overview of SQL Support in PL/SQL
- •Data Manipulation
- •Transaction Control
- •SQL Functions
- •SQL Pseudocolumns
- •SQL Operators
- •Performing DML Operations from PL/SQL (INSERT, UPDATE, and DELETE)
- •Overview of Implicit Cursor Attributes
- •Using PL/SQL Records in SQL INSERT and UPDATE Statements
- •Issuing Queries from PL/SQL
- •Selecting At Most One Row: SELECT INTO Statement
- •Selecting Multiple Rows: BULK COLLECT Clause
- •Looping Through Multiple Rows: Cursor FOR Loop
- •Performing Complicated Query Processing: Explicit Cursors
- •Querying Data with PL/SQL
- •Querying Data with PL/SQL: Implicit Cursor FOR Loop
- •Querying Data with PL/SQL: Explicit Cursor FOR Loops
- •Overview of Explicit Cursors
- •Using Subqueries
- •Using Correlated Subqueries
- •Writing Maintainable PL/SQL Queries
- •Using Cursor Attributes
- •Overview of Explicit Cursor Attributes
- •Using Cursor Variables (REF CURSORs)
- •What Are Cursor Variables (REF CURSORs)?
- •Why Use Cursor Variables?
- •Declaring REF CURSOR Types and Cursor Variables
- •Controlling Cursor Variables: OPEN-FOR, FETCH, and CLOSE
- •Avoiding Errors with Cursor Variables
- •Restrictions on Cursor Variables
- •Using Cursor Expressions
- •Restrictions on Cursor Expressions
- •Example of Cursor Expressions
- •Constructing REF CURSORs with Cursor Subqueries
- •Overview of Transaction Processing in PL/SQL
- •Using COMMIT, SAVEPOINT, and ROLLBACK in PL/SQL
- •How Oracle Does Implicit Rollbacks
- •Ending Transactions
- •Setting Transaction Properties with SET TRANSACTION
- •Overriding Default Locking
- •Doing Independent Units of Work with Autonomous Transactions
- •Advantages of Autonomous Transactions
- •Controlling Autonomous Transactions
- •Using Autonomous Triggers
- •Calling Autonomous Functions from SQL
- •7 Performing SQL Operations with Native Dynamic SQL
- •What Is Dynamic SQL?
- •Why Use Dynamic SQL?
- •Using the EXECUTE IMMEDIATE Statement
- •Specifying Parameter Modes for Bind Variables in Dynamic SQL Strings
- •Building a Dynamic Query with Dynamic SQL
- •Examples of Dynamic SQL for Records, Objects, and Collections
- •Using Bulk Dynamic SQL
- •Using Dynamic SQL with Bulk SQL
- •Examples of Dynamic Bulk Binds
- •Guidelines for Dynamic SQL
- •When to Use or Omit the Semicolon with Dynamic SQL
- •Improving Performance of Dynamic SQL with Bind Variables
- •Passing Schema Object Names As Parameters
- •Using Duplicate Placeholders with Dynamic SQL
- •Using Cursor Attributes with Dynamic SQL
- •Passing Nulls to Dynamic SQL
- •Using Database Links with Dynamic SQL
- •Using Invoker Rights with Dynamic SQL
- •Using Pragma RESTRICT_REFERENCES with Dynamic SQL
- •Avoiding Deadlocks with Dynamic SQL
- •Backward Compatibility of the USING Clause
- •8 Using PL/SQL Subprograms
- •What Are Subprograms?
- •Advantages of PL/SQL Subprograms
- •Understanding PL/SQL Procedures
- •Understanding PL/SQL Functions
- •Using the RETURN Statement
- •Declaring Nested PL/SQL Subprograms
- •Passing Parameters to PL/SQL Subprograms
- •Actual Versus Formal Subprogram Parameters
- •Using Positional, Named, or Mixed Notation for Subprogram Parameters
- •Specifying Subprogram Parameter Modes
- •Using Default Values for Subprogram Parameters
- •Overloading Subprogram Names
- •Guidelines for Overloading with Numeric Types
- •Restrictions on Overloading
- •How Subprogram Calls Are Resolved
- •How Overloading Works with Inheritance
- •Using Invoker's Rights Versus Definer's Rights (AUTHID Clause)
- •Advantages of Invoker's Rights
- •Specifying the Privileges for a Subprogram with the AUTHID Clause
- •Who Is the Current User During Subprogram Execution?
- •How External References Are Resolved in Invoker's Rights Subprograms
- •Overriding Default Name Resolution in Invoker's Rights Subprograms
- •Granting Privileges on Invoker's Rights Subprograms
- •Using Roles with Invoker's Rights Subprograms
- •Using Views and Database Triggers with Invoker's Rights Subprograms
- •Using Database Links with Invoker's Rights Subprograms
- •Using Object Types with Invoker's Rights Subprograms
- •Using Recursion with PL/SQL
- •What Is a Recursive Subprogram?
- •Calling External Subprograms
- •Creating Dynamic Web Pages with PL/SQL Server Pages
- •Controlling Side Effects of PL/SQL Subprograms
- •Understanding Subprogram Parameter Aliasing
- •9 Using PL/SQL Packages
- •What Is a PL/SQL Package?
- •What Goes In a PL/SQL Package?
- •Example of a PL/SQL Package
- •Advantages of PL/SQL Packages
- •Understanding The Package Specification
- •Referencing Package Contents
- •Understanding The Package Body
- •Some Examples of Package Features
- •Private Versus Public Items in Packages
- •Overloading Packaged Subprograms
- •How Package STANDARD Defines the PL/SQL Environment
- •About the DBMS_ALERT Package
- •About the DBMS_OUTPUT Package
- •About the DBMS_PIPE Package
- •About the UTL_FILE Package
- •About the UTL_HTTP Package
- •Guidelines for Writing Packages
- •Separating Cursor Specs and Bodies with Packages
- •10 Handling PL/SQL Errors
- •Overview of PL/SQL Runtime Error Handling
- •Guidelines for Avoiding and Handling PL/SQL Errors and Exceptions
- •Advantages of PL/SQL Exceptions
- •Summary of Predefined PL/SQL Exceptions
- •Defining Your Own PL/SQL Exceptions
- •Declaring PL/SQL Exceptions
- •Scope Rules for PL/SQL Exceptions
- •Associating a PL/SQL Exception with a Number: Pragma EXCEPTION_INIT
- •How PL/SQL Exceptions Are Raised
- •Raising Exceptions with the RAISE Statement
- •How PL/SQL Exceptions Propagate
- •Reraising a PL/SQL Exception
- •Handling Raised PL/SQL Exceptions
- •Handling Exceptions Raised in Declarations
- •Handling Exceptions Raised in Handlers
- •Branching to or from an Exception Handler
- •Retrieving the Error Code and Error Message: SQLCODE and SQLERRM
- •Catching Unhandled Exceptions
- •Tips for Handling PL/SQL Errors
- •Continuing after an Exception Is Raised
- •Retrying a Transaction
- •Using Locator Variables to Identify Exception Locations
- •Overview of PL/SQL Compile-Time Warnings
- •PL/SQL Warning Categories
- •Controlling PL/SQL Warning Messages
- •Using the DBMS_WARNING Package
- •11 Tuning PL/SQL Applications for Performance
- •How PL/SQL Optimizes Your Programs
- •When to Tune PL/SQL Code
- •Guidelines for Avoiding PL/SQL Performance Problems
- •Avoiding CPU Overhead in PL/SQL Code
- •Avoiding Memory Overhead in PL/SQL Code
- •Profiling and Tracing PL/SQL Programs
- •Using The Trace API: Package DBMS_TRACE
- •Reducing Loop Overhead for DML Statements and Queries (FORALL, BULK COLLECT)
- •Using the FORALL Statement
- •Retrieving Query Results into Collections with the BULK COLLECT Clause
- •Writing Computation-Intensive Programs in PL/SQL
- •Tuning Dynamic SQL with EXECUTE IMMEDIATE and Cursor Variables
- •Tuning PL/SQL Procedure Calls with the NOCOPY Compiler Hint
- •Restrictions on NOCOPY
- •Compiling PL/SQL Code for Native Execution
- •Setting Up Transformation Pipelines with Table Functions
- •Overview of Table Functions
- •Using Pipelined Table Functions for Transformations
- •Writing a Pipelined Table Function
- •Returning Results from Table Functions
- •Pipelining Data Between PL/SQL Table Functions
- •Querying Table Functions
- •Optimizing Multiple Calls to Table Functions
- •Fetching from the Results of Table Functions
- •Passing Data with Cursor Variables
- •Performing DML Operations Inside Table Functions
- •Performing DML Operations on Table Functions
- •Handling Exceptions in Table Functions
- •12 Using PL/SQL Object Types
- •Overview of PL/SQL Object Types
- •What Is an Object Type?
- •Why Use Object Types?
- •Structure of an Object Type
- •Components of an Object Type
- •What Languages can I Use for Methods of Object Types?
- •How Object Types Handle the SELF Parameter
- •Overloading
- •Changing Attributes and Methods of an Existing Object Type (Type Evolution)
- •Defining Object Types
- •Overview of PL/SQL Type Inheritance
- •Declaring and Initializing Objects
- •Declaring Objects
- •Initializing Objects
- •How PL/SQL Treats Uninitialized Objects
- •Accessing Object Attributes
- •Defining Object Constructors
- •Calling Object Constructors
- •Calling Object Methods
- •Sharing Objects through the REF Modifier
- •Manipulating Objects through SQL
- •Selecting Objects
- •Inserting Objects
- •Updating Objects
- •Deleting Objects
- •13 PL/SQL Language Elements
- •Assignment Statement
- •AUTONOMOUS_TRANSACTION Pragma
- •Blocks
- •CASE Statement
- •CLOSE Statement
- •Collection Methods
- •Collections
- •Comments
- •COMMIT Statement
- •Constants and Variables
- •Cursor Attributes
- •Cursor Variables
- •Cursors
- •DELETE Statement
- •EXCEPTION_INIT Pragma
- •Exceptions
- •EXECUTE IMMEDIATE Statement
- •EXIT Statement
- •Expressions
- •FETCH Statement
- •FORALL Statement
- •Functions
- •GOTO Statement
- •IF Statement
- •INSERT Statement
- •Literals
- •LOCK TABLE Statement
- •LOOP Statements
- •MERGE Statement
- •NULL Statement
- •Object Types
- •OPEN Statement
- •OPEN-FOR Statement
- •OPEN-FOR-USING Statement
- •Packages
- •Procedures
- •RAISE Statement
- •Records
- •RESTRICT_REFERENCES Pragma
- •RETURN Statement
- •ROLLBACK Statement
- •%ROWTYPE Attribute
- •SAVEPOINT Statement
- •SCN_TO_TIMESTAMP Function
- •SELECT INTO Statement
- •SERIALLY_REUSABLE Pragma
- •SET TRANSACTION Statement
- •SQL Cursor
- •SQLCODE Function
- •SQLERRM Function
- •TIMESTAMP_TO_SCN Function
- •%TYPE Attribute
- •UPDATE Statement
- •Where to Find PL/SQL Sample Programs
- •Exercises for the Reader
- •Assigning Character Values
- •Comparing Character Values
- •Inserting Character Values
- •Selecting Character Values
- •Advantages of Wrapping PL/SQL Procedures
- •Running the PL/SQL Wrap Utility
- •Input and Output Files for the PL/SQL Wrap Utility
- •Limitations of the PL/SQL Wrap Utility
- •What Is Name Resolution?
- •Examples of Qualified Names and Dot Notation
- •Differences in Name Resolution Between SQL and PL/SQL
- •Understanding Capture
- •Inner Capture
- •Same-Scope Capture
- •Outer Capture
- •Avoiding Inner Capture in DML Statements
- •Qualifying References to Object Attributes and Methods
- •Calling Parameterless Subprograms and Methods
- •Name Resolution for SQL Versus PL/SQL
- •When Should I Use Bind Variables with PL/SQL?
- •When Do I Use or Omit the Semicolon with Dynamic SQL?
- •How Can I Use Regular Expressions with PL/SQL?
- •How Do I Continue After a PL/SQL Exception?
- •How Do I Pass a Result Set from PL/SQL to Java or Visual Basic (VB)?
- •How Do I Specify Different Kinds of Names with PL/SQL's Dot Notation?
- •What Can I Do with Objects and Object Types in PL/SQL?
- •How Do I Create a PL/SQL Procedure?
- •How Do I Input or Output Data with PL/SQL?
- •How Do I Perform a Case-Insensitive Query?
- •Index
- •Symbols

Avoiding Inner Capture in DML Statements
In the following example, the reference to col2 in the inner SELECT statement binds to column col2 in table tab1 because table tab2 has no column named col2:
CREATE TABLE tab1 (col1 NUMBER, col2 NUMBER); CREATE TABLE tab2 (col1 NUMBER);
CREATE PROCEDURE proc AS
CURSOR c1 IS SELECT * FROM tab1
WHERE EXISTS (SELECT * FROM tab2 WHERE col2 = 10);
BEGIN
...
END;
In the preceding example, if you add a column named col2 to table tab2:
ALTER TABLE tab2 ADD (col2 NUMBER);
then procedure proc is invalidated and recompiled automatically upon next use. However, upon recompilation, the col2 in the inner SELECT statement binds to column col2 in table tab2 because tab2 is in the inner scope. Thus, the reference to col2 is captured by the addition of column col2 to table tab2.
Using collections and object types can cause more inner capture situations. In the following example, the reference to s.tab2.a resolves to attribute a of column tab2 in table tab1 through table alias s, which is visible in the outer scope of the query:
CREATE TYPE type1 AS OBJECT (a NUMBER); CREATE TABLE tab1 (tab2 type1);
CREATE TABLE tab2 (x NUMBER);
SELECT * FROM tab1 s -- alias with same name as schema name WHERE EXISTS (SELECT * FROM s.tab2 WHERE x = s.tab2.a);
-- note lack of alias
In the preceding example, you might add a column named a to table s.tab2, which appears in the inner subquery. When the query is processed, an inner capture occurs because the reference to s.tab2.a resolves to column a of table tab2 in schema s. You can avoid inner captures by following the rules given in "Avoiding Inner Capture in DML Statements" on page D-4. According to those rules, you should revise the query as follows:
SELECT * FROM s.tab1 p1
WHERE EXISTS (SELECT * FROM s.tab2 p2 WHERE p2.x = p1.tab2.a);
Same-Scope Capture
In SQL scope, a same-scope capture occurs when a column is added to one of two tables used in a join, so that the same column name exists in both tables. Previously, you could refer to that column name in a join query. To avoid an error, now you must qualify the column name with the table name.
Outer Capture
An outer capture occurs when a name in an inner scope, which once resolved to an entity in an inner scope, is resolved to an entity in an outer scope. SQL and PL/SQL are designed to prevent outer captures. You do not need to take any action to avoid this condition.
Avoiding Inner Capture in DML Statements
You can avoid inner capture in DML statements by following these rules:
D-4 PL/SQL User's Guide and Reference

Calling Parameterless Subprograms and Methods
■
■
■
■
Specify an alias for each table in the DML statement.
Keep table aliases unique throughout the DML statement.
Avoid table aliases that match schema names used in the query.
Qualify each column reference with the table alias.
Qualifying a reference with schema_name.table_name does not prevent inner capture if the statement refers to tables with columns of a user-defined object type.
Qualifying References to Object Attributes and Methods
Columns of a user-defined object type allow for more inner capture situations. To minimize problems, the name-resolution algorithm includes the following rules:
■All references to attributes and methods must be qualified by a table alias. When referencing a table, if you reference the attributes or methods of an object stored in that table, the table name must be accompanied by an alias. As the following examples show, column-qualified references to an attribute or method are not allowed if they are prefixed with a table name:
CREATE TYPE t1 AS OBJECT (x NUMBER); |
|
CREATE TABLE tb1 (col t1); |
|
SELECT col.x FROM tb1; |
-- not allowed |
SELECT tb1.col.x FROM tb1; |
-- not allowed |
SELECT scott.tb1.col.x FROM scott.tb1; |
-- not allowed |
SELECT t.col.x FROM tb1 t; |
|
UPDATE tb1 SET col.x = 10; |
-- not allowed |
UPDATE scott.tb1 SET scott.tb1.col.x=10; |
-- not allowed |
UPDATE tb1 t set t.col.x = 1; |
|
DELETE FROM tb1 WHERE tb1.col.x = 10; |
-- not allowed |
DELETE FROM tb1 t WHERE t.col.x = 10; |
|
■Row expressions must resolve as references to table aliases. You can pass row expressions to operators REF and VALUE, and you can use row expressions in the SET clause of an UPDATE statement. Some examples follow:
CREATE TYPE t1 AS OBJECT (x number); |
|
CREATE TABLE ot1 OF t1; |
-- object table |
SELECT REF(ot1) FROM ot1; |
-- not allowed |
SELECT REF(o) FROM ot1 o; |
|
SELECT VALUE(ot1) FROM ot1; |
-- not allowed |
SELECT VALUE(o) FROM ot1 o; |
|
DELETE FROM ot1 WHERE VALUE(ot1) = (t1(10)); |
-- not allowed |
DELETE FROM ot1 o WHERE VALUE(o) = (t1(10)); |
|
UPDATE ot1 SET ot1 = ... |
-- not allowed |
UPDATE ot1 o SET o = .... |
|
The following ways to insert into an object table are allowed and do not require an alias because there is no column list:
INSERT |
INTO |
ot1 |
VALUES |
(t1(10)); -- |
no |
row |
expression |
|
INSERT |
INTO |
ot1 |
VALUES |
(10); |
-- |
no |
row |
expression |
Calling Parameterless Subprograms and Methods
If a subprogram does not take any parameters, you can include an empty set of parentheses or omit the parentheses, both in PL/SQL and in functions called from SQL queries.
How PL/SQL Resolves Identifier Names D-5

Name Resolution for SQL Versus PL/SQL
For calls to a method that takes no parameters, an empty set of parentheses is optional within PL/SQL scopes but required within SQL scopes.
Name Resolution for SQL Versus PL/SQL
The name-resolution rules for SQL and PL/SQL are similar. You can avoid the few minor differences if you follow the capture avoidance rules.
For compatibility, the SQL rules are more permissive than the PL/SQL rules. That is, the SQL rules, which are mostly context sensitive, recognize as legal more situations and DML statements than the PL/SQL rules do.
D-6 PL/SQL User's Guide and Reference
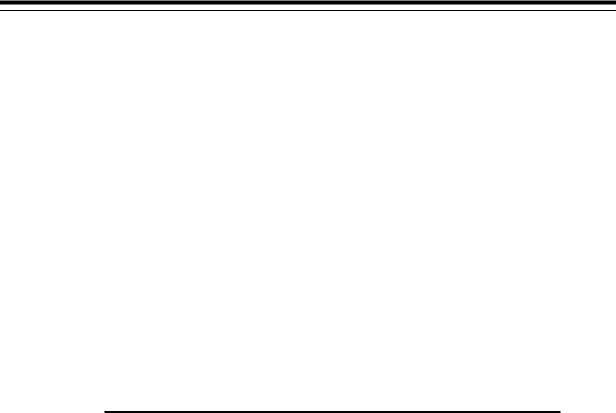
E
PL/SQL Program Limits
This appendix discusses the program limits that are imposed by the PL/SQL language.
PL/SQL is based on the programming language Ada. As a result, PL/SQL uses a variant of Descriptive Intermediate Attributed Notation for Ada (DIANA), a tree-structured intermediate language. It is defined using a meta-notation called Interface Definition Language (IDL). DIANA is used internally by compilers and other tools.
At compile time, PL/SQL source code is translated into machine-readable m-code. Both the DIANA and m-code for a procedure or package are stored in the database. At run time, they are loaded into the shared memory pool. The DIANA is used to compile dependent procedures; the m-code is simply executed.
In the shared memory pool, a package spec, object type spec, standalone subprogram, or anonymous block is limited to 2**26 DIANA nodes (which correspond to tokens such as identifiers, keywords, operators, and so on). This allows for ~6,000,000 lines of code unless you exceed limits imposed by the PL/SQL compiler, some of which are given in Table E–1.
Table E–1 PL/SQL Compiler Limits
Item |
Limit |
|
|
bind variables passed to a program unit |
32K |
exception handlers in a program unit |
64K |
fields in a record |
64K |
levels of block nesting |
255 |
levels of record nesting |
32 |
levels of subquery nesting |
254 |
levels of label nesting |
98 |
magnitude of a BINARY_INTEGER value |
2G |
magnitude of a PLS_INTEGER value |
2G |
objects referenced by a program unit |
64K |
parameters passed to an explicit cursor |
64K |
parameters passed to a function or procedure |
64K |
precision of a FLOAT value (binary digits) |
126 |
precision of a NUMBER value (decimal digits) |
38 |
PL/SQL Program Limits E-1
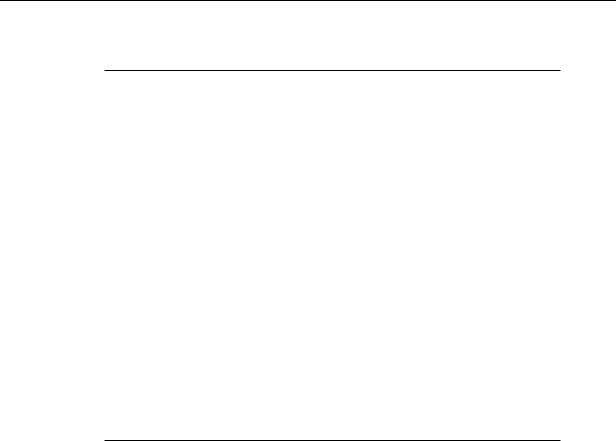
Table E–1 |
(Cont.) PL/SQL Compiler Limits |
|
|
Item |
Limit |
precision of a REAL value (binary digits) size of an identifier (characters)
size of a string literal (bytes) size of a CHAR value (bytes) size of a LONG value (bytes) size of a LONG RAW value (bytes) size of a RAW value (bytes)
size of a VARCHAR2 value (bytes) size of an NCHAR value (bytes)
size of an NVARCHAR2 value (bytes) size of a BFILE value (bytes)
size of a BLOB value (bytes)
size of a CLOB value (bytes)
size of an NCLOB value (bytes)
63
30
32K
32K
32K-7
32K-7
32K
32K
32K
32K
4G * value of DB_BLOCK_SIZE parameter
4G * value of DB_BLOCK_SIZE parameter
4G * value of DB_BLOCK_SIZE parameter
4G * value of DB_BLOCK_SIZE parameter
To estimate how much memory a program unit requires, you can query the data dictionary view user_object_size. The column parsed_size returns the size (in bytes) of the "flattened" DIANA. For example:
SQL> SELECT * FROM user_object_size WHERE name = 'PKG1';
NAME TYPE SOURCE_SIZE PARSED_SIZE CODE_SIZE ERROR_SIZE
--------------------------------------------------------------------
PKG1 |
PACKAGE |
46 |
165 |
119 |
0 |
PKG1 |
PACKAGE BODY |
82 |
0 |
139 |
0 |
Unfortunately, you cannot estimate the number of DIANA nodes from the parsed size. Two program units with the same parsed size might require 1500 and 2000 DIANA nodes, respectively (because, for example, the second unit contains more complex SQL statements).
When a PL/SQL block, subprogram, package, or object type exceeds a size limit, you get an error such as program too large. Typically, this problem occurs with packages or anonymous blocks. With a package, the best solution is to divide it into smaller packages. With an anonymous block, the best solution is to redefine it as a group of subprograms, which can be stored in the database.
E-2 PL/SQL User's Guide and Reference

F
List of PL/SQL Reserved Words
The words listed in this appendix are reserved by PL/SQL. You should not use them to name program objects such as constants, variables, or cursors. Some of these words (marked by an asterisk) are also reserved by SQL. You should not use them to name schema objects such as columns, tables, or indexes.
List of PL/SQL Reserved Words F-1

ALL* |
FUNCTION |
PLS_INTEGER |
VARCHAR* |
ALTER* |
GOTO |
POSITIVE |
VARCHAR2* |
AND* |
GROUP* |
POSITIVEN |
VARIANCE |
ANY* |
HAVING* |
PRAGMA |
VIEW* |
ARRAY |
HEAP |
PRIOR* |
WHEN |
AS* |
HOUR |
PRIVATE |
WHENEVER* |
ASC* |
IF |
PROCEDURE |
WHERE* |
AT |
IMMEDIATE* |
PUBLIC* |
WHILE |
AUTHID |
IN* |
RAISE |
WITH* |
AVG |
INDEX* |
RANGE |
WORK |
BEGIN |
INDICATOR |
RAW* |
WRITE |
BETWEEN* |
INSERT* |
REAL |
YEAR |
BINARY_INTEGER |
INTEGER* |
RECORD |
ZONE |
BODY |
INTERFACE |
REF |
|
BOOLEAN |
INTERSECT* |
RELEASE |
|
BULK |
INTERVAL |
RETURN |
|
BY* |
INTO* |
REVERSE |
|
CASE |
IS* |
ROLLBACK |
|
CHAR* |
ISOLATION |
ROW* |
|
CHAR_BASE |
JAVA |
ROWID* |
|
CHECK* |
LEVEL* |
ROWNUM* |
|
CLOSE |
LIKE* |
ROWTYPE |
|
CLUSTER* |
LIMITED |
SAVEPOINT |
|
COALESCE |
LOCK* |
SECOND |
|
COLLECT |
LONG* |
SELECT* |
|
COMMENT* |
LOOP |
SEPARATE |
|
COMMIT |
MAX |
SET* |
|
COMPRESS* |
MIN |
SHARE* |
|
CONNECT* |
MINUS* |
SMALLINT* |
|
CONSTANT |
MINUTE |
SPACE |
|
CREATE* |
MLSLABEL* |
SQL |
|
CURRENT* |
MOD |
SQLCODE |
|
CURRVAL |
MODE* |
SQLERRM |
|
CURSOR |
MONTH |
START* |
|
DATE* |
NATURAL |
STDDEV |
|
DAY |
NATURALN |
SUBTYPE |
|
DECLARE |
NEW |
SUCCESSFUL* |
|
DECIMAL* |
NEXTVAL |
SUM |
|
DEFAULT* |
NOCOPY |
SYNONYM* |
|
DELETE* |
NOT* |
SYSDATE* |
|
DESC* |
NOWAIT* |
TABLE* |
|
DISTINCT* |
NULL* |
THEN* |
|
DO |
NULLIF |
TIME |
|
DROP* |
NUMBER* |
TIMESTAMP |
|
ELSE* |
NUMBER_BASE |
TIMEZONE_REGION |
|
ELSIF |
OCIROWID |
TIMEZONE_ABBR |
|
END |
OF* |
TIMEZONE_MINUTE |
|
EXCEPTION |
ON* |
TIMEZONE_HOUR |
|
EXCLUSIVE* |
OPAQUE |
TO* |
|
EXECUTE |
OPEN |
TRIGGER* |
|
EXISTS* |
OPERATOR |
TRUE |
|
EXIT |
OPTION* |
TYPE |
|
EXTENDS |
OR* |
UID* |
|
EXTRACT |
ORDER* |
UNION* |
|
FALSE |
ORGANIZATION |
UNIQUE* |
|
FETCH |
OTHERS |
UPDATE* |
|
FLOAT* |
OUT |
USE |
|
FOR* |
PACKAGE |
USER* |
|
FORALL |
PARTITION |
VALIDATE* |
|
FROM* |
PCTFREE* |
VALUES* |
|
F-2 PL/SQL User's Guide and Reference