
- •Contents
- •Send Us Your Comments
- •Preface
- •What's New in PL/SQL?
- •1 Overview of PL/SQL
- •Advantages of PL/SQL
- •Tight Integration with SQL
- •Support for SQL
- •Better Performance
- •Higher Productivity
- •Full Portability
- •Tight Security
- •Support for Object-Oriented Programming
- •Understanding the Main Features of PL/SQL
- •Block Structure
- •Variables and Constants
- •Processing Queries with PL/SQL
- •Declaring PL/SQL Variables
- •Control Structures
- •Writing Reusable PL/SQL Code
- •Data Abstraction
- •Error Handling
- •PL/SQL Architecture
- •In the Oracle Database Server
- •In Oracle Tools
- •2 Fundamentals of the PL/SQL Language
- •Character Set
- •Lexical Units
- •Delimiters
- •Literals
- •Comments
- •Declarations
- •Using DEFAULT
- •Using NOT NULL
- •Using the %TYPE Attribute
- •Using the %ROWTYPE Attribute
- •Restrictions on Declarations
- •PL/SQL Naming Conventions
- •Scope and Visibility of PL/SQL Identifiers
- •Assigning Values to Variables
- •Assigning Boolean Values
- •Assigning a SQL Query Result to a PL/SQL Variable
- •PL/SQL Expressions and Comparisons
- •Logical Operators
- •Boolean Expressions
- •CASE Expressions
- •Handling Null Values in Comparisons and Conditional Statements
- •Summary of PL/SQL Built-In Functions
- •3 PL/SQL Datatypes
- •PL/SQL Number Types
- •PL/SQL Character and String Types
- •PL/SQL National Character Types
- •PL/SQL LOB Types
- •PL/SQL Boolean Types
- •PL/SQL Date, Time, and Interval Types
- •Datetime and Interval Arithmetic
- •Avoiding Truncation Problems Using Date and Time Subtypes
- •Overview of PL/SQL Subtypes
- •Using Subtypes
- •Converting PL/SQL Datatypes
- •Explicit Conversion
- •Implicit Conversion
- •Choosing Between Implicit and Explicit Conversion
- •DATE Values
- •RAW and LONG RAW Values
- •4 Using PL/SQL Control Structures
- •Overview of PL/SQL Control Structures
- •Testing Conditions: IF and CASE Statements
- •Using the IF-THEN Statement
- •Using the IF-THEN-ELSE Statement
- •Using the IF-THEN-ELSIF Statement
- •Using the CASE Statement
- •Guidelines for PL/SQL Conditional Statements
- •Controlling Loop Iterations: LOOP and EXIT Statements
- •Using the LOOP Statement
- •Using the EXIT Statement
- •Using the EXIT-WHEN Statement
- •Labeling a PL/SQL Loop
- •Using the WHILE-LOOP Statement
- •Using the FOR-LOOP Statement
- •Sequential Control: GOTO and NULL Statements
- •Using the GOTO Statement
- •Using the NULL Statement
- •5 Using PL/SQL Collections and Records
- •What Is a Collection?
- •Understanding Nested Tables
- •Understanding Varrays
- •Understanding Associative Arrays (Index-By Tables)
- •How Globalization Settings Affect VARCHAR2 Keys for Associative Arrays
- •Choosing Which PL/SQL Collection Types to Use
- •Choosing Between Nested Tables and Associative Arrays
- •Choosing Between Nested Tables and Varrays
- •Defining Collection Types
- •Declaring PL/SQL Collection Variables
- •Initializing and Referencing Collections
- •Referencing Collection Elements
- •Assigning Collections
- •Comparing Collections
- •Using PL/SQL Collections with SQL Statements
- •Using PL/SQL Varrays with INSERT, UPDATE, and SELECT Statements
- •Manipulating Individual Collection Elements with SQL
- •Using Multilevel Collections
- •Using Collection Methods
- •Checking If a Collection Element Exists (EXISTS Method)
- •Counting the Elements in a Collection (COUNT Method)
- •Checking the Maximum Size of a Collection (LIMIT Method)
- •Finding the First or Last Collection Element (FIRST and LAST Methods)
- •Looping Through Collection Elements (PRIOR and NEXT Methods)
- •Increasing the Size of a Collection (EXTEND Method)
- •Decreasing the Size of a Collection (TRIM Method)
- •Deleting Collection Elements (DELETE Method)
- •Applying Methods to Collection Parameters
- •Avoiding Collection Exceptions
- •What Is a PL/SQL Record?
- •Using Records as Procedure Parameters and Function Return Values
- •Assigning Values to Records
- •Comparing Records
- •Inserting PL/SQL Records into the Database
- •Updating the Database with PL/SQL Record Values
- •Restrictions on Record Inserts/Updates
- •Querying Data into Collections of Records
- •6 Performing SQL Operations from PL/SQL
- •Overview of SQL Support in PL/SQL
- •Data Manipulation
- •Transaction Control
- •SQL Functions
- •SQL Pseudocolumns
- •SQL Operators
- •Performing DML Operations from PL/SQL (INSERT, UPDATE, and DELETE)
- •Overview of Implicit Cursor Attributes
- •Using PL/SQL Records in SQL INSERT and UPDATE Statements
- •Issuing Queries from PL/SQL
- •Selecting At Most One Row: SELECT INTO Statement
- •Selecting Multiple Rows: BULK COLLECT Clause
- •Looping Through Multiple Rows: Cursor FOR Loop
- •Performing Complicated Query Processing: Explicit Cursors
- •Querying Data with PL/SQL
- •Querying Data with PL/SQL: Implicit Cursor FOR Loop
- •Querying Data with PL/SQL: Explicit Cursor FOR Loops
- •Overview of Explicit Cursors
- •Using Subqueries
- •Using Correlated Subqueries
- •Writing Maintainable PL/SQL Queries
- •Using Cursor Attributes
- •Overview of Explicit Cursor Attributes
- •Using Cursor Variables (REF CURSORs)
- •What Are Cursor Variables (REF CURSORs)?
- •Why Use Cursor Variables?
- •Declaring REF CURSOR Types and Cursor Variables
- •Controlling Cursor Variables: OPEN-FOR, FETCH, and CLOSE
- •Avoiding Errors with Cursor Variables
- •Restrictions on Cursor Variables
- •Using Cursor Expressions
- •Restrictions on Cursor Expressions
- •Example of Cursor Expressions
- •Constructing REF CURSORs with Cursor Subqueries
- •Overview of Transaction Processing in PL/SQL
- •Using COMMIT, SAVEPOINT, and ROLLBACK in PL/SQL
- •How Oracle Does Implicit Rollbacks
- •Ending Transactions
- •Setting Transaction Properties with SET TRANSACTION
- •Overriding Default Locking
- •Doing Independent Units of Work with Autonomous Transactions
- •Advantages of Autonomous Transactions
- •Controlling Autonomous Transactions
- •Using Autonomous Triggers
- •Calling Autonomous Functions from SQL
- •7 Performing SQL Operations with Native Dynamic SQL
- •What Is Dynamic SQL?
- •Why Use Dynamic SQL?
- •Using the EXECUTE IMMEDIATE Statement
- •Specifying Parameter Modes for Bind Variables in Dynamic SQL Strings
- •Building a Dynamic Query with Dynamic SQL
- •Examples of Dynamic SQL for Records, Objects, and Collections
- •Using Bulk Dynamic SQL
- •Using Dynamic SQL with Bulk SQL
- •Examples of Dynamic Bulk Binds
- •Guidelines for Dynamic SQL
- •When to Use or Omit the Semicolon with Dynamic SQL
- •Improving Performance of Dynamic SQL with Bind Variables
- •Passing Schema Object Names As Parameters
- •Using Duplicate Placeholders with Dynamic SQL
- •Using Cursor Attributes with Dynamic SQL
- •Passing Nulls to Dynamic SQL
- •Using Database Links with Dynamic SQL
- •Using Invoker Rights with Dynamic SQL
- •Using Pragma RESTRICT_REFERENCES with Dynamic SQL
- •Avoiding Deadlocks with Dynamic SQL
- •Backward Compatibility of the USING Clause
- •8 Using PL/SQL Subprograms
- •What Are Subprograms?
- •Advantages of PL/SQL Subprograms
- •Understanding PL/SQL Procedures
- •Understanding PL/SQL Functions
- •Using the RETURN Statement
- •Declaring Nested PL/SQL Subprograms
- •Passing Parameters to PL/SQL Subprograms
- •Actual Versus Formal Subprogram Parameters
- •Using Positional, Named, or Mixed Notation for Subprogram Parameters
- •Specifying Subprogram Parameter Modes
- •Using Default Values for Subprogram Parameters
- •Overloading Subprogram Names
- •Guidelines for Overloading with Numeric Types
- •Restrictions on Overloading
- •How Subprogram Calls Are Resolved
- •How Overloading Works with Inheritance
- •Using Invoker's Rights Versus Definer's Rights (AUTHID Clause)
- •Advantages of Invoker's Rights
- •Specifying the Privileges for a Subprogram with the AUTHID Clause
- •Who Is the Current User During Subprogram Execution?
- •How External References Are Resolved in Invoker's Rights Subprograms
- •Overriding Default Name Resolution in Invoker's Rights Subprograms
- •Granting Privileges on Invoker's Rights Subprograms
- •Using Roles with Invoker's Rights Subprograms
- •Using Views and Database Triggers with Invoker's Rights Subprograms
- •Using Database Links with Invoker's Rights Subprograms
- •Using Object Types with Invoker's Rights Subprograms
- •Using Recursion with PL/SQL
- •What Is a Recursive Subprogram?
- •Calling External Subprograms
- •Creating Dynamic Web Pages with PL/SQL Server Pages
- •Controlling Side Effects of PL/SQL Subprograms
- •Understanding Subprogram Parameter Aliasing
- •9 Using PL/SQL Packages
- •What Is a PL/SQL Package?
- •What Goes In a PL/SQL Package?
- •Example of a PL/SQL Package
- •Advantages of PL/SQL Packages
- •Understanding The Package Specification
- •Referencing Package Contents
- •Understanding The Package Body
- •Some Examples of Package Features
- •Private Versus Public Items in Packages
- •Overloading Packaged Subprograms
- •How Package STANDARD Defines the PL/SQL Environment
- •About the DBMS_ALERT Package
- •About the DBMS_OUTPUT Package
- •About the DBMS_PIPE Package
- •About the UTL_FILE Package
- •About the UTL_HTTP Package
- •Guidelines for Writing Packages
- •Separating Cursor Specs and Bodies with Packages
- •10 Handling PL/SQL Errors
- •Overview of PL/SQL Runtime Error Handling
- •Guidelines for Avoiding and Handling PL/SQL Errors and Exceptions
- •Advantages of PL/SQL Exceptions
- •Summary of Predefined PL/SQL Exceptions
- •Defining Your Own PL/SQL Exceptions
- •Declaring PL/SQL Exceptions
- •Scope Rules for PL/SQL Exceptions
- •Associating a PL/SQL Exception with a Number: Pragma EXCEPTION_INIT
- •How PL/SQL Exceptions Are Raised
- •Raising Exceptions with the RAISE Statement
- •How PL/SQL Exceptions Propagate
- •Reraising a PL/SQL Exception
- •Handling Raised PL/SQL Exceptions
- •Handling Exceptions Raised in Declarations
- •Handling Exceptions Raised in Handlers
- •Branching to or from an Exception Handler
- •Retrieving the Error Code and Error Message: SQLCODE and SQLERRM
- •Catching Unhandled Exceptions
- •Tips for Handling PL/SQL Errors
- •Continuing after an Exception Is Raised
- •Retrying a Transaction
- •Using Locator Variables to Identify Exception Locations
- •Overview of PL/SQL Compile-Time Warnings
- •PL/SQL Warning Categories
- •Controlling PL/SQL Warning Messages
- •Using the DBMS_WARNING Package
- •11 Tuning PL/SQL Applications for Performance
- •How PL/SQL Optimizes Your Programs
- •When to Tune PL/SQL Code
- •Guidelines for Avoiding PL/SQL Performance Problems
- •Avoiding CPU Overhead in PL/SQL Code
- •Avoiding Memory Overhead in PL/SQL Code
- •Profiling and Tracing PL/SQL Programs
- •Using The Trace API: Package DBMS_TRACE
- •Reducing Loop Overhead for DML Statements and Queries (FORALL, BULK COLLECT)
- •Using the FORALL Statement
- •Retrieving Query Results into Collections with the BULK COLLECT Clause
- •Writing Computation-Intensive Programs in PL/SQL
- •Tuning Dynamic SQL with EXECUTE IMMEDIATE and Cursor Variables
- •Tuning PL/SQL Procedure Calls with the NOCOPY Compiler Hint
- •Restrictions on NOCOPY
- •Compiling PL/SQL Code for Native Execution
- •Setting Up Transformation Pipelines with Table Functions
- •Overview of Table Functions
- •Using Pipelined Table Functions for Transformations
- •Writing a Pipelined Table Function
- •Returning Results from Table Functions
- •Pipelining Data Between PL/SQL Table Functions
- •Querying Table Functions
- •Optimizing Multiple Calls to Table Functions
- •Fetching from the Results of Table Functions
- •Passing Data with Cursor Variables
- •Performing DML Operations Inside Table Functions
- •Performing DML Operations on Table Functions
- •Handling Exceptions in Table Functions
- •12 Using PL/SQL Object Types
- •Overview of PL/SQL Object Types
- •What Is an Object Type?
- •Why Use Object Types?
- •Structure of an Object Type
- •Components of an Object Type
- •What Languages can I Use for Methods of Object Types?
- •How Object Types Handle the SELF Parameter
- •Overloading
- •Changing Attributes and Methods of an Existing Object Type (Type Evolution)
- •Defining Object Types
- •Overview of PL/SQL Type Inheritance
- •Declaring and Initializing Objects
- •Declaring Objects
- •Initializing Objects
- •How PL/SQL Treats Uninitialized Objects
- •Accessing Object Attributes
- •Defining Object Constructors
- •Calling Object Constructors
- •Calling Object Methods
- •Sharing Objects through the REF Modifier
- •Manipulating Objects through SQL
- •Selecting Objects
- •Inserting Objects
- •Updating Objects
- •Deleting Objects
- •13 PL/SQL Language Elements
- •Assignment Statement
- •AUTONOMOUS_TRANSACTION Pragma
- •Blocks
- •CASE Statement
- •CLOSE Statement
- •Collection Methods
- •Collections
- •Comments
- •COMMIT Statement
- •Constants and Variables
- •Cursor Attributes
- •Cursor Variables
- •Cursors
- •DELETE Statement
- •EXCEPTION_INIT Pragma
- •Exceptions
- •EXECUTE IMMEDIATE Statement
- •EXIT Statement
- •Expressions
- •FETCH Statement
- •FORALL Statement
- •Functions
- •GOTO Statement
- •IF Statement
- •INSERT Statement
- •Literals
- •LOCK TABLE Statement
- •LOOP Statements
- •MERGE Statement
- •NULL Statement
- •Object Types
- •OPEN Statement
- •OPEN-FOR Statement
- •OPEN-FOR-USING Statement
- •Packages
- •Procedures
- •RAISE Statement
- •Records
- •RESTRICT_REFERENCES Pragma
- •RETURN Statement
- •ROLLBACK Statement
- •%ROWTYPE Attribute
- •SAVEPOINT Statement
- •SCN_TO_TIMESTAMP Function
- •SELECT INTO Statement
- •SERIALLY_REUSABLE Pragma
- •SET TRANSACTION Statement
- •SQL Cursor
- •SQLCODE Function
- •SQLERRM Function
- •TIMESTAMP_TO_SCN Function
- •%TYPE Attribute
- •UPDATE Statement
- •Where to Find PL/SQL Sample Programs
- •Exercises for the Reader
- •Assigning Character Values
- •Comparing Character Values
- •Inserting Character Values
- •Selecting Character Values
- •Advantages of Wrapping PL/SQL Procedures
- •Running the PL/SQL Wrap Utility
- •Input and Output Files for the PL/SQL Wrap Utility
- •Limitations of the PL/SQL Wrap Utility
- •What Is Name Resolution?
- •Examples of Qualified Names and Dot Notation
- •Differences in Name Resolution Between SQL and PL/SQL
- •Understanding Capture
- •Inner Capture
- •Same-Scope Capture
- •Outer Capture
- •Avoiding Inner Capture in DML Statements
- •Qualifying References to Object Attributes and Methods
- •Calling Parameterless Subprograms and Methods
- •Name Resolution for SQL Versus PL/SQL
- •When Should I Use Bind Variables with PL/SQL?
- •When Do I Use or Omit the Semicolon with Dynamic SQL?
- •How Can I Use Regular Expressions with PL/SQL?
- •How Do I Continue After a PL/SQL Exception?
- •How Do I Pass a Result Set from PL/SQL to Java or Visual Basic (VB)?
- •How Do I Specify Different Kinds of Names with PL/SQL's Dot Notation?
- •What Can I Do with Objects and Object Types in PL/SQL?
- •How Do I Create a PL/SQL Procedure?
- •How Do I Input or Output Data with PL/SQL?
- •How Do I Perform a Case-Insensitive Query?
- •Index
- •Symbols

Avoiding Collection Exceptions
n NumList := NumList(10,20,30,40,50,60,70,80,90,100);
TYPE NickList IS TABLE OF VARCHAR2(64) INDEX BY VARCHAR2(32); nicknames NickList;
BEGIN |
|
|
n.DELETE(2); |
-- deletes element 2 |
|
n.DELETE(3,6); |
-- deletes elements 3 |
through 6 |
n.DELETE(7,7); |
-- deletes element 7 |
|
n.DELETE(6,3); |
-- does nothing since |
6 > 3 |
n.DELETE; |
-- deletes all elements |
nicknames('Bob') := 'Robert'; nicknames('Buffy') := 'Esmerelda'; nicknames('Chip') := 'Charles'; nicknames('Dan') := 'Daniel'; nicknames('Fluffy') := 'Ernestina'; nicknames('Rob') := 'Robert';
nicknames.DELETE('Chip'); -- deletes element denoted by this key nicknames.DELETE('Buffy','Fluffy'); -- deletes elements with keys in this
alphabetic range END;
/
Varrays always have consecutive subscripts, so you cannot delete individual elements except from the end (by using the TRIM method).
If an element to be deleted does not exist, DELETE simply skips it; no exception is raised. PL/SQL keeps placeholders for deleted elements, so you can replace a deleted element by assigning it a new value.
DELETE lets you maintain sparse nested tables. You can store sparse nested tables in the database, just like any other nested tables.
The amount of memory allocated to a nested table can increase or decrease dynamically. As you delete elements, memory is freed page by page. If you delete the entire table, all the memory is freed.
Applying Methods to Collection Parameters
Within a subprogram, a collection parameter assumes the properties of the argument bound to it. You can apply the built-in collection methods (FIRST, LAST, COUNT, and so on) to such parameters. You can create general-purpose subprograms that take collection parameters and iterate through their elements, add or delete elements, and so on.
Note: For varray parameters, the value of LIMIT is always derived from the parameter type definition, regardless of the parameter mode.
Avoiding Collection Exceptions
In most cases, if you reference a nonexistent collection element, PL/SQL raises a predefined exception. Consider the following example:
DECLARE |
|
|
TYPE NumList IS TABLE OF NUMBER; |
|
|
nums NumList; |
-- atomically null |
|
BEGIN |
|
|
/* Assume execution continues despite the raised exceptions. */ |
|
|
nums(1) := 1; |
-- raises COLLECTION_IS_NULL |
(1) |
5-30 PL/SQL User's Guide and Reference
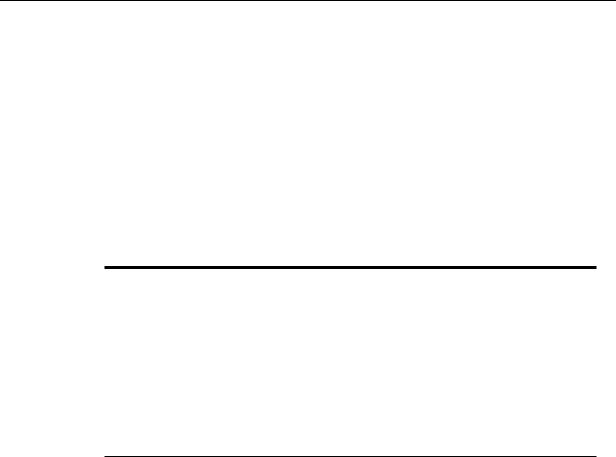
Avoiding Collection Exceptions
nums := NumList(1,2); nums(NULL) := 3; nums(0) := 3; nums(3) := 3; nums.DELETE(1);
IF nums(1) = 1 THEN NULL; END IF; END;
/
-- |
initialize nested table |
|
-- |
raises VALUE_ERROR |
(2) |
--raises SUBSCRIPT_OUTSIDE_LIMIT (3)
--raises SUBSCRIPT_BEYOND_COUNT (4)
--delete element 1
-- raises NO_DATA_FOUND |
(5) |
In the first case, the nested table is atomically null. In the second case, the subscript is null. In the third case, the subscript is outside the allowed range. In the fourth case, the subscript exceeds the number of elements in the table. In the fifth case, the subscript designates a deleted element.
The following list shows when a given exception is raised:
Collection Exception |
Raised when... |
|
|
COLLECTION_IS_NULL |
you try to operate on an atomically null collection. |
NO_DATA_FOUND |
a subscript designates an element that was deleted, or a |
|
nonexistent element of an associative array. |
SUBSCRIPT_BEYOND_COUNT |
a subscript exceeds the number of elements in a |
|
collection. |
SUBSCRIPT_OUTSIDE_LIMIT a subscript is outside the allowed range.
VALUE_ERROR a subscript is null or not convertible to the key type. This exception might occur if the key is defined as a PLS_ INTEGER range, and the subscript is outside this range.
In some cases, you can pass invalid subscripts to a method without raising an exception. For instance, when you pass a null subscript to procedure DELETE, it does nothing. You can replace deleted elements by assigning values to them, without raising NO_DATA_FOUND:
DECLARE |
|
|
TYPE NumList IS TABLE OF NUMBER; |
|
|
nums NumList := NumList(10,20,30); |
-- initialize table |
|
BEGIN |
|
|
nums.DELETE(-1); |
-- does not raise SUBSCRIPT_OUTSIDE_LIMIT |
|
nums.DELETE(3); |
-- delete 3rd element |
|
dbms_output.put_line(nums.COUNT); |
-- prints 2 |
|
nums(3) := 30; |
-- allowed; does not raise NO_DATA_FOUND |
|
dbms_output.put_line(nums.COUNT); |
-- prints 3 |
|
END; |
|
|
/ |
|
|
Packaged collection types and local collection types are never compatible. For example, suppose you want to call the following packaged procedure:
CREATE PACKAGE pkg |
AS |
|
|
TYPE NumList IS |
TABLE OF NUMBER; |
|
|
PROCEDURE print_numlist (nums NumList); |
|||
END pkg; |
|
|
|
/ |
|
|
|
DECLARE |
|
|
|
TYPE NumList IS |
TABLE OF NUMBER; |
|
|
n1 |
pkg.NumList |
:= pkg.NumList(2,4); -- Type from the package. |
|
n2 |
NumList := NumList(6,8); |
-- Local type. |
Using PL/SQL Collections and Records 5-31

What Is a PL/SQL Record?
BEGIN pkg.print_numlist(n1);
--The packaged procedure can't accept a value of the local type. pkg.print_numlist(n2); -- Causes a compilation error.
END;
/
DROP PACKAGE pkg;
The second procedure call fails, because the packaged and local VARRAY types are incompatible despite their identical definitions.
What Is a PL/SQL Record?
A record is a group of related data items stored in fields, each with its own name and datatype. You can think of a record as a variable that can hold a table row, or some columns from a table row. The fields correspond to table columns.
The %ROWTYPE attribute lets you declare a record that represents a row in a database table, without listing all the columns. Your code keeps working even after columns are added to the table. If you want to represent a subset of columns in a table, or columns from different tables, you can define a view or declare a cursor to select the right columns and do any necessary joins, and then apply %ROWTYPE to the view or cursor.
Defining and Declaring Records
To create records, you define a RECORD type, then declare records of that type. You can also create or find a table, view, or PL/SQL cursor with the values you want, and use the %ROWTYPE attribute to create a matching record.
You can define RECORD types in the declarative part of any PL/SQL block, subprogram, or package. When you define your own RECORD type, you can specify a NOT NULL constraint on fields, or give them default values.
DECLARE
-- Declare a record type with 3 fields.
TYPE rec1_t IS RECORD (field1 VARCHAR2(16), field2 NUMBER, field3 DATE); -- For any fields declared NOT NULL, we must supply a default value.
TYPE rec2_t IS RECORD (id INTEGER NOT NULL := -1, name VARCHAR2(64) NOT NULL := '[anonymous]');
--Declare record variables of the types declared above. rec1 rec1_t;
rec2 rec2_t;
--Declare a record variable that can hold a row from the EMPLOYEES table.
--The fields of the record automatically match the names and types of the columns.
--Don't need a TYPE declaration in this case.
rec3 employees%ROWTYPE;
-- Or we can mix fields that are table columns with user-defined fields. TYPE rec4_t IS RECORD (first_name employees.first_name%TYPE, last_name
employees.last_name%TYPE, rating NUMBER); rec4 rec4_t;
BEGIN
-- Read and write fields using dot notation rec1.field1 := 'Yesterday';
5-32 PL/SQL User's Guide and Reference

Defining and Declaring Records
rec1.field2 := 65;
rec1.field3 := TRUNC(SYSDATE-1);
-- We didn't fill in the NAME field, so it takes the default value declared above. dbms_output.put_line(rec2.name);
END;
/
To store a record in the database, you can specify it in an INSERT or UPDATE statement, if its fields match the columns in the table:
...
You can use %TYPE to specify a field type corresponding to a table column type. Your code keeps working even if the column type is changed (for example, to increase the length of a VARCHAR2 or the precision of a NUMBER). The following example defines RECORD types to hold information about a department:
DECLARE
--Best: use %ROWTYPE instead of specifying each column.
--Using <cursor>%ROWTYPE instead of <table>%ROWTYPE since we only want some columns.
--Declaring the cursor doesn't run the query, so no performance hit. CURSOR c1 IS SELECT department_id, department_name, location_id FROM
departments;
rec1 c1%ROWTYPE;
-- Use <column>%TYPE in field declarations to avoid problems if the column types change.
TYPE DeptRec2 IS RECORD (dept_id departments.department_id%TYPE, dept_name departments.department_name%TYPE, dept_loc departments.location_id%TYPE);
rec2 DeptRec2;
--Final technique, writing out each field name and specifying the type directly,
is
--clumsy and unmaintainable for working with table data. Use only for all-PL/SQL code.
TYPE DeptRec3 IS RECORD (dept_id NUMBER, dept_name VARCHAR2(14), dept_loc VARCHAR2(13));
rec3 DeptRec3; BEGIN
NULL;
END;
/
PL/SQL lets you define records that contain objects, collections, and other records (called nested records). However, records cannot be attributes of object types.
Using Records as Procedure Parameters and Function Return Values
Records are easy to process using stored procedures because you can pass just one parameter, instead of a separate parameter for each field. For example, you might fetch a table row from the EMPLOYEES table into a record, then pass that row as a parameter to a function that computed that employee's vacation allowance or some other abstract value. The function could access all the information about that employee by referring to the fields in the record.
The next example shows how to return a record from a function. To make the record type visible across multiple stored functions and stored procedures, declare the record type in a package specification.
Using PL/SQL Collections and Records 5-33