
Beginning Visual Basic 2005 Express Edition - From Novice To Professional (2006)
.pdf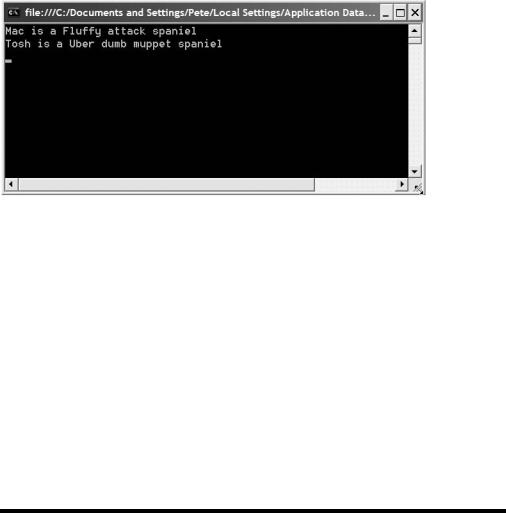
50 |
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
Figure 2-6. When the program runs, the two dog objects output information about themselves into the console window.
There are a couple of interesting things to note about the code before we move on. First, I added an extra line to the end of the program, the same line that you saw in earlier console applications, to make the program wait until the user presses the Enter key. Without this, the console window would vanish from view as soon as the program finished running and you wouldn’t be able to see the application do anything. What I’m doing here is calling a method called ReadLine() on the Console class.
Now, pay attention here. I’m calling a method on a class. I didn’t create a Console object in the same way that we’d already created Dog objects. The reason for this is that Microsoft defined the ReadLine() method on the Console class as a shared method. This makes it a method that you can call without actually having to create an object. You’ll see a lot more uses of Shared in Chapter 4.
Calling the ShowInfo() methods on our two Dog objects takes exactly the same form as calling ReadLine() on the Console class. You simply specify the name of the object followed by a period, followed by the name of the method to run.
Inheritance and Polymorphism
The real power of object-oriented programming starts to make itself known when you learn about subclassing and overloading. We have a whole chapter on that and some of the other more in-depth features of object-oriented programming in Chapter 4, but let’s whet your appetites.

C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
51 |
In the previous example you created a Dog class and then two dog objects. If you were going to write a serious application to keep track of a number of dogs, you’d structure it a little differently. For example, some dogs can jump really well, others make good guard dogs, and others still (my two in particular) excel at drooling.
Try It Out: Subclassing
Fire up another console application (or just delete all the code out of the one you already have). Get your Main() subroutine looking like this one:
Module Module1
Sub Main()
Dim butch As Dog = New Rottweiler("Butch")
Dim mac As Dog = New spaniel("Mac", "yips")
butch.Bark()
mac.Bark()
butch.DoYourThing()
mac.DoYourThing()
Console.ReadLine()
End Sub
End Module
Obviously, this program won’t do much yet—it’s not complete. Pop quiz: how many classes do you need to write to get this program running? It’s a trick question. You could do it with two, but as the program grows more complex (perhaps actually drawing the dogs, getting them to do other dogly things like sleeping and so on), the code would grow significantly. The idea behind programming is to keep things simple, and so the correct answer is three classes. I’ll explain why in a second.
First, take a look at the two lines of code where you’re actually creating the dog objects. They look a little different from the last time you created an object. In fact, they look more like method calls:
Dim butch As Dog = New Rottweiler("Butch")
Dim mac As Dog = New spaniel("Mac", "yips")
52 |
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
In fact, they are method calls. There’s a special kind of method called a constructor that you can put into a class. When you create an object from the class, you can pass values to the constructor. In both cases here, you are passing in the name of the dog. In Mac’s case you’re also passing in the sound he makes. That’s called polymorphism, or overloading—more on that in a second.
Back to the three classes concept. The reason the correct number of classes to have in this application is three is really quite simple, if you stop thinking about computers for a second. Both Butch and Mac are specialized versions of a dog. So, the three classes you’ll need are Dog (which does all the basic stuff that dogs do), Rottweiler (does all the things normal dogs can do, but with very large teeth), and Spaniel (does all the things normal dogs do, when it feels like it). You can see this in the code already. You’re creating two Dogs here, but each is a specific breed of dog. They are breeds that “inherit” everything a dog can do, and then some.
Let’s go ahead and get the Dog class built first—I’ll explain exactly what all the weird new stuff is after you’ve finished typing it in:
MustInherit Class Dog
Protected _name As String
Protected _sound As String
Public Sub New()
'
End Sub
Public Sub New(ByVal name As String) _name = name
_sound = "barks" End Sub
Public Sub New(ByVal name As String, ByVal sound As String) _name = name
_sound = sound End Sub
Public Sub Bark()
Console.WriteLine("{0} {1}", _name, _sound)
End Sub
Public MustOverride Sub DoYourThing()
End Class
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
53 |
The first thing you’ll notice is the word MustInherit in front of the usual class declaration. Why? Well, you don’t typically go to a pet shop and ask for a dog. You go to the pet shop and ask for a specific breed. MustInherit, then, makes sure that no one ever creates just a plain old dog. You can’t create objects from this class; you must inherit the class into another. Don’t panic, all will become clear in a second.
The next thing you’ll notice is that the variables that were declared public in the preceding example (to make them properties) now have the word Protected in front of them:
Protected _name As String
Protected _sound As String
When you set up a variable inside a class as public, it can be treated as a property when you create an object. Adding Private or Protected instead hides them. So, you can’t now say mydog.Name = something. The difference between private and protected will become clear in a very short while.
Directly after the two variables come the constructors I mentioned earlier:
Public Sub New()
'
End Sub
Public Sub New(ByVal name As String) _name = name
_sound = "barks" End Sub
Public Sub New(ByVal name As String, ByVal sound As String) _name = name
_sound = sound End Sub
When you create a Dog object, one of these methods will run. Which method runs depends on whether you pass in one or two strings. In the case of our Rottweiler in the Main() subroutine, you just pass in one string, so you’re saying “When the class becomes an object, set up its name.” When the Spaniel gets created you pass in two strings, effectively saying, “When the Spaniel comes to life, set up its name and the sound it makes.” The third constructor—the New() subroutine with no code in it—is simply required. We’ll never use it. In fact, in a real application there would be code in here to make the program throw an error to effectively tell whoever called it, “You need to tell me a name, or a name and a sound to make.”
The Bark() method should be fairly obvious to you now. It writes out the dog’s name, followed by the sound it makes.
54 |
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
The DoYourThing() method, though, is very weird. Take a look:
Public MustOverride Sub DoYourThing()
This method has absolutely no code in it and uses the Visual Basic keyword MustOverride. What this is telling Visual Basic is that this method must be replaced with something else before it’s called. What do I mean by that? Well, to see that, you’re going to have to key in the other two classes. Pay special attention to the code editor as soon as you type in each Inherits line. You’ll see Visual Basic instantly kick in and write some code for you:
Class Rottweiler
Inherits Dog
Public Sub New(ByVal name As String)
MyBase.New(name)
End Sub
Public Overrides Sub DoYourThing()
Console.WriteLine( _
"{0} snarls at you in a menacing fashion.", _
_name)
End Sub
End Class
Class spaniel
Inherits Dog
Public Sub New(ByVal name As String, ByVal sound As String)
MyBase.New(name, sound)
End Sub
Public Overrides Sub DoYourThing()
Console.WriteLine( _
"{0} licks you all over, then drools on you.", _
_name)
End Sub
End Class
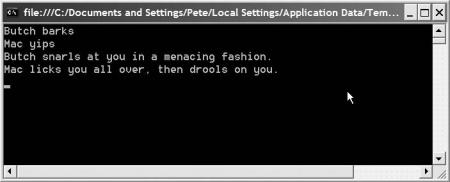
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
55 |
You could run the program now and you’d see a console window just like the one in Figure 2-7.
Figure 2-7. When the program runs, even though you have two “dog” objects, they behave differently, thanks to inheritance.
Both these classes use inheritance. To put it another technical and confusing way, they subclass the Dog class. Take a look at how the Rottweiler class starts out:
Class Rottweiler
Inherits Dog
What this code is saying is that Rottweiler, although it’s a whole new class, is based on Dog. Everything a Dog can do, the Rottweiler can automatically do too. Hopefully you noticed that as soon as you entered Inherits Dog on the line of its own, the VB Express editor provided you with an empty DoYourThing() method. Why? Because it spotted that in Dog we had marked DoYourThing() as MustOverride, so it knew you were going to have to write your own method for that. Handy, isn’t it. More on this in a second.
Now, you set up a couple of constructors in the Dog class to set the name and/or the sound the dog makes. Visual Basic requires that you also write matching constructors in any subclass. The reason for this is that it lets you perform any specific operations in the subclass when it gets created, which may be different from the base class (the one you inherited—Dog). In this case you don’t want to do anything different, so the Rottweiler’s constructor looks quite strange:
Public Sub New(ByVal name As String)
MyBase.New(name)
End Sub
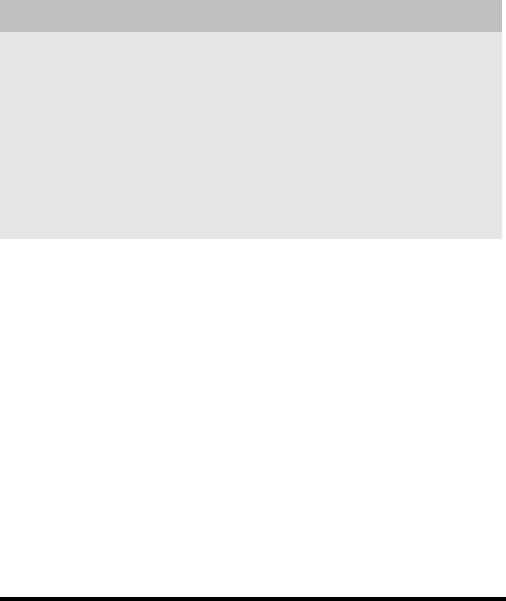
56 |
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
NEW TO .NET?
Classes, objects, and object-oriented programming (OOP) play an important role in the .NET Framework. Everything you work with in the .NET Framework class library itself is an object (well, it’s actually a type, and types include classes, objects, and a bunch of other stuff you’ll get into soon enough). This provides you with a great deal of power after you start to understand just what OOP lets you do.
For example, you could subclass a standard user interface control, and override some of the methods it contains to quickly and easily develop your own custom control. You could develop a form that acts as a base class for a whole bunch of other forms, in a wide range of applications, but which itself subclasses the standard form class in the framework.
Aside from OOP being a cool and productive way to develop code, you really can’t make full use of .NET itself without a good understanding of OOP concepts. It’s for that reason you’ll be spending a lot of time looking at OOP from Chapter 4 onward.
This just says that when a Rottweiler object is created, do what the base class (Dog) did, and nothing else.
Now, remember that MustOverride empty DoYourThing() method in the Dog class? In the Rottweiler class (and in the Spaniel class), you can actually put in some code to show this specific breed of dog (subclass of dog) doing what it does best:
Public Overrides Sub DoYourThing()
Console.WriteLine( _
"{0} snarls at you in a menacing fashion.", _
_name)
End Sub
It’s just the same as writing any other method in a class, but the use of the word Overrides tells Visual Basic that when this object is asked to DoYourThing(), the object will completely ignore what a generic dog would do, and do this instead.
That, in a nutshell, is inheritance, polymorphism, and object-oriented programming for you. I know that a lot of you reading this may be quite confused. Don’t panic. It’s such an important part of Visual Basic that I had to cover it in this overview, but you’ll spend a lot of time going much deeper and slower through the various concepts in later chapters in the book.
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
57 |
Control Structures
The last thing I need to cover in this whirlwind look at Visual Basic is control structures. So far you’ve seen how to group your code into subroutines, and how to group those subroutines into classes. I’ve also touched on variables and storing data (by the end of the next chapter you’ll be eating sleeping, and breathing variables). What you haven’t seen yet is how to actually do anything. How do you breathe life into your programs to make them seem to “think” for themselves? That’s where control structures come in.
Visual Basic’s control structures provide you with a way to make your programs repeatedly do things (loop), do things for a set number of times (another loop), or decide to do something if some condition is met (conditional statements).
Conditional statements give your program life. They enable the program to make decisions. For example, if the dog is a Rottweiler, run away; otherwise, pet the dog. The format for a conditional statement looks like this:
If somecondition Then
Else
End If
If and Else are Visual Basic keywords that enable you to build up your conditional statements.
Do you remember back in school when the computer science teacher would drone on about binary and all that supremely tedious stuff? Well, it’s come back to haunt you, but it’s a lot simpler to understand than he made out. That somecondition thing is a statement (it could call a subroutine, it could compare two or more values) that must only result in True or False (it stems from binary, which has only two digits, 1 or 0). For example, you could write a condition that compares an object’s name to, say, Apress. If the object’s name is Apress, you could say that the result is True. On the other hand, if the object’s name were Joe Schmo Publishing, the condition would be False. Easy, isn’t it.
Let’s take a look at a simple example—a Windows login form.
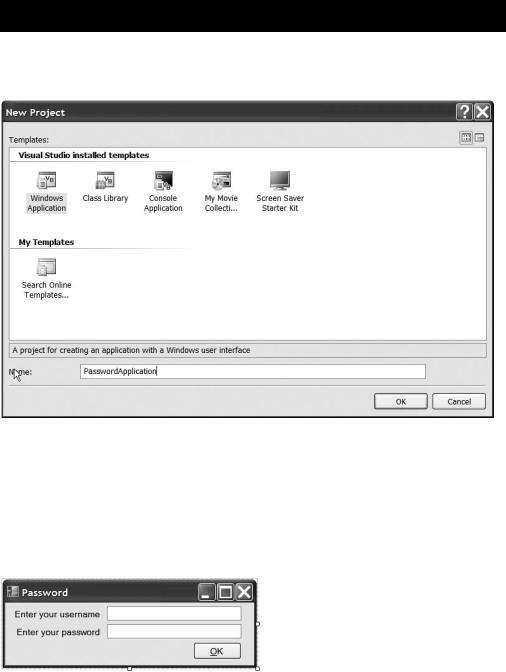
58 |
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
Try It Out: Writing Conditional Statements
Start up a new project in Visual Basic 2005 Express, but unlike last time, choose to create a Windows Application project, as shown in Figure 2-8.
Figure 2-8. Choose a Windows Application project from the New Project dialog box as shown.
When the form editor appears, click on the form and set its text property to Password.
Now add some controls to the form so that it looks roughly like the one in Figure 2-9. Remember, you can use the positioning guides when you drop controls onto the form to align the controls consistently with one another and to conform to Windows standards.
Figure 2-9. Drop some controls onto the form, and perhaps even resize it, so that yours looks
like this one.
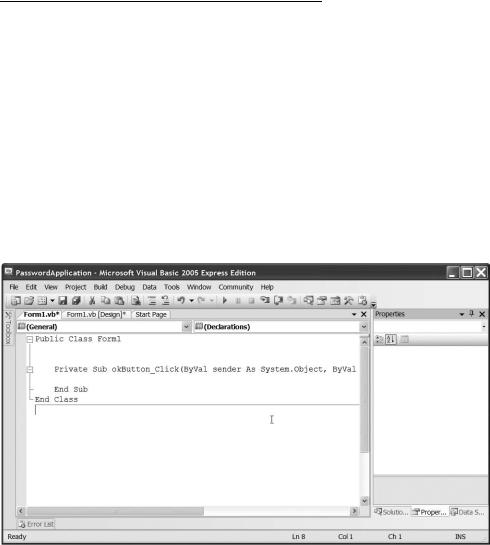
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
59 |
To make sure that the code you’re going to type in shortly is the same on your machine as on mine, set up the controls’ properties shown in Table 2-1 by using the Properties window (press F4 if you can’t see it for some reason).
Table 2-1. Properties of the Controls on Your Password Form
Control |
Property |
Value |
First label control |
Text |
Enter your username |
Second label control |
Text |
Enter your password |
First text box |
Name |
usernameBox |
Second text box |
Name |
passwordBox |
|
PasswordChar |
* |
Button |
Text |
&OK |
|
Name |
okButton |
|
|
|
What you’re going to do here is check the username and password when the user clicks the OK button, and then display a message to the user indicating whether they managed to log in. So, go ahead and double-click the OK button in the form designer to pop open the code editor, shown in Figure 2-10.
Figure 2-10. Double-clicking a control drops you into the code editor to work on the standard/
most common event for that control.