
Beginning Visual Basic 2005 Express Edition - From Novice To Professional (2006)
.pdf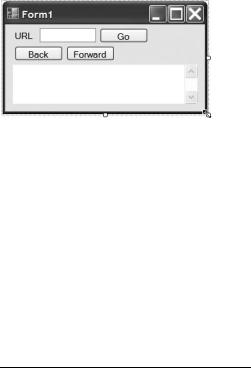
30 |
C H A P T E R 1 ■ W E L C O M E T O V I S U A L B A S I C E X P R E S S |
Figure 1-34. Anchoring controls lets you move and/or resize them as the form changes in size.
So far you’ve managed to set up a pretty neat-looking user interface for the web browser that will actually respond to users changing the size of the form. The next step is to add some functionality to this, to make the program useable.
However, before diving into writing some code, you first need to rename some of those controls. The reason for this is that you will be writing code that tells the controls what to do by name. Having a button called Button1 is okay for just messing around and trying things out, but your code will be a whole lot clearer and easier to follow if you give everything a decent name. So with that in mind, set the Name properties as shown in Table 1-2.
Table 1-2. Name Properties
Control |
Name Property |
URL text box (not the label) |
urlBox |
Go button |
goButton |
Back button |
backButton |
Forward button |
forwardButton |
The WebBrowser control |
browser |
|
|
Perhaps the most important bit of code you need to write is the code that responds to the user hitting that Go button. When the user clicks it, you want the web browser to navigate straight to the URL that the user entered into the text box.
Double-click the Go button, and the code window appears.
The way to make a WebBrowser control navigate to a new page is a method called Navigate. All you need to do is tell that Navigate method where to go.
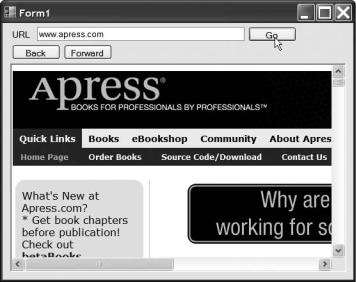
C H A P T E R 1 ■ W E L C O M E T O V I S U A L B A S I C E X P R E S S |
31 |
Type in code so that your goButton_Click code looks like this:
Public Class Form1
Private Sub goButton_Click(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles goButton.Click browser.Navigate(urlBox.Text)
End Sub
End Class
What’s happening here? Well, when you double-clicked the button on the form, you told VB Express that you wanted to write some code to deal with the button’s default event. An event is something that happens in response to something else. For example, when a user clicks a button, a Click event happens, and you can write code to deal with that.
The code that’s most interesting here is the bit that you have to write. You’re simply using the “browser’s” Navigate function to navigate to the text entered into the text box. Text is a property of the TextBox control that you can look at in the Properties window. You can refer to it in code as shown.
Go ahead and run the application now. Type in a URL, click the Go button, and watch what happens—see Figure 1-35.
Figure 1-35. The Go button’s Click event brings the web browser to life, telling it exactly where
to navigate.

32 |
C H A P T E R 1 ■ W E L C O M E T O V I S U A L B A S I C E X P R E S S |
To stop the application, just close the form as you would any other window.
All that remains now is to get those Back and Forward buttons working.
Double-click the Back button to edit its Click event (you’ll be looking at the code window, so to get back to the form in order to double-click the Back button, click the tab labeled Form1.vb [Design] at the top of the code editor).
To get the browser to go back a page (assuming there is a page to go back to), you just need to tell it GoBack(). Type in code so that the Back button’s Click event looks like this:
Private Sub backButton_Click(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles backButton.Click
browser.GoBack() End Sub
Do the same thing for the Forward button, but set its code to look like this:
Private Sub forwardButton_Click(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles forwardButton.Click
browser.GoForward() End Sub
Voilà – you’re done. Run the program, navigate to a web page, click some links, and then start using those Back and Forward buttons.
Those of you who have never written a program before in your life are probably quite worried by the typing that went on. Aside from that, what this example demonstrates is the very essence of Visual Basic 2005 Express. Express makes application development easy. In fact, the .NET Framework 2.0 makes application development easy, and VB Express just adds even more to the mix to make the whole deal so much more satisfying.
Summary
You covered a lot of ground in this introduction to Visual Basic 2005 Express. You saw how to change the look and feel of the IDE, how to create projects, and how to write code. You also took a look at IntelliSense, and hopefully I managed to give you a sense of just what a great time-saver that feature is in VB Express. Finally, we wrapped everything up by developing a simple but fully functioning web browser application.
After this whirlwind tour of the IDE, you’ll be pleased to know that in the next chapter I slow things down a bit so you can take a long hard look at just what a Visual Basic program is and what it all means. Those of you who have never written code will soon
C H A P T E R 1 ■ W E L C O M E T O V I S U A L B A S I C E X P R E S S |
33 |
understand all the things that may have worried you in the earlier examples. Those of you coming here from other languages are about to wonder just how on earth you ever got along before Visual Basic 2005 Express came along.
See you in Chapter 2.
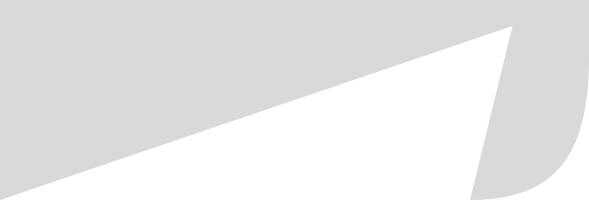
C H A P T E R 2
■ ■ ■
The Basics of Visual Basic
This chapter in the C# version of this book begins with a description of how C# can be quite a difficult, bewildering language to read. It’s an intentionally terse language, with its commands succinct to the point of obscurity in some cases.
Thankfully, Visual Basic 2005 doesn’t suffer from that problem. Visual Basic is an extremely descriptive language. This can lead to other problems, though. Because the language is so descriptive, it’s easy for new programmers to find the language running away with them as they pound on the keyboard hour after hour. Without care, a Visual Basic program can soon become an immense list of seemingly unrelated instructions. To be fair, this is more a carryover of its non-object-oriented legacy. With a little effort and focus, Visual Basic programs these days can appear almost artistic.
In this chapter you’ll take a brief whirlwind tour of the Visual Basic language, its features, and how to use it. If you’ve never programmed before, you will no doubt find some of the concepts in this chapter confusing. Stick with it, though. This chapter gives you a foundation to build on as you dive deeper into Visual Basic through the rest of the book.
Don’t be put off reading this chapter if you’ve done some programming in some other language, even classic Visual Basic; I’ll be dropping pointers throughout to put the new Visual Basic 2005 programming language into a context that you old hands should appreciate.
The Basic Structure
The focus of Visual Basic 2005 is to keep things as simple as possible. It’s a productive programming language, and the designers were keen to make sure that your attention is always where it should be—on writing new features for your application. For this reason, the Solution Explorer is quite uncluttered, as shown in Figure 2-1.
35
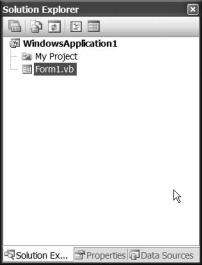
36 |
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
Figure 2-1. The Solution Explorer in Visual Basic 2005 Express is always clean and uncluttered.
When you create a new project in Visual Basic 2005 Express, you usually see just two items in the Solution Explorer. The first, My Project, provides quick access to the project options. Double-clicking it replaces the form designer, or code view, with a neat view into the project’s main properties, as shown in Figure 2-2.
The tabs down the side of the options display let you drill down into specialist settings for tasks such as debugging and compiling. You don’t need to know about these just yet. The vast majority of the time, you’ll never even change any of these option settings. The common things that you would want to change, such as the project’s name, can be done by other means.
The other item that you’ll see in the Solution Explorer is your main form file, usually named Form1.vb. Double-clicking this brings up the form in the form designer mode. This is the mode that we used in the walk-throughs in Chapter 1. As you add new forms and classes (more on those in Chapter 4) to the project, you’ll see the Solution Explorer start to fill up with more items whose names end in .vb.
Forms, as you saw in the previous chapter, consist of two elements. On the one hand, there are the visual elements of the forms, controls that build up your application’s user interface. On the other hand, there is the actual Visual Basic code that you’ll need to write to respond to events on the user interface. This is known as your program’s source, and it’s this that we’ll be focusing on in the rest of this chapter.
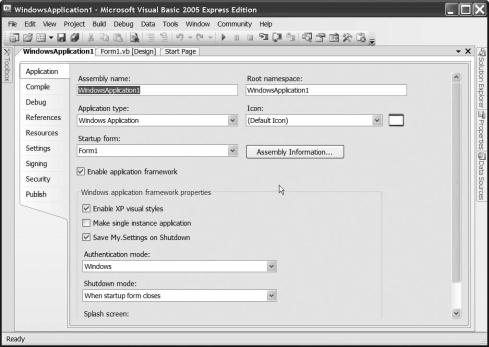
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
37 |
Figure 2-2. Double-clicking My Project in the Solution Explorer takes you into the project properties view.
Source Code and the Two Project Types
Every project you create in Visual Basic 2005 Express will start with a single source file. In a console application project, this is a module. In a Windows application project, it’s a form. (There is another type of project you can create called a class library, but its source file, on the surface at least, looks the same as the form you get with a Windows application.) Let’s look at the differences between them.
The module that you get with a console-type application looks like this:
Module Module1
Sub Main()
End Sub
End Module
38 |
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
The form you get with a Windows application looks like this:
Public Class Form1
End Class
There are two obvious differences between the two files.
First, a console application starts off with a module. You can see this because the first line of code in the source file actually has the word Module, followed by the module’s name. A Windows application, on the other hand, starts out with a class, called Form1. The most basic (excuse the pun) type of source file you can create in VB Express is a module. It’s just a container of code. Classes, on the other hand, are the root of everything that is object oriented and hence they get a chapter all of their very own in Chapter 4.
The second difference is that in a console application, the module starts out with a subroutine that is always called Main(). When you run the program, the .NET runtime system looks for the subroutine called Main() and runs it. Actually the same thing happens when you start up a Windows application, but VB Express hides the implementation of the Main() method away from you. All it does in a Windows application is set up the .NET runtime and then fire up the first window of the application before waiting for the user to do something.
That’s the reason a console application has a subroutine waiting for you to add code, and a Windows application doesn’t. A console application can’t do anything unless you give it some code inside that Main() subroutine. A Windows application on the other hand can do a bunch of stuff, including starting up a form as a window. The window comes preequipped with a bunch of functionality of its own, such as the ability to show and hide, change its size, shut down when its Close icon is clicked, and so on. If you want or need the program to do anything else, it’s up to you to add controls to the form and then write code to respond to events on those controls. If I added a button to the form, for example, and double-clicked it to get at its Click event, the code in my form would change, like this:
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
End Sub
End Class
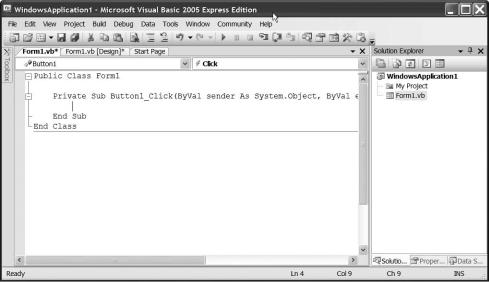
C H A P T E R 2 ■ T H E B A S I C S O F V I S U A L B A S I C |
39 |
Now it looks a little similar to the module, doesn’t it. At the very least you may have noticed that this event handler is a Sub just like Main (albeit with a bunch of things inside parentheses following the name of the Sub).
These are subroutines. Inside a class, as the preceding event handler is, they are more commonly referred to as methods. Basically a Sub is a small unit of code with a name. In our console application, we add code to the subroutine called Main(), and that code (anything between the Sub Main() line and the End Sub line) is what gets run when the program starts. Similarly, in our preceding event handler, any code that lives between the Sub Button1_Click line and the End Sub line is run whenever the user clicks on a button.
You may also have noticed by now that there’s a slight difference between some of the code printed on these pages and what you’re seeing on-screen. Take a look at the following code and then compare it with Figure 2-3:
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
End Sub
Figure 2-3. Notice the long line of code that marks the start of the subroutine.