
Beginning Visual Basic 2005 Express Edition - From Novice To Professional (2006)
.pdf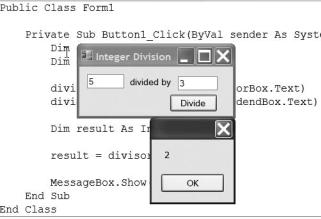
70 |
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
Figure 3-4. Dividing integers can produce some very strange results.
Obviously, that answer is wrong; 5 divided by 3 is 1.666666667, or less depending on the precision of your calculator.
When you divide two integers, or store the result of a division as an integer, VB rounds the answer. So, if the answer were really 1.1, VB would round the answer down to 1. On the other hand, if the answer were 1.666666667 as it is here, VB would round the result up to 2. That’s definitely something to be aware of.
Let’s extend the program a bit more and calculate the modulus to see a different answer:
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim divisor As Integer
Dim dividend As Integer
divisor = Integer.Parse(divisorBox.Text) dividend = Integer.Parse(dividendBox.Text)
Dim result As Integer
Dim modulus As Integer
result = divisor / dividend modulus = divisor Mod dividend
MessageBox.Show("The answer is " + result.ToString() + _ " with " + modulus.ToString() + " left over")
End Sub
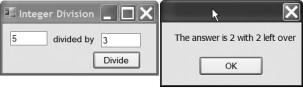
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
71 |
Notice how you can add strings together by using the + sign (technically, it’s called concatenating strings). Because modulus and result are both integers, we need to call their ToString() methods to turn them into strings.
This code also uses the modulus operator Mod to calculate the remainder of the division.
If you run the program now, you’ll see a result like that in Figure 3-5.
Figure 3-5. Modulus can be used to show the remainder result of a division.
Well, that’s not really any better at all. The Mod operator is working just fine. It tells us that 5 divided by 3 doesn’t work out cleanly, and that there would be 2 left over. But that rounding problem is still giving us issues; 5 divided by 3 should be 1 with 2 left over.
The solution to this is incredibly subtle. Take a look:
Private Sub Button1_Click(ByVal sender As System.Object,_
ByVal e As System.EventArgs) Handles Button1.Click
Dim divisor As Integer
Dim dividend As Integer
divisor = Integer.Parse(divisorBox.Text) dividend = Integer.Parse(dividendBox.Text)
Dim result As Integer
Dim modulus As Integer
result = divisor \ dividend modulus = divisor Mod dividend
MessageBox.Show("The answer is " + result.ToString() + _ " with " + modulus.ToString() + " left over")
End Sub
The change here is in the line that divides the two numbers and stores the product in our result variable. I changed the divide symbol from a forward slash (/) to a backslash (\). In VB Express the forward slash divides two numbers and returns a Double—a value that may have digits (lots of them) after the decimal point. It’s
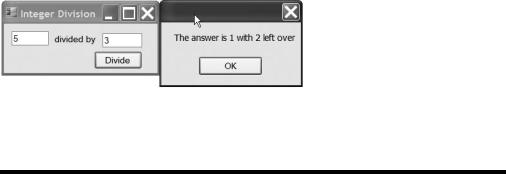
72 |
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
not a whole number. If you try to store a Double value into an Integer, Visual Basic will quite happily, and quietly, round the number to the closest integer. After mentioning earlier that I like to be explicit in my code, it might seem strange that I let Visual Basic get away with this. The alternative is to tell Visual Basic exactly what I expect it to do, like this:
result = CType(divisor \ dividend, Integer)
CType does something called a cast, converting what would normally be a Double answer into an Integer. In simple math like this VB will do it for me without me having to CType it, but there is of course an argument that typing in CType shows anyone reading the code explicitly what is going on behind the scenes. In fact many languages (C# and Java, for example) force you to do an explicit cast of data types. I’ll leave it to you to decide which style you prefer.
The backslash here is an integer divide. It expects to divide two numbers and return a whole number result. If the result is not a whole number, the backslash truncates it. In other words, the integer divide cuts off everything after the decimal place. Try running the program now and you’ll see the result in Figure 3-6.
Figure 3-6. You need to be very careful about which divide symbol you use, to prevent rounding errors.
Other Number Types
If the only kind of number you could work with in VB was an integer, a lot of people would wander off to other development languages. Thankfully, VB has some more-complex numeric data types to play with. The most common are Decimal and Double, the rest being variations on that theme (search the online help for “built-in data types” to see the full list).
The Decimal variables can hold values with a staggering number of decimal places, but a smaller number range than Double. Conversely, Double variables have fewer decimal places than Decimal numbers, but a much greater physical number range. The golden rule when working with them is that if you are doing something that requires an incredibly high degree of precision (such as financial calculations or some scientific work), use Decimal. For anything else use Double.
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
73 |
Both Decimal and Double are declared exactly the same way that you would declare an Integer variable. For example:
Dim myDouble As Double
Dim myDecimal As Decimal
Boolean Values
I want to touch on a simple data type here that I’ll talk about in much greater detail toward the end of this chapter: the Boolean data type.
There was a famous English chap by the name of George Boole. George is known today as the inventor of calculus law and Boolean algebra. The latter, in a nutshell, is responsible for much of the logic that goes on in computers today because it focuses on logic, trues and falses, 1s and 0s, the heartbeat of your PC.
A Boolean data type can hold just one of two values, True or False:
Dim trulyStunningAuthor As Boolean = False
Dim macTheDogDrools As Boolean = True
The True and False values are keywords in VB that you can write out longhand just as in those two examples.
There’s not much more to say—you’ll see a lot of these guys later in this chapter, when you start to look at conditions and loops.
Characters and Text
Aside from numbers, perhaps the most common type of data you’ll work with in your programs will be text. There are two types of text—characters (which are the individual letters and symbols you see on this page), and strings (which are combinations of characters that form pretty much anything from a word to a novel). I’ll explain characters first, since they’re the more trivial.
A character is declared with the Char keyword, and you specify Char values by placing them in double quotes:
Dim aCharacter As Char = "s"
It’s actually quite rare these days that you need to do much with single characters, so I’ll move on.
Strings (text) are declared with the keyword String, and string data is also always held inside double quotes:
Dim aString as String = "Pete was here!"
74 |
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
As you can see, you can store pretty much anything you want in a string, including letters, numbers, punctuation, and a lot more besides.
The String class also has a ton of methods that you can use to manipulate the characters in the string itself. You’ll soon find, when you start writing your own programs, that these will get used a lot.
If you want to find something inside a string, there are five methods that you can use:
Contains(), IndexOf(), LastIndexOf()x, StartsWith(), and EndsWith(). Contains(),
StartsWith(), and EndsWith() just return a True or False value. For example:
Sub Main()
Dim person As String
person = "Joseph R. Bloggs"
If person.EndsWith("Bloggs") Then
Console.WriteLine("Yup, we have a Bloggs here")
End If
If person.StartsWith("Joseph") Then
Console.WriteLine("
This is definitely a Joseph")
End If
If person.Contains("R.") Then
Console.WriteLine("The middle initial is R")
End If
Console.ReadLine()
End Sub
If you wish to go ahead and key that into a console application, feel free. When the program runs, it produces output like that in Figure 3-7.
As you can see, the Contains(),StartsWith(), and EndsWith() methods are really easy to use. You just pass them the string you want to search for, and they return True or False depending on whether they could find a match.
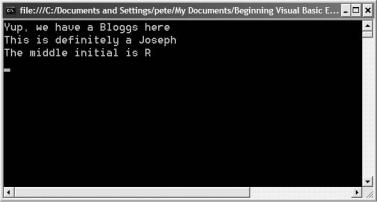
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
75 |
Figure 3-7. The Contains(), StartsWith(), and EndsWith() methods let you easily see what’s inside a string.
IndexOf() and LastIndexOf()work a little differently. Both methods will tell you at which character position another string was found, or –1 if there was no match. If you have a string that might contain more than one instance of another, LastIndexOf() will return the last character position that a match was found. Here’s an example:
Sub Main()
Dim quote As String
quote = "Alas, poor Yorik, I knew him well."
Console.WriteLine(quote.IndexOf(","))
Console.WriteLine(quote.LastIndexOf(","))
Console.WriteLine(quote.IndexOf("Bob"))
Console.ReadLine()
End Sub
If you key this into a console application project, the output will look like Figure 3-8.
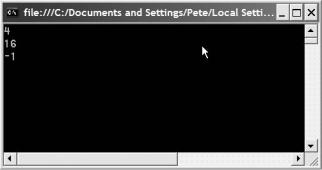
76 |
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
Figure 3-8. The IndexOf() methods let you find where one string exists in another.
As you can see, the first call to IndexOf() returns 4, telling us that there is a comma at position 4 in the string. But wait a second. If you look at the actual string, the first comma is really the fifth character. The reason IndexOf() returns 4 is that it counts the first character as character number 0. Think of IndexOf() then as telling you how many characters to ignore before it finds a match. If you look at it that way, IndexOf() is telling us that the comma appears after the fourth character in the string.
LastIndexOf()works the same way. It tells us that the last comma in the string appears after character number 16.
If you try to find a string that doesn’t exist, like “Bob,” both IndexOf() and
LastIndexOf() return –1.
You’ll most often use these methods in conjunction with another one, called Substring(). Substring() effectively slices up the string into pieces. You can use it to find everything in a string after a certain character, or you can tell it to give you a certain number of characters from the string, starting at a certain position. For example:
Sub Main()
Dim numbers As String
numbers = "One Two Three Four"
Dim position As Integer = numbers.IndexOf("Two")
Console.WriteLine(numbers.Substring(position))
Console.WriteLine(numbers.Substring(position, 3))
Console.ReadLine()
End Sub
If you key that in and run it, you’ll see the output in Figure 3-9.
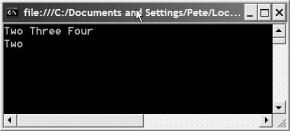
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
77 |
Figure 3-9. IndexOf() and Substring() together let you slice up a string.
This program first uses IndexOf() to find where in the string the word “Two” is. It then passes the value to Substring(). If you pass a single number into Substring(), it gives you everything from the position you specify onward. That’s why the program outputs “Two Three Four.”
You can also pass a second number to Substring(), which is the number of characters to extract. The second call then passes in 3 as that second number, meaning we just pull the word “Two” out of the string.
Incidentally, the more ambitious among you may be interested to know that .NET has an incredibly powerful set of objects that live in System.Text.RegularExpressions. These objects provide methods you can call to do some pretty complex stuff to strings of text. In fact, regular expressions use a language all of their own, so for now I’ll leave it to you to explore the online help if you are already familiar with them and want to get a grip on how they work in .NET.
Arrays
Knowing how to work with single pieces of data is useful, but what if you want to work with a bunch of the same type? For example, you might want to hold a list of people’s names in your program, or perhaps a list of stock symbols in a stock-tracking application. That’s where arrays come in.
Arrays are not a special new data type, but rather a way of telling Visual Basic that you want a variable to hold more than one of a specific type of data. You declare them like this:
Dim stockTickers(9) As String
This example sets up an array to hold 10 strings (numbered 0, 1, 2, and so on up to 9). The parentheses following the variable name show VB that you want to work with
an array.

78 |
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
You could also set up an array by specifying the values that it should hold on the same line as the declaration:
Dim tickers() As String = {"MSFT", "IBM", "APPL"}
In this case, you have an array of three strings. After the strings are in the array, they get numbered. The first is number 0 (remember how IndexOf() also treats the first element as number 0), and the last is the size of the array minus 1. So, if you have an array of four strings, the strings are numbered 0, 1, 2, and 3. This lets you get at them individually. You can also find out just how many items are in the array by using the array’s Length property. This all sounds very weird and cryptic, so let’s look at an example.
Try It Out: Working with Arrays
Start up a new console application. When the code window appears, declare a string array just as in the preceding example. You can see the code here:
Sub Main()
Dim names() As String = {"Pete", "John", "Dave", "Gary"}
End Sub
Add some code now to print out the length of the array and also each string in the array:
Sub Main()
Dim names() As String = {"Pete", "John", "Dave", "Gary"}
Console.WriteLine( _
"The array contains {0} items", names.Length)
Console.WriteLine(names(0))
Console.WriteLine(names(1))
Console.WriteLine(names(2))
Console.WriteLine(names(3))
Console.ReadLine()
End Sub
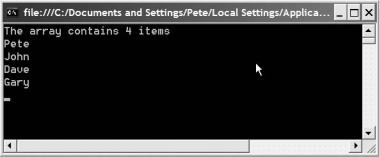
C H A P T E R 3 ■ W O R K I N G W I T H V A R I A B L E S |
79 |
If you run the application now, you’ll see a console window like the one in Figure 3-10, showing the array length and each item in the array.
Figure 3-10. You can access elements of the array by typing the array name, followed by the index of the element you want inside parentheses.
Imagine, though, that you had an array with a thousand items in it. Typing Console.WriteLine() for each one would be tedious in the extreme. Thankfully, VB gives us the handy For Each command. Go ahead and change the code so that it looks like this (I’ve highlighted the changes for you):
Sub Main()
Dim names() As String = {"Pete", "John", "Dave", "Gary"}
Console.WriteLine( _
"The array contains {0} items", names.Length)
For Each name As String In names Console.WriteLine(name)
Next
Console.ReadLine()
End Sub
As you can see, the For Each command is quite easy to use. It’s a loop, a block of code that runs over and over (more on these in a while). In the case of the For Each command, the block runs, walking through each element of the array, storing each element into a variable that you specify. In this case, you set up a new string variable called name that you’ll use to grab each element “in” the names array.