
- •Preface
- •Contents
- •1.1 What Operating Systems Do
- •1.2 Computer-System Organization
- •1.4 Operating-System Structure
- •1.5 Operating-System Operations
- •1.6 Process Management
- •1.7 Memory Management
- •1.8 Storage Management
- •1.9 Protection and Security
- •1.10 Kernel Data Structures
- •1.11 Computing Environments
- •1.12 Open-Source Operating Systems
- •1.13 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •2.3 System Calls
- •2.4 Types of System Calls
- •2.5 System Programs
- •2.6 Operating-System Design and Implementation
- •2.9 Operating-System Generation
- •2.10 System Boot
- •2.11 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •3.1 Process Concept
- •3.2 Process Scheduling
- •3.3 Operations on Processes
- •3.4 Interprocess Communication
- •3.5 Examples of IPC Systems
- •3.7 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •4.1 Overview
- •4.2 Multicore Programming
- •4.3 Multithreading Models
- •4.4 Thread Libraries
- •4.5 Implicit Threading
- •4.6 Threading Issues
- •4.8 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •5.1 Background
- •5.3 Peterson’s Solution
- •5.4 Synchronization Hardware
- •5.5 Mutex Locks
- •5.6 Semaphores
- •5.7 Classic Problems of Synchronization
- •5.8 Monitors
- •5.9 Synchronization Examples
- •5.10 Alternative Approaches
- •5.11 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •6.1 Basic Concepts
- •6.2 Scheduling Criteria
- •6.3 Scheduling Algorithms
- •6.4 Thread Scheduling
- •6.5 Multiple-Processor Scheduling
- •6.6 Real-Time CPU Scheduling
- •6.8 Algorithm Evaluation
- •6.9 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •7.1 System Model
- •7.2 Deadlock Characterization
- •7.3 Methods for Handling Deadlocks
- •7.4 Deadlock Prevention
- •7.5 Deadlock Avoidance
- •7.6 Deadlock Detection
- •7.7 Recovery from Deadlock
- •7.8 Summary
- •Practice Exercises
- •Bibliography
- •8.1 Background
- •8.2 Swapping
- •8.3 Contiguous Memory Allocation
- •8.4 Segmentation
- •8.5 Paging
- •8.6 Structure of the Page Table
- •8.7 Example: Intel 32 and 64-bit Architectures
- •8.8 Example: ARM Architecture
- •8.9 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •9.1 Background
- •9.2 Demand Paging
- •9.3 Copy-on-Write
- •9.4 Page Replacement
- •9.5 Allocation of Frames
- •9.6 Thrashing
- •9.8 Allocating Kernel Memory
- •9.9 Other Considerations
- •9.10 Operating-System Examples
- •9.11 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •10.2 Disk Structure
- •10.3 Disk Attachment
- •10.4 Disk Scheduling
- •10.5 Disk Management
- •10.6 Swap-Space Management
- •10.7 RAID Structure
- •10.8 Stable-Storage Implementation
- •10.9 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •11.1 File Concept
- •11.2 Access Methods
- •11.3 Directory and Disk Structure
- •11.4 File-System Mounting
- •11.5 File Sharing
- •11.6 Protection
- •11.7 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •12.2 File-System Implementation
- •12.3 Directory Implementation
- •12.4 Allocation Methods
- •12.5 Free-Space Management
- •12.7 Recovery
- •12.9 Example: The WAFL File System
- •12.10 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •13.1 Overview
- •13.2 I/O Hardware
- •13.3 Application I/O Interface
- •13.4 Kernel I/O Subsystem
- •13.5 Transforming I/O Requests to Hardware Operations
- •13.6 STREAMS
- •13.7 Performance
- •13.8 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •14.1 Goals of Protection
- •14.2 Principles of Protection
- •14.3 Domain of Protection
- •14.4 Access Matrix
- •14.5 Implementation of the Access Matrix
- •14.6 Access Control
- •14.7 Revocation of Access Rights
- •14.8 Capability-Based Systems
- •14.9 Language-Based Protection
- •14.10 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •15.1 The Security Problem
- •15.2 Program Threats
- •15.3 System and Network Threats
- •15.4 Cryptography as a Security Tool
- •15.5 User Authentication
- •15.6 Implementing Security Defenses
- •15.7 Firewalling to Protect Systems and Networks
- •15.9 An Example: Windows 7
- •15.10 Summary
- •Exercises
- •Bibliographical Notes
- •Bibliography
- •16.1 Overview
- •16.2 History
- •16.4 Building Blocks
- •16.5 Types of Virtual Machines and Their Implementations
- •16.6 Virtualization and Operating-System Components
- •16.7 Examples
- •16.8 Summary
- •Exercises
- •Bibliographical Notes
- •Bibliography
- •17.1 Advantages of Distributed Systems
- •17.2 Types of Network-based Operating Systems
- •17.3 Network Structure
- •17.4 Communication Structure
- •17.5 Communication Protocols
- •17.6 An Example: TCP/IP
- •17.7 Robustness
- •17.8 Design Issues
- •17.9 Distributed File Systems
- •17.10 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •18.1 Linux History
- •18.2 Design Principles
- •18.3 Kernel Modules
- •18.4 Process Management
- •18.5 Scheduling
- •18.6 Memory Management
- •18.7 File Systems
- •18.8 Input and Output
- •18.9 Interprocess Communication
- •18.10 Network Structure
- •18.11 Security
- •18.12 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •19.1 History
- •19.2 Design Principles
- •19.3 System Components
- •19.4 Terminal Services and Fast User Switching
- •19.5 File System
- •19.6 Networking
- •19.7 Programmer Interface
- •19.8 Summary
- •Practice Exercises
- •Bibliographical Notes
- •Bibliography
- •20.1 Feature Migration
- •20.2 Early Systems
- •20.3 Atlas
- •20.7 CTSS
- •20.8 MULTICS
- •20.10 TOPS-20
- •20.12 Macintosh Operating System and Windows
- •20.13 Mach
- •20.14 Other Systems
- •Exercises
- •Bibliographical Notes
- •Bibliography
- •Credits
- •Index
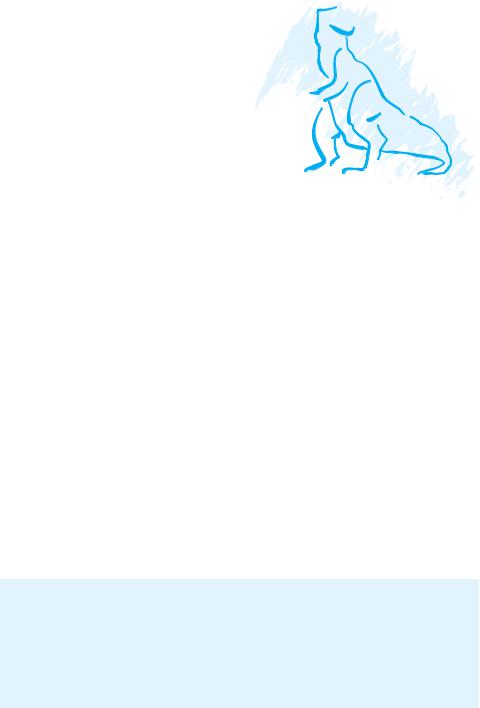
C H7A P T E R
Deadlocks
In a multiprogramming environment, several processes may compete for a finite number of resources. A process requests resources; if the resources are not available at that time, the process enters a waiting state. Sometimes, a waiting process is never again able to change state, because the resources it has requested are held by other waiting processes. This situation is called a deadlock. We discussed this issue briefly in Chapter 5 in connection with semaphores.
Perhaps the best illustration of a deadlock can be drawn from a law passed by the Kansas legislature early in the 20th century. It said, in part: “When two trains approach each other at a crossing, both shall come to a full stop and neither shall start up again until the other has gone.”
In this chapter, we describe methods that an operating system can use to prevent or deal with deadlocks. Although some applications can identify programs that may deadlock, operating systems typically do not provide deadlock-prevention facilities, and it remains the responsibility of programmers to ensure that they design deadlock-free programs. Deadlock problems can only become more common, given current trends, including larger numbers of processes, multithreaded programs, many more resources within a system, and an emphasis on long-lived file and database servers rather than batch systems.
CHAPTER OBJECTIVES
•To develop a description of deadlocks, which prevent sets of concurrent processes from completing their tasks.
•To present a number of different methods for preventing or avoiding deadlocks in a computer system.
7.1System Model
A system consists of a finite number of resources to be distributed among a number of competing processes. The resources may be partitioned into several
315
316Chapter 7 Deadlocks
types (or classes), each consisting of some number of identical instances. CPU cycles, files, and I/O devices (such as printers and DVD drives) are examples of resource types. If a system has two CPUs, then the resource type CPU has two instances. Similarly, the resource type printer may have five instances.
If a process requests an instance of a resource type, the allocation of any instance of the type should satisfy the request. If it does not, then the instances are not identical, and the resource type classes have not been defined properly. For example, a system may have two printers. These two printers may be defined to be in the same resource class if no one cares which printer prints which output. However, if one printer is on the ninth floor and the other is in the basement, then people on the ninth floor may not see both printers as equivalent, and separate resource classes may need to be defined for each printer.
Chapter 5 discussed various synchronization tools, such as mutex locks and semaphores. These tools are also considered system resources, and they are a common source of deadlock. However, a lock is typically associated with protecting a specific data structure —that is, one lock may be used to protect access to a queue, another to protect access to a linked list, and so forth. For that reason, each lock is typically assigned its own resource class, and definition is not a problem.
A process must request a resource before using it and must release the resource after using it. A process may request as many resources as it requires to carry out its designated task. Obviously, the number of resources requested may not exceed the total number of resources available in the system. In other words, a process cannot request three printers if the system has only two.
Under the normal mode of operation, a process may utilize a resource in only the following sequence:
1.Request. The process requests the resource. If the request cannot be granted immediately (for example, if the resource is being used by another process), then the requesting process must wait until it can acquire the resource.
2.Use. The process can operate on the resource (for example, if the resource is a printer, the process can print on the printer).
3.Release. The process releases the resource.
The request and release of resources may be system calls, as explained in Chapter 2. Examples are the request() and release() device, open() and close() file, and allocate() and free() memory system calls. Similarly, as we saw in Chapter 5, the request and release of semaphores can be accomplished through the wait() and signal() operations on semaphores or through acquire() and release() of a mutex lock. For each use of a kernel-managed resource by a process or thread, the operating system checks to make sure that the process has requested and has been allocated the resource. A system table records whether each resource is free or allocated. For each resource that is allocated, the table also records the process to which it is allocated. If a process requests a resource that is currently allocated to another process, it can be added to a queue of processes waiting for this resource.
A set of processes is in a deadlocked state when every process in the set is waiting for an event that can be caused only by another process in the set. The
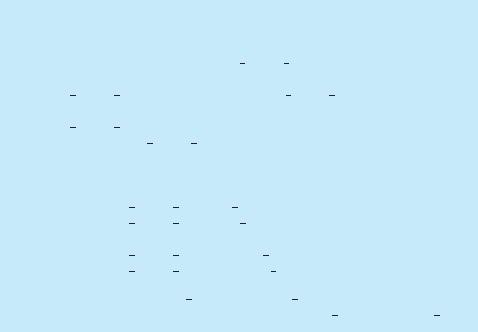
7.2 Deadlock Characterization |
317 |
events with which we are mainly concerned here are resource acquisition and release. The resources may be either physical resources (for example, printers, tape drives, memory space, and CPU cycles) or logical resources (for example, semaphores, mutex locks, and files). However, other types of events may result in deadlocks (for example, the IPC facilities discussed in Chapter 3).
To illustrate a deadlocked state, consider a system with three CD RW drives. Suppose each of three processes holds one of these CD RW drives. If each process now requests another drive, the three processes will be in a deadlocked state. Each is waiting for the event “CD RW is released,” which can be caused only by one of the other waiting processes. This example illustrates a deadlock involving the same resource type.
Deadlocks may also involve different resource types. For example, consider a system with one printer and one DVD drive. Suppose that process Pi is holding the DVD and process Pj is holding the printer. If Pi requests the printer and Pj requests the DVD drive, a deadlock occurs.
Developers of multithreaded applications must remain aware of the possibility of deadlocks. The locking tools presented in Chapter 5 are designed to avoid race conditions. However, in using these tools, developers must pay careful attention to how locks are acquired and released. Otherwise, deadlock can occur, as illustrated in the dining-philosophers problem in Section 5.7.3.
7.2Deadlock Characterization
In a deadlock, processes never finish executing, and system resources are tied up, preventing other jobs from starting. Before we discuss the various methods for dealing with the deadlock problem, we look more closely at features that characterize deadlocks.
DEADLOCK WITH MUTEX LOCKS
Let’s see how deadlock can occur in a multithreaded Pthread program using mutex locks. The pthread mutex init() function initializes an unlocked mutex. Mutex locks are acquired and released using pthread mutex lock() and pthread mutex unlock(), respectively. If a thread attempts to acquire a locked mutex, the call to pthread mutex lock() blocks the thread until the owner of the mutex lock invokes pthread mutex unlock().
Two mutex locks are created in the following code example:
/* Create and initialize the mutex locks */ pthread mutex t first mutex;
pthread mutex t second mutex;
pthread mutex init(&first mutex,NULL); pthread mutex init(&second mutex,NULL);
Next, two threads — thread one and thread two— are created, and both these threads have access to both mutex locks. thread one and thread two
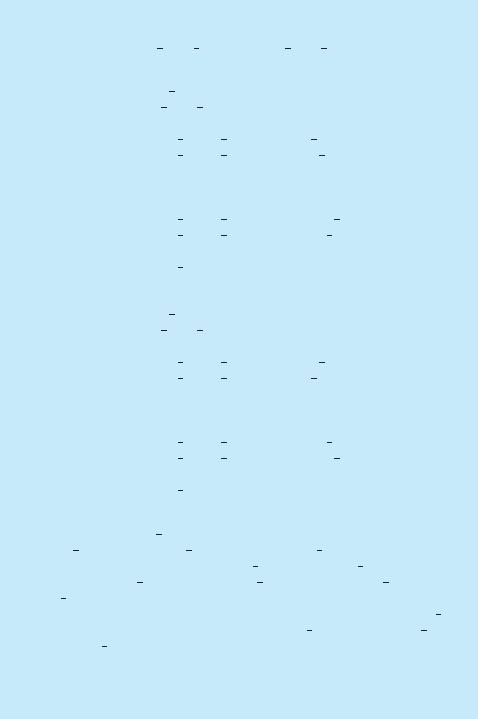
318 |
Chapter 7 Deadlocks |
DEADLOCK WITH MUTEX LOCKS (Continued)
run in the functions do work one() and do work two(), respectively, as shown below:
/* thread one runs in this function */ void *do work one(void *param)
{
pthread mutex lock(&first mutex); pthread mutex lock(&second mutex); /**
* Do some work */
pthread mutex unlock(&second mutex); pthread mutex unlock(&first mutex);
pthread exit(0);
}
/* thread two runs in this function */ void *do work two(void *param)
{
pthread mutex lock(&second mutex); pthread mutex lock(&first mutex); /**
* Do some work */
pthread mutex unlock(&first mutex); pthread mutex unlock(&second mutex);
pthread exit(0);
}
In this example, thread one attempts to acquire the mutex locks in the order
(1) first mutex, (2) second mutex, while thread two attempts to acquire the mutex locks in the order (1) second mutex, (2) first mutex. Deadlock is possible if thread one acquires first mutex while thread two acquires second mutex.
Note that, even though deadlock is possible, it will not occur if thread one can acquire and release the mutex locks for first mutex and second mutex before thread two attempts to acquire the locks. And, of course, the order in which the threads run depends on how they are scheduled by the CPU scheduler. This example illustrates a problem with handling deadlocks: it is difficult to identify and test for deadlocks that may occur only under certain scheduling circumstances.
7.2.1 Necessary Conditions
A deadlock situation can arise if the following four conditions hold simultaneously in a system:
7.2 Deadlock Characterization |
319 |
1.Mutual exclusion. At least one resource must be held in a nonsharable mode; that is, only one process at a time can use the resource. If another process requests that resource, the requesting process must be delayed until the resource has been released.
2.Hold and wait. A process must be holding at least one resource and waiting to acquire additional resources that are currently being held by other processes.
3.No preemption. Resources cannot be preempted; that is, a resource can be released only voluntarily by the process holding it, after that process has completed its task.
4.Circular wait. A set {P0, P1, ..., Pn} of waiting processes must exist such that P0 is waiting for a resource held by P1, P1 is waiting for a resource held by P2, ..., Pn−1 is waiting for a resource held by Pn, and Pn is waiting for a resource held by P0.
We emphasize that all four conditions must hold for a deadlock to occur. The circular-wait condition implies the hold-and-wait condition, so the four conditions are not completely independent. We shall see in Section 7.4, however, that it is useful to consider each condition separately.
7.2.2Resource-Allocation Graph
Deadlocks can be described more precisely in terms of a directed graph called a system resource-allocation graph. This graph consists of a set of vertices V and a set of edges E. The set of vertices V is partitioned into two different types of nodes: P = {P1, P2, ..., Pn}, the set consisting of all the active processes in the system, and R = {R1, R2, ..., Rm}, the set consisting of all resource types in the system.
A directed edge from process Pi to resource type Rj is denoted by Pi → Rj ; it signifies that process Pi has requested an instance of resource type Rj and is currently waiting for that resource. A directed edge from resource type Rj to process Pi is denoted by Rj → Pi ; it signifies that an instance of resource type Rj has been allocated to process Pi . A directed edge Pi → Rj is called a request edge; a directed edge Rj → Pi is called an assignment edge.
Pictorially, we represent each process Pi as a circle and each resource type Rj as a rectangle. Since resource type Rj may have more than one instance, we represent each such instance as a dot within the rectangle. Note that a request edge points to only the rectangle Rj , whereas an assignment edge must also designate one of the dots in the rectangle.
When process Pi requests an instance of resource type Rj , a request edge is inserted in the resource-allocation graph. When this request can be fulfilled, the request edge is instantaneously transformed to an assignment edge. When the process no longer needs access to the resource, it releases the resource. As a result, the assignment edge is deleted.
The resource-allocation graph shown in Figure 7.1 depicts the following situation.
• The sets P, R, and E:
◦ P = {P1, P2, P3}

320 |
Chapter 7 Deadlocks |
R1 R3
P1 |
P2 |
P3 |
R2
R4
Figure 7.1 Resource-allocation graph.
◦R = {R1, R2, R3, R4}
◦E = {P1 → R1, P2 → R3, R1 → P2, R2 → P2, R2 → P1, R3 → P3}
•Resource instances:
◦One instance of resource type R1
◦Two instances of resource type R2
◦One instance of resource type R3
◦Three instances of resource type R4
•Process states:
◦Process P1 is holding an instance of resource type R2 and is waiting for an instance of resource type R1.
◦Process P2 is holding an instance of R1 and an instance of R2 and is waiting for an instance of R3.
◦Process P3 is holding an instance of R3.
Given the definition of a resource-allocation graph, it can be shown that, if the graph contains no cycles, then no process in the system is deadlocked. If the graph does contain a cycle, then a deadlock may exist.
If each resource type has exactly one instance, then a cycle implies that a deadlock has occurred. If the cycle involves only a set of resource types, each of which has only a single instance, then a deadlock has occurred. Each process involved in the cycle is deadlocked. In this case, a cycle in the graph is both a necessary and a sufficient condition for the existence of deadlock.
If each resource type has several instances, then a cycle does not necessarily imply that a deadlock has occurred. In this case, a cycle in the graph is a necessary but not a sufficient condition for the existence of deadlock.
To illustrate this concept, we return to the resource-allocation graph depicted in Figure 7.1. Suppose that process P3 requests an instance of resource
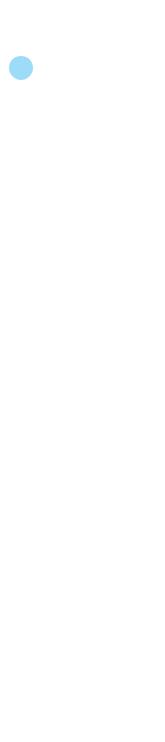
7.2 Deadlock Characterization |
321 |
R1 R3
P1 |
P2 |
P3 |
R2
R4
Figure 7.2 Resource-allocation graph with a deadlock.
type R2. Since no resource instance is currently available, we add a request edge P3 → R2 to the graph (Figure 7.2). At this point, two minimal cycles exist in the system:
P1 → R1 → P2 → R3 → P3 → R2 → P1
P2 → R3 → P3 → R2 → P2
Processes P1, P2, and P3 are deadlocked. Process P2 is waiting for the resource R3, which is held by process P3. Process P3 is waiting for either process P1 or process P2 to release resource R2. In addition, process P1 is waiting for process P2 to release resource R1.
Now consider the resource-allocation graph in Figure 7.3. In this example, we also have a cycle:
P1 → R1 → P3 → R2 → P1
P2
R1
P3
P1
R2
P4
Figure 7.3 Resource-allocation graph with a cycle but no deadlock.