
lafore_robert_objectoriented_programming_in_c
.pdf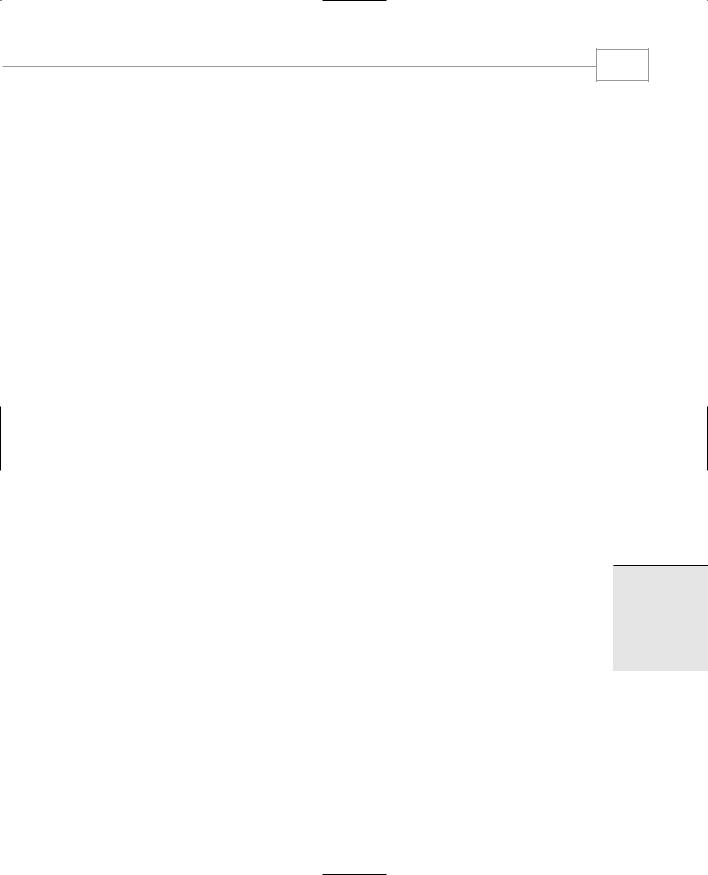
Structures
This is a cleaner approach than using const variables. We know exactly what the possible values of the suit are; attempts to use other values, as in
card1.suit = 5;
result in warnings from the compiler.
Specifying Integer Values
We said that in an enum declaration the first enumerator was given the integer value 0, the second the value 1, and so on. This ordering can be altered by using an equal sign to specify a starting point other than 0. For example, if you want the suits to start with 1 instead of 0, you can say
enum Suit { clubs=1, diamonds, hearts, spades };
Subsequent names are given values starting at this point, so diamonds is 2, hearts is 3, and spades is 4. Actually you can use an equal sign to give a specified value to any enumerator.
Not Perfect
One annoying aspect of enum types is that they are not recognized by C++ input/output (I/O) statements. As an example, what do you think the following code fragment will cause to be displayed?
enum direction { north, south, east, west }; direction dir1 = south;
cout << dir1;
Did you guess the output would be south? That would be nice, but C++ I/O treats variables of enum types as integers, so the output would be 1.
Other Examples
Here are some other examples of enumerated data declarations, to give you a feeling for possible uses of this feature:
enum months { Jan, Feb, Mar, Apr, May, Jun, Jul, Aug, Sep, Oct, Nov, Dec };
enum switch { off, on };
enum meridian { am, pm };
enum chess { pawn, knight, bishop, rook, queen, king };
enum coins { penny, nickel, dime, quarter, half-dollar, dollar };
155
4
STRUCTURES
We’ll see other examples in future programs.

Chapter 4
156
Summary
We’ve covered two topics in this chapter: structures and enumerations. Structures are an important component of C++, since their syntax is the same as that of classes. In fact, classes are (syntactically, at least) nothing more than structures that include functions. Structures are typically used to group several data items together to form a single entity. A structure definition lists the variables that make up the structure. Other definitions then set aside memory for structure variables. Structure variables are treated as indivisible units in some situations (such as setting one structure variable equal to another), but in other situations their members are accessed individually (often using the dot operator).
An enumeration is a programmer-defined type that is limited to a fixed list of values. A declaration gives the type a name and specifies the permissible values, which are called enumerators. Definitions can then create variables of this type. Internally the compiler treats enumeration variables as integers.
Structures should not be confused with enumerations. Structures are a powerful and flexible way of grouping a diverse collection of data into a single entity. An enumeration allows the definition of variables that can take on a fixed set of values that are listed (enumerated) in the type’s declaration.
Questions
Answers to these questions can be found in Appendix G.
1.A structure brings together a group of
a.items of the same data type.
b.related data items.
c.integers with user-defined names.
d.variables.
2.True or false: A structure and a class use similar syntax.
3.The closing brace of a structure is followed by a __________.
4.Write a structure specification that includes three variables—all of type int—called hrs, mins, and secs. Call this structure time.
5.True or false: A structure definition creates space in memory for a variable.
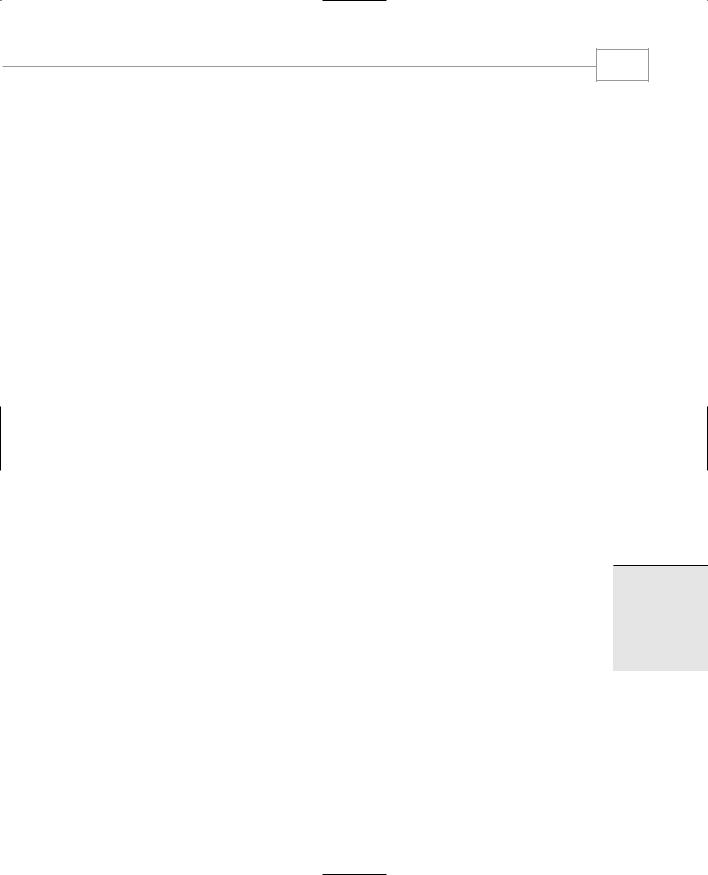
Structures
157
6.When accessing a structure member, the identifier to the left of the dot operator is the name of
a.a structure member.
b.a structure tag.
c.a structure variable.
d.the keyword struct.
7.Write a statement that sets the hrs member of the time2 structure variable equal to 11.
8.If you have three variables defined to be of type struct time, and this structure contains three int members, how many bytes of memory do the variables use together?
9.Write a definition that initializes the members of time1—which is a variable of type struct time, as defined in Question 4—to hrs = 11, mins = 10, secs = 59.
10.True or false: You can assign one structure variable to another, provided they are of the same type.
11.Write a statement that sets the variable temp equal to the paw member of the dogs member of the fido variable.
12.An enumeration brings together a group of
a.items of different data types.
b.related data variables.
c.integers with user-defined names.
d.constant values.
13.Write a statement that declares an enumeration called players with the values B1, B2, SS, B3, RF, CF, LF, P, and C.
14.Assuming the enum type players as declared in Question 13, define two variables joe and tom, and assign them the values LF and P, respectively.
15.Assuming the statements of Questions 13 and 14, state whether each of the following statements is legal.
a.joe = QB;
b.tom = SS;
c.LF = tom;
d.difference = joe - tom;
16.The first three enumerators of an enum type are normally represented by the values
_________, _________, and _________.
17.Write a statement that declares an enumeration called speeds with the enumerators obsolete, single, and album. Give these three names the integer values 78, 45, and 33.
4
STRUCTURES

Chapter 4
158
18. State the reason that
enum isWord{ NO, YES };
is better than
enum isWord{ YES, NO };
Exercises
Answers to the starred exercises can be found in Appendix G.
*1. A phone number, such as (212) 767-8900, can be thought of as having three parts: the area code (212), the exchange (767), and the number (8900). Write a program that uses a structure to store these three parts of a phone number separately. Call the structure phone. Create two structure variables of type phone. Initialize one, and have the user input a number for the other one. Then display both numbers. The interchange might look like this:
Enter your area code, exchange, and number: 415 555 1212
My number is (212) 767-8900
Your number is (415) 555-1212
*2. A point on the two-dimensional plane can be represented by two numbers: an x coordinate and a y coordinate. For example, (4,5) represents a point 4 units to the right of the vertical axis, and 5 units up from the horizontal axis. The sum of two points can be defined as a new point whose x coordinate is the sum of the x coordinates of the two points, and whose y coordinate is the sum of the y coordinates.
Write a program that uses a structure called point to model a point. Define three points, and have the user input values to two of them. Then set the third point equal to the sum of the other two, and display the value of the new point. Interaction with the program might look like this:
Enter coordinates for p1: 3 4
Enter coordinates for p2: 5 7
Coordinates of p1+p2 are: 8, 11
*3. Create a structure called Volume that uses three variables of type Distance (from the ENGLSTRC example) to model the volume of a room. Initialize a variable of type Volume to specific dimensions, then calculate the volume it represents, and print out the result. To calculate the volume, convert each dimension from a Distance variable to a variable of type float representing feet and fractions of a foot, and then multiply the resulting three numbers.
4.Create a structure called employee that contains two members: an employee number (type int) and the employee’s compensation (in dollars; type float). Ask the user to fill in this data for three employees, store it in three variables of type struct employee, and then display the information for each employee.

Structures
159
5.Create a structure of type date that contains three members: the month, the day of the month, and the year, all of type int. (Or use day-month-year order if you prefer.) Have the user enter a date in the format 12/31/2001, store it in a variable of type struct date, then retrieve the values from the variable and print them out in the same format.
6.We said earlier that C++ I/O statements don’t automatically understand the data types of enumerations. Instead, the (>>) and (<<) operators think of such variables simply as integers. You can overcome this limitation by using switch statements to translate between the user’s way of expressing an enumerated variable and the actual values of the enumerated variable. For example, imagine an enumerated type with values that indicate an employee type within an organization:
enum etype { laborer, secretary, manager, accountant, executive,
researcher };
Write a program that first allows the user to specify a type by entering its first letter (‘l’, ‘s’, ‘m’, and so on), then stores the type chosen as a value of a variable of type enum etype, and finally displays the complete word for this type.
Enter employee type (first letter only) laborer, secretary, manager, accountant, executive, researcher): a
Employee type is accountant.
You’ll probably need two switch statements: one for input and one for output.
7.Add a variable of type enum etype (see Exercise 6), and another variable of type struct date (see Exercise 5) to the employee class of Exercise 4. Organize the resulting program so that the user enters four items of information for each of three employees: an employee number, the employee’s compensation, the employee type, and the date of first employment. The program should store this information in three variables of type
employee, and then display their contents.
8.Start with the fraction-adding program of Exercise 9 in Chapter 2, “C++ Programming Basics.” This program stores the numerator and denominator of two fractions before adding them, and may also store the answer, which is also a fraction. Modify the program so that all fractions are stored in variables of type struct fraction, whose two members are the fraction’s numerator and denominator (both type int). All fractionrelated data should be stored in structures of this type.
9.Create a structure called time. Its three members, all type int, should be called hours, minutes, and seconds. Write a program that prompts the user to enter a time value in hours, minutes, and seconds. This can be in 12:59:59 format, or each number can be entered at a separate prompt (“Enter hours:”, and so forth). The program should then store the time in a variable of type struct time, and finally print out the total number of seconds represented by this time value:
4
STRUCTURES
long totalsecs = t1.hours*3600 + t1.minutes*60 + t1.seconds

Chapter 4
160
10.Create a structure called sterling that stores money amounts in the old-style British system discussed in Exercises 8 and 11 in Chapter 3, “Loops and Decisions.” The members could be called pounds, shillings, and pence, all of type int. The program should ask the user to enter a money amount in new-style decimal pounds (type double), convert it to the old-style system, store it in a variable of type struct sterling, and then display this amount in pounds-shillings-pence format.
11.Use the time structure from Exercise 9, and write a program that obtains two time values from the user in 12:59:59 format, stores them in struct time variables, converts each one to seconds (type int), adds these quantities, converts the result back to hours- minutes-seconds, stores the result in a time structure, and finally displays the result in 12:59:59 format.
12.Revise the four-function fraction calculator program of Exercise 12 in Chapter 3 so that each fraction is stored internally as a variable of type struct fraction, as discussed in Exercise 8 in this chapter.
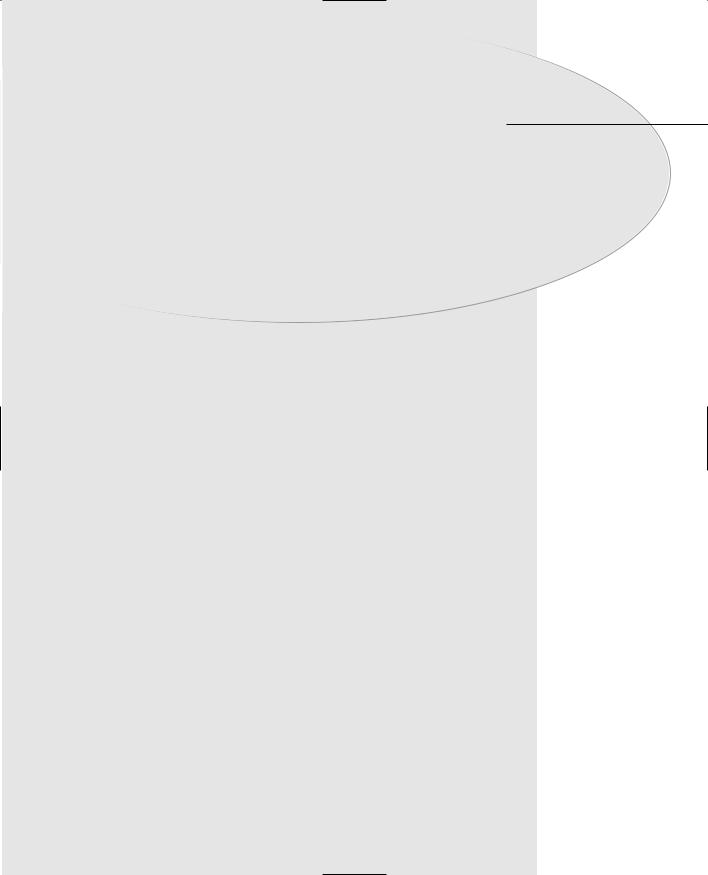
Functions
IN THIS CHAPTER
• |
Simple Functions |
162 |
|
|
|
• |
Passing Arguments to Functions |
167 |
|||
• |
Returning Values from Functions |
176 |
|||
• Reference Arguments |
182 |
|
|||
• |
Overloaded Functions |
188 |
|
||
• |
Recursion 193 |
|
|
|
|
• |
Inline Functions |
195 |
|
|
|
• |
Default Arguments 197 |
|
|
||
• |
Scope and Storage Class |
199 |
|
||
• |
Returning by Reference |
206 |
|
||
• |
const Function Arguments |
208 |
|
CHAPT E R
5
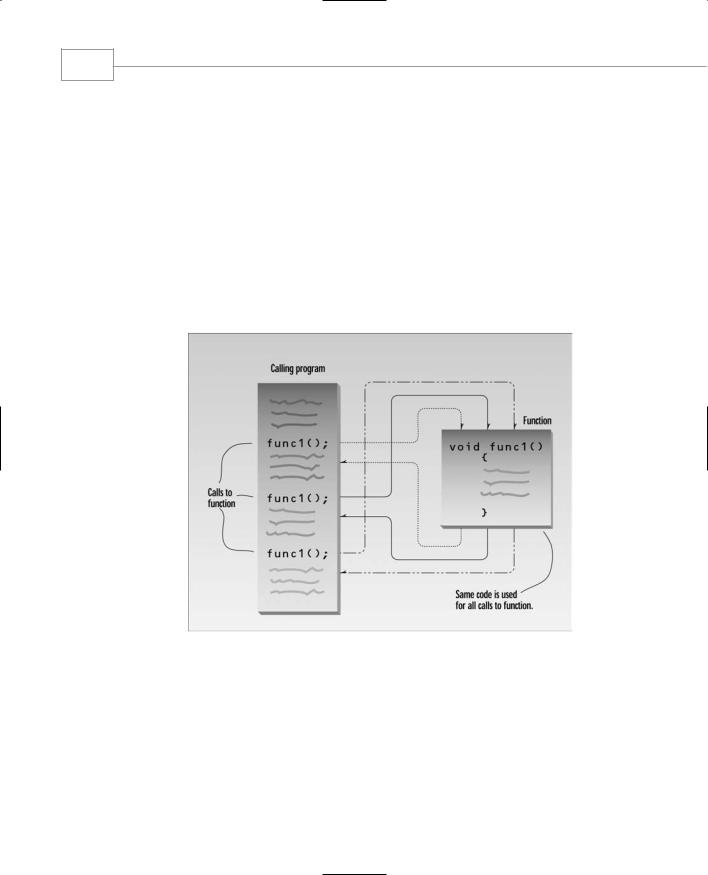
Chapter 5
162
A function groups a number of program statements into a unit and gives it a name. This unit can then be invoked from other parts of the program.
The most important reason to use functions is to aid in the conceptual organization of a program. Dividing a program into functions is, as we discussed in Chapter 1, “The Big Picture,” one of the major principles of structured programming. (However, object-oriented programming provides additional, more powerful ways to organize programs.)
Another reason to use functions (and the reason they were invented, long ago) is to reduce program size. Any sequence of instructions that appears in a program more than once is a candidate for being made into a function. The function’s code is stored in only one place in memory, even though the function is executed many times in the course of the program. Figure 5.1 shows how a function is invoked from different sections of a program.
FIGURE 5.1
Flow of control to a function.
Functions in C++ (and C) are similar to subroutines and procedures in various other languages.
Simple Functions
Our first example demonstrates a simple function whose purpose is to print a line of 45 asterisks. The example program generates a table, and lines of asterisks are used to make the table more readable. Here’s the listing for TABLE:

Functions
163
//table.cpp
//demonstrates simple function #include <iostream>
using namespace std;
void starline(); |
|
//function declaration |
|
|
// (prototype) |
int main() |
|
|
{ |
|
|
starline(); |
|
//call to function |
cout << “Data type |
Range” << endl; |
|
starline(); |
|
//call to function |
cout << “char |
-128 to 127” << endl |
|
<< “short |
-32,768 to 32,767” << endl |
|
<< “int |
System dependent” << endl |
|
<< “long |
-2,147,483,648 to 2,147,483,647” << endl; |
|
starline(); |
|
//call to function |
return 0; |
|
|
} |
|
|
//-------------------------------------------------------------- |
|
|
// starline() |
|
|
// function definition |
|
|
void starline() |
|
//function declarator |
{ |
|
|
for(int j=0; j<45; j++) |
//function body |
|
cout << ‘*’; |
|
|
cout << endl; |
|
|
} |
|
|
The output from the program looks like this:
*********************************************
Data type Range
*********************************************
char |
-128 to |
127 |
short |
-32,768 |
to 32,767 |
int |
System dependent |
|
long |
-2,147,483,648 to 2,147,483,647 |
*********************************************
The program consists of two functions: main() and starline(). You’ve already seen many programs that use main() alone. What other components are necessary to add a function to the program? There are three: the function declaration, the calls to the function, and the function definition.
5
FUNCTIONS

Chapter 5
164
The Function Declaration
Just as you can’t use a variable without first telling the compiler what it is, you also can’t use a function without telling the compiler about it. There are two ways to do this. The approach we show here is to declare the function before it is called. (The other approach is to define it before it’s called; we’ll examine that next.) In the TABLE program, the function starline() is declared in the line
void starline();
The declaration tells the compiler that at some later point we plan to present a function called starline. The keyword void specifies that the function has no return value, and the empty parentheses indicate that it takes no arguments. (You can also use the keyword void in parentheses to indicate that the function takes no arguments, as is often done in C, but leaving them empty is the more common practice in C++.) We’ll have more to say about arguments and return values soon.
Notice that the function declaration is terminated with a semicolon. It is a complete statement in itself.
Function declarations are also called prototypes, since they provide a model or blueprint for the function. They tell the compiler, “a function that looks like this is coming up later in the program, so it’s all right if you see references to it before you see the function itself.” The information in the declaration (the return type and the number and types of any arguments) is also sometimes referred to as the function signature.
Calling the Function
The function is called (or invoked, or executed) three times from main(). Each of the three calls looks like this:
starline();
This is all we need to call the function: the function name, followed by parentheses. The syntax of the call is very similar to that of the declaration, except that the return type is not used. The call is terminated by a semicolon. Executing the call statement causes the function to execute; that is, control is transferred to the function, the statements in the function definition (which we’ll examine in a moment) are executed, and then control returns to the statement following the function call.
The Function Definition
Finally we come to the function itself, which is referred to as the function definition. The definition contains the actual code for the function. Here’s the definition for starline():