
Real - World ASP .NET—Building a Content Management System - StephenR. G. Fraser
.pdf
Params = m_UpdateCommand.Parameters;
Params.Add(new SqlParameter("@AccountID", |
SqlDbType.Int)); |
|
|
|
|
|
|
|
|
Params.Add(new SqlParameter("@UserName", |
SqlDbType.Char, 32)); |
|||
|
|
|
||
Params.Add(new SqlParameter("@Password", |
SqlDbType.Char, 40)); |
|
||
|
|
|||
Params.Add(new SqlParameter("@Email", |
SqlDbType.Char, 64)); |
|
Params.Add(new SqlParameter("@ModifiedDate", SqlDbType.DateTime));
}
Params = m_UpdateCommand.Parameters;
Params["@AccountID"].Value |
= AccountID; |
|
||
|
|
|
|
|
Params["@UserName"].Value |
= UserName; |
|||
|
|
|
||
Params["@Password"].Value |
= Password; |
|
||
|
|
|||
Params["@Email"].Value |
= Email; |
|
Params["@ModifiedDate"].Value = DateTime.Now;
try
{
m_Connection.Open(); m_UpdateCommand.ExecuteNonQuery();
}
finally
{
m_Connection.Close();
}
}
The Exist() method (see Listing 10-14) is a simple select on the Account table, seeing if the AccountID parameter passed exists. For a change—and to show how to

create an SqlDataAdapter using an SqlConnection (I should probably be using a
stored procedure here as well)—the method uses a DataSet to capture all AccountIDs that match the method's parameter. There should always be only one.
Listing 10-14: The Account.Exist() Method
public bool Exist(int AccountID)
{
string Command = "Select * from Account WHERE AccountID="+AccountID;
SqlDataAdapter DSCmd = new SqlDataAdapter(Command, m_Connection);
DataSet ds = new DataSet();
DSCmd.Fill(ds, "Account");
return (ds.Tables["Account"].Rows.Count > 0);
}
The two stored procedures for the Account table are pretty standard fare and, for someone with SQL knowledge, should be trivial. The first is simply a standard SQL insert (see Listing 10-15).
Listing 10-15: The Account_Insert Stored Procedure
CREATE PROCEDURE dbo.Account_Insert
(
@UserName NVARCHAR(32) = NULL,
@Password NVARCHAR(40) = NULL,
@Email NVARCHAR(64) = NULL,
@ModifiedDate DATETIME = NULL,
@CreationDate DATETIME = NULL
)
AS
SET NOCOUNT ON
INSERT INTO Account (UserName, Password, Email, ModifiedDate,

CreationDate)
VALUES |
(@UserName, @Password, @Email, @ModifiedDate, |
@CreationDate)
RETURN
The second stored procedure is a standard update. It uses AccountID as the key to determine where to insert (see Listing 10-16).
Listing 10-16: The UpdateAccount Stored Procedure
CREATE PROCEDURE dbo.Account_Update
(
@AccountID INT = NULL,
@UserName NVARCHAR(32) = NULL,
@Password NVARCHAR(40) = NULL,
@Email NVARCHAR(64) = NULL,
@ModifiedDate DATETIME = NULL
)
AS
SET NOCOUNT ON
UPDATE Account
SET UserName = @UserName,
Password = @Password,
Email = @Email,
ModifiedDate = @ModifiedDate
WHERE AccountID = @AccountID

RETURN
DataAccess/AccountProperty.cs
The second half of the two parts of a user account is stored in the AccountProperty table. As previously described, AccountProperty holds all user information that is not essential for CMS.NET to function. This database helper function aids in the storing and retrieving of data into and out of the AccountProperty database table.
For the setup process, you only have to worry about the storing of data. This means you only need the Insert() and Update() methods. In later chapters, we will add some methods to retrieve data out of the table.
The constructor (see Listing 10-17) is identical to all database helper classes and requires an SqlConnection passed as a parameter. The SqlConnection is then used later to open a connection to the CMSNET database.
Listing 10-17: The AccountProperty Constructor Method
public class AccountProperty
{
private SqlConnection m_Connection;
private SqlCommand m_InsertCommand;
private SqlCommand m_UpdateCommand;
public AccountProperty(SqlConnection Connection)
{
m_Connection = Connection;
}
As you saw in the Account database helper class, the AccountProperty's Insert() method (see Listing 10-18) is coded to simply set up the call to the AccountProperty_Insert stored procedure, store the setup for later calls to insert, initialize all the parameters, and then make the call to the stored procedure. You will see virtually the same thing in all database helper classes.
Listing 10-18: The AccountProperty.Insert() Method
public void Insert(int AccountID, string Property, string Value)
{
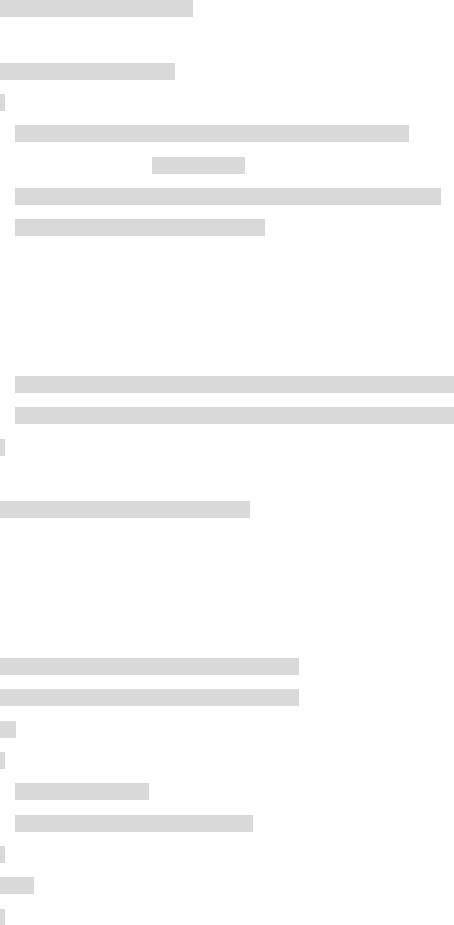
SqlParameterCollection Params;
if (m_InsertCommand == null)
{
m_InsertCommand = new SqlCommand("AccountProperty_Insert", m_Connection);
m_InsertCommand.CommandType = CommandType.StoredProcedure;
Params = m_InsertCommand.Parameters;
Params.Add(new SqlParameter("@AccountID", |
SqlDbType.Int)); |
|
|
|
|
|
|
Params.Add(new SqlParameter("@Property", |
SqlDbType.Char, 32)); |
||
|
|
||
Params.Add(new SqlParameter("@Value", |
SqlDbType.Text)); |
|
Params.Add(new SqlParameter("@ModifiedDate", SqlDbType.DateTime));
Params.Add(new SqlParameter("@CreationDate", SqlDbType.DateTime));
}
Params = m_InsertCommand.Parameters;
Params["@AccountID"].Value |
= AccountID; |
||
|
|
|
|
Params["@Property"].Value |
= Property; |
|
|
|
|
||
Params["@Value"].Value |
= Value; |
|
Params["@ModifiedDate"].Value = DateTime.Now; Params["@CreationDate"].Value = DateTime.Now; try
{
m_Connection.Open();
m_InsertCommand.ExecuteNonQuery();
}
finally
{

m_Connection.Close();
}
}
AccountProperty's Update() method (see Listing 10-19) is virtually the same as the Insert() method. The only differences are that the UpdateAccountProperty stored procedure is called and the CreationDate is not set up as a parameter. This is because it will not be updated because, obviously, you are not creating the record now.
Listing 10-19: The AccountProperty.Update() Method
public void Update(int AccountID, string Property, string Value)
{
SqlParameterCollection Params;
if ( m_UpdateCommand == null)
{
m_UpdateCommand = new SqlCommand("AccountProperty_Update",
m_Connection);
m_UpdateCommand.CommandType = CommandType.StoredProcedure;
Params = m_UpdateCommand.Parameters;
Params.Add(new SqlParameter("@AccountID", |
SqlDbType.Int)); |
|
|
|
|
|
|
Params.Add(new SqlParameter("@Property", |
SqlDbType.Char, 32)); |
||
|
|
||
Params.Add(new SqlParameter("@Value", |
SqlDbType.Text)); |
|
Params.Add(new SqlParameter("@ModifiedDate", SqlDbType.DateTime));
}
Params = m_UpdateCommand.Parameters;
Params["@AccountID"].Value |
= AccountID; |
|
|
|
|
Params["@Property"].Value |
= Property; |
|

Params["@Value"].Value |
= Value; |
Params["@ModifiedDate"].Value = DateTime.Now;
try
{
m_Connection.Open();
m_UpdateCommand.ExecuteNonQuery();
}
finally
{
m_Connection.Close();
}
}
The real difference in the Insert() and Update() methods occurs in their stored procedures. In the case of the AccountProperty_Insert stored procedure (see Listing 10-20), it is simply a standard SQL insert. This is hardly rocket science when it comes to SQL.
Listing 10-20: The AccountProperty_Insert Stored Procedure
CREATE PROCEDURE dbo.AccountProperty_Insert
(
@AccountID INT |
= NULL, |
|
|
|
|
||
@Property NVARCHAR(32) = NULL, |
|||
|
|
||
@Value TEXT |
= NULL, |
|
@ModifiedDate DATETIME = NULL,
@CreationDate DATETIME = NULL
)
AS
SET NOCOUNT ON
INSERT INTO AccountProperty (AccountID, Property, Value, ModifiedDate,
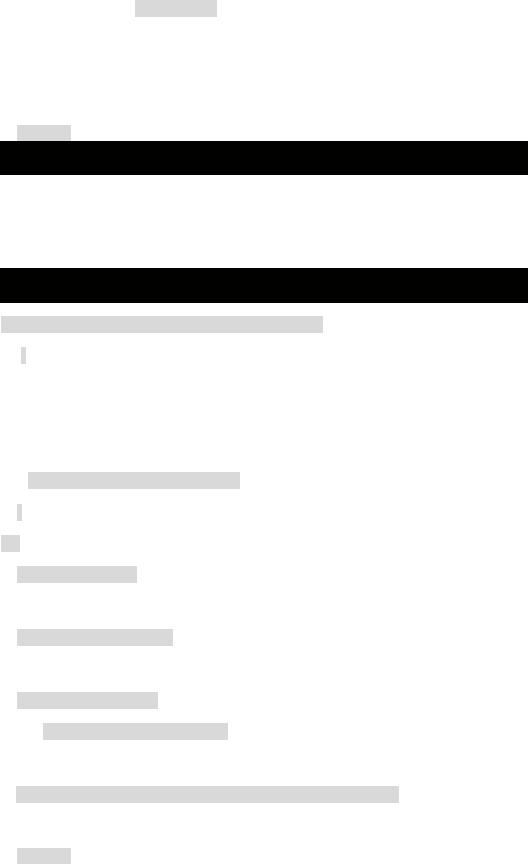
CreationDate)
VALUES |
(@AccountID, @Property, @Value, @ModifiedDate, @CreationDate) |
RETURN
The UpdateAccountProperty stored procedure (see Listing 10-21) is simply a standard SQL update. In this case, the insertion uses a key of both the AccountID and the Property (the key of the key/value pair).
Listing 10-21: The UpdateAccountProperty Stored Procedure
CREATE PROCEDURE dbo.AccountProperty_Update
(
@AccountID INT |
= NULL, |
|
|
|
|
||
@Property NVARCHAR(32) = NULL, |
|||
|
|
||
@Value TEXT |
= NULL, |
|
@ModifiedDate DATETIME = NULL
)
AS
SET NOCOUNT ON
UPDATE AccountProperty
SET Value = @Value,
ModifiedDate = @ModifiedDate
WHERE (AccountID = @AccountID AND Property = @Property)
RETURN

Finally, Wrap Up the Installation
It is always a good idea to let users know that they are finished and that they completed successfully. I hate getting statements at the end of setup such as "Successfully completed but some nonfatal errors occurred during setup." Believe it or not, these types of messages actually appear quite frequently at the end of software setup procedures. I always wonder what is going to happen if I try to access a program in which a nonfatal error occurred. Will my software work properly? As far as I am concerned, a setup was successful or it was not. A "but" clause has no place at the end of a setup procedure.
Okay, I'll get off my soapbox—for now.
The Setup/se tup4.aspx Web Page
Setup4 is designed to tell the user that everything is fine, now go ahead and play. As you see in Figure 10-7, there is no guesswork on the part of the user. The process has ended, and it completed successfully. It even provides a button so that the user can go and have fun immediately.
Figure 10-7: Setup/setup4.aspx
The Setup/setup4.cs Codebehind
Setup4 still requires a little bit of work before it is free to display the cheery message of successful completion. It needs to set the <appSettings> add setup attribute value in the web.config file to true. By setting it to true, the admin.aspx (which was discussed at the beginning of the chapter) knows that it can now run CMS.NET's administration system.
The code for the Page_Load() method (see Listing 10-22) is virtually the same as the UpdateConfigWeb() method found in setup2.cs. First, you open the web.config. Then,
navigate to the <appSettings> add setup attribute. Next, you set the value to true. Finally, you update web.config to disk.
Listing 10-22: The setup4.Page_load Method
private void Page_Load(object sender, System.EventArgs e)
{
if (!IsPostBack)
{
XmlReader xtr = new
XmlTextReader(File.OpenRead(Server.MapPath("..\\web.config")));

XmlDocument doc = new XmlDocument();
doc.Load(xtr);
xtr.Close();
XmlNodeList nodes =
doc.DocumentElement.GetElementsByTagName("appSettings");
for (int i = 0; i < nodes.Count; i++)
{
XmlNodeList appnodes = ((XmlElement)(nodes.Item(i))).GetElementsByTagName("add");
for (int j = 0; j < appnodes.Count; j++)
{
XmlAttributeCollection attrColl = appnodes.Item(j).Attributes; XmlAttribute tmpNode =
(XmlAttribute)attrColl.GetNamedItem("key"); if (tmpNode.Value.Equals("setup"))
{
((XmlAttribute)attrColl.GetNamedItem("value")).Value = "true";
}
}
}
File.Copy(Server.MapPath("..\\web.config"), Server.MapPath("..\\web.config.002"), true);
File.Delete(Server.MapPath("..\\web.config"));
StreamWriter sr = new
StreamWriter(File.OpenWrite(Server.MapPath("..\\web.config")));
doc.Save(sr);
sr.Close();
}
}
The final method I need to cover is the btnLogin_Click() method (see Listing 10-23). This method enables the happy event of leaving the setup process and starting the administration process of the Web site.
Listing 10-23: The setup4.btnLogin_Click Method
private void btnLogin_Click (object sender, System.EventArgs e)
{
Response.Redirect("../admin.aspx");