
Real - World ASP .NET—Building a Content Management System - StephenR. G. Fraser
.pdf
Updating Content
Most authors don't get their stories perfect the first time. Thus, there will always be a need for an AutUpdate.aspx Web form.
The AutUpdate Web Page
As you see in Figure 11-9, there is nothing special about the form. In fact, it is very similar to the content -creation Web form shown earlier except that it displays a few autogenerated fields as read-only so that the author can make sure she is updating the correct version.
Figure 11-9: The AutUpdate Web page
There is nothing special about the code used to design the form, as Listing 11-12 shows. It is made up of five labels, four text boxes, and five buttons, none of which have any special features.
Listing 11-12: The AutUpdate Web Design
<form id="AutUpdate" method="post" runat="server">
<H2><FONT color=darkslategray >Author : Content Update</FONT></H2>
<P>
<asp:ValidationSummary id=ValSum runat="server"
HeaderText="Error(s) occurred while creating content">
</asp:ValidationSummary >
<TABLE cellSpacing=1 cellPadding=1 width="95%" border=1>
<TR>
<TD style="WIDTH: 16%"><STRONG>ContentID: </STRONG></TD >
<TD style="WIDTH: 80%">
<asp:label id=lbContentID runat="server" Width="100%"></asp:label>
</TD>
<TD> </TD>
</TR > <TR>
<TD style="WIDTH: 16%"><STRONG>Version: </STRONG></TD > <TD style="WIDTH: 80%">
<asp:Label id=lbVersion runat="server"></asp:Label>
</TD>
<TD> </TD>
</TR > <TR>
<TD style="WIDTH: 16%"><STRONG>Headline:</STRONG></TD> <TD style="WIDTH: 80%">
<asp:TextBox id=tbHeadline runat="server" Width="100%" TextMode="MultiLine">
</asp:TextBox> </TD>
<TD>
<asp:RequiredFieldValidator id=rfvHeadline runat="server" ErrorMessage="A Headline must be entered." Display="Dynamic"
ControlToValidate="tbHeadline" EnableClientScript="False" >*
</asp:RequiredFieldValidator>
</TD>
</TR > <TR>
<TD style="WIDTH: 16%"><STRONG>Byline: </STRONG></TD > <TD style="WIDTH: 80%">
<asp:label id=lbByline runat="server" Width="100%"></asp:label> </TD>
<TD width="100%"> </TD> </TR >
<TR>
<TD style="WIDTH: 16%"><STRONG>Teaser:</STRONG></TD > <TD style="WIDTH: 80%">
<asp:TextBox id=tbTeaser runat="server" Width="100%" TextMode="MultiLine" Rows="4">
</asp:TextBox>
</TD>
<TD> </TD>
</TR >
<TR>
<TD style="WIDTH: 16%"><STRONG>Body:</STRONG></TD >
<TD style="WIDTH: 80%">
<asp:TextBox id=tbBody runat=" server" Width="100%" TextMode="MultiLine" Rows="16" >
</asp:TextBox> </TD>
<TD>
<asp:RequiredFieldValidator id=rfvBody runat="server" ErrorMessage="A body must be entered." Display="Dynamic"
ControlToValidate="tbBody" EnableClientScript="False">*
</asp:RequiredFieldValidator>
</TD>
</TR > <TR>
<TD style="WIDTH: 16%"><STRONG>Tagline:</STRONG></TD> <TD style="WIDTH: 80%">
<asp:TextBox id=tbTagline runat="server" Width="100%" TextMode="MultiLine">
</asp:TextBox>
</TD>
<TD> </TD>
</TR > <TR>
<TD style="WIDTH: 16%"><STRONG>Modified Date: </STRONG></TD > <TD style="WIDTH: 80%">
<asp:Label id=lbModifiedDate runat="server" Width="100%"></asp:Label>
</TD>
<TD> </TD>
</TR > <TR>
<TD style="WIDTH: 16%"><STRONG>Creation Date: </STRONG></TD > <TD style="WIDTH: 80%">
<asp:Label id=lbCreationDate runat="server" Width="100%"></asp:Label>
</TD>
<TD> </TD>
</TR > </TABLE>
</P>
<asp:Button id=bnReturn runat="server" Text="Return" ></asp:Button>
<asp:Button id=bnInsert runat="server" Text="Update with new version" > </asp:Button>
<asp:Button id=bnUpdate runat="server" Text="Update this version" > </asp:Button>

<asp:Button id=bnRestore runat="server" Text="Restore" ></asp:Button>
<asp:Button id=bnRemove runat="server" Text="Remove" ></asp:Button>
</form>
The AutUpdate Codebehind
The only thing special about the AutUpdate Web form is that it supports rudimentary versioning. For CMS.NET, versioning is simply saving the updated Web form into a new database record with a new version number.
Most of the code for AutUpdate class (see Listing 11-13) is pretty standard update logic:
Listing 11-13: The AutUpdate Web Page Codebehind
§Get the record to update.
§Populate the update table.
§Display the Web page for updating.
§When a valid form is returned, save content to the database.
private void BuildOrigPage()
{
DataRow dr = dt.Rows[0];
lbContentID.Text = dr["ContentID"].ToString();
lbVersion.Text = dr["Version"].ToString();
tbHeadline.Text = dr["Headline"].ToString();
lbByline.Text |
= dr["Byline"].ToString(); |
|
|
|
|
|
|
tbTeaser.Text |
= dr["Teaser"].ToString(); |
||
|
|
||
tbBody.Text |
= dr["Body"].ToString(); |
|
|
|
|
||
tbTagline.Text |
= dr["Tagline"].ToString(); |
lbModifiedDate.Text = dr["ModifiedDate"].ToString();
lbCreationDate.Text = dr["CreationDate"].ToString();
}
private void Page_Load(object sender, System.EventArgs e)
{

int cid = Convert.ToInt32(Request.QueryString["ContentID"]);
if (cid == 0)
{
Response.Redirect("error.aspx?ErrMsg=" +
HttpUtility.UrlEncode("ContentID Missing"));
}
dt = new Content(
new AppEnv(Context).GetConnection()).GetContentForID(cid);
if (!IsPostBack)
{
BuildOrigPage();
}
}
...
private void bnRestore_Click(object sender, System.EventArgs e)
{
BuildOrigPage();
}
private void bnReturn_Click(object sender, System.EventArgs e)
{
Response.Redirect("AutList.aspx");
}
private void bnRemove_Click(object sender, System.EventArgs e)
{
Response.Redirect("AutRemove.aspx?ContentID=" + lbContentID.Text);
}

private void bnInsert_Click(object sender, System.EventArgs e)
{
if (Page.IsValid)
{
try
{
Content content = new Content(new AppEnv(Context).GetConnection()); content.Insert(Convert.ToInt32(lbContentID.Text),
Convert.ToInt32(lbVersion.Text) + 1, tbHeadline.Text, 1, tbTeaser.Text, tbBody.Text, tbTagline.Text, 1);
}
catch (Exception err)
{
Response.Redirect("Error.aspx?ErrMsg=" + HttpUtility.UrlEncode("The following error occurred: "+ err.Message));
}
Response.Redirect("AutList.aspx");
}
}
private void bnUpdate_Click(object sender, System.EventArgs e)
{
if (Page.IsValid)
{
try
{
Content content = new Content(new AppEnv(Context).GetConnection());

content.Update(Convert.ToInt32(lbContentID.Text),
Convert.ToInt32(lbVersion.Text),
tbHeadline.Text, 1, tbTeaser.Text, tbBody.Text,
tbTagline.Text, 1);
}
catch (Exception err)
{
Response.Redirect("Error.aspx?ErrMsg=" +
HttpUtility.UrlEncode("The following error occurred: "+
err.Message));
}
Response.Redirect("AutList.aspx");
}
}
The loading of the Web table is only allowed if the ID of the content is passed to AutUpdate in the ContentID parameter. If ContentID is empty, the Web page jumps to error.aspx. The loading of the Web form table from the database is done using the Content helper class GetContentForID() method (see Listing 11-14).
Listing 11-14: The Update and GetContentForID Content Database Helper Methods
public void Update(int ContentID, int Version, string Headline, int Byline, string Teaser, string Body, string Tagline, int UpdUserID)
{
//UPDATE Content
//SET
// |
Headline |
= @Headline, |
|||
|
|
|
|
|
|
// |
Byline |
= @Byline, |
|
|
|
|
|
|
|
||
// |
Teaser |
= @Teaser, |
|
||
|
|
|
|||
// |
Body |
= @Body, |
|

// |
Tagline |
= @Tagline, |
|
|
|
|
|
// |
Status |
= @Status, |
|
//UpdateUserID = @UpdateUserID,
//ModifiedDate = @ModifiedDate
//WHERE ContentID = @ContentID
//AND Version = @Version
SqlCommand Command = new SqlCommand("Content_Update", m_Connection); Command.CommandType = CommandType.StoredProcedure;
Command.Parameters.Add(new SqlParameter("@ContentID", SqlDbType.Int));
Command.Parameters.Add(new SqlParameter("@Version", SqlDbType.Int));
Command.Parameters.Add(new SqlParameter("@Headline", SqlDbType.Text));
Command.Parameters.Add(new SqlParameter("@Byline", SqlDbType.Int));
Command.Parameters.Add(new SqlParameter("@Teaser", SqlDbType.Text));
Command.Parameters.Add(new SqlParameter("@Body", SqlDbType.Text));
Command.Parameters.Add(new SqlParameter("@Tagline", SqlDbType.Text));
Command.Parameters.Add(new SqlParameter("@Status", SqlDbType.Int));
Command.Parameters.Add(new SqlParameter("@UpdateUserID", SqlDbType.Int));
Command.Parameters.Add(new SqlParameter("@ModifiedDate",
SqlDbType.DateTime));
Command.Parameters["@ContentID"].Value |
= ContentID; |
|
|||||
|
|
|
|
|
|
||
Command.Parameters["@Version"].Value |
= Version; |
|
|
||||
|
|
|
|
|
|||
Command.Parameters["@Headline"].Value |
= Headline; |
|
|||||
|
|
|
|
||||
Command.Parameters["@Byline"].Value |
= Byline; |
|
|
||||
|
|
|
|
||||
Command.Parameters["@Teaser"].Value |
= Teaser; |
|
|||||
|
|
|
|||||
Command.Parameters["@Body"].Value |
= Body; |
|
|
||||
|
|
||||||
Command.Parameters["@Tagline"].Value |
= Tagline; |
|
|||||
|
|
||||||
Command.Parameters["@Status"].Value |
= StatusCodes.Creating; |
Command.Parameters["@UpdateUserID"].Value = UpdUserID;
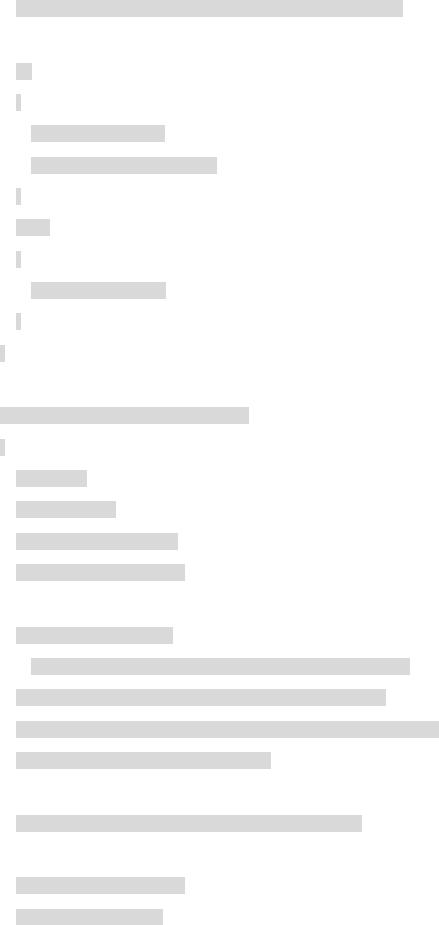
Command.Parameters["@ModifiedDate"].Value = DateTime.Now;
try
{
m_Connection.Open();
Command.ExecuteNonQuery();
}
finally
{
m_Connection.Close();
}
}
public DataTable GetContentForID(int cid)
{
//SELECT *
//FROM Content
//WHERE ContentID=@cid
//ORDER BY Version DESC
SqlCommand Command =
new SqlCommand("Content_GetContentForID", m_Connection); Command.CommandType = CommandType.StoredProcedure; Command.Parameters.Add(new SqlParameter("@cid", SqlDbType.Int)); Command.Parameters["@cid"].Value = cid;
SqlDataAdapter DAdpt = new SqlDataAdapter(Command);
DataSet ds = new DataSet();
DAdpt.Fill(ds, "Content");

return ds.Tables["Content"];
}
Three of the buttons need a quick look over.
The Restore button, handled by the bnRestore_Click() method, is used to restore the Web page back to its original version before any edits. A simple trick is used to do this. The button simply reloads the Web page from the database.
The Update With New Version button, handled by the bnInsert_Click() method, calls the Content database help classes' Insert() method, which was covered earlier. The helper method used this time is the Insert() method with all the parameters. To figure out what the next version is, simply add one to the current version. This is possible because only the last version, the one with the highest version number, can be updated. The Update This Version button, handled by the bnUpdate_Click() method, calls a standard update database helper method (see Listing 11-14). You've already seen a near carbon copy of this updater code before (and you will see it again).
Viewing a Piece of Content
The view form is a little terse because it only shows the content's headline, so you have to come up with another way of viewing a piece of content. Viewing content using the update function is a little risky because it runs the risk of changing the content by accident. So, to view the entire piece of content, I added the AutView.aspx Web page.
The AutView Web Page
As you can see in Figure 11-10, the Web page is just a table of all the content columns for a particular piece of content. It also has five buttons at the bottom. The first button returns to the content list. The next two buttons navigate through all the versions of the content. The next button updates the content, and the last button removes the current version.
Figure 11-10: The AutView Web page