
Visual CSharp .NET Developer's Handbook (2002) [eng]
.pdf// Fill the labels with data. lblName.Text =
dataSet11.Tables[0].Rows[CurrRec].ItemArray.GetValue(1).ToString();
lblRating.Text = dataSet11.Tables[0].Rows[CurrRec].ItemArray.GetValue(2).ToString();
lblDescription.Text = dataSet11.Tables[0].Rows[CurrRec].ItemArray.GetValue(3).ToString();
lblActor.Text = dataSet11.Tables[0].Rows[CurrRec].ItemArray.GetValue(4).ToString();
lblActress.Text = dataSet11.Tables[0].Rows[CurrRec].ItemArray.GetValue(5).ToString();
}
private void btnFirst_Click(object sender, System.EventArgs e)
{
//Go to the first record. CurrRec = 0;
//Set the current record label. lblCurrentRecord.Text = CurrRec.ToString();
//Fill the display with data.
DoFill();
}
private void btnPrevious_Click(object sender, System.EventArgs e)
{
//Validate the current record pointer and change if necessary. if (CurrRec > 0)
CurrRec--;
//Set the current record label.
lblCurrentRecord.Text = CurrRec.ToString();
// Fill the display with data. DoFill();
}
private void btnNext_Click(object sender, System.EventArgs e)
{
//Validate the current record pointer and change if necessary. if (CurrRec < dataSet11.Tables[0].Rows.Count - 1)
CurrRec++;
//Set the current record label.
lblCurrentRecord.Text = CurrRec.ToString();
// Fill the display with data. DoFill();
}
private void btnLast_Click(object sender, System.EventArgs e)
{
// Go to the last record.
CurrRec = dataSet11.Tables[0].Rows.Count - 1;
// Set the current record label.

lblCurrentRecord.Text = CurrRec.ToString();
// Fill the display with data. DoFill();
}
The code begins in the usual manner by filling a dataset, dataSet11, with information from the data adapter, sqlDataAdapter1. In this respect, it doesn't matter what type of ADO.NET application you want to create, you still need to create the necessary links. Given the way that MMIT applications run, however, you'll want to ensure the application makes maximum use of data pooling by setting it up correctly in IIS.
As you can see from Listing 17.1, data movement is approximately the same as in any database application. A current record variable, CurrRec, tracks the current record position. Of course, there's one major difference: MMIT applications, like ASP.NET applications, lack any type of state storage information. Therefore, the application relies on a hidden label to store the current record position so that clicking Next actually displays the next record.
Testing
Unlike an ASP.NET application, you won't see multiple pages when working with an MMIT application, so getting the forms in the right order is essential. Testing means checking the form order as well as the application execution. The first form you should see when you begin this application is the one that asks the user to click the button. Figure 17.6 shows the output of this form.
Figure 17.6: The first form is the one that asks for user input, so that's the first form you should see.
Note You might wonder how IIS keeps track of the various mobile sessions. Unlike ASP.NET, MMIT uses a special cookie embedded directly into the URL for the website after the first use of the URL. For example, here's the URL that resulted on my first visit to the example website from using the Cassiopeia:
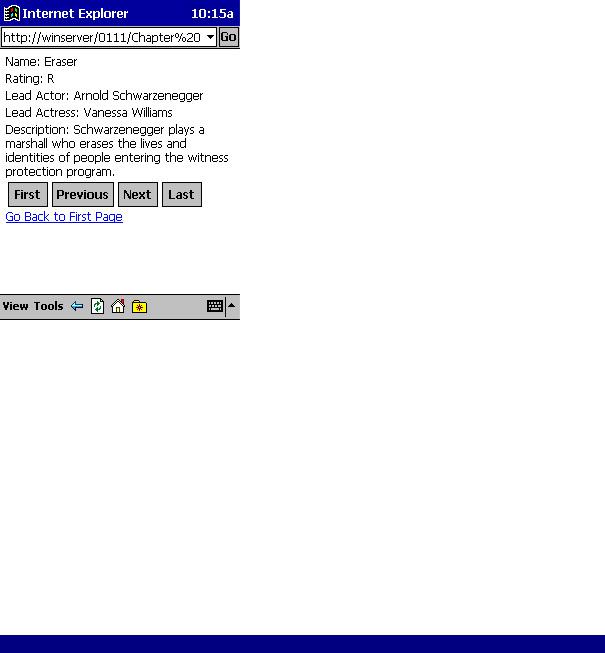
http://winserver/0111/Chapter%2017/MobileWebApp/(1tsrsbnjrs0fct45ck0jxm55)/Default.aspx. As you can see, everything is the same as on the first visit, except for the addition of a cookie (in parentheses).
Clicking the link displays the second page shown in Figure 17.7. Notice that the page includes a link for getting back to the first page. Because mobile users will look at a multitude of screens, you should always provide some means of getting back to the base page for a series of screens. Note that the four record positioning buttons are fully functional, so you can move from record to record using a PDA or other mobile device.
Figure 17.7: The database screen provides a summary of the movies found in the MovieGuide database.
Writing a SOAP PDA Application
The SOAP PDA example relies on the same CompName component we used in Chapter 16. The big difference is in the client for this example. Microsoft doesn't provide a client yet for the PDA, so we need to rely on the third-party client found in the "Getting SOAP for Your PDA" sidebar.
The client code for this section is somewhat complex compared to other SOAP examples in the book, because we have to use a third-party toolkit. For one thing, we need to service two buttons instead of one. However, the Microsoft SOAP Toolkit also requires slightly different input than pocketSOAP, so there are differences in the message formatting code as well.
Listing 17.2 shows the client source code for this example.
Listing 17.2: pocketSOAP Helps You Create PDA SOAP Applications
<HTML>
<HEAD>
<TITLE>CompName JScript Example</TITLE>
<SCRIPT LANGUAGE="JScript"> function cmdGetSingleName_Click()
{
var SOAPEnv; |
// SOAP envelope |
var Transport; |
// SOAP transport |
var Param; |
// Parameter list |
var SOAPParam; |
// SOAP method call parameters. |
var RecData; |
// Received data holder |
// Create the envelope.
SOAPEnv = new ActiveXObject("pocketSOAP.Envelope"); SOAPEnv.MethodName = "GetCompName";
SOAPEnv.URI = "http://tempuri.org/message/";
//Create a parameter to place within the envelope. Param = SOAPEnv.CreateParameter("NameType",
window.document.SampleForm1.comboName.value, "");
//Send the request and receive the data.
Transport = new ActiveXObject("pocketSOAP.HTTPTransport"); Transport.SOAPAction =
"http://tempuri.org/action/NameValuesProc.GetCompName"
Transport.Send(
"http://WinServer/soapexamples/ComputerName/CompNameProc.WSDL",
SOAPEnv.Serialize()); RecData = Transport.Receive(); SOAPEnv.Parse(RecData);
// Display the result.
RecData = SOAPEnv.Parameters.Item(0); window.document.SampleForm1.Results.value = RecData.Value;
}
function cmdGetAllNames_Click()
{ |
// SOAP envelope |
var SOAPEnv; |
|
var Transport; |
// SOAP transport |
var Param; |
// Parameter list |
var SOAPParam; |
// SOAP method call parameters. |
var RecData; |
// Received data holder |
// Create the envelope.
SOAPEnv = new ActiveXObject("pocketSOAP.Envelope"); SOAPEnv.MethodName = "GetAllNames";
SOAPEnv.URI = "http://tempuri.org/message/";
//Create a parameter to place within the envelope. Param = SOAPEnv.CreateParameter("NameType",
window.document.SampleForm1.comboName.value, "");
//Send the request and receive the data.
Transport = new ActiveXObject("pocketSOAP.HTTPTransport"); Transport.SOAPAction =
"http://tempuri.org/action/NameValuesProc.GetAllNames"
Transport.Send(
"http://WinServer/soapexamples/ComputerName/CompNameProc.WSDL",
SOAPEnv.Serialize()); RecData = Transport.Receive(); SOAPEnv.Parse(RecData);
// Display the result.
RecData = SOAPEnv.Parameters.Item(0); window.document.SampleForm1.Results.value = RecData.Value;

}
</SCRIPT>
</HEAD>
<BODY>
<!Add a heading->
<CENTER><H1>Computer Name Component Test</H1></CENTER>
<!Use a form to test out the VBScript.-> <FORM NAME="SampleForm1">
<!Create a text entry control.->
Select a single computer name if needed:<BR>
<select name=comboName>
<option value=0>ComputerNameNetBIOS <option value=1>ComputerNameDnsHostname <option value=2>ComputerNameDnsDomain
<option value=3>ComputerNameDnsFullyQualified <option value=4>ComputerNamePhysicalNetBIOS <option value=5>ComputerNamePhysicalDnsHostname <option value=6>ComputerNamePhysicalDnsDomain
<option value=7>ComputerNamePhysicalDnsFullyQualified <option value=8>ComputerNameMax
</select><P><P>
<!Define a place to put the results.-> Result values: <BR>
<TEXTAREA ROWS=11 COLS=60 NAME="Results"> </TEXTAREA><P><P>
<!-Create the two required buttons.-> <INPUT TYPE=button
NAME=cmdGetSingleName
VALUE="Get Single Name" ONCLICK=cmdGetSingleName_Click()>
<INPUT TYPE=button NAME=cmdGetAllNames VALUE="Get All Names"
ONCLICK=cmdGetAllNames_Click()>
</FORM>
</BODY>
</HTML>
This example uses a five-step process for each function. First, you create an envelope. Second, you place data within the envelope. Third, you need to initialize the data. Fourth, you send and receive the data. Finally, you display the data on screen. The HTML code is difficult to optimize for a small screen, in this case, because of the amount of data we need to display. We'll see later how you can overcome these problems without modifying the server-side component.
The Computer Name example doesn't work quite the same on a PDA as it does on the desktop. The reason is simple—a PDA lacks the screen real estate to display this application fully. Part of the testing process is to ensure the screen remains close to the original (to keep
training costs down) while ensuring readability. Figure 17.1 shows the output from this example if you click Get All Names.
The application does work the same as a desktop application would from a functionality perspective. You still use a drop-down list box to select a computer name type. The buttons still produce the same results as before. Maintaining this level of compatibility between a desktop and PDA application is essential to the success of the application. Any application that requires the user to relearn a business process is doomed to failure, no matter how nice the display looks.
Fortunately, this example is reasonably small. Your testing process must include resource usage and other factors that affect PDA application performance. Complex database applications won't run very well on a PDA because they require too many resources. Of course, you can always get around the problem by using more server-side processing and presenting the PDA with semi-finished, rather than raw results.
Beyond PDAs to Telephones
You won't find any telephones that run distributed applications today, but many developers are experimenting with telephones because they represent a potential not found with other devices. In fact, I was surprised to find that some vendors are already creating distributed applications for embedded devices. Embedded applications reside within toasters, your car, and even your television set. As distributed applications move from the desktop to the PDA, it's only logical that they'll move to the telephone as well.
The interesting thing about telephones is that we already use them for so many business and personal purposes. For example, it's possible to check your bank statement or send correspondence to your credit card company using the telephone. All you need to know how to do, in most cases, is push buttons. In addition, many companies now offer e-mail access and limited website access using a telephone; although the uses for such applications are understandably limited.
Of course, speaking into the telephone or entering some numbers for an account is hardly the same thing as using an application on a desktop computer. Eventually, technologies such as SOAP will enable two-way communications in a way that users haven't seen before. For example, an employee could enter order information using a telephone (assuming the orders are small and relatively simple).
Will telephone distributed applications appear overnight? Probably not. Will we use the telephone to accomplish the same things we do on the PC? Again, probably not. However, the telephone does present some interesting opportunities for application developers in the future.
Understanding PDA Security Issues
Many network administrators view the PDA with more than a little suspicion and for good reason. The media has painted the PDA as a device that it so open that anyone can access it at any time. Smart users keep all of their sensitive data on the company computer and just place lists of tasks on their PDA. Of course, such a view defeats the purpose of having a PDA in the first place. A PDA should be an extension of your workplace, not a hindrance to avoid.
Many of the security issues surrounding PDAs today come from a perception that they're all wireless devices. Many PDAs use network or modem connections, not wireless connections. The PDAs that do provide wireless access tend to err on the side of safety whenever possible. Vendors realize that wireless access is both a blessing and curse for many companies.
However, the wireless issue probably isn't much of an issue for the SOAP developer. Count on many of your users to rely on modem or direct network interface card (NIC) connections. For example, the Pocket PC provides a slot that will accommodate an Ethernet card that provides a direct network connection.
One of the biggest security issues for all PDA users is data storage. There are actually two threats to consider. The first is that someone could access sensitive data if they stole your PDA (or, at least, borrowed it for a while). Wireless or not, the person who has the PDA also has access to the data it contains. The second threat is data loss. Many PDAs lose all of their information once the main battery is exhausted. (Some PDAs provide a backup battery that retains the contents of memory until the user replaces or recharges the main battery.) Unless the user backs up on a regular basis while on the road, the data is lost.
A distributed application can come to the rescue, in this case, by allowing the user to store all data on a central server in a secure location. The application remains on the client machine, but the data remains secure at the main office. Of course, there has to be some level of flexibility built into the security plan, so that users can operate their PDA in a disconnected mode. PDA vendors will likely add some form of biometric protection to their PDAs in the future. For example, the user might have to press their thumb in a certain area in the back when starting the PDA so the PDA can check their identity.
Some security issues involve limitations in the PDA itself. For example, when working with a desktop application, you can ask the user to enter their name and password. The application asks the server for verification before it requests any data access. The pointer a PDA user must use to enter data hampers the security effort. It's possible that they'll end up entering the password more than the three times that good applications normally allow. The next thing you'll hear is a frustrated user on the telephone asking why they can't get into their application. Security is required, but any attempt to implement security that causes user frustration is almost certain to fail.
Another PDA-specific security issue to consider is one of resources. Heavy security consumes many resources. A desktop user notices some slowing of their computer when they're working with a secure application. The slowing is due to the work of the security protocol in the background. With the limited resources of a PDA, you probably can't implement any level of heavy security because the application would run too slowly. The PDA already runs distributed applications slower because it uses a browser-based application over a relatively slow connection. Adding to this problem is one way to lose user confidence in your application.
PDAs do suffer from every other security problem that distributed applications that rely on any form of XML (such as SOAP) normally experience. A lack of built-in security will allow prying eyes to see the data that the user transfers to the home office (at least if someone is actually looking). Unfortunately, the list of available security add-ons for PDAs is extremely limited. Theoretically, you can use technologies such as Secure Sockets Layer (SSL) with a PDA. We've already discussed the limitations of some of these technologies in previous
chapters, so I won't go into them again here. Needless to say, using a PDA involves some security risk that you'll need to overcome.
Troubleshooting PDA Application Problems
PDAs present some interesting challenges no matter which programming language you use to develop a client and no matter how you transfer data. Fortunately, most distributed application environments allow you to preserve the server side of your development investment. If you use a PDA such as the Pocket PC, you can also reuse some of your client code. In short, distributed application development solves significant PDA development problems. The following sections will examine some of the problems developers have when creating browser-based distributed applications for the PDA and hopefully provide the answers you need to add distributed application development to your PDA application toolkit. (Always feel free to contact me at JMueller@mwt.net if you have additional questions.)
Missing Screen Data or No Display at All
The number one problem for PDAs running applications from a web server is security. The PDA will often run into an access problem and simply ignore it. You won't see any kind of an error message in many cases. All that will happen is that the PDA will perform whatever local tasks it can perform and then stop when the error happens.
You'll also see this problem if you use a scripting language the PDA doesn't understand. Again, the PDA will try to accomplish all that it can locally, and then it'll simply stop. Remember that Windows CE doesn't provide VBScript support, but it does provide JScript support. Other PDA setups have similar limitations, and you need to check for them during the project definition stage.
Some developers ignore the small screen that a PDA provides; you may not see a result because it appears off screen. Moving the scroll bar up and down may show an answer that you couldn't see before. Unfortunately, users will normally complain about the lack of data long before they move the display. In short, make sure that your application fills a single screen at a time so the user doesn't have to look for the information.
In a very few cases, you'll find that all of the client files need to appear on the PDA instead of the web server. For example, downloading a web page so that the PDA can access the scripts may not work. Test all of the machines your application targets to ensure you won't run into a problem of this type.
Lack of Support for More Than One PDA
During the development process for the examples in this chapter and a few personal projects, I found that even though a PDA runs the same operating system as another PDA, there are differences for which you need to account. For example, PDAs use different processors, so you can't assume anything about low-level code. Consequently, it's important to use highlevel languages if you plan to work with the code on more than one type of machine.
You'll also find that PDAs require driver and other software updates, just as desktop machines do from time to time. Make sure you download and install all patches for your PDA before you begin development.
Some toolkit implementations (especially those for SOAP) require support files. Make sure you install all of the required support before you attempt to run an application on the PDA. For example, a Windows CE machine will likely require a copy of pocketSOAP (or other SOAP toolkit) before they can run a SOAP application. The Palm systems required both the SOAP toolkit and the XML parser. Palm systems require two separate installations, so leaving one part of the support mechanism out of the picture is relatively easy.
Client Messaging Problems
Most messaging problems appear when you use more than one toolkit to accomplish a task. The server may expect something other than the normal client output. However, you can normally modify the data stream enough to accommodate the requirements of client or server. The various tracing tools allow you to compare message formats and change the standard code as needed to create a message the server will understand. Likewise, you can normally modify the server output to accommodate client needs.
Another problem is live connection confusion. A PDA requires a live connection with the server, not a synchronized connection. A live connection allows data exchange between client and server, while a synchronized connection allows the host workstation to update the PDA's files. In some cases, a synchronized connection may look live, but it isn't. PDAs always require some type of direct network connection to provide data connectivity, plus a driver that allows network configuration. In short, don't assume all wireless connections are live simply because they tap into the network—many simply provide a better way to perform synchronization.
Always double-check a failed PDA connection using a desktop client that you know works. PDA connections can fail in numerous ways that a desktop connection won't. In at least a few cases, you'll find that the server has stopped responding and the desktop application will provide an error message to this effect. PDAs often suppress error messages, making debugging especially difficult.
Where Do You Go From Here?
By now, you have a better idea of how PDA development will shape the world of distributed applications. While PDA applications currently perform mundane tasks such as reading e- mail, they'll eventually provide the basis for distributed applications. For example, a salesperson might use a PDA to enter client contact information that appears instantly on the company database and helps the company deliver products faster. In some cases, PDAs could represent the new laptop device—a laptop that fits in your pocket.
We've looked at a simple PDA example in this chapter. However, this example forms the basis of most of the code that you'll eventually write for distributed PDA applications. While many PDA applications today are of the utility type used to enhance PDA functionality, you'll eventually want to write applications that exchange data with a remote server. Enhancing the application in this chapter is one way to gain additional insights into PDA development.
Now that you have a better idea of what PDA development is like, you might want additional information about what .NET can do for you. .NET Wireless Programming by Mark Ridgeway (Sybex, 2002, see http://www.sybex.com/sybexbooks.nsf/2604971535a28b098825693
d0053081b/bafc7295f4222914882569fa003710fb!OpenDocument for more information) provides additional insights into this new type of application development. This book includes five case studies that show various types of applications you can create using the .NET Framework.
This is the last chapter of the book. However, the content isn't over yet. You'll find several appendices after this chapter that help you learn more about how C# compares to the rest of the world. You'll also find an interesting example of how to build an MMC snap-in using a combination of C# and Visual C++. Finally, make sure you check the Glossary when you have questions about terms and acronyms in the book.
Part V: Appendices
Chapter List
Appendix A: C# for Visual C++ Developers
Appendix B: C# for Visual Basic Developers
Appendix C: Accessing C Library Routines
Appendix A: C# for Visual C++ Developers
Overview
C# promises much for Visual C++ developers who need the low-level support that Visual C++ provides, but also need to become more productive. Some people have said that C# is Microsoft's answer to Java. In some respects, it probably is. Other people have pointed to C# as the mid-point between Visual Basic and Visual C++. This view is also partially true. However, C# is also a new language that has a lot to offer in its own right, so those of you converting from Visual C++ to C# should look at C# as a new language, not as the next step for Visual C++ developers.
This appendix presents information about how you can make your C# experience better if you already know how to use Visual C++. There are some obvious similarities between the two languages that make C# easier for the Visual C++ developer to learn. However, these similarities can become a trap for the unwary developer who assumes that everything will work as it did in the past. C# is a distinct language and you need to consider it as such. While you can't port all of your Visual C++ code to C# (especially considering your Visual C++ code is written for an unmanaged environment) you will be able to port some of it. We'll discuss the issues in porting code in this appendix as well.
An Overview of the Differences
If you take a cursory look at C#, it bears many similarities to Visual C++. In fact, it might be easy to confuse the two in some circumstances, because you use curly braces and other features that people associate with C in general and Visual C++ in specific. The fact is that the cursory glance doesn't tell the whole story. You'll find that while C# and Visual C++ do have some features in common, you'll also see many differences.