
Introduction to 3D Game Programming with DirectX.9.0 - F. D. Luna
.pdf

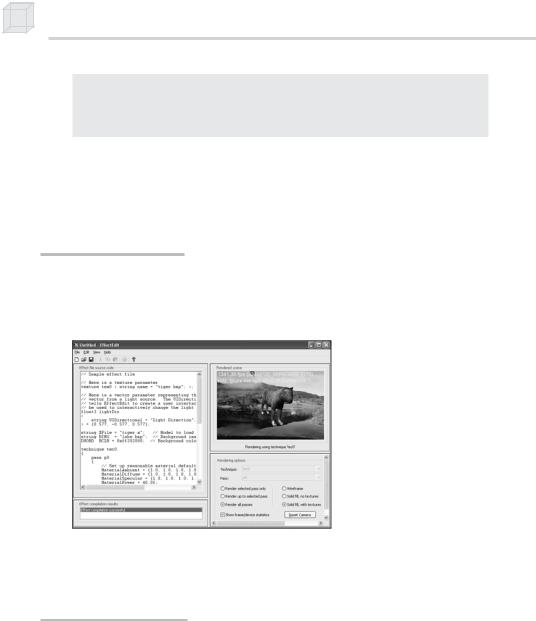
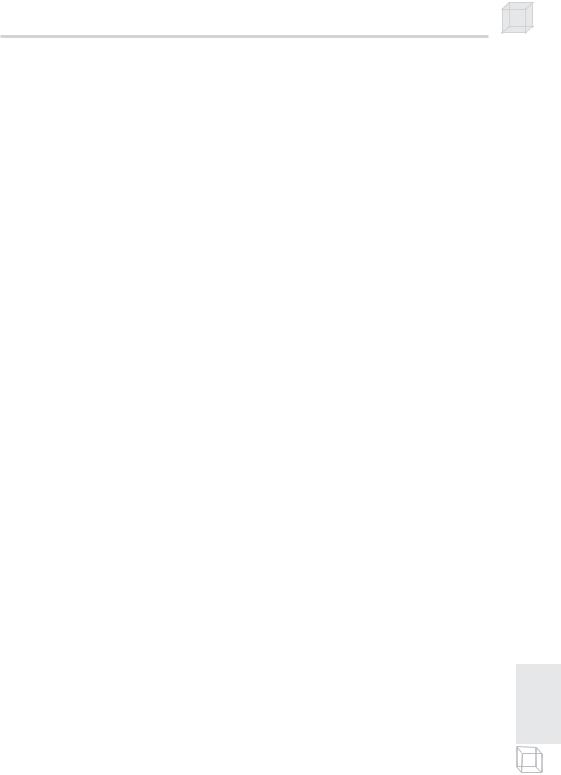
The Effects Framework 357
A technique is a particular implementation of a special effect. Typically, an effect file will consist of several techniques that all implement the same effect but in different ways. Each implementation will utilize the capabilities of a specific generation of hardware. Thus, the application can choose the technique that is most fitting for the target hardware. For example, to implement multitexturing, we might define two techniques—one that uses pixel shaders and one that uses the fixed function pipeline. In this way, users with a pixel shader-capable 3D card can use the pixel shader technique, and users with a 3D card that does not support pixel shaders can still execute the effect in the fixed function version.
A technique consists of one or more rendering passes. A rendering pass consists of the device states and shaders used to render the geometry for that particular pass. Multiple rendering passes are necessary because some special effects require the same geometry to be rendered several times, each time with different device states and/or shaders.
P a r t I V
This page intentionally left blank.

Appendix
An Introduction to Windows Programming
To use the Direct3D API (application programming interface), it is necessary to create a Windows (Win32) application with a main window, upon which we render our 3D scenes. This appendix serves as an introduction to writing Windows applications using the native Win32 API. Loosely, the Win32 API is a set of low-level functions and structures exposed to us in the C programming language that enables our application and the Windows operating system (OS) to communicate with each other. For example, to notify Windows to show a particular window, we use the Win32 API function ShowWindow.
Windows programming is a huge subject, and this appendix introduces only what is necessary for us to use Direct3D. For readers interested in learning more about Windows programming with the Win32 API, the book Programming Windows (now in its fifth edition) by Charles Petzold is the standard text on the subject. Another invaluable resource when working with Microsoft technologies is the MSDN library, which is usually included with Microsoft’s Visual Studio but can also be read online at www.msdn.microsoft.com. In general, if you come upon a Win32 function or structure that you would like to know more about, go to MSDN and search for that function or structure. Often in this appendix we direct you to look up a function or structure on MSDN for more elaborate details.
Objectives
To learn and understand the event-driven programming model used in Windows programming
To learn the minimal code necessary to create a Windows application that is necessary to use Direct3D
359
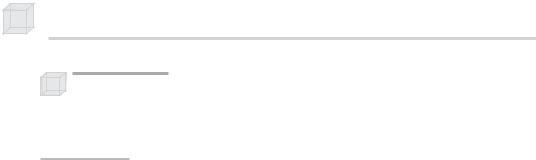

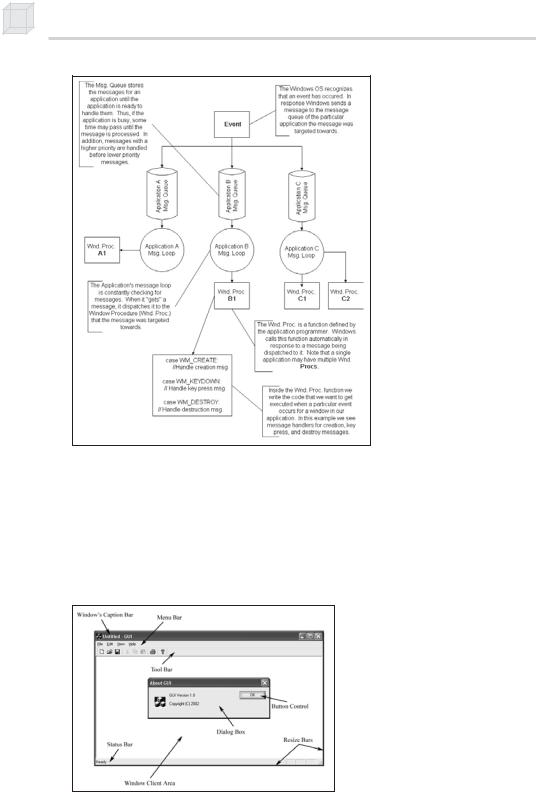
