
Introduction to 3D Game Programming with DirectX.9.0 - F. D. Luna
.pdf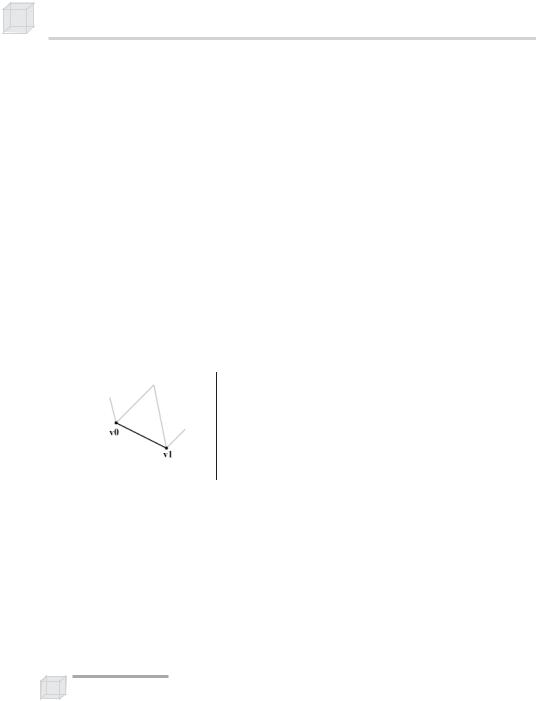

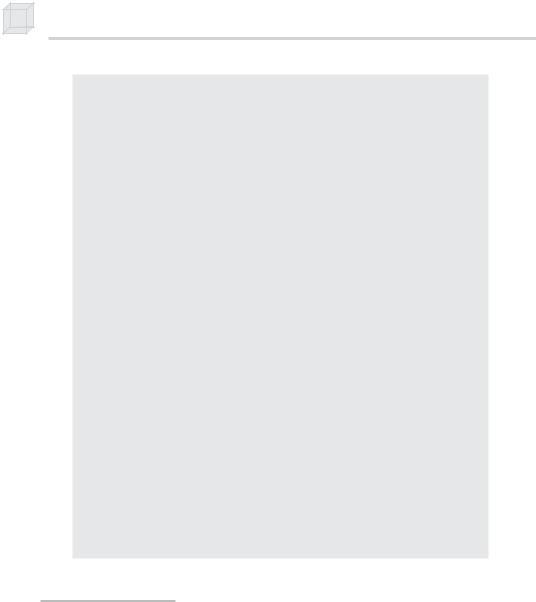

Introduction to Vertex Shaders 317
For input, usage semantics specify how vertex components are mapped from the vertex declaration to variables in the HLSL program. For output, usage semantics specify what a vertex component is going to be used for (e.g., position, color, texture coordinate, etc.).
P a r t I V

Chapter 18
Introduction to Pixel Shaders
A pixel shader is a program executed on the graphics card’s GPU during the rasterization process for each pixel. (Unlike vertex shaders, Direct3D will not emulate pixel shader functionality in software.) It essentially replaces the multitexturing stage of the fixed function pipeline and gives us the ability to manipulate individual pixels directly and access the texture coordinate for each pixel. This direct access to pixels and texture coordinates allows us to achieve a variety of special effects, such as multitexturing, per pixel lighting, depth of field, cloud simulation, fire simulation, and sophisticated shadowing techniques.
You can test the pixel shader version that your graphics card supports by checking the PixelShaderVersion member of the D3DCAPS9 structure and the macro D3DPS_VERSION. The following code snippet illustrates this:
// If the device’s supported version is less than version 2.0 if( caps.PixelShaderVersion < D3DPS_VERSION(2, 0) )
// Then pixel shader version 2.0 is not supported on this device.
Objectives
To obtain a basic understanding of the concepts of multitexturing
To learn how to write, create, and use pixel shaders
To learn how to implement multitexturing using a pixel shader
318

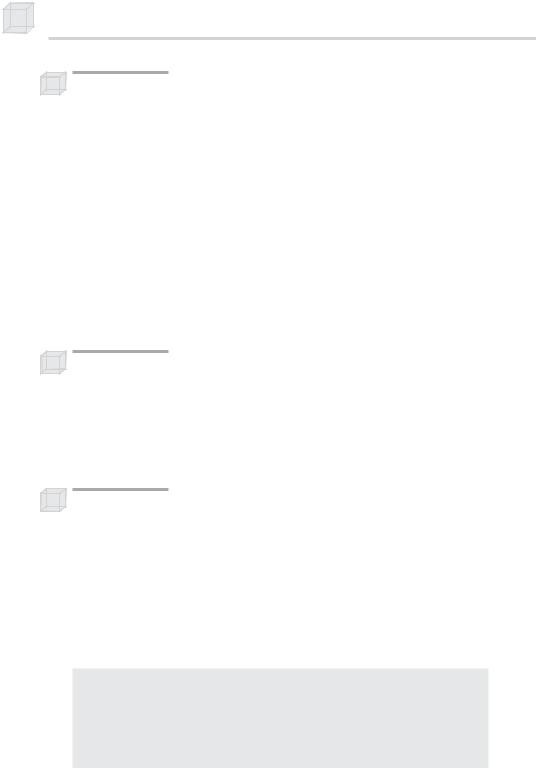


