
Introduction to 3D Game Programming with DirectX.9.0 - F. D. Luna
.pdf

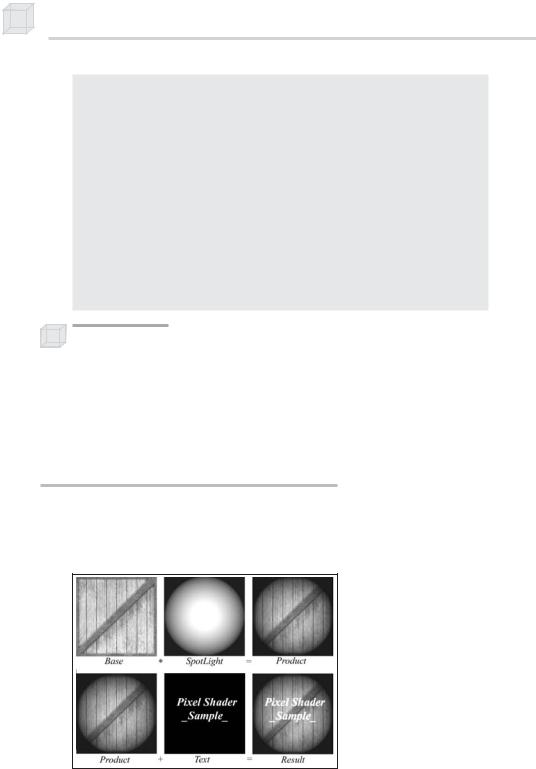
326Chapter 18
//Get a description of the constant: D3DXCONSTANT_DESC FirstTexDesc;
UINT count;
MultiTexCT->GetConstantDesc(FirstTexHandle, &FirstTexDesc, &count);
.
.
.
//Set texture/sampler states for the sampler FirstTex. We identify
//the stage FirstTex is associated with from the
//D3DXCONSTANT_DESC::RegisterIndex member:
Device->SetTexture(FirstTexDesc.RegisterIndex,
Tex);
Device->SetSamplerState(FirstTexDesc.RegisterIndex,
D3DSAMP_MAGFILTER, D3DTEXF_LINEAR);
Device->SetSamplerState(FirstTexDesc.RegisterIndex,
D3DSAMP_MINFILTER, D3DTEXF_LINEAR);
Device->SetSamplerState(FirstTexDesc.RegisterIndex,
D3DSAMP_MIPFILTER, D3DTEXF_LINEAR);
Note: Alternatively, instead of using the sampler type, you can use the more specific and strongly typed sampler1D, sampler2D, sampler3D, and samplerCube types. These types are more type safe and ensure that they are only used with the appropriate tex* functions. For example, a sampler2D object can only be used with tex2D* functions. Similarly, a sampler3D object can only be used with tex3D* functions.
18.5 Sample Application:
Multitexturing in a Pixel Shader
The sample application for this chapter demonstrates multitexturing using a pixel shader. The sample will texture a quad based on the “result” in Figure 18.2 by blending together a crate texture, a spotlight texture, and a texture that contains the string “Pixel Shader Sample.”
Figure 18.2: Combining the textures. Let b, s, and t be the colors of corresponding texels from the crate texture, spotlight texture, and text texture, respectively. We define how these colors are combined as c = b s + t, where denotes compo- nent-wise multiplication.





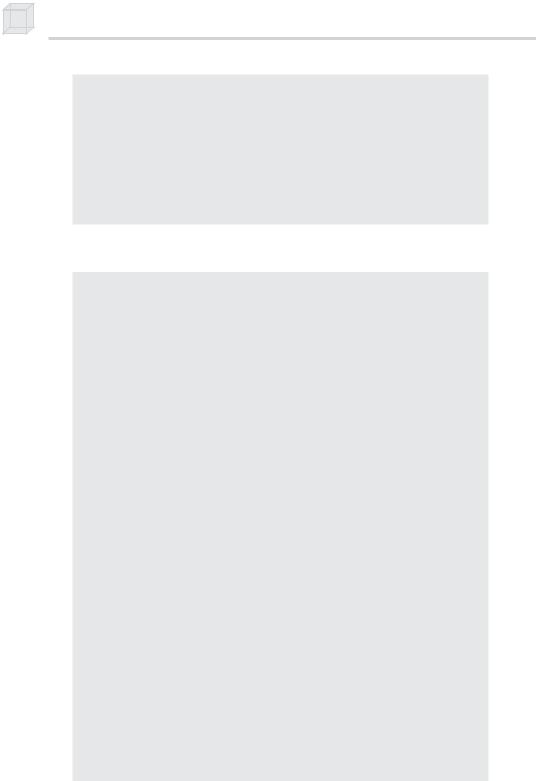

Introduction to Pixel Shaders 333
Device->SetSamplerState(StringTexDesc.RegisterIndex,
D3DSAMP_MINFILTER, D3DTEXF_LINEAR);
Device->SetSamplerState(StringTexDesc.RegisterIndex,
D3DSAMP_MIPFILTER, D3DTEXF_LINEAR);
// draw the quad Device->DrawPrimitive(D3DPT_TRIANGLELIST, 0, 2);
Device->EndScene(); Device->Present(0, 0, 0, 0);
}
return true;
}
Of course we must remember to free our allocated interfaces in the Cleanup function:
void Cleanup()
{
d3d::Release<IDirect3DVertexBuffer9*>(QuadVB);
d3d::Release<IDirect3DTexture9*>(BaseTex);
d3d::Release<IDirect3DTexture9*>(SpotLightTex);
d3d::Release<IDirect3DTexture9*>(StringTex);
d3d::Release<IDirect3DPixelShader9*>(MultiTexPS);
d3d::Release<ID3DXConstantTable*>(MultiTexCT);
}
18.6Summary
Pixel shaders replace the multitexturing stage of the fixed function pipeline. Furthermore, pixel shaders give us the ability to modify pixels on an individual basis in any way that we choose and access texture data, thereby empowering us to implement many special effects that could not be achieved in the fixed function pipeline.
Multitexturing is the process of enabling several textures at once and blending them together to produce a desired result. Multitexturing is typically used to implement a complete lighting engine for static geometry.
The HLSL intrinsic sampler objects identify a particular texture/sampler stage. A sampler object is used to refer to a texture/sampler stage from the pixel shader.
P a r t I V