
Introduction to 3D Game Programming with DirectX.9.0 - F. D. Luna
.pdf

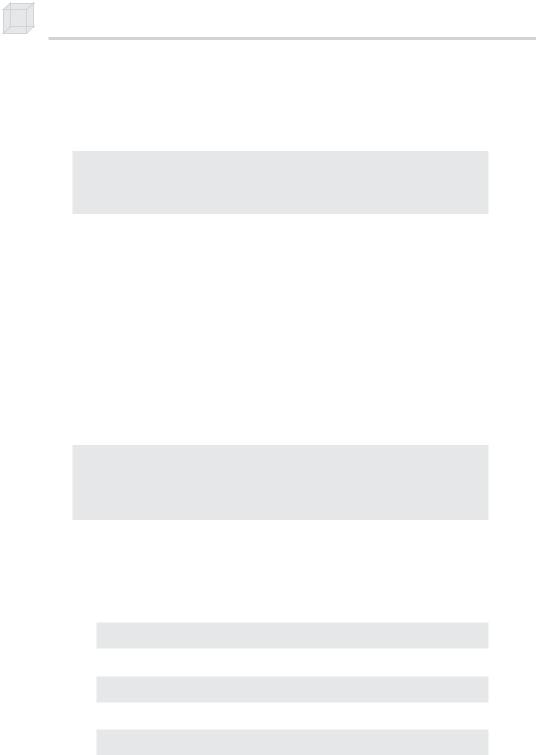


278Chapter 16
SetVector—Used to set a variable of type D3DXVECTOR4. Sample call:
D3DXVECTOR4 v(1.0f, 2.0f, 3.0f, 4.0f);
ConstTable->SetVector(Device, handle, &v);
SetVectorArray—Used to set a variable that is a vector array. Sample call:
D3DXVECTOR4 v[3];
// ...Initialize vectors
ConstTable->SetVectorArray(Device, handle, v, 3);
SetValue—Used to set an arbitrarily sized type, such as a structure. In the sample call, we use SetValue to set a D3DXMATRIX:
D3DXMATRIX M(…);
ConstTable->SetValue(Device, handle, (void*)&M, sizeof(M));
16.2.1.3 Setting the Constant Default Values
This next method simply sets the constants to their default values, which are the values they are initialized with when they are declared. This method should be called once during application setup.
HRESULT ID3DXConstantTable::SetDefaults( LPDIRECT3DDEVICE9 pDevice
);
pDevice—Pointer to the device that is associated with the constant table
16.2.2 Compiling an HLSL Shader
We can compile a shader, which we have saved to a text file, using the following function:
HRESULT D3DXCompileShaderFromFile(
LPCSTR |
pSrcFile, |
CONST D3DXMACRO* |
pDefines, |
LPD3DXINCLUDE |
pInclude, |
LPCSTR |
pFunctionName, |
LPCSTR |
pTarget, |
DWORD |
Flags, |
LPD3DXBUFFER* |
ppShader, |
LPD3DXBUFFER* |
ppErrorMsgs, |
LPD3DXCONSTANTTABLE* ppConstantTable
);

Introduction to the High-Level Shading Language 279
pSrcFile—Name of the text file that contains the shader source code that we want to compile
pDefines—This parameter is optional, and we specify null for it in this book.
pInclude—Pointer to an ID3DXInclude interface. This interface is designed to be implemented by the application so that we can override default include behavior. In general, the default behavior is fine and we can ignore this parameter by specifying null.
pFunctionName—A string specifying the name of the entry point function. For example, if the shader’s entry point function were called Main, we would pass “Main” for this parameter.
pTarget—A string specifying the shader version to compile the HLSL source code to. Valid vertex shader versions are: vs_1_1, vs_2_0, vs_2_sw. Valid pixel shader versions are: ps_1_1, ps_1_2, ps_1_3, ps_1_4, ps_2_0, ps_2_sw. For example, if we wanted to compile our vertex shader to version 2.0, we would pass vs_2_0 for this parameter.
Remark: The ability to compile to different shader versions is one of the major benefits of using HLSL over assembly language. With HLSL we can almost instantly port a shader to a different version by simply recompiling to the desired target. Using assembly, we would have to port the code by hand.
Flags—Optional compiling flags; specify 0 for no flags. Valid options are:
D3DXSHADER_DEBUG—Instructs the compiler to write debug information
D3DXSHADER_SKIPVALIDATION—Instructs the compiler not to do any code validation. This should only be used when you are using a shader that is known to work.
D3DXSHADER_SKIPOPTIMIZATION—Instructs the compiler |
|
not to perform any code optimization. In practice this would |
|
only be used in debugging, where you would not want the com- |
|
piler to alter the code in any way. |
|
|
|
ppShader—Returns a pointer to an ID3DXBuffer that contains |
V |
the compiled shader code. This compiled shader code is then used |
t I |
as a parameter to another function to actually create the ver- |
ar |
tex/pixel shader. |
P |
|
|
ppErrorMsgs—Returns a pointer to an ID3DXBuffer that con- |
|
tains a string of error codes and messages |
|

280Chapter 16
ppConstantTable—Returns a pointer to an ID3DXConstantTable that contains the constant table data for this shader
Here is an example call of D3DXCompileShaderFromFile:
//
// Compile shader
//
ID3DXConstantTable* TransformConstantTable = 0;
ID3DXBuffer* shader = 0;
ID3DXBuffer* errorBuffer = 0;
hr = D3DXCompileShaderFromFile(
"transform.txt", |
// shader filename |
0, |
|
0, |
|
"Main", |
// entry point function name |
"vs_2_0", |
// shader version to compile to |
D3DXSHADER_DEBUG, |
// debug compile |
&shader, |
|
&errorBuffer, |
|
&TransformConstantTable);
// output any error messages if( errorBuffer )
{
::MessageBox(0, (char*)errorBuffer->GetBufferPointer(), 0, 0); d3d::Release<ID3DXBuffer*>(errorBuffer);
}
if(FAILED(hr))
{
::MessageBox(0, "D3DXCreateEffectFromFile() - FAILED", 0, 0); return false;
}
16.3 Variable Types
Note: In addition to the types that are described in the following sections, HLSL also has some built-in object types (e.g., texture object). However, since these object types are primarily used only in the effects framework, we defer a discussion of them until Chapter 19.
16.3.1 Scalar Types
HLSL supports the following scalar types:
bool—True or false value. Note that HLSL provides the true and false keywords.
int—32-bit signed integer
half—16-bit floating-point number

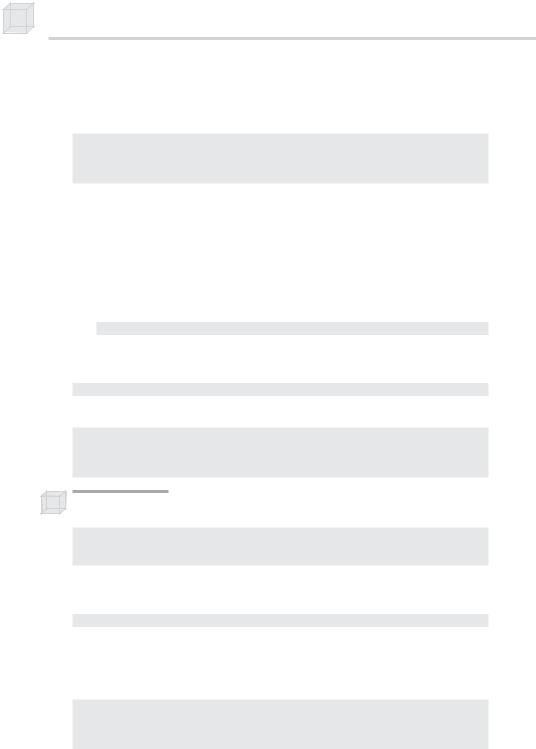
