
C# ПІДРУЧНИКИ / c# / Premier Press - C# Professional Projects
.pdf
8Part I INTRODUCTION TO C#
Y
L
FIGURE 1-1 Language interoperability featureFof the .NET Framework
By now, you know thatEinteroperabilityMprovided by CTS is a desired feature of all programmers. Following are some of the benefits of the interoperability offered by CTS. T
A
Inherit a class from a class you created in another language.
Create an object of a class created in another language. You can also access the methods of the object that you have created.
Pass an object or a reference of an object as a parameter to the methods of the class that you have written in another language.
Debug an application that contains the objects of classes written in different languages. The debugger of the .NET Framework also enables you to switch between source code of the applications you have written in different .NET languages.
Common Language Specification (CLS)
In addition to CTS, another feature that ensures language interoperability in the
.NET Framework is CLS. CLS is defined as a set of rules that a .NET language should follow to allow you to create applications that are interoperable with other languages. However, to achieve interoperability across languages, you can only use objects with features listed in the CLS. These features are called CLS-compliant features.
For example, C# supports uint32, which is a 32-bit unsigned integer data type that is not CLS-compliant. The data type is not supported by Visual Basic
Team-Fly®

OVERVIEW OF THE .NET FRAMEWORK |
Chapter 1 |
9 |
|
|
|
.NET. If you use the uint32 data type in a C# class, the class might not be interoperable with Visual Basic .NET applications.Only a CLS-compliant code is fully interoperable across languages. Although you can use the non-CLS feature in a
.NET language class, the class might not be available to other .NET languages.
CLS works closely with CTS and MSIL to ensure language interoperability. You can also call CLS as a subset of CTS and MSIL, because CLS does not include all the features of CTS and MSIL. A compiler might not support some of the features of CTS and MSIL and still be CLS-compliant. Following are some of the features of CLS:
Global methods and variables are not allowed in a CLS-compliant language.
Some data types, such as unsigned data types, are not allowed in a CLScompliant language.
Unique names should be used in a CLS-compliant language. Even if the language is case-sensitive, you must use distinct names for different variables. Languages that are case-insensitive should be able to differentiate between the names.
Any exception that you want to handle must be derived from the base
class Exception.
Pointers are not supported by a CLS-compliant language.
Garbage Collector
Consider a situation in which you write extensive code for an application, such as that for an airline reservation system. In the application, you define a large number of variables of different data types, but you do not remove the variables from the system memory. In such a case, variables that are not required occupy memory space, resulting in reduced application performance to the extent that the application might even stop running.
The garbage collection feature of CLR enables automatic management of system memory. If a variable is not referenced for a long period of time by any application, the garbage collector automatically releases the memory assigned to the variable. This memory clean-up process ensures that there is no unnecessary wastage of system memory and, therefore, prevents memory leakage from the application.
In .NET, the CLR handles the process of garbage collection. To understand the garbage collection system, first look at the mechanism of allocating memory to an
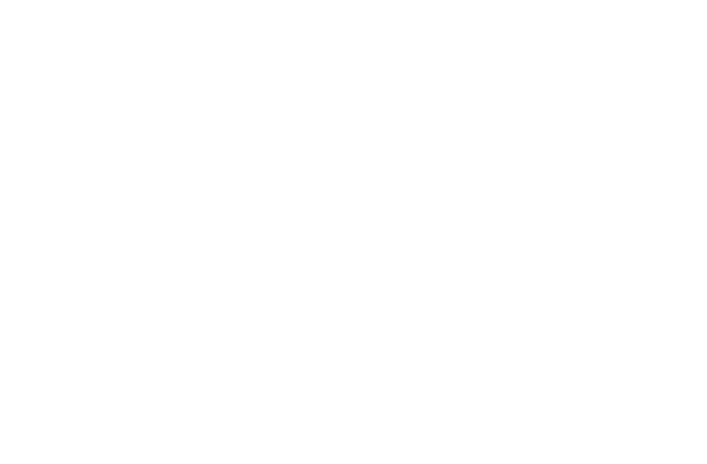
10 |
Part I |
INTRODUCTION TO C# |
|
|
|
application. When you create an application, some memory space is allocated to it.Therefore, all the variables, classes, objects,and other resources that you declare in the application are added to this memory space.This process is called heap allocation to an application. When you go on adding data to this heap, there comes a time when the memory allocated to your application becomes full and you cannot add more data to it. This is the time when the garbage collector becomes active.
The garbage collector scans the entire heap and deallocates memory to resources that are no longer in use, thereby creating free spaces in the heap. You can now add more objects to the memory.
Class Library
As discussed earlier, .NET provides you with several base classes. These base classes are available as a library of classes called the .NET base class library, which is an API similar to MFCs (Microsoft Foundation Classes) used with Visual C++ 6.0.
In addition to the base classes, the class library includes interfaces, value types, enumerations, and methods that allow you to perform a wide variety of tasks to make programming easier. Further, the classes contained in the .NET class library have a user-friendly name. This helps you to easily identify the classes that you need to use in your program. For example, if you need to create a thread, you use the Thread class. Similarly, to create an exception, you use the Exception class. You will learn about these classes later in this chapter.
A class library provides classes that help you create interoperable applications. This implies that the classes defined in the class library can be used to create a Visual C++ .NET, Visual Basic .NET, or C# application. In addition, the methods defined in the classes can also be used by any other .NET programming language.
Assembly
An assembly is a logical structure that contains complied code for the .NET Framework. An assembly can be stored in one file or multiple files and can be .dll or .exe files. You may also include files using COM objects, resource files, or metadata in an assembly. As discussed earlier, metadata stores information about managed code in .NET. Similarly, metadata in an assembly contains information about the assembly. Therefore, assemblies are self-describing.

OVERVIEW OF THE .NET FRAMEWORK |
Chapter 1 |
11 |
|
|
|
Before developing applications for the .NET platform, programmers used to create applications by using DLLs. Assemblies offer you several advantages over .dll files. Assemblies contain types, resources, and metadata. Whatever resources you might require for your application, you can simply include them in the assembly. For example, you may include the namespaces containing the classes that you require for your application. This makes developing an application easier for you.
In addition, while working with assemblies, you need not worry about registering your assembly and managing and versioning of your application. Registering your application with the operating system is as simple as copying assembly files to your application directory.
Another important advantage of using assemblies is that they can be either shared or private. The following section will discuss shared and private assemblies in detail.
Private Assembly
As the name suggests, a private assembly is available only to the application for which you create it. When you create a private assembly, you need to provide the assembly along with the executable application. You create a private assembly when you do not need the assembly for another application. For example, if you create an assembly for a skills inventory system of the employees of an organization, you might not require the assembly for another application, such as the hardware inventory system of the organization.
Private assemblies offer you several advantages, such as:
Registering the assembly. You need not register your assembly. To use a private assembly, you only need to copy it to the directory or subdirectory of your application.
Securing the application. A private assembly makes your application safe to use because no other application can access the resources of the private assembly. This implies that no other application can make changes to the private assembly, giving the application full control over the assembly. You do not require security permissions for a private assembly, as these permissions are contained in the application’s directory.

12Part I INTRODUCTION TO C#
Applying naming conventions to the resources. Because the resources in the private assembly are only accessible to your application, you need not worry about the naming convention of these resources. Even if two namespaces in different private assemblies have the same name, it does not affect the performance of any of the application.
Shared Assembly
Consider a situation in which you need to create an application for different processes of the HR system, such as payroll generation, leave processing, and employee appraisal system. All these processes need to use the Employee class. In such a scenario, it is preferable to reuse the same class in all the listed systems instead of creating a class for each application. Therefore, you can create an assembly that you can use in multiple applications. Such an assembly is called a shared assembly. You can also use a shared assembly in all .NET languages if the assembly is created according to the CLS standards discussed earlier.
Multiple applications use shared assemblies; therefore, the assemblies cannot be stored with a specific application. Shared assemblies are stored in the assembly cache, which is a special directory in the file system. To store a shared assembly in the assembly cache, you can use .NET utilities, such as Gacutil.exe and Regasm.exe.
Working with shared assemblies is not as simple as working with private assemblies. Because the resources in the shared assembly can be accessed across applications, you need to be careful with the versioning and naming convention of these resources. Shared assemblies are given a strong name, which is a unique name that applications need to specify to access the shared assembly. However, versioning problems can be solved by accessing the resources with the correct version number.
The following features make assembly an important component of .NET applications:
Self-describing
Side-by-side
Version dependency
Application domain
Zero-impact installation
These features are discussed in detail in the following subsections.

OVERVIEW OF THE .NET FRAMEWORK |
Chapter 1 |
|
13 |
|
|||
|
|
|
|
Self-Describing
An assembly is self-describing because it consists of metadata that stores information about the assembly, such as the data type of the variables and the methods declared in the assembly. This implies that you need not register an assembly with the registry in the operating system.
Side-by-Side
The side-by-side feature of an assembly enables you to install multiple versions of the same assembly in an application. Consider a situation in which you need to work with an application for the airline reservation system. The airline company needs to coordinate with different locations of the airline worldwide.
In this case, you need to create and refer to the two versions of the assembly at a time.The side-by-side feature of an assembly enables you to use both versions of the assembly in the same application without resulting in any conflict in the application.
Version Dependency
An assembly manifest is used to maintain versions of the resources in an assembly. The manifest is a part of the assembly that contains metadata. When you refer an assembly from an application, the version of the referenced assembly is stored in the manifest of the application. This enables you to identify the version number of a referenced assembly that you have used during application development, thus taking care of the versioning problems of the assembly.
Application Domain
The application domain feature of an assembly enables you to execute multiple applications that are independent of each other. These applications are executed as a part of the same process. Because each application is independent of the other, any error in one application does not affect other applications that are a part of the same process.
Zero-Impact Installation
As discussed earlier, to install an assembly, you do not need to register the assembly with the operating system. You can simply use copy or xcopy commands to install an assembly. This is called the zero-impact installation feature of assemblies.

14 |
Part I |
INTRODUCTION TO C# |
|
|
|
Versioning
A well-known fact about the software industry is the regular change in the requirements of users. In such a dynamic scenario, new and improved versions of applications need to be developed. At numerous instances, when you upgrade an application, it may result in errors in the existing application. This can happen because the component that you upgrade might not be compatible with the earlier versions of the application. Problems in maintaining versions may also lead to problems in maintaining and debugging an application. An assembly includes features such as side-by-side and version dependency, which enable you to install multiple versions of the same assembly simultaneously.
NOTE
Every version of an assembly has the following four parts:
<Application name> <Major version>.<Minor version>.<Build>.<Revision>
Here, Major version is the main version number of the application, Minor version is a part of the version of the application, and Build and Revision numbers are generally based on the system date. If you specify “*” in place of Build and Revision, they are automatically generated based on the system date. Build is the number of days since 01/01/00, and Revision is the number of seconds since midnight, based on the system time.
For example, if the version name of an application is ABC 1.2.90.2670, then ABC is the application name. The number 1 refers to the Major version, 2 is the Minor version, and 90 is the Build. The Build value of 90 refers to the 90th day after 01/01/00, and 2670 is the number of seconds since the midnight of the 90th day after 01/01/00.
You looked at the components of the .NET Framework, class library being one of them. Now look at some of the .NET base classes contained in the class library.
An Overview of .NET Framework
Base Classes
As discussed earlier, .NET provides you with several predefined base classes in the
.NET class library. These classes contain methods that help you to create appli-

OVERVIEW OF THE .NET FRAMEWORK |
Chapter 1 |
|
15 |
|
|||
|
|
|
|
cations easily. Working with these classes is simple because of their user-friendly names.
The .NET class library consists of numerous base classes. However, in this chapter, you will be introduced to some of the most frequently used classes. You will learn more about these classes in the subsequent chapters.
Exceptions
Just as in C++, the .NET Framework is designed to handle exceptions. An exception is the erroneous execution of an application that results in an unpredicted output. When an exception is generated in a .NET application, an object of the Exception class, a .NET base class, is thrown. This object contains information about the error that is generated and the way that the .NET Framework handles the error. For example, the object might contain information about the message to be displayed when the compiler encounters an error or the details of the area within the code where the error was detected.
You can handle exceptions in the .NET Framework by using try{}, catch{}, and finally{} statements.These statements are similar to the statements that you use in C++ for handling exceptions.
Threads
A thread is single executable sequence of code. It is good practice to execute different sections of the application code, which are independent and parallel to each other. You can execute the code in sections by creating threads for each section and executing them simultaneously. For example, until an application prints data on a printer, you can use another thread to read from a file.This process is known as multithreading. To use the concept of multithreading in your application, you need to derive your class from the Thread class, which is another .NET base class.
Delegates
Delegate is yet another type of special class that consists only of method definitions. Delegates are objects that allow you to pass methods as parameters to another method. Just as with a class, you need to instantiate a delegate. The instance of the delegate is created from the Delegate class of the .NET base class library.

16 |
Part I |
INTRODUCTION TO C# |
|
|
|
In C#, events are special types of delegates that are assigned to trap an event. Any activity performed by a system is known as an event. For example, when you press a key or move the mouse pointer, an event is generated. To create an event-driven application, you need to trap the events generated by the system and perform the necessary action. For example, you need to trap the action following a key press or a click of a mouse. To do this, C# provides you with the Event delegate.
Summary
In this chapter, you were introduced to the .NET Framework.The .NET Framework is a new API provided by Microsoft to help programmers develop applications for the distributed environment. In addition, .NET enables you to write applications for the Windows platform. Next, you learned about some of the components of the .NET Framework that make it a user-friendly development environment. These components include the CLR and the .NET class library.
The CLR is the run-time environment for the code written in .NET languages. The CLR includes CLS and CTS, which help you to achieve interoperability of the applications created for the .NET Framework. CTS is a set of guidelines and standard data types that you can use to create user-defined classes and objects for the .NET Framework. CLS is defined as a set of rules that a .NET language should follow to create applications that are interoperable with other languages.
In this chapter, you also learned about a class library. Visual Studio .NET is a development environment that provides you with several base classes containing methods. These base classes are contained in a library of classes called the .NET base class library, which is an API. Finally, you learned about some of the base classes in the class library, such as Exception, Thread, and Delegate.

Chapter 2
C# Basics