
C# ПІДРУЧНИКИ / c# / Premier Press - C# Professional Projects
.pdf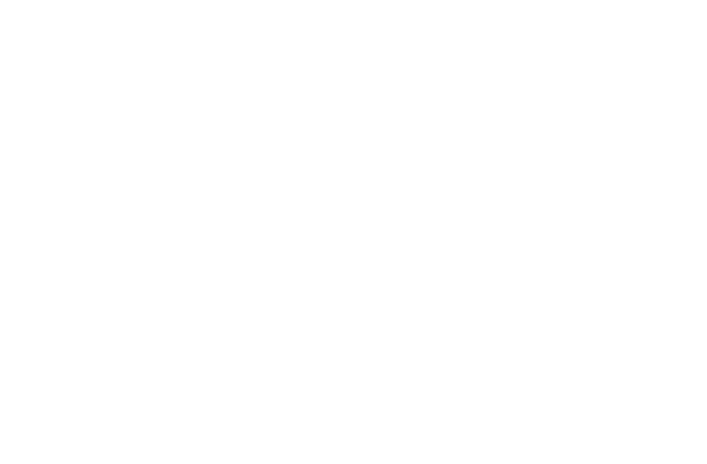
68 |
Part II |
HANDLING DATA |
|
|
|
In Chapter 3, “Components of C#,” you learned about some of the components of C#, such as classes, namespaces, and interfaces. In Chapter 4, you will learn about other components provided by C#. These components include arrays, collections, and indexers. This chapter will also cover data conversion by using box-
ing and unboxing. Finally, you will look at the preprocessor directives in C#. |
|||
Arrays |
|
|
Y |
|
L |
||
|
|
F |
|
We introduced the concept of arrays in Chapter 2, “C# Basics,” in the section |
|||
“Arrays.”This section will look at arrays in detail. An array is a data structure used |
|||
|
|
M |
|
to store a number of variables and has one or more indices attached to it. Based |
|||
on the number of indicesAassociated with arrays, arrays are classified as single- |
|||
dimensional arrays and multidimensional arrays. |
|||
|
E |
|
|
|
T |
|
|
Single-Dimensional Arrays
A single-dimensional ar ray has an index attached to its elements. You can initialize a single-dimensional array as follows:
<data type> [] <array1> = new <data type> [size];
Here, data type is the type of data stored in the array, and size defines the number of elements in the array.
Multidimensional Arrays
A multidimensional ar ray has more than one index associated with its elements. C# supports two types of multidimensional arrays:
Rectangular array
Orthogonal or jagged array
A rectangular array has an equal number of columns in each row. An array of rank two is called a two-dimensional array. Therefore, a two-dimensional rectangular
Team-Fly®
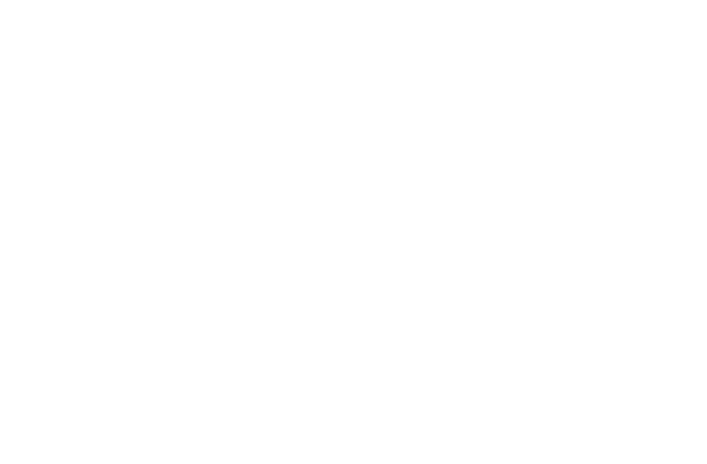
MORE ABOUT COMPONENTS |
Chapter 4 |
|
69 |
|
|||
|
|
|
|
array will have two columns in each row. Look at the following statement that declares a two-dimensional rectangular array of three rows.
int [,] Integer = { {2,3}, {3,4}, {4,5} };
The dimension of an array is not specified while declaring an array. However, the dimension of an array is defined by the number of commas (,) in an array declaration statement. Look at the following example to declare a three-dimensional array with three rows.
int [, ,] Integer = { {1,2,3}, {2,3,4}, {3,4,5} };
In C#, you can initialize an array by using a for loop.
int [,] Integer = new int [5,10]; for (int x = 0; x < 5; x++)
{
for (int y = 0; y < 10; y++) Integer [x,y] = x*y;
}
The previous code creates a two-dimensional array with five rows and 10 columns. The variables x and y denote the number of rows and columns, respectively, in the array Integer. The values of x and y change in a for loop. The elements of the array are initialized by the product of the values of the variables x and y.
As discussed earlier, C# also supports orthogonal or jagged arrays. An orthogonal array can have a different number of columns in each row. Therefore, while declaring a jagged array, you specify only the number of rows in the array. Just as in a rectangular array, you do not use commas to declare an orthogonal array. Instead, the dimension of a jagged array is specified by the number of square brackets ([]).
int [] [] Integer = new int [2] []; Integer [0] = new int [2];
Integer [1] = new int [5];
This code declares a two-dimensional array with two rows.The first row contains two columns and the second row contains five columns.

70 |
Part II |
HANDLING DATA |
After declaring an array, you need to perform operations on the array. To do this, C# provides you with several methods. Some of the commonly used methods in arrays are discussed in the next section.
Methods in Arrays
An array in C# is an object and, therefore, has its own methods. Now look at some of the common methods used with arrays.
The Length property is used to determine the size or number of elements in an array. To find out the number of elements in the one-dimensional array Integer, you use the following statement:
int I = Integer.Length;
In this code, the Length property derives the size of the array Integer, which is then stored in the integer variable I.
Similarly, to determine the size of a multidimensional array, you use the GetLength() method.The GetLength() method returns the number of elements in a specified dimension of a multidimensional array. The dimension of the array is specified as a parameter to the GetLength() method.
int I = Integer.GetLength (1);
Here, the GetLength() method is used to find out the size of the second dimension of the multidimensional array Integer. The number of elements in the second dimension of the array are then stored in the integer variable I.
You can use the Reverse() method to reverse the order of the elements of an array. The Reverse() method is a static method, so the elements of an array that need to be reversed are sent as a parameter to the Reverse() method.
Array.Reverse (Integer);
This code reverses the order of the elements of the array. The name of the array is passed as a parameter to the method.
The elements of an array can be sorted using the Sort() method. Similar to the Reverse() method, the name of the array to be sorted is sent as a parameter to the method Sort(). The Sort() method arranges the elements of an array in ascending order. The elements of the array Integer can be sorted as:

MORE ABOUT COMPONENTS |
Chapter 4 |
|
71 |
|
|||
|
|
|
|
Array.Sort (Integer);
Consider an example of an array that stores the marks of students. This data is sorted to know the maximum and minimum marks obtained by the students.
int [] Marks = {70,62,53,44,75,68}; int I = Marks.Length;
Array.Sort (Marks);
for (int x = 0; x < I; x++)
{
Console.WriteLine (x);
}
This code initializes an integer array with the values as specified in the program code.The code then calculates the size of the one-dimensional array Integer and stores its value in the variable I. The size of the array is determined using the Length property. The Sort() method is then used to sort the elements of Integer. The sorted elements are displayed in the Console window by using the Line() method of the Console class.
The output of the previous code is:
44,53,62,68,70,75
In this section, you learned about arrays. An array is a special type of collection in C#. The next section will look at collections in C#.
Collections
A collection is defined as a group of objects that you can access using the foreach loop. For example, look at the following code:
foreach (string str1 in collection1)
{
Console.WriteLine (str1):
}
In this code, collection1 is a collection, and the foreach loop is used to access
objects of collection1.

72 |
Part II |
HANDLING DATA |
|
|
|
Creating Collections
All collections in C# are implemented by the System.Collections.IEnumerable interface. You have learned about interfaces in Chapter 3 in the section “Interfaces.” Interfaces are components used to declare a set of methods that are never implemented. There are several predefined interfaces provided by C#. One of these predefined interfaces is the IEnumerable interface that has a GetEnumerator() method. This method returns an object of the type enumerator. Therefore, every collection has one or more enumerator objects associated with it. These objects are used to access data from the associated collection. You can use the enumerator object only to read data from a collection, not to modify the collection.
To access the elements of a collection, you create an object that implements the IEnumerable interface. To initialize this object, the MoveNext() method is called. This method is used to move across the elements of the collection. When the MoveNext() method is called for the first time, it moves the enumerator object to the first element of the collection.
Once the enumerator object is initialized with the first element of the collection, you can then move across the elements of the collection by calling the MoveNext() method. The value referred by the enumerator object can be read by the Current property. This property returns only a reference to the elements of the collection. Therefore, to get the actual value of the element, you can type cast the reference to the type of the element. To find out more about collections, consider the following code sample.
public interface IEnumerable
{
IEnumerator GetEnumerator ();
}
public interface IEnumerator
{
bool MoveNext(); object Current
{
get;
}
void Reset();
}
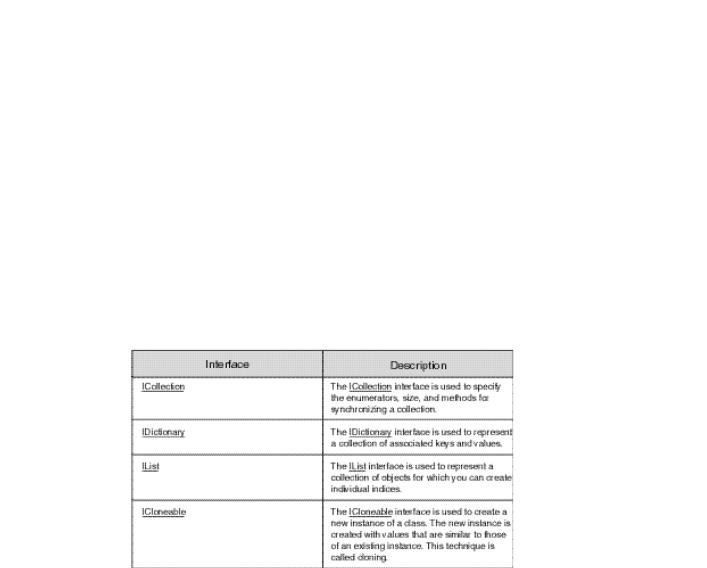
MORE ABOUT COMPONENTS |
Chapter 4 |
73 |
|
|
|
This code declares the GetEnumerator() method of the IEnumerable interface. Next, the MoveNext() method of the IEnumerator interface, which returns a Boolean type variable, is called.The Current property of the type object is used to read the current element of the collection. You use the get property to read the elements of a collection. You can use the Reset() method to reset the value of the
enumerator object.
Working with Collections
After creating a collection, you can work with it. To do this, you can use the interfaces provided by C#. Figure 4-1 lists some of the interfaces that you can use to work with collections.
FIGURE 4-1 Interfaces used with collections
Each of these interfaces is present in the System.Collections namespace. These interfaces have several classes and methods associated with them. The ArrayList class will be discussed in detail.
ArrayList is an important class present in the System.Collections namespace that you can use to create a dynamically increasing array. The ArrayList class implements the IList interface. When you create an object of the ArrayList class, C#
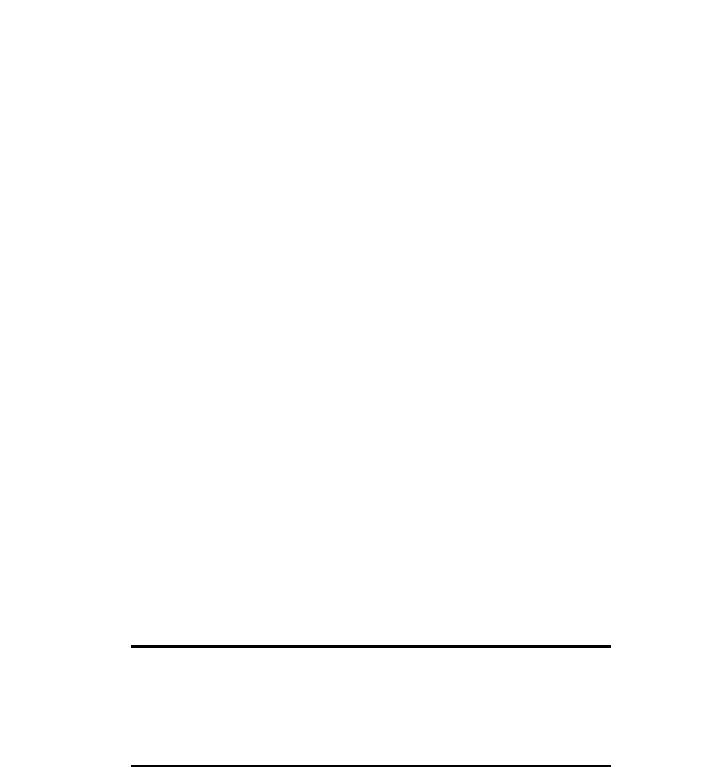
74 |
Part II |
HANDLING DATA |
|
|
|
allocates memory to this object. You can specify the initial size of the ArrayList object while creating the instance of the ArrayList class by using the new keyword. You can then add elements to this object. However, if you add more elements to the ArrayList than its capacity (the number of elements that an object of ArrayList can hold), C# automatically allocates more memory to the ArrayList object. Consider the following example to learn about the ArrayList class.
using System;
using System.Collections; public class ArrayList1
{
public static void Main()
{
ArrayList list1 = new ArrayList(); list1.Add(“This”); list1.Add(“is”);
list1.Add(“a”); list1.Add(“sample”); list1.Add(“ArrayList.”);
}
}
This code creates an object of the ArrayList class with the name list1 and then adds elements to this object by using the Add() method.
Some of the methods present in the interfaces used with collections are discussed in the following list.
ICollection Interface:
CopyTo(). The CopyTo() method is used to copy the elements of the ICollection interface to a specified array. You can also specify the starting index from which you want to copy the elements.
IDictionary Interface:
Add(). The Add() method is used to add an element to the IDictionary interface. You can specify the key and value of the element that is added.
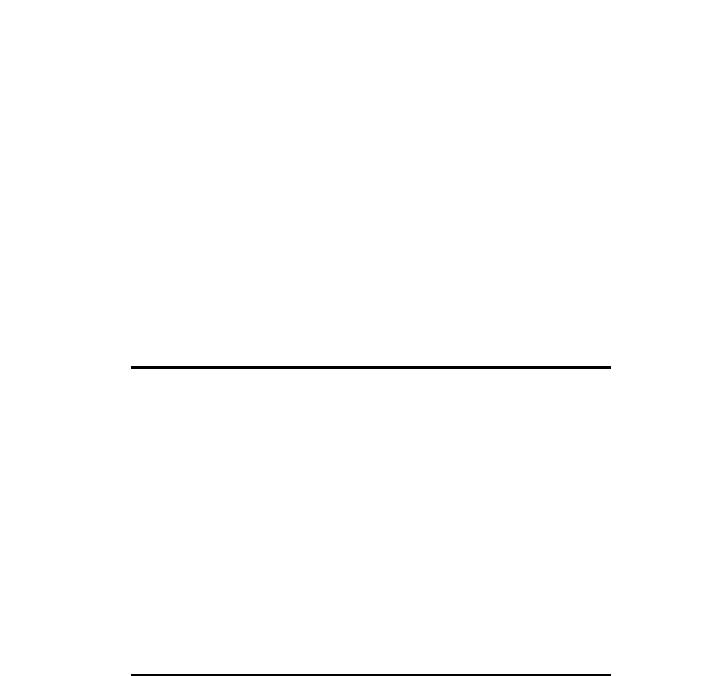
MORE ABOUT COMPONENTS |
Chapter 4 |
75 |
|
|
|
Remove(). The Remove() method is used to delete an element from the IDictionary interface. You need to specify the key of the element to be deleted.
Clear(). The Clear() method is used to delete all the elements from the IDictionary interface.
GetEnumerator(). The GetEnumerator() method is used to return an IDictionaryEnumerator object for the IDictionary interface.
Contains(). The Contains() method is used to locate a particular element in the IDictionary interface. You need to specify the key of the element to be located.
IList Interface:
Add(). The Add() method of the IList interface is used to add elements to the IList interface.
Remove(). The Remove() method is used to delete the first occurrence of the object from the IList interface.
RemoveAt(). The RemoveAt() method is used to delete the element present at the index value that you specify.
Clear(). The Clear() method is used to delete all the elements from the IList interface.
Insert(). The Insert() method is used to insert an element at the specified index in the IList interface.
IndexOf(). The IndexOf() method is used to find the index value of the specified element.
ICloneable Interface:
Clone(). The Clone() method is used to create clones of an existing instance of a class.
Having learned about arrays and collections, you need to learn about indexers. Indexers are members that allow you to access objects as if they were the elements of an array.
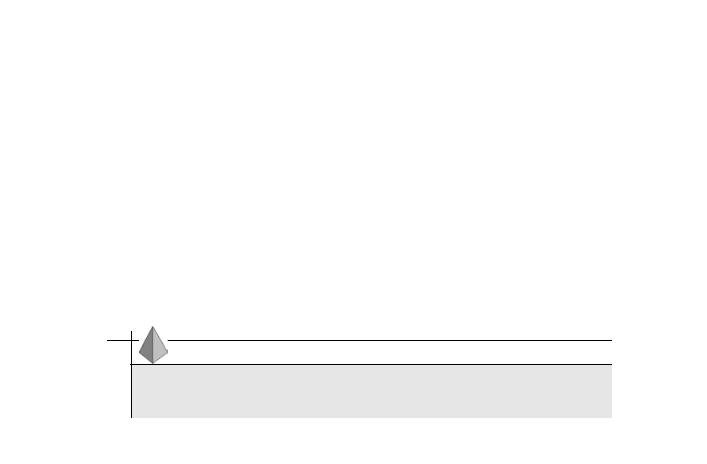
76 |
Part II |
HANDLING DATA |
|
|
|
Indexers
There may be instances where you need to access the elements of a class as an array. You can do this by using indexers provided by C#. To be able to use indexers in classes, you first need to declare an indexer. Indexers are declared as follows:
<modifier> <type> this [parameter-list]
Here, modifier is the indexer modifier and type defines the return type of the indexer. The this keyword is used as a name of the indexer. Indexers do not have an explicit name. The parameter-list in the square brackets defines the data type of the object that has the elements to be accessed.
TIP
In the indexer declaration statement, you can specify any data type as the index of the elements to be accessed.
For example, consider the following sample code:
public int this [int x]
This code declares a public indexer with the return type as integer. Here, the data type of the object is of the integer type.
C# allows you to define both read-only and write-only indexers. To read and write data to an indexer, you use the get and set properties, respectively. The get and set properties do not take any parameter. However, the get property returns the elements of the type as specified in the indexer declaration statement. The set property is used to assign values to indexer elements.Consider the following code:
class Class1
{
int variable1, variable2;
public int this [int x]
set
{
switch (x)
{

MORE ABOUT COMPONENTS |
Chapter 4 |
77 |
|
|
|
case 0:
variable1 = 10;
break; case 1:
variable2 = 20;
break;
}
}
get
{
switch (x)
{
case 0:
return variable1;
case 1:
return variable2;
}
}
}
In this code, the switch statements are used to read and write data to the indexer.
In this section, you learned about arrays, collections, and indexers that are used to store and access variables and objects. However, you also need to learn about type casting variables into objects and vice versa. To do this, C# provides you with the techniques of boxing and unboxing.
Boxing and Unboxing
Boxing is a data type conversion technique that is used to implicitly convert a value type to either an object type or a reference type. When you convert a value type to an object type, C# creates an instance of the object type and then copies the value type to that instance.
Consider the following example of an implicit data conversion by using boxing.
class Class1
{
public static void Main ()