
Beginning ActionScript 2.0 2006
.pdf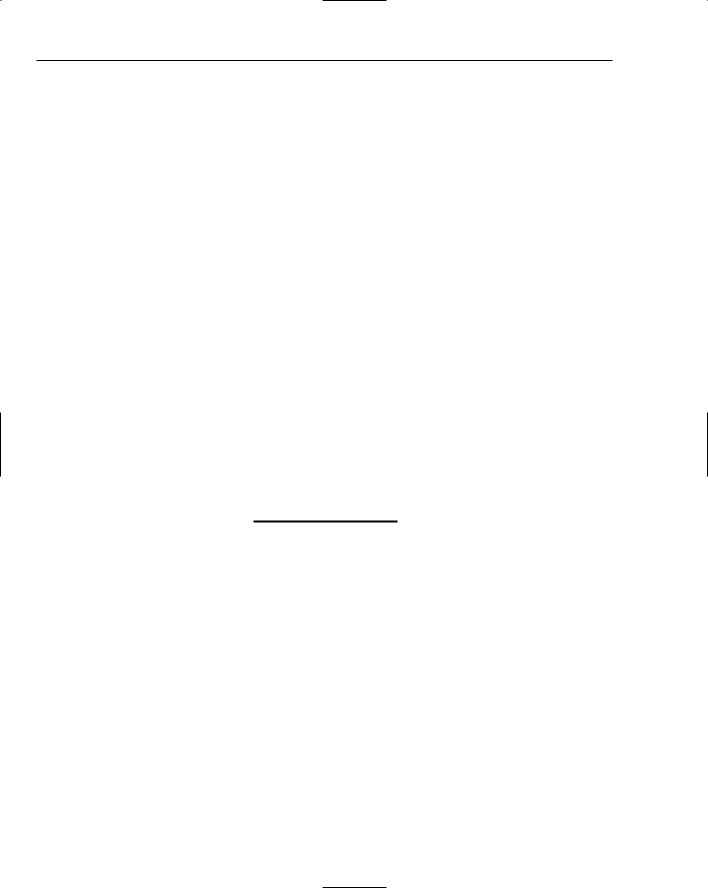


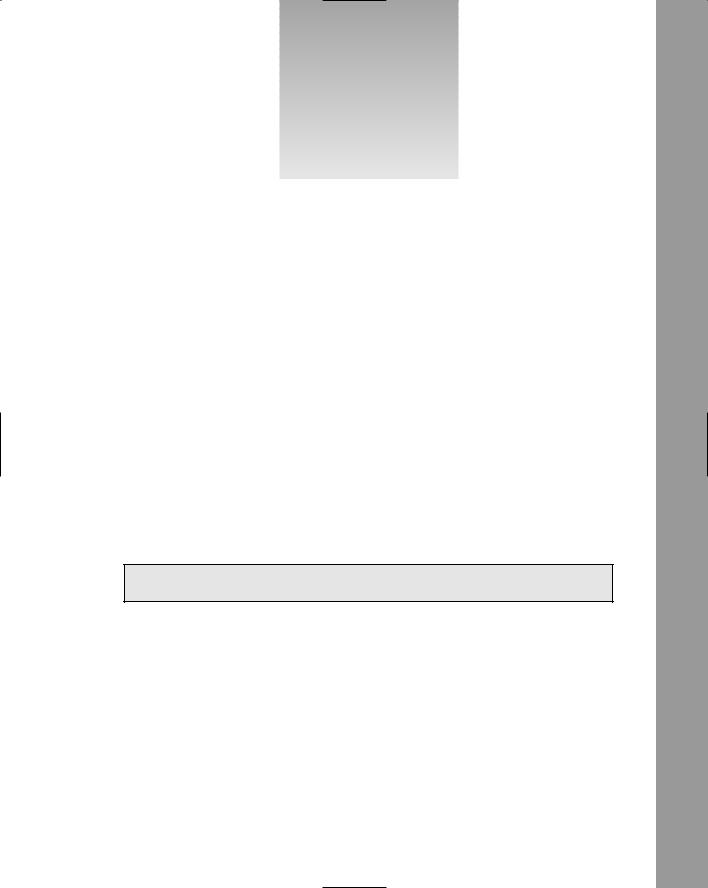
27
Creating Custom Classes
By this point, you have become reasonably familiar with how to code using ActionScript. Hopefully, you have applied your new skills to a personal project or two and are thinking ahead to bigger and better things. In this chapter, you learn some of the next steps to ActionScript coding, specifically the process of creating custom classes. Custom classes are immensely useful for any developer because they take you to the next level of organization and reusability.
Working with Classes
In Chapter 5 you were introduced to a number of object-oriented programming principles. You saw these principles as they apply to the classes built into the Flash player. Although you can achieve many things with just that knowledge, you can do so much more by creating your own custom classes.
Recall the definition of object-oriented programming from Chapter 5:
To package data and the code that acts on that data into a single entity.
The mechanism to make this possible is the class. By working with your own classes, you allow yourself to organize and package your own code in a way that makes re-use significantly easier, and that makes your code so much cleaner. Chapter 5 already went through much of the motivation for object-oriented programming with classes, so this chapter gets right into the mechanics of it.
Defining the Class
The first requirement for a class is that it must exist in its own file, which is named the same as the class itself. If you create a class called MyClass, you would define it in a file called MyClass.as and save it in the same folder as the FLA file that uses it. This enforcement ensures that class files are self-contained and separate from everything else.
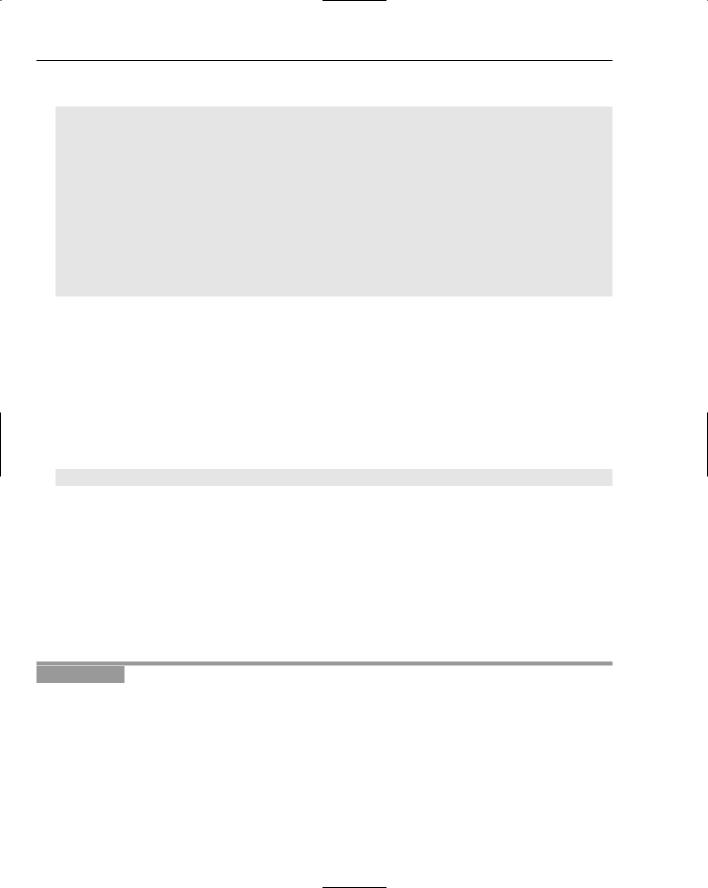

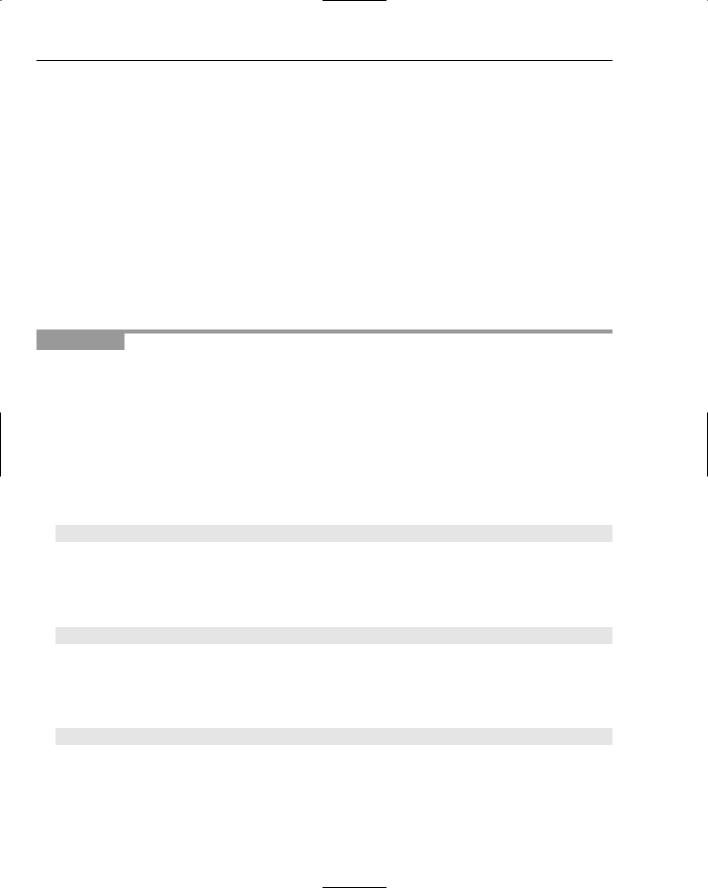
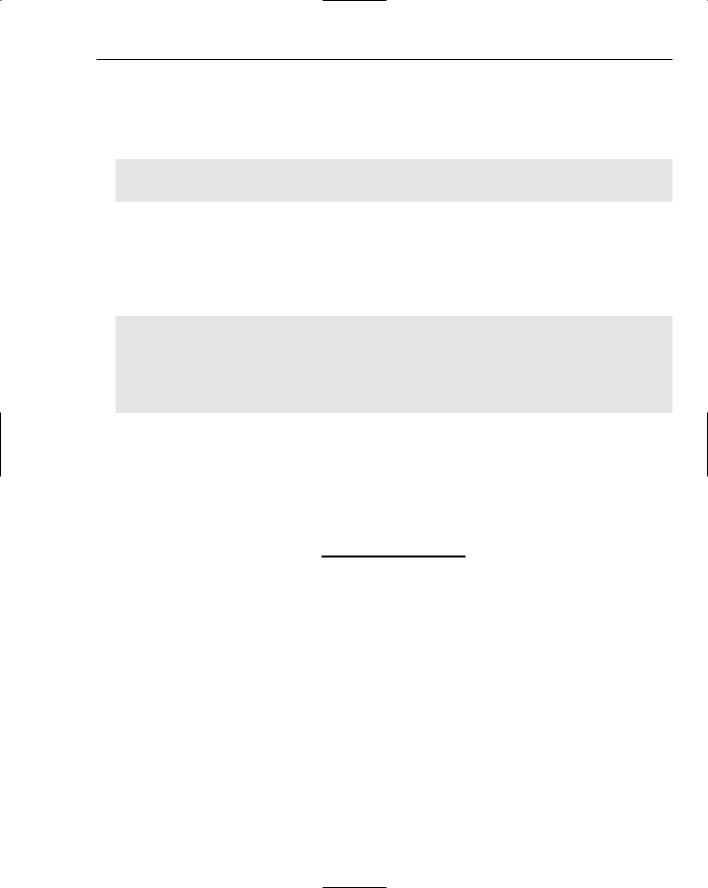
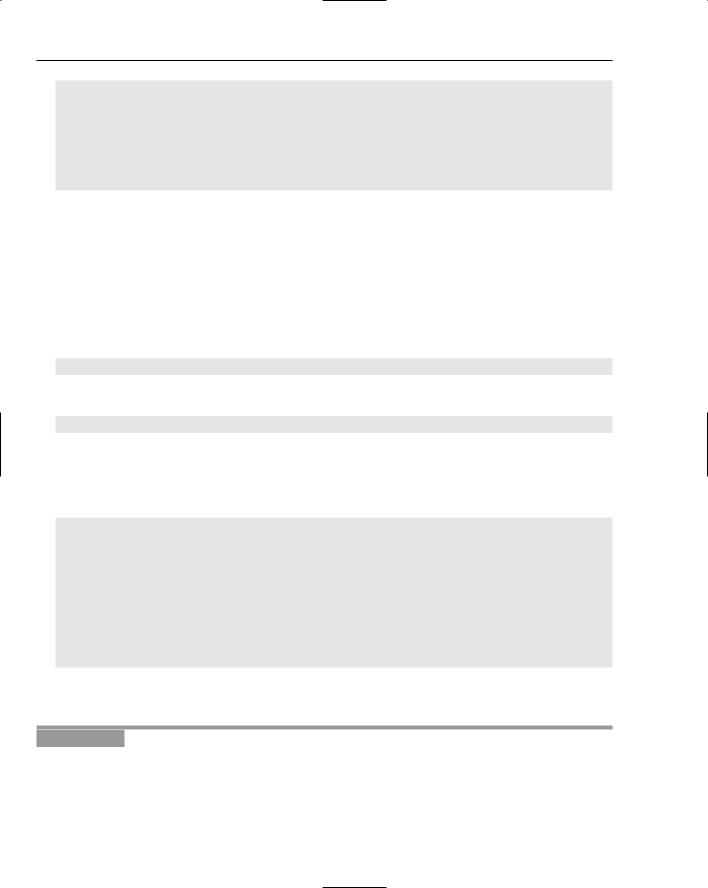
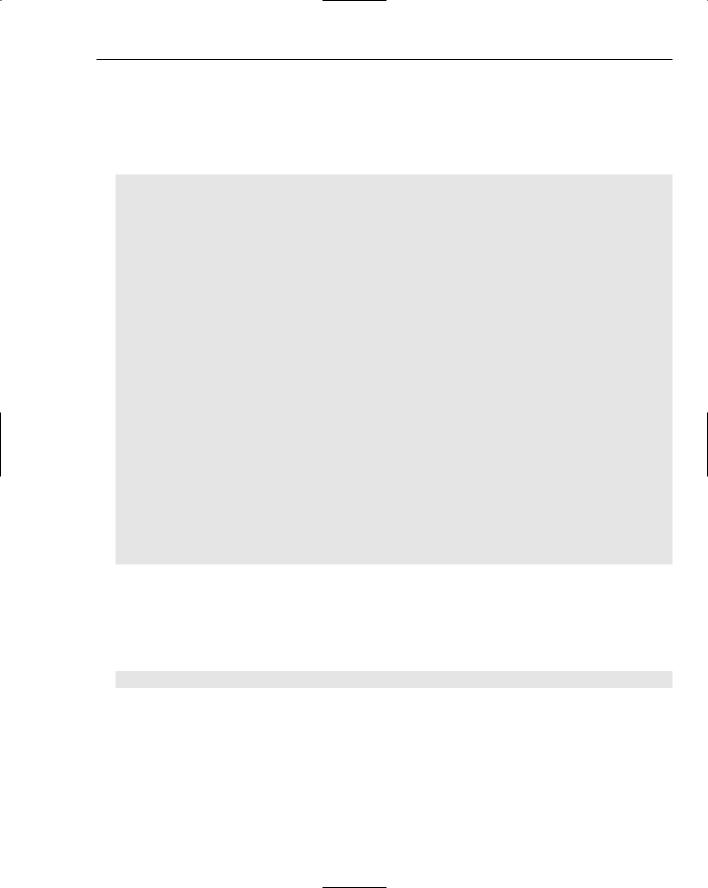