
Beginning ActionScript 2.0 2006
.pdf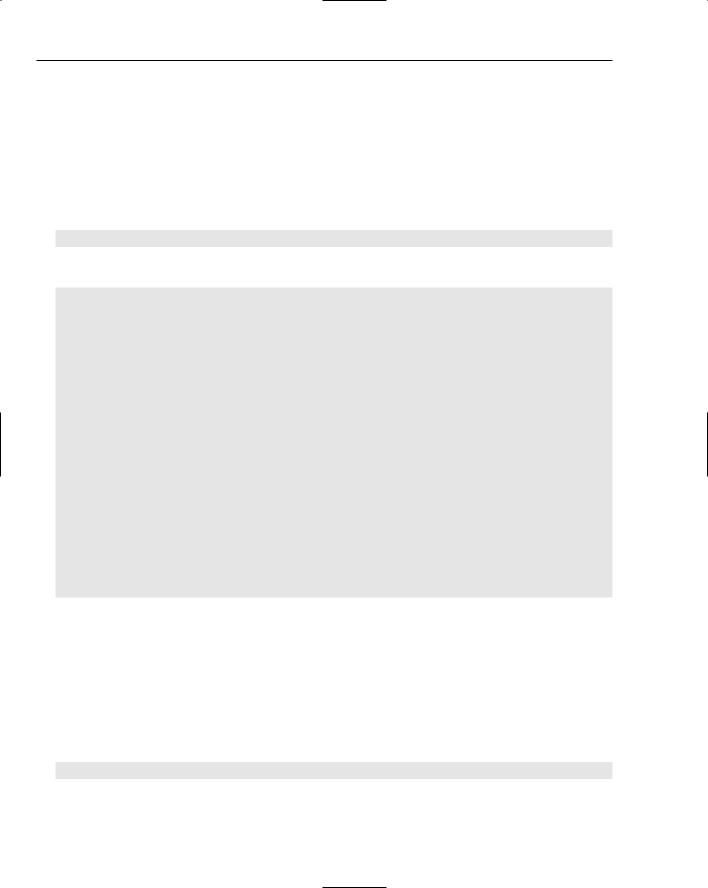
Chapter 25
The following sections show you how to use these methods.
Using browse()
The browse() method brings up a dialog box to allow the user to choose a file. It is used in conjunction with the onSelect() event and the upload() method.
This method takes one parameter, which is an array of objects describing the file types to allow for upload. The general form is as follows:
fileRef.browse(fileTypes);
A sample implementation might look like the following:
import flash.net.FileReference;
var fileTypes:Array = new Array(); var allTypes:Object = new Object(); allTypes.description = “All Types”; allTypes.extension = “*.*”; fileTypes.push(allTypes);
var fileRefListener:Object = new Object(); fileRefListener.onSelect = function(file:FileReference):Void
{
trace(“File selected: “ + file.name); var uploadSuccess:Boolean = ;
file.upload(“http://www.yourdomain.com/yourUploadHandlerScript.cfm”); if(uploadSuccess = false)
{
trace(“The upload failed.”);
}
}
var fileRef:FileReference = new FileReference(); fileRef.addListener(fileRefListener); fileRef.browse(fileTypes);
This functionality needs a server-side upload script to perform the actual upload. A variety of scripts are available for specific server technologies. The next Try It Out exercise shows one example upload script.
Using cancel()
The cancel() method aborts the upload or download of the referenced file. It enables you to provide the user with a cancel button that will stop the operation. The method takes no parameters.
Here’s its syntax:
fileRef.cancel();
638

Uploading and Downloading Files
Using download()
The download() method brings up a dialog box to enable the user to choose a file for download. The file to be downloaded can be any file on any server, not just the local server.
The method takes either one or two parameters. The first is the URL for the file to be downloaded. The optional second parameter is the default filename to place in the filename field in the Save File dialog box. If this parameter is omitted, the actual name of the file being downloaded is used.
Here’s the syntax for download():
fileRef.browse(fileURL:String, [defaultFileName:String]);
A sample implementation might look like the following:
import flash.net.FileReference;
var fileRefListener:Object = new Object(); fileRefListener.onComplete = function(file:FileReference)
{
trace(“onComplete : “ + file.name);
}
var url:String = “http://www.macromedia.com/platform/whitepapers/platform_overview.pdf”; var fileRef:FileReference = new FileReference(); fileRef.addListener(fileRefListener);
fileRef.download(url);
Using upload()
The upload() method starts the upload of the referenced file. It requires that the browse() method be called beforehand to enable the user to select a file.
The method takes one parameter, which is the URL to a server script to handle the actual upload. The script to be used depends on the server technology being used. Some languages that may be used to implement such a script include PHP, Perl, Java, or ColdFusion.
fileRef.upload(uploadScriptURL:String);
A sample implementation might look like the following:
import flash.net.FileReference;
var fileTypes:Array = new Array(); var allTypes:Object = new Object(); allTypes.description = “All Types”; allTypes.extension = “*.*”; fileTypes.push(allTypes);
var fileRefListener:Object = new Object();
639

Chapter 25
fileRefListener.onSelect = function(file:FileReference):Void
{
trace(“File selected: “ + file.name); var uploadSuccess:Boolean = ;
file.upload(“http://www.yourdomain.com/yourUploadHandlerScript.cfm”); if(uploadSuccess = false)
{
trace(“The upload failed.”);
}
}
var fileRef:FileReference = new FileReference(); fileRef.addListener(fileRefListener); fileRef.browse(fileTypes);
FileReference Class Properties
The FileReference class has several properties that you can use. They’re described in the following table:
Event |
Type |
Description |
|
|
|
creationDate |
Date |
The creation date of the local file. |
creator |
String |
The creator type of the file, if the file was created on a |
|
|
Macintosh. |
modificationDate |
Date |
The date and time when the local file was last modified. |
name |
String |
The name of the local file. |
size |
Number |
The size of the local file. |
type |
String |
The file type. |
|
|
|
The FileReference class also has a number of events. The following table describes them:
Event |
Type |
Description |
|
|
|
onCancel |
Listener |
Invoked when the user cancels the file browse dialog box. |
onComplete |
Listener |
Invoked when the file upload or the file download has |
|
|
completed. |
onHTTPError |
Listener |
Invoked when there is a network communication error |
|
|
that halts the file upload. |
onIOError |
Listener |
Invoked when a disk error occurs. |
onOpen |
Listener |
Invoked when the upload or download process has |
|
|
begun. |
onProgress |
Listener |
Invoked on regular intervals during the upload or |
|
|
download process. |
|
|
|
640
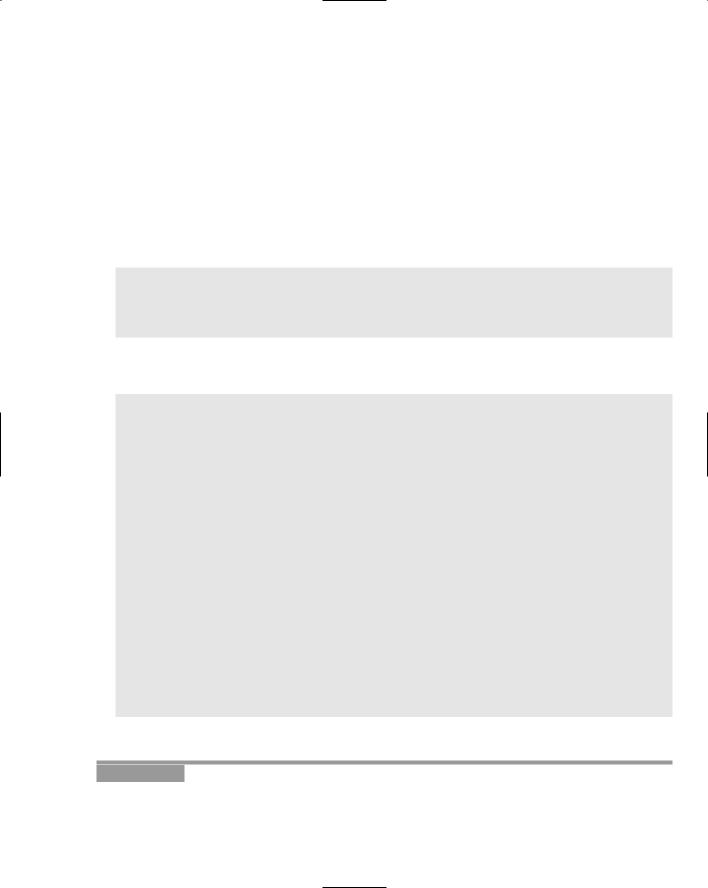
|
|
|
Uploading and Downloading Files |
|
|
|
|
|
|
|
|
|
Event |
Type |
Description |
|
|
|
|
|
onSecurityError |
Listener |
Invoked when the upload or download fails because of a |
|
|
|
security issue. |
|
onSelect |
Listener |
Invoked when the user has selected a file for upload or a |
|
|
|
directory for download from the file browse dialog box. |
|
|
|
|
Downloading Files
The simplest code that can be used to initiate a file download to the user’s own machine is the following:
import flash.net.FileReference; var url:String = ;
“http://www.macromedia.com/platform/whitepapers/platform_overview.pdf”; var fileRef:FileReference = new FileReference();
fileRef.download(url);
This prompts the user to select a download directory and then performs the download. It works but provides no feedback. You can make use of some of the available events to do more. Here’s an example:
import flash.net.FileReference;
var fileRefListener:Object = new Object(); fileRefListener.onOpen = function(file:FileReference)
{
trace(“Starting file download: “ + file.name);
}
fileRefListener.onProgress = function(file:FileReference, bytesLoaded:Number, ; bytesTotal:Number)
{
trace(“percentComplete: “ + (100 * bytesLoaded / bytesTotal));
}
fileRefListener.onComplete = function(file:FileReference)
{
trace(“Download complete : “ + file.name);
}
var url:String = ; “http://www.macromedia.com/platform/whitepapers/platform_overview.pdf”;
var fileRef:FileReference = new FileReference(); fileRef.addListener(fileRefListener); fileRef.download(url);
You work with a file download project in the following Try It Out.
Try It Out |
Downloading Files |
In this exercise, you work with the FileReference class for downloading files and learn how to integrate it with the progress bar component to provide download feedback.
641
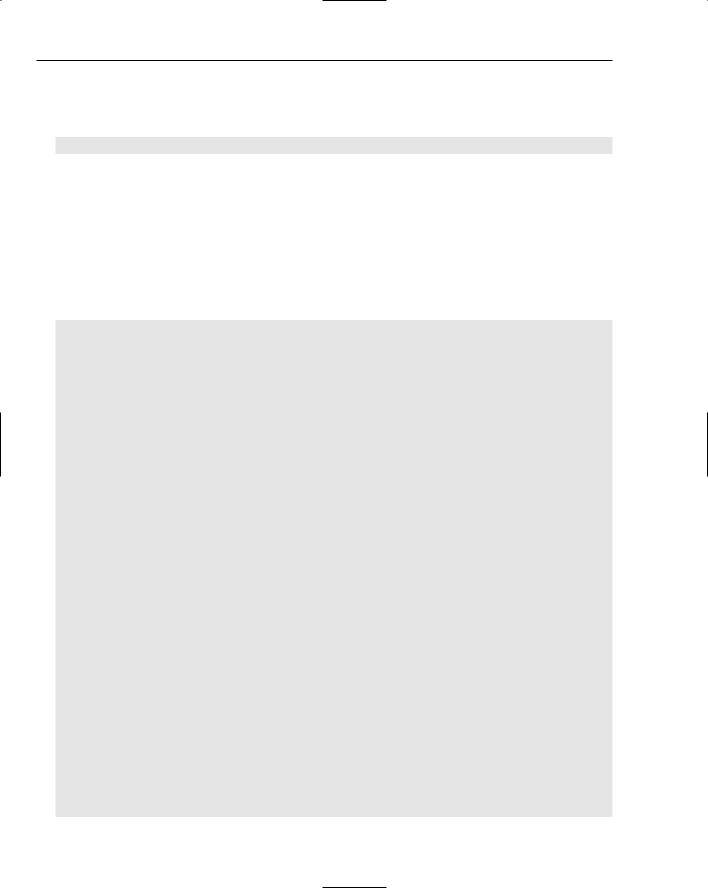
Chapter 25
1.Create a new Macromedia Flash document.
2.Click the first frame in the timeline, open the Actions panel (Window Development Panels Actions), and type the following ActionScript code:
#include “tryItOut_downloadFiles.as”
3.Open the User Interface section of the Components panel and open the Library panel. Drag the Button, Label, TextInput, and ProgressBar components to the Library panel.
4.Select File Save As, name the file tryItOut_downloadFiles.fla, choose an appropriate directory, and save it.
5.Create a new Macromedia Flash document by selecting File New and choosing ActionScript File from the New Document panel.
6.Save it as tryItOut_downloadFiles.as in the directory containing the Flash project file.
7.Type the following code into the new ActionScript file:
import mx.controls.Button; import mx.controls.Label; import mx.controls.TextInput; import mx.controls.ProgressBar; import flash.net.FileReference;
var eventHandler:Object = new Object(); var fileRefListener:Object = new Object();
var fileRef:FileReference = new FileReference();
setupInterface();
function setupInterface()
{
this.createClassObject(Label, “urlLabel”, this.getNextHighestDepth(), ; {_x:10, _y:13});
urlLabel.text = “URL:”;
this.createClassObject(TextInput, “urlInput”, this.getNextHighestDepth(), ; {_x:60, _y:10, _width:450});
urlInput.text = ; “http://www.macromedia.com/platform/whitepapers/platform_overview.pdf”;
this.createClassObject(Button, “openWindowButton”, ; this.getNextHighestDepth(), {_x:10, _y:50});
openWindowButton.label = “Download”; openWindowButton.addEventListener(“click”, eventHandler);
this.createClassObject(ProgressBar, “fileTransferProgressBar”, ; this.getNextHighestDepth());
fileTransferProgressBar._x = 120; fileTransferProgressBar._y = 50; fileTransferProgressBar.mode = “manual”; fileTransferProgressBar.label = “Downloaded %3%%”; fileTransferProgressBar._visible = false;
this.createTextField(“feedbackField”, this.getNextHighestDepth(), ;
642
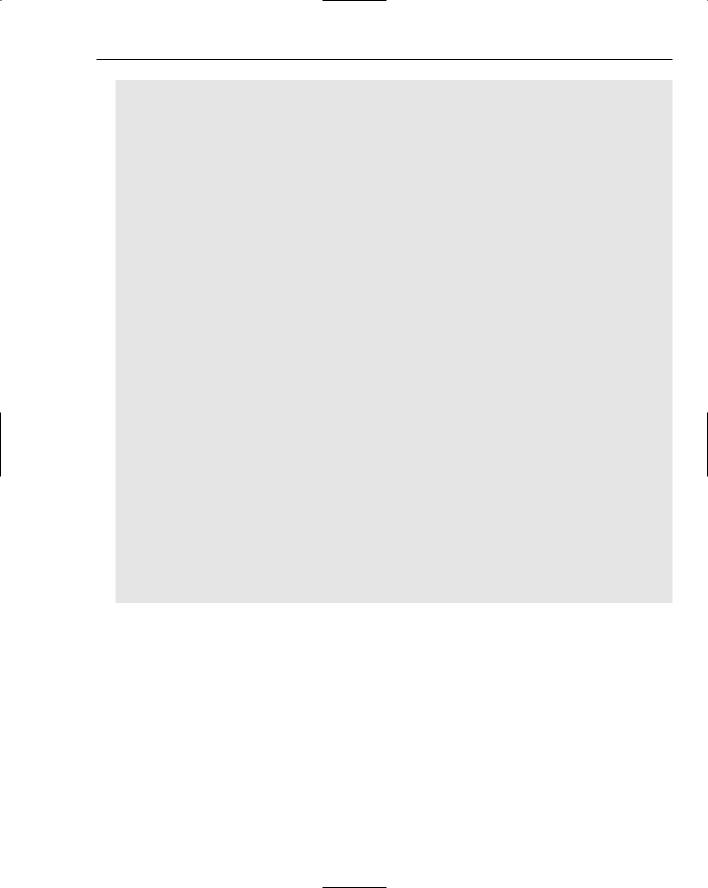
Uploading and Downloading Files
10, 80, 400, 30);
}
fileRefListener.onOpen = function(file:FileReference)
{
feedbackField.text = “Starting file download.”; fileTransferProgressBar._visible = true;
}
fileRefListener.onProgress = function(file:FileReference, bytesLoaded:Number, ; bytesTotal:Number)
{
feedbackField.text = “Download in progress.”; fileTransferProgressBar.setProgress(bytesLoaded, bytesTotal);
}
fileRefListener.onComplete = function(file:FileReference)
{
feedbackField.text = “Download complete.”; fileTransferProgressBar._visible = false;
}
fileRefListener.onIOError = function (file:FileReference)
{
feedbackField.text = “Download failed - disk error.”; fileTransferProgressBar._visible = false;
}
fileRefListener.onSecurityError = function (file:FileReference)
{
feedbackField.text = “Download failed - security/permissions error.”; fileTransferProgressBar._visible = false;
}
eventHandler.click = function()
{
fileRef.addListener(fileRefListener);
fileRef.download(urlInput.text);
}
8.Save the file, return to the Macromedia Flash project file, and select Control Test Movie.
How It Works
First, the relevant classes are imported, to make referring to component names and class names a bit easier:
import mx.controls.Button; import mx.controls.Label; import mx.controls.TextInput; import mx.controls.ProgressBar; import flash.net.FileReference;
Next, a few variables are declared. Even though you can download multiple files, you only need one instance of the FileReference class. If you wanted to be able to download several files at once, you would need one instance for each simultaneous connection:
643
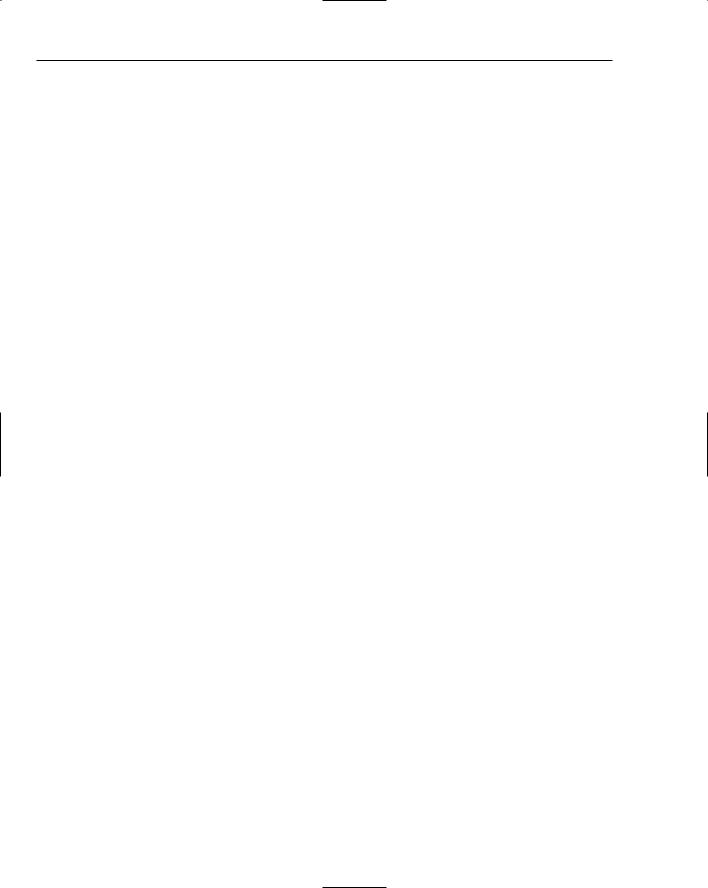
Chapter 25
var eventHandler:Object = new Object(); var fileRefListener:Object = new Object();
var fileRef:FileReference = new FileReference();
The user interface is set up, which involves placing the Label, TextInput, Button, and ProgressBar components on the stage:
setupInterface();
function setupInterface()
{
this.createClassObject(Label, “urlLabel”, this.getNextHighestDepth(), ; {_x:10, _y:13});
urlLabel.text = “URL:”;
this.createClassObject(TextInput, “urlInput”, this.getNextHighestDepth(), ; {_x:60, _y:10, _width:450});
urlInput.text = ; “http://www.macromedia.com/platform/whitepapers/platform_overview.pdf”;
this.createClassObject(Button, “openWindowButton”, ; this.getNextHighestDepth(), {_x:10, _y:50});
openWindowButton.label = “Download”; openWindowButton.addEventListener(“click”, eventHandler);
this.createClassObject(ProgressBar, “fileTransferProgressBar”, ; this.getNextHighestDepth());
fileTransferProgressBar._x = 120; fileTransferProgressBar._y = 50; fileTransferProgressBar.mode = “manual”; fileTransferProgressBar.label = “Downloaded %3%%”; fileTransferProgressBar._visible = false;
this.createTextField(“feedbackField”, this.getNextHighestDepth(), ; 10, 80, 400, 30);
}
Next, you define event handlers for the FileReference instance. The onOpen event is a good place for startup code:
fileRefListener.onOpen = function(file:FileReference)
{
feedbackField.text = “Starting file download.”; fileTransferProgressBar._visible = true;
}
The onProgress event passes on the bytes loaded and total bytes information to the progress bar:
fileRefListener.onProgress = function(file:FileReference, bytesLoaded:Number, ; bytesTotal:Number)
{
feedbackField.text = “Download in progress.”; fileTransferProgressBar.setProgress(bytesLoaded, bytesTotal);
}
644
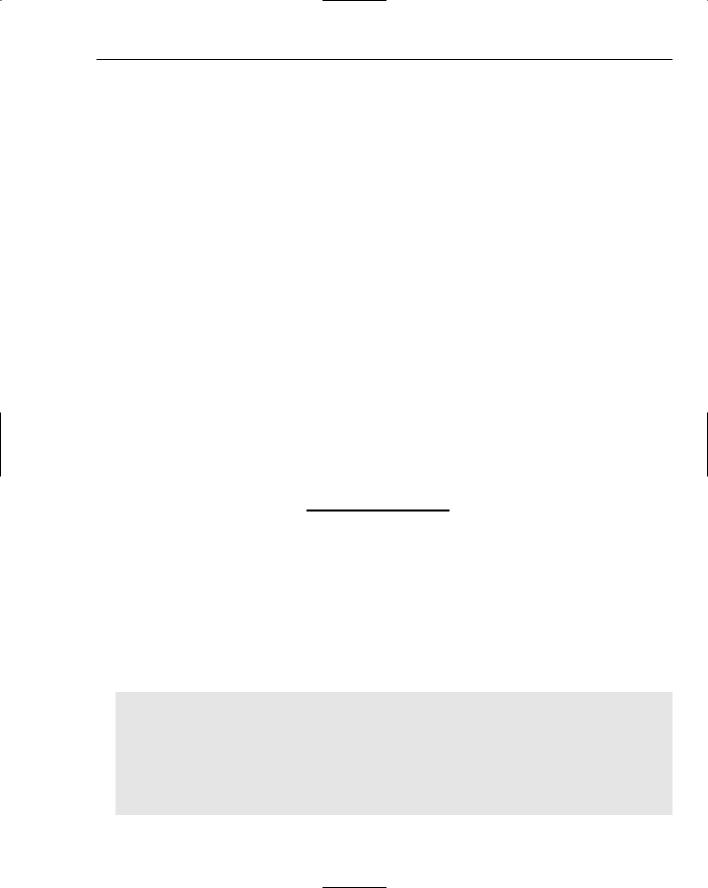
Uploading and Downloading Files
The onComplete event hides the progress bar:
fileRefListener.onComplete = function(file:FileReference)
{
feedbackField.text = “Download complete.”; fileTransferProgressBar._visible = false;
}
The error events are captured in case there is a download issue. There is no onHTTPError event handler defined because for file downloads, network errors only generate an onIOError event:
fileRefListener.onIOError = function (file:FileReference)
{
feedbackField.text = “Download failed - disk error.”; fileTransferProgressBar._visible = false;
}
fileRefListener.onSecurityError = function (file:FileReference)
{
feedbackField.text = “Download failed - security/permissions error.”; fileTransferProgressBar._visible = false;
}
Finally, the event handler for the button is defined, which actually initiates the download process:
eventHandler.click = function()
{
fileRef.addListener(fileRefListener);
fileRef.download(urlInput.text);
}
Uploading Files
Uploading files is only a bit more work than downloading files. The main difference is the need for a server-side upload script to handle the actual uploading. Flash cannot do that part because it loads on the user’s machine, whereas the file upload capability requires write access to the server. What Flash provides is the packaging mechanism so that the file to be uploaded is sent to the server in a standardized format that any file upload script can handle.
Here’s the base ActionScript code needed to make this work:
import flash.net.FileReference;
var fileTypes:Array = new Array(); var allTypes:Object = new Object(); allTypes.description = “All Types”; allTypes.extension = “*.*”; fileTypes.push(allTypes);
var fileRefListener:Object = new Object();
645
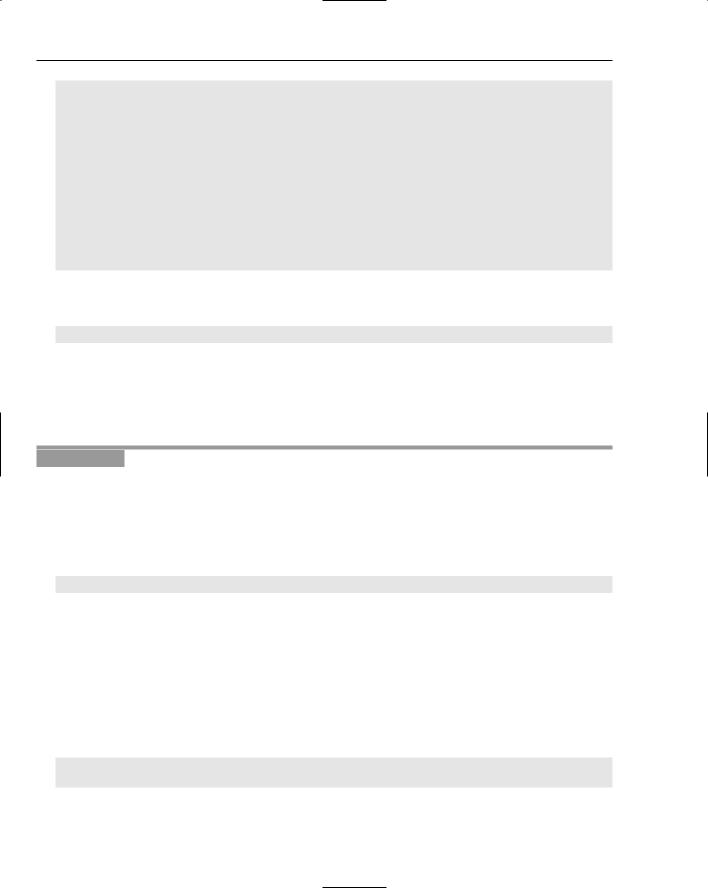
Chapter 25
fileRefListener.onSelect = function(file:FileReference):Void
{
trace(“File selected: “ + file.name); var uploadSuccess:Boolean = ;
file.upload(“http://www.yourdomain.com/yourUploadHandlerScript.cfm”); if(uploadSuccess = false)
{
trace(“The upload failed.”);
}
}
var fileRef:FileReference = new FileReference(); fileRef.addListener(fileRefListener); fileRef.browse(fileTypes);
Starting from the top, a list of file types that the user can choose from is provided. In this case the user can choose any file type. The file type list is an array of objects where each object is in the format
{description:”<User viewable description>”, extension:”*.png; *.gif; *.jpg”}
Next, the onSelect() event is defined. This event is triggered only after the user has selected a file and is the proper place to put the upload() method.
Finally, a FileReference instance is created, is bound to the event listener, and the browse() method is called to bring up the file browser dialog box.
Try It Out |
Upload Files |
In this exercise, you work with the FileReference class to upload files and learn how to integrate it with the progress bar component to provide upload feedback.
1.Create a new Macromedia Flash document.
2.Click the first frame in the timeline, open the Actions panel, and type the following ActionScript code:
#include “tryItOut_uploadFiles.as”
3.Open the User Interface section of the Components panel and open the Library panel. Drag the Button and ProgressBar components to the Library panel.
4.Select File Save As, name the file tryItOut_uploadFiles.fla, choose an appropriate directory, and save it.
5.Create a new Macromedia Flash document by selecting File New and choosing ActionScript File from the New Document panel.
6.Save the file as tryItOut_uploadFiles.as in the directory containing the Flash project file.
7.Type the following code into the new ActionScript file:
import mx.controls.Button;
import mx.controls.ProgressBar;
646
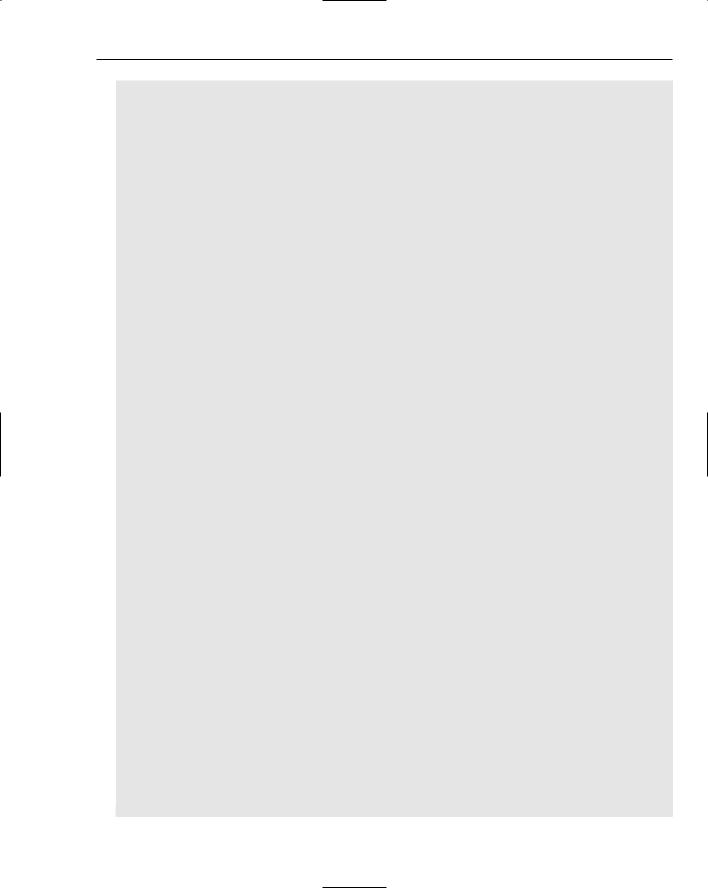
Uploading and Downloading Files
import flash.net.FileReference;
var eventHandler:Object = new Object(); var fileRefListener:Object = new Object();
var fileRef:FileReference = new FileReference();
var fileTypes:Array = new Array();
var imageTypes:Object = new Object();
imageTypes.description = “Images (*.jpg, *.jpeg, *.gif, *.png)”; imageTypes.extension = “*.jpg; *.jpeg; *.gif; *.png”; fileTypes.push(imageTypes);
var htmlTypes:Object = new Object(); htmlTypes.description = “HTML (*.html, *.htm)”; htmlTypes.extension = “*.html; *.png”; fileTypes.push(htmlTypes);
setupInterface();
function setupInterface()
{
this.createClassObject(Button, “openWindowButton”, ; this.getNextHighestDepth(), {_x:10, _y:20});
openWindowButton.label = “Upload”; openWindowButton.addEventListener(“click”, eventHandler);
this.createClassObject(ProgressBar, “fileTransferProgressBar”, ; this.getNextHighestDepth());
fileTransferProgressBar._x = 120; fileTransferProgressBar._y = 20; fileTransferProgressBar.mode = “manual”; fileTransferProgressBar.label = “Uploaded %3%%”; fileTransferProgressBar._visible = false;
this.createTextField(“feedbackField”, this.getNextHighestDepth(), ; 10, 50, 400, 30);
}
fileRefListener.onSelect = function(file:FileReference)
{
feedbackField.text = “File selected: “ + file.name; file.upload(“http://www.yourdomain.com/yourUploadHandlerScript.cfm”)
}
fileRefListener.onOpen = function(file:FileReference)
{
feedbackField.text = “Starting file upload.”; fileTransferProgressBar._visible = true;
}
fileRefListener.onProgress = function(file:FileReference, bytesLoaded:Number, ; bytesTotal:Number)
{
feedbackField.text = “Upload in progress.”;
647