
Beginning ActionScript 2.0 2006
.pdf
Chapter 23
The following table describes the LocalConnection class’s methods:
Method |
Returns |
Description |
|
|
|
close() |
Nothing |
Closes the local connection. |
connect() |
Boolean |
Establishes a local connection. |
send() |
String |
Sends a localConnection object a request, specifying the method to |
|
|
be invoked. |
domain() |
String |
Returns the domain the SWF is running from. |
The close() method has no parameters. Here’s its syntax:
localConnection.close();
The connect() method has a single parameter of type String. The string value is the name of an available local connection. Following is its syntax:
localConnection.connect(name:String);
This send() method has three parameters:
1.The name of the available LocalConnection. It must be a String.
2.The name of the method being accessed in the receiving localConnection object. This also must be a String.
3.An object representing variables to be sent to the receiving method. This method returns a Boolean if the localConnection object was found and if the method was valid.
Here’s the syntax for send():
localConnection.send(name:String, method:String, parameters:Object)
The domain() method has no parameters and simply returns a String representation of the domain from which the SWF file comes. Here’s its syntax:
localConnection.domain();
The following table describes the LocalConnection class’s events:
Event |
Type |
Description |
|
|
|
onStatus |
Function |
Fires when a request is made to a localConnection object |
|
|
using the send() method. |
allowDomain |
Function |
Fires whenever an SWF attempts to make a connection |
|
|
via the send() method. |
allowInsecureDomain |
Function |
Fires whenever an SWF attempts to make a connection |
|
|
via the send() method. |
578
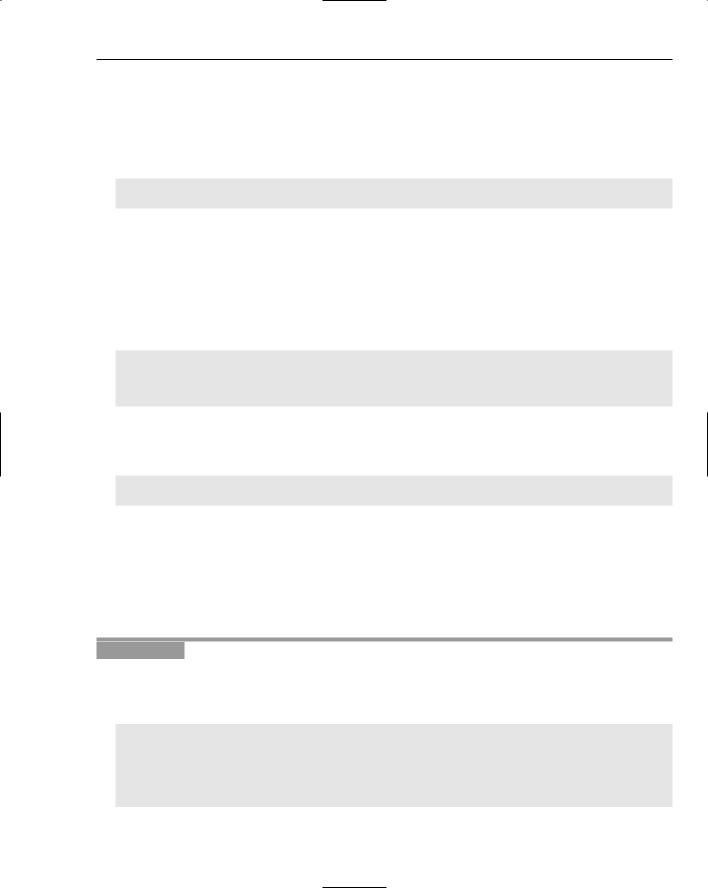
Communicating Between the Macromedia Flash Plug-in and the Browser
Creating a localConnection Object
In this section, you take a look at how to create a localConnection object. To establish a connection between two .swf files, a channel needs to be established between the two files. One of the SWF files is responsible for doing the initial setup, using the connect() method. The code to perform this task looks like this:
var receiving_lc:LocalConnection = new LocalConnection();
receiving_lc.connect(“sampleLCchannel”);
Once that’s done, the SWF file running this code is registered as the recipient for any data transmitted along the channel called sampleLCchannel, and it is the only SWF file that is allowed to receive data on this channel. Next, an action needs to be created to respond anytime data is sent along this channel. Just create a method with the localConnection object, giving the new method the name that you want the other side of the connection to call. The following code adds a method called sendVar() that responds when another .swf sends data along the channel. The name of the method can be whatever you want, as long as the same method name is used on the other side of the connection:
var receiving_lc:LocalConnection = new LocalConnection(); receiving_lc.sendVar = function(sentString:String)
{
trace(sentString);
}; receiving_lc.connect(“sampleLCchannel”);
The SWF file doing the sending makes the following call to send data to the SWF file that initially set up the connection. The code sends a string to the sendVar() method on sampleLCchannel:
var movie1LocalConnect = new LocalConnection();
movie1LocalConnect.send(“sampleLCchannel”, “sendVar”, “The quick brown fox...”);
For this to work, the receiving SWF file has to have already set up the connection. Otherwise, the transmission is simply dropped and the send() method returns false.
Any number of SWF files can send data along a channel, but only one SWF file can receive data on a channel.
Try setting up a connection between two SWF files.
Try It Out |
Create a Connection between Two SWF Files |
1.Open a new Flash Document. Save the Flash document as lcmovie1.fla in your work folder.
2.Click the first frame in the timeline, open the Actions panel, and type the following ActionScript code:
var movie1LocalConnect = new LocalConnection(); submitButton.onRelease = function()
{
trace(movie1LocalConnect);
movie1LocalConnect.send(“sampleLCchannel”, “sendVar”, dataToSend.text);
};
579
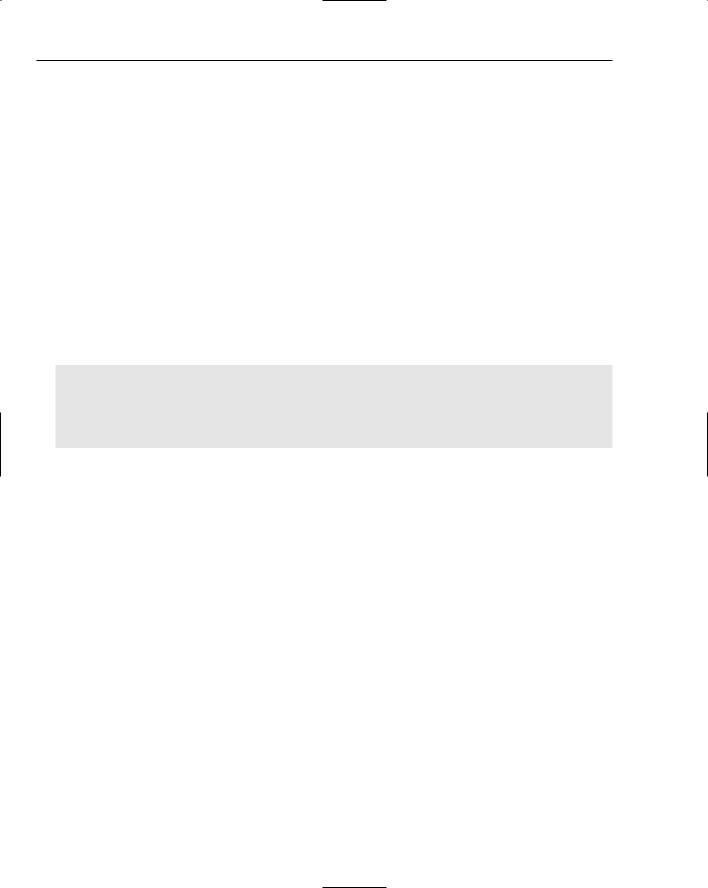
Chapter 23
3.Open the Components panel. Expand the User Interface tab and drag a Button component to the center of the stage.
4.In the Parameters tab of the Properties panel for the button you just added to the stage, find the label parameter and change the word Button to Send. In the Properties panel give the button an instance name of submitButton.
5.Now create an input text field. You could do this with ActionScript, but draw one on the stage just above the send button, using the Text tool in the Tools panel. Be sure to select a border and change the field to Input Text in the properties panel for the textField. Name the textField dataToSend in the Instance Name field in the Properties panel.
6.Publish lcmovie1 with HTML. You open this HTML file in step 11.
7.Now open another new Flash document. Save the Flash document as lcmovie2.fla in your work folder.
8.Using the Text tool in the Tools panel, create a textField on the lcmovie2 stage. In the Properties panel give this text field instance the name incomingText_txt. In the Properties panel, be sure to select Dynamic Text as the field type.
9.In the first frame of lcmovie2, type the following code:
var receiving_lc:LocalConnection = new LocalConnection(); receiving_lc.sendVar = function(aVar:String)
{
_root.incomingText_txt.text = aVar;
}; receiving_lc.connect(“sampleLCchannel”);
10.Publish lcmovie2.
11.Navigate to your work folder to which you have published your SWF files. Open the lcmovie1.html file in your favorite browser.
12.With the HTML window still open, navigate to your work folder. Open the lcmovie2.swf file directly, or within an HTML page.
13.In lcmovie1 type some information to see it populate within the lcmovie2.swf.
How It Works
In this example you examined how you can use a local connection to populate data into one SWF from another SWF. In each SWF you were required to instantiate a localConnection object; however, you were not required to give them the same name. The common bond between the two was the parameter specified in lcmovie2 within the connect() method. When connect() fires, a localConnection object is started, in this case, with the String sampleLCchannel.
Any SWF file that knows to poll a localConnection object residing on the system with the String name sampleLCchannel can access lcmovie2. This is what is happening in lcmovie1. Because lcmovie1 knows the name of an available localConnection object, it attempts to pass the localConnection a method to send to that available localConnection.
580
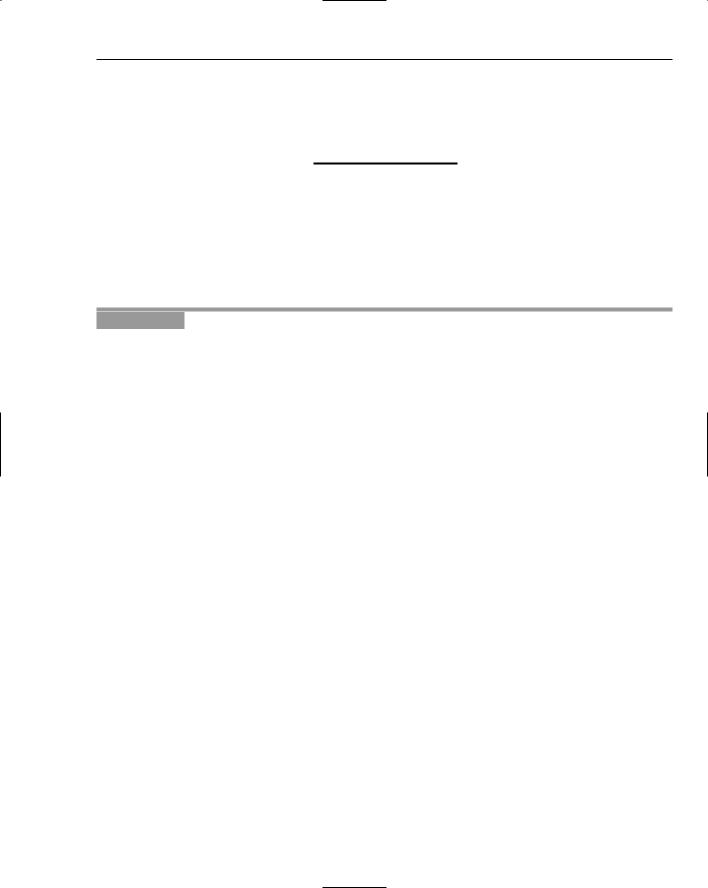
Communicating Between the Macromedia Flash Plug-in and the Browser
sampleLCchannel ultimately is the public name of the receiving_lc localConnection object. Any methods you define within receiving_lc will be publicly available. One SWF file can offer several localConnection objects with their own method sets.
Had lcmovie2 and lcmovie1 hailed from different domains, this example wouldn’t have worked at all.
Security
As with shim movies, script access is always a security concern. The localConnection object uses its own allowDomain() method that works similarly to the System.security.allowDomain() method covered in Chapter 22. It is important to remember that policy files and the System.security.allowDomain() method do not enable LocalConnection to communicate between SWF files that originate from different servers.
Try It Out |
Using allowDomain with LocalConnection |
In this exercise you add to the lcmovie.fla file from the preceding Try It Out to allow the localConnection object within lcmovie1 to communicate.
1.Open the lcmovie2 FLA.
2.Click the first frame in the timeline and open the Actions panel. Note the line edition and be sure your code looks like the following code. You should substitute your own domain for someserver.com:
var receiving_lc:LocalConnection = new LocalConnection(); receiving_lc.allowDomain(“*.someserver.com”); receiving_lc.sendVar = function(aVar:String)
{
_root.incomingText_txt.text = aVar;
};
receiving_lc.connect(“sampleLCchannel”);
3.Upload lcmovie1.swf and lcmovie1.html to your server.
4.Navigate to lcmovie1.html in your browser. This address might be something like http://www
.someserver.com/lcmovie1.html.
5.After you have the browser open to the SWF, go back to your work folder and open lcmovie2.swf.
6.With both now open, attempt to type in a String and send the String from the browser-based SWF to the SWF file on the local computer.
How It Works
In this example you saw a much more powerful use of LocalConnection. You were able to specify a domain as allowed to access an SWF running on the local machine.
581
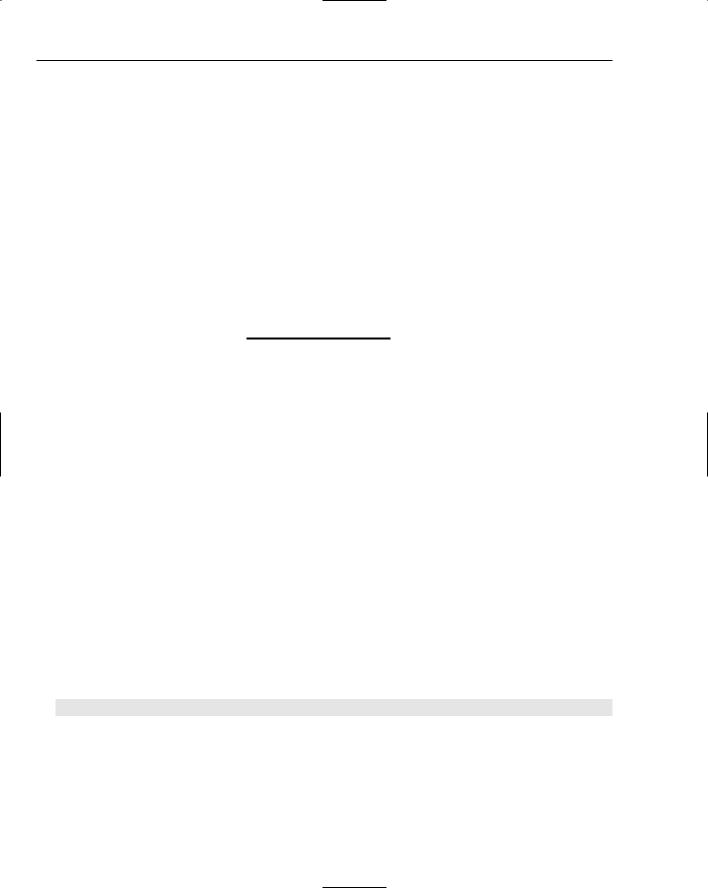
Chapter 23
There may be times, however, when you would prefer any SWF from any domain to access your localConnection objects in your local SWF. As with the policy file and the System.security.allowDomain methods, you can use wildcards to allow for access control. You saw in this example a use of a wildcard to include any subdomains. You can use a wildcard more generally by using it alone, such as
receiving_lc.allowDomain(“*”);
The example you just completed might seem a lot like the first example at first glance. However, consider a scenario in which the local SWF is residing within an SWF wrapper such as Northcode. The LocalConnection would allow the local SWF file, which has extended abilities on the local machine, to open an API to browser-based SWF files.
You might imagine creating an SWF that runs locally, which connects to browser-based SWF files, giving your intranet applications unprecedented agility on the user’s system.
Because you are required to use allowDomain() for this type of instance, you can be confident that malicious coders who seek to use the localConnection object are thwarted.
Storing Data Locally with Shared Objects
The previous section talked about SWF files communicating with one another in a simple and effective manner. This section talks about the SharedObject class. Shared objects are often referred to in Flash as Flash cookies. Flash cookies can be an effective method for displaying information left over from the previous visit or tailoring a visit based on previous experience. The SharedObject class gives your SWF a memory.
The following table describes the SharedObject class’s methods:
Method |
Return |
Description |
|
|
|
getSize() |
Number |
Returns the size of the sharedObject in bytes. |
getLocal() |
SharedObject |
Returns a sharedObject containing all the values saved via |
|
|
the flush method. |
flush() |
Object |
Writes a value to the local disk and returns the value. |
clear() |
Nothing |
Removes data created by the flush method. |
|
|
|
The getSize() method has no parameters. Here’s its syntax:
sharedObject.getSize();
This method is usually employed to check that the data object hasn’t reached a maximum allowed size limit.
The getLocal() method has three parameters: the name of the available sharedObject, which must be a String; the URL of the SWF that wrote the local shared object, also a String; and a Boolean stating whether the SWF that created the sharedObject came from an HTTPS connection. If true, the accessing
582
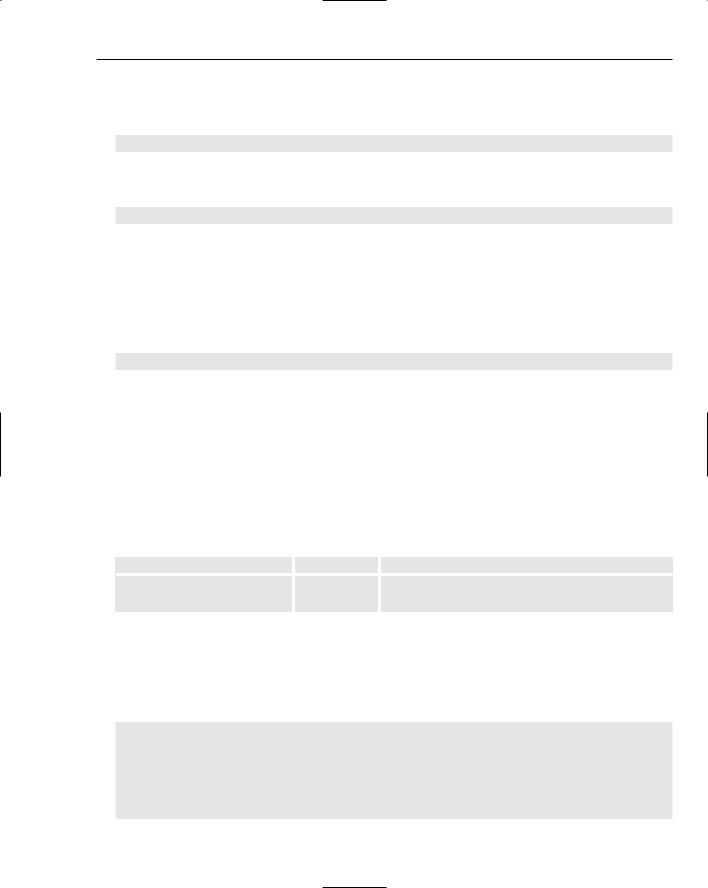
Communicating Between the Macromedia Flash Plug-in and the Browser
SWF must be served from a web server that has established a secure connection between itself and your browser, as is the case when you browse to a URL that starts with https://. Here’s the getLocal() syntax:
sharedOBject.getLocal(name:String,URL:String,HTTPS:Boolean);
The flush() method has one optional parameter: a Number representing a disk space allotment request in bytes. Here’s the method’s syntax:
sharedObject.flush(byteSpace:Number);
If your sharedObject requires only 20 bytes, but you think the shared object will grow, you can request a larger value such as 100 via the byteSpace parameter. You can avoid having Flash prompt the user to manually allow for a larger disk allotment with each subsequent flush by asking for the full allotment in the first alert.
The clear() method has no parameters and simply removes the shared object from the local disk. Here’s its syntax:
sharedObject.clear();
The SharedObject class has one property:
|
Event |
|
Type |
Description |
|
|
|
|
|
|
data |
|
Object |
Objects placed within the data object are the items |
|
|
|
|
that are saved to the sharedObject on the local disk |
|
|
|
|
when the flush method is called. |
|
The SharedObject class has one event: |
|
||
|
|
|
|
|
|
Event |
|
Type |
Description |
|
|
|
|
|
|
sharedObject.onStatus |
Function |
Fires whenever a change in the sharedObject is |
|
|
|
|
|
detected. |
|
|
|||
Try It Out |
Create a Shared Object and Save Some Data |
1.Open a new Flash Document. Save it as sharedObjectSample.fla in your work folder.
2.Click the first frame in the timeline, open the Actions panel, and enter the following ActionScript code:
var myPersistentObject:SharedObject = SharedObject.getLocal(“myData”); if (myPersistentObject.data.test1 != 1)
{
myPersistentObject.data.test1 = 1; var save = myPersistentObject.flush(); trace(save);
}
583

Chapter 23
3.
4.
Test the SWF by pressing Ctrl+Enter.
Save your FLA in your work folder. You’ll be using it in the next Try It Out.
How It Works
In this example you call the static function getLocal() to create a sharedObject. When you do this, Flash automatically checks for any existence of a previous object with the same name specified in the name parameter of the getLocal method. Because it loads the data property with any pre-existing objects, you can test whether or not you should save new data or work with the existing data.
Saving a variable is easy. You populate the data object as if it were any other object, by declaring a variable upon it. In this case you declare the variable test1 as equal to four.
You then commit the new data object by calling the flush method. Because the flush method returns a Boolean, depending on success, you can populate a variable with the return value of flush. In this case you have traced it. In the following examples you see how you can use this value to determine actions that affect the user.
Acceptable Data Types
Unlike using LoadVars, you can save many different types of data in flashObjects, and they will retain their type and values.
You can save arrays, objects, Booleans, strings, and so on. But there are limitations. More complex objects such as XML or LoadVars will fail. You also can’t define a function or class and store it in the sharedObject.
Try It Out |
Retrieving Data Objects |
1.Open the sharedObjectSample.fla you created in the previous Try It Out.
2.Click the first frame in the timeline, open the Actions panel, and change the ActionScript to the following code:
var myPersistentObject:SharedObject = SharedObject.getLocal(“myData”); if (myPersistentObject.data.test2 != 2)
{
myPersistentObject.data.test2 = 2; var save = myPersistentObject.flush(); trace(save);
}
else
{
trace(myPersistentObject.data.test2);
}
3.Test the SWF by pressing Ctrl+Enter. The SWF appears to do nothing.
4.Close the SWF and test it again by pressing Ctrl+Enter.
5.Verify that the number 2 is now in the output window.
6.Save your FLA in your work folder. You’ll continue working in it in the next Try It Out.
584

Communicating Between the Macromedia Flash Plug-in and the Browser
How It Works
In this example you saw how you can set a sharedObject and then use the exact same function to retrieve the data. You didn’t have to do this, but in this way you can see how the sharedObject can be made compact due to its static method set and multifunctional getLocal method.
This example also showed how immediately you can begin to access a pre-existing sharedObject via accessing the data property directly as if it were any other object.
Using Shared Objects as Cookies
Now that you know how to save and retrieve sharedObjects, you’re going to try something more useful. Say, for example, that you want to remember the name of a visitor. Say also that when the visitor returns, you don’t want to ask them their name again, and so the user interface should instead show a welcome message. Also record the time.
You use a textField and a component button to complete the example.
Try It Out |
An SWF That Remembers You |
1.Open sharedObjectSample.fla.
2.Open the Components panel. Expand the User Interface tab and drag a Button component to the center of the stage.
3.In the Parameters tab of the Properties panel for the button that you just added to the stage, find the label parameter and change the word Button to Submit. In the Properties panel give the button an instance name of submitButton.
4.Now create an input text field. Draw one on the stage just above the Send button using the Text tool in the Tools panel. Be sure to select the border property and change the field to Input Text in the Properties panel for the textField. Name the textField dataToSave_txt in the Instance Name field in the Properties panel.
5.Select the textField you just created. Copy and paste this field, so that two fields now exist on the stage. Change the instance name of the new field to instructions_txt and change the field type to Dynamic Text. Place the dynamic textField just above the existing input textField. Deselect the border property for this textField.
6.Click the first frame in the timeline, open the Actions panel, and change the ActionScript to the following code:
stop();
instructions_txt.text = “Please enter your name:”;
var myPersistentObject:SharedObject = SharedObject.getLocal(“myData”); if (myPersistentObject.data.username != undefined)
{
initialVisit = false;
this.gotoAndStop(2);
}
else
{
submitButton.onRelease = function()
{
585

Chapter 23
initialVisit = true;
myPersistentObject.data.username = dataToSave_txt.text; var login_date = new Date(); myPersistentObject.data.date = ;
(login_date.getMonth()+1)+”.”+login_date.getDate();
+”.”+login_date.getFullYear(); myPersistentObject.flush(); gotoAndStop(2);
};
}
7.Now that you have your actions for frame 1, create a new empty frame that will be frame 2.
8.On frame 2, create a multiline text field in the center of the stage. Be sure that the multiline property is selected and give this field an instance name of welcome_txt.
9.On frame 2, open the Components panel and drag a Button component onto the center of the stage.
10.In the Parameters tab of the Properties panel for the button that you just added to the stage, find the label parameter, and change the word Button to Clear User. In the Properties panel give the button an instance name of clearButton.
11.Click the second frame in the timeline, open the Actions panel, and change the ActionScript to the following code, which constructs the welcome message and adds a function for clearing the information:
if (initialVisit == true)
{
welcome_txt.text = “Welcome “+myPersistentObject.data.username+”!”+newline; welcome_txt.text += “Today is “+myPersistentObject.data.date+”.”;
}
else
{
welcome_txt.text = “Welcome back “ ;
+myPersistentObject.data.username+”!”+newline;
welcome_txt.text += “You last visited on “+myPersistentObject.data.date+”.”;
}
clearButton.onRelease = function()
{
myPersistentObject.clear();
gotoAndStop(1);
}
12.Test the SWF by pressing Ctrl+Enter.
13.Enter a name and click Submit.
14.You will now see a welcome message and the Clear User button. Do not click the Clear User button. Close the SWF.
15.Test the SWF again by pressing Ctrl+Enter.
16.You will now see a welcome screen instead of a login.
17.To clear the information and try again, click the Clear User button.
18.You will now be back at frame 1, and you can enter in a new name.
19.Save your FLA in your work folder. You’ll need it for the next Try It Out.
586
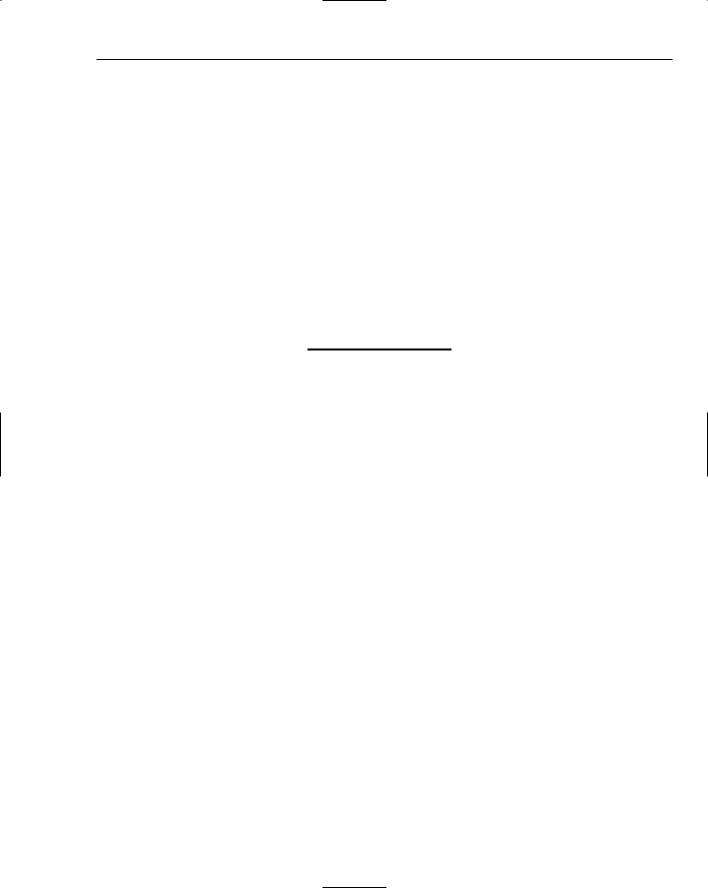
Communicating Between the Macromedia Flash Plug-in and the Browser
How It Works
In this example you expanded on the principles of the previous example to save a String. You then used the existence of that String to change the behavior of the SWF when it loads. In this case, you set a username and logindate as properties of the data object. You check the existence of a username in the cookie to determine whether or not to proceed directly to the welcome interface, or stay and display the login interface. By extracting the data from the sharedObject before anything else, you can populate the user interface with customized content based on the user’s last visit.
What this code also does is prepare for the fact that a sharedObject can be removed from the local system without your knowledge, and so the code needs to be able to reset the data, or otherwise recover in some way. Had the data been lost, and you just assumed the data would be there, your welcome message in frame 2 might look a bit incomplete and broken.
The other important method you saw in action in this example was the clear method. You can see that you made a Clear User button that used the clear method and returned the application to its initial cookieless state. When you call clear, it clears all of the data within the data property in the sharedObject.
Working with the User
One thing you have to keep in mind, especially with larger sharedObjects, is that the user has full control over whether you can store data locally. He can set size limits, forbid access, and clear data himself.
You can do several things to work with the user to change the settings and request access. It is conceivable, however, that the user will block you completely from saving data. Your application should still be able to respond in this case, by either working without the data, or fail gracefully, while letting the user know why.
One common method for managing user settings is via the bytes parameter in the flush method. When flush is called without this parameter, Flash measures the size of the data being saved. If the available size of the allotted disk space for your cookie is larger than the size of the data object, Flash will automatically open the settings dialog box of the Flash player to the Local Storage section. Your SWF file continues playing, but the flush fails for the session. If the user has elected to allot disk space, flush returns true.
To deal with a pending or false response from the flush object, you can use the onStatus event to make decisions about what the application should do in that case. onStatus populates its parameter with an object. This object is returned as an object. Iterating through the object reveals the code of the response from the user. If the user denies an allocation, and there isn’t enough room in the existing disk space, you will see the value code=SharedObject.Flush.Failed. In this case you can decide to go to a different welcome page or alert the user. If the user allows the allotment request, the code returns
SharedObject.Flush.Success.
Managing Disk Space
If and when you make a sharedObject request, think about how your application might be doing so, and how often. After you hit the limit, every time you call the flush method, the user may be presented the settings dialog. That could get fairly annoying. If you plan to have more data saved as your application works, request an allocation early, and make it a sensible size. For example, when the application starts,
587