
Beginning ActionScript 2.0 2006
.pdf
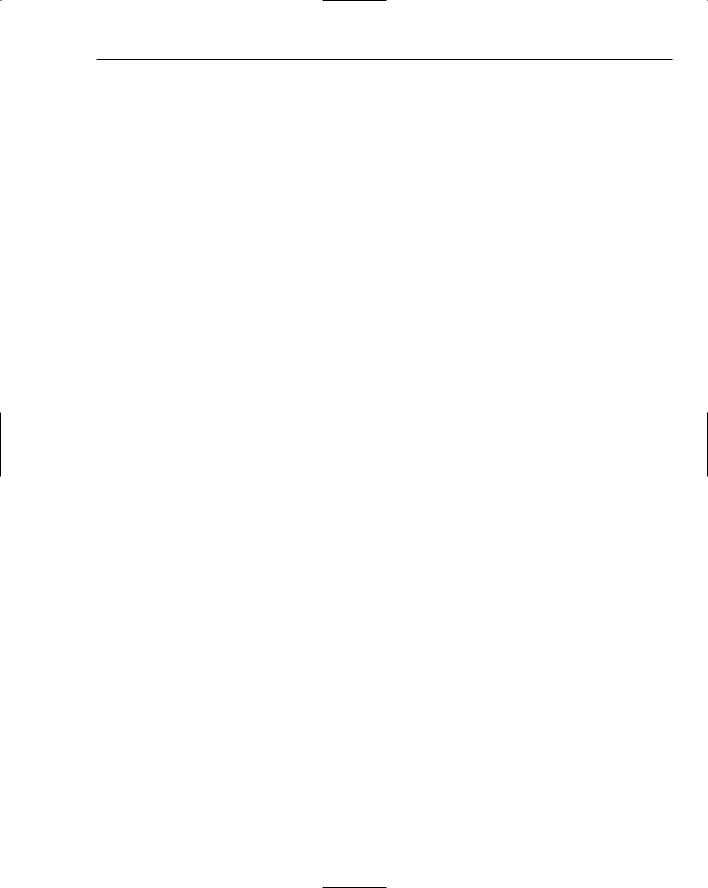

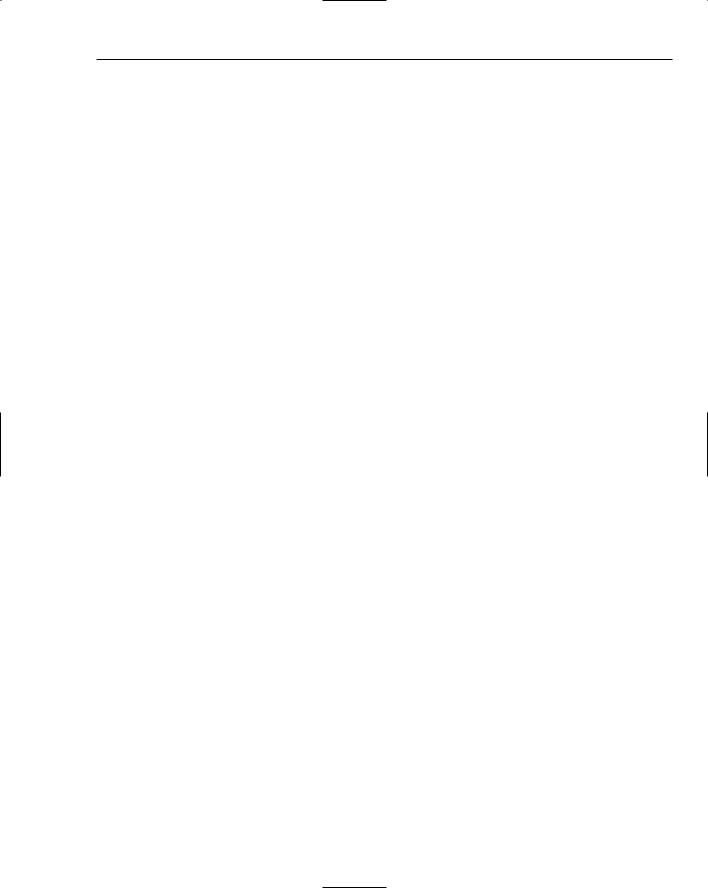
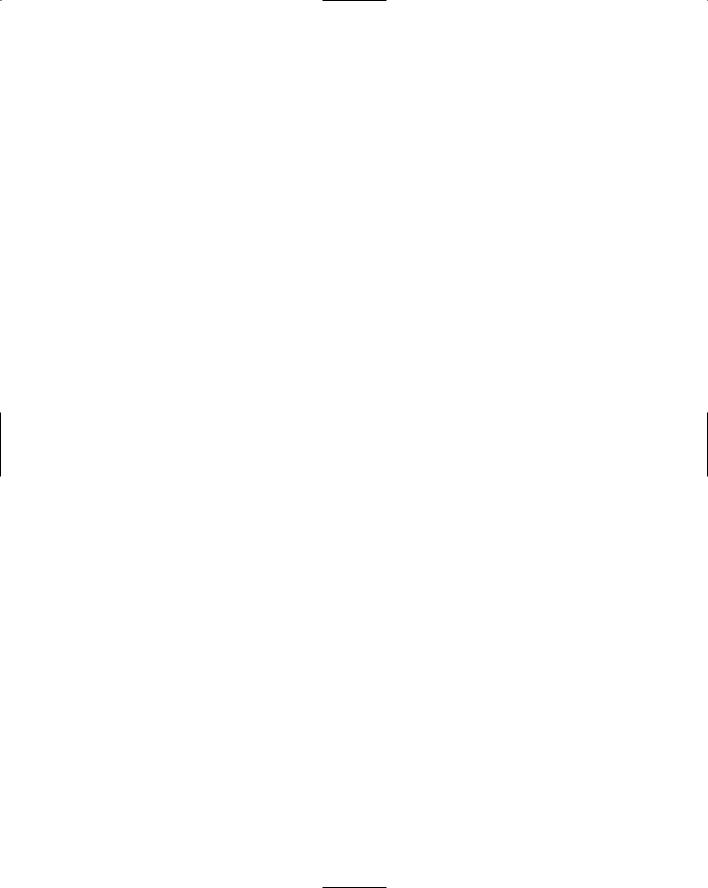
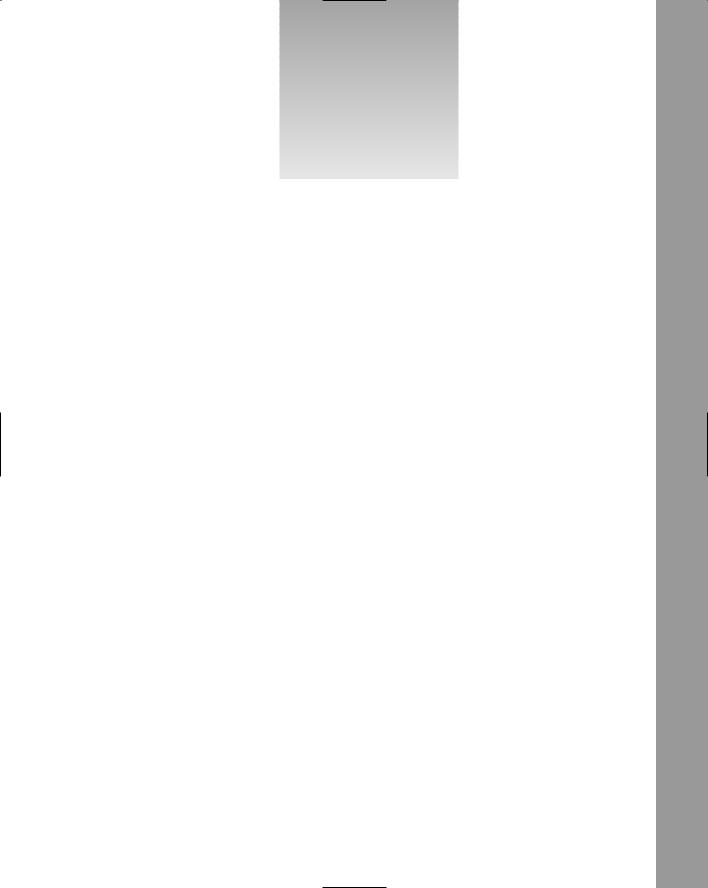
26
Communicating between the Flash Plug-in and the Operating System
The term Flash wrapper is used loosely to describe any environment that’s capable of displaying an
.swf file. The wrapper defines how Flash works with its surroundings. The Macromedia Flash browser plug-in, for example, is a Flash wrapper. A plug-in permits only limited access to the system. The Macromedia Flash Projector wrapper allows for a little bit more communication. Third-party wrappers such as Northcode (www.northcode.com) provide robust ActionScript object additions, allowing the development of sophisticated applications capable of interacting with the system in a much more unrestricted manner. With Northcode, you can save text files, make screen grabs, play external video formats, open web pages directly within Flash, and much more. Macromedia also provides an SDK (Software Development Kit) to enable anyone to include .swf files within larger applications built with languages such as Visual Basic.
One aspect of the Flash player that can help you create a more thorough and stable application is Flash’s capability to obtain information about the system in which it is running. There are times when you may be targeting an array of devices, operating systems, and hardware where the capability to access different aspects of multimedia such as sound, video, and input devices varies from channel to channel. Ascertaining the existence of these system attributes enables you to change the behavior of your application so that it performs at its best, no matter which system it resides on.
Using the System Object
to Retrieve System Information
The System object is a global object that holds properties about the system, player settings, and plugin version. It also has a few useful functions for setting security parameters for the application.
In the following Try It Out, you use a simple loop to display the values available within the System object. You don’t need to do this every time you want to fetch a System value. It’s just a simple way to display all the values available.



