
Beginning ActionScript 2.0 2006
.pdf
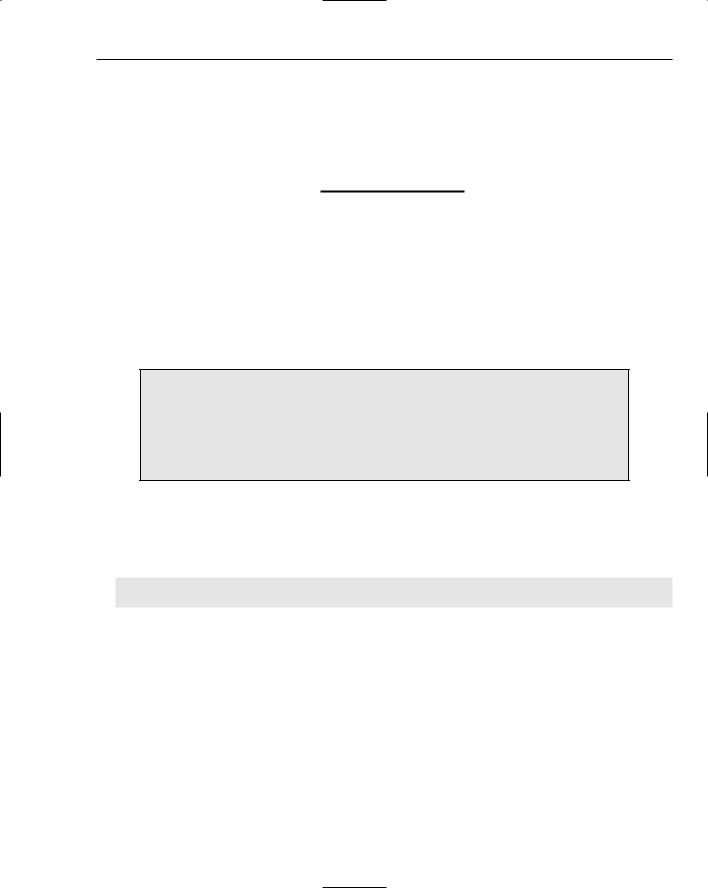


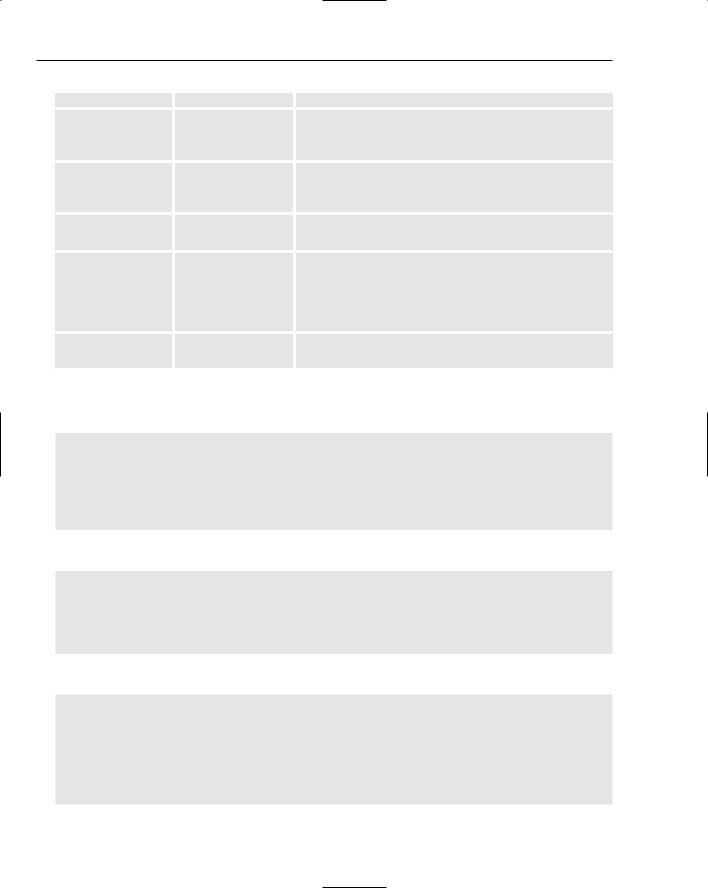

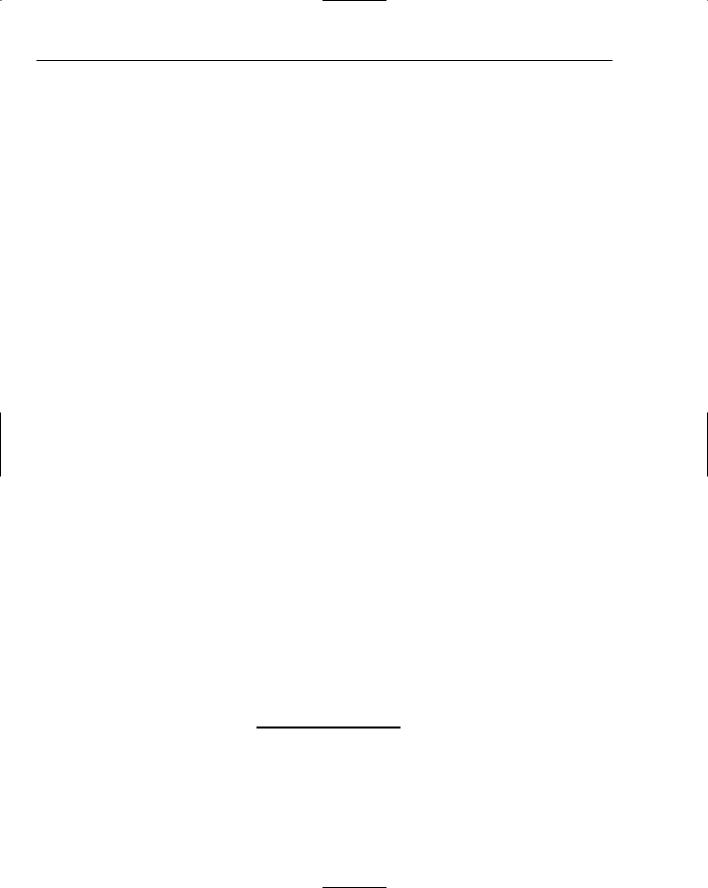
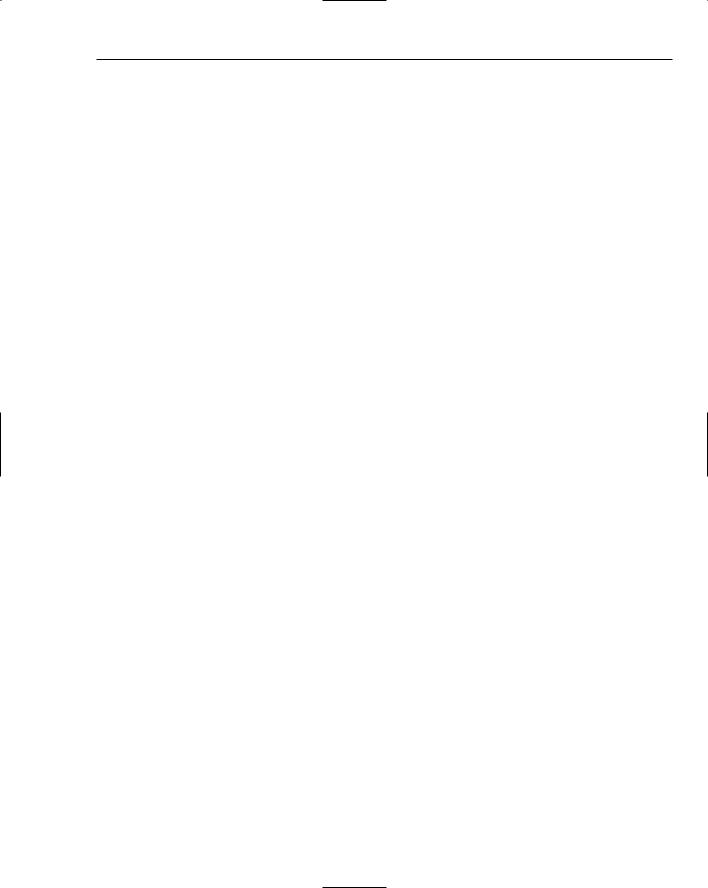
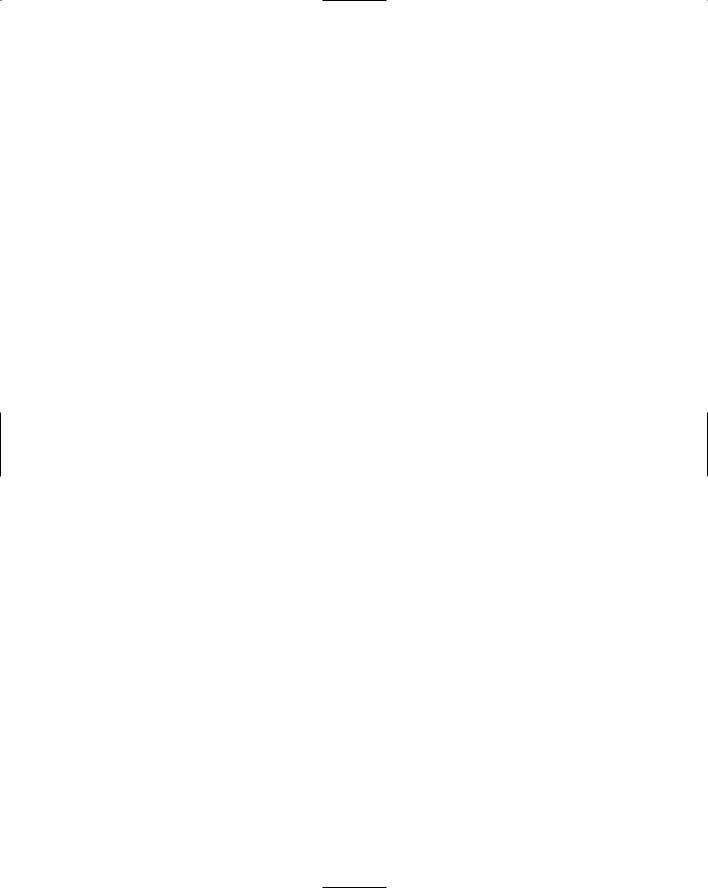

25
Uploading and
Downloading Files
A long-time bane for Flash applications has been the lack of capability to control file uploads and downloads. File uploads were generally performed using a pop-up web page that presented a standard HTML file upload component. Unfortunately, there was no easy way of informing the Flash movie when the upload had completed, progress could not be given, and it offered little control over the process. The main technique for downloading was to use getURL() to invoke the browser’s default file handling behavior for whichever file was to be downloaded. Once again, progress could not be shown, and overall control of the file was handed over to the browser.
Flash 8 introduces the FileReference class, which provides greater control over file uploads and downloads. The class makes use of file open and file save dialog boxes that are native to the operating system. It also provides access to progress and error events, so that better feedback can be given to the user.
FileReference Class Methods
The FileReference class has four methods, which are introduced in the following table:
Method |
Returns |
Description |
|
|
|
browse |
A Boolean |
Prompts the user to select a file to upload. |
cancel |
Nothing |
Cancels any upload or download operation currently in |
|
|
progress. |
download |
A Boolean |
Prompts the user to select a target folder for the file to be |
|
|
downloaded. |
upload |
A Boolean |
Starts the upload of a user-selected file to the server. |