
- •Contents
- •What Is C#?
- •C# Versus Other Programming Languages
- •Preparing to Program
- •The Program Development Cycle
- •Your First C# Program
- •Types of C# Programs
- •Summary
- •Workshop
- •C# Applications
- •Basic Parts of a C# Application
- •Structure of a C# Application
- •Analysis of Listing 2.1
- •Object-Oriented Programming (OOP)
- •Displaying Basic Information
- •Summary
- •Workshop
- •Variables
- •Using Variables
- •Understanding Your Computer’s Memory
- •C# Data Types
- •Numeric Variable Types
- •Literals Versus Variables
- •Constants
- •Reference Types
- •Summary
- •Workshop
- •Types of Operators
- •Punctuators
- •The Basic Assignment Operator
- •Mathematical/Arithmetic Operators
- •Relational Operators
- •Logical Bitwise Operators
- •Type Operators
- •The sizeof Operator
- •The Conditional Operator
- •Understanding Operator Precedence
- •Converting Data Types
- •Understanding Operator Promotion
- •For Those Brave Enough
- •Summary
- •Workshop
- •Controlling Program Flow
- •Using Selection Statements
- •Using Iteration Statements
- •Using goto
- •Nesting Flow
- •Summary
- •Workshop
- •Introduction
- •Abstraction and Encapsulation
- •An Interactive Hello World! Program
- •Basic Elements of Hello.cs
- •A Few Fundamental Observations
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Essential Elements of SimpleCalculator.cs
- •A Closer Look at SimpleCalculator.cs
- •Simplifying Your Code with Methods
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Lexical Structure
- •Some Thoughts on Elevator Simulations
- •Concepts, Goals and Solutions in an Elevator Simulation Program: Collecting Valuable Statistics for Evaluating an Elevator System
- •A Deeper Analysis of SimpleElevatorSimulation.cs
- •Class Relationships and UML
- •Summary
- •Review Questions
- •Programming Exercises
- •The Hello Windows Forms Application
- •Creating and Using an Event Handler
- •Defining the Border Style of the Form
- •Adding a Menu
- •Adding a Menu Shortcut
- •Handling Events from Menus
- •Dialogs
- •Creating Dialogs
- •Using Controls
- •Data Binding Strategies
- •Data Binding Sources
- •Simple Binding
- •Simple Binding to a DataSet
- •Complex Binding of Controls to Data
- •Binding Controls to Databases Using ADO.NET
- •Creating a Database Viewer with Visual Studio and ADO.NET
- •Resources in .NET
- •Localization Nuts and Bolts
- •.NET Resource Management Classes
- •Creating Text Resources
- •Using Visual Studio.NET for Internationalization
- •Image Resources
- •Using Image Lists
- •Programmatic Access to Resources
- •Reading and Writing RESX XML Files
- •The Basic Principles of GDI+
- •The Graphics Object
- •Graphics Coordinates
- •Drawing Lines and Simple Shapes
- •Using Gradient Pens and Brushes
- •Textured Pens and Brushes
- •Tidying up Your Lines with Endcaps
- •Curves and Paths
- •The GraphicsPath Object
- •Clipping with Paths and Regions
- •Transformations
- •Alpha Blending
- •Alpha Blending of Images
- •Other Color Space Manipulations
- •Using the Properties and Property Attributes
- •Demonstration Application: FormPaint.exe
- •Why Use Web Services?
- •Implementing Your First Web Service
- •Testing the Web Service
- •Implementing the Web Service Client
- •Understanding How Web Services Work
- •Summary
- •Workshop
- •How Do Web References Work?
- •What Is UDDI?
- •Summary
- •Workshop
- •Passing Parameters and Web Services
- •Accessing Data with Web Services
- •Summary
- •Workshop
- •Managing State in Web Services
- •Dealing with Slow Services
- •Workshop
- •Creating New Threads
- •Synchronization
- •Summary
- •The String Class
- •The StringBuilder Class
- •String Formatting
- •Regular Expressions
- •Summary
- •Discovering Program Information
- •Dynamically Activating Code
- •Reflection.Emit
- •Summary
- •Simple Debugging
- •Conditional Debugging
- •Runtime Tracing
- •Making Assertions
- •Summary
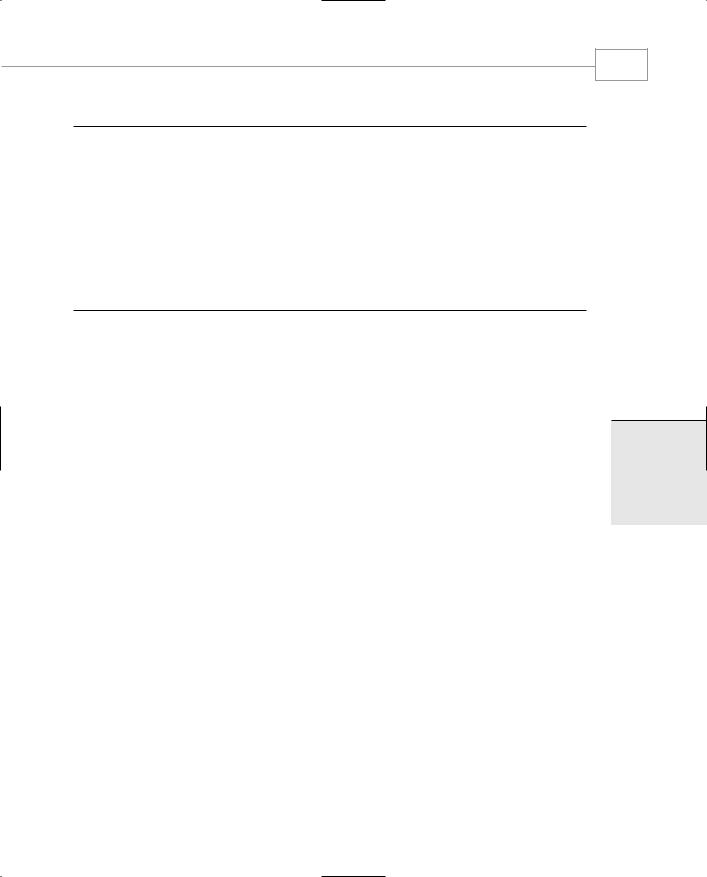
Data Bound Controls
283
CHAPTER 3.3
LISTING 3.3.1 Continued
128:
129:protected void DoneButton_Click (object sender, System.EventArgs e)
130:{
131:Application.Exit();
132:}
133:
134:public static void Main()
135:{
136:Application.Run(new databound());
137:}
138:}
139:}
Compile this program with the command line:
csc t:/winexe databound.cs
The setup of the objects on the form will be familiar to you so we don’t need to re-iterate those principles. The interesting parts are on lines 91–95 which creates and populates a simple StringCollection. Remember that the .NET collections all implement the IList interface, making them candidates for databinding. Lines 98 and 99 perform the actual binding of the data to the textboxes. Finally, the click handlers on lines 114–119 and 122–127 move forward or backward through the data by moving the position, analogous to the cursor in a database, through the data.
Simple Binding to a DataSet
Binding of all sorts of controls is an important feature of .NET. Databound controls are available in Windows Forms, WebForms, and ASP.NET. All these controls can use ADO.NET to bind to database tables either on the local machine or somewhere else on the network. The vehicle for database binding is the DataSet. This class represents a set of cached data that is held in memory. The data cache can be created manually or populated with data drawn from a database using ADO.NET’s database connection system. After the information is in that memory store on your machine, you can bind controls to individual columns of data and select rows for editing or viewing.
The easiest way to understand a DataSet is to use it. Listing 3.3.2 shows an application that generates and manipulates a simple DataSet on your machine using simple binding to controls.
3.3
OUNDB ATAD
ONTROLSC
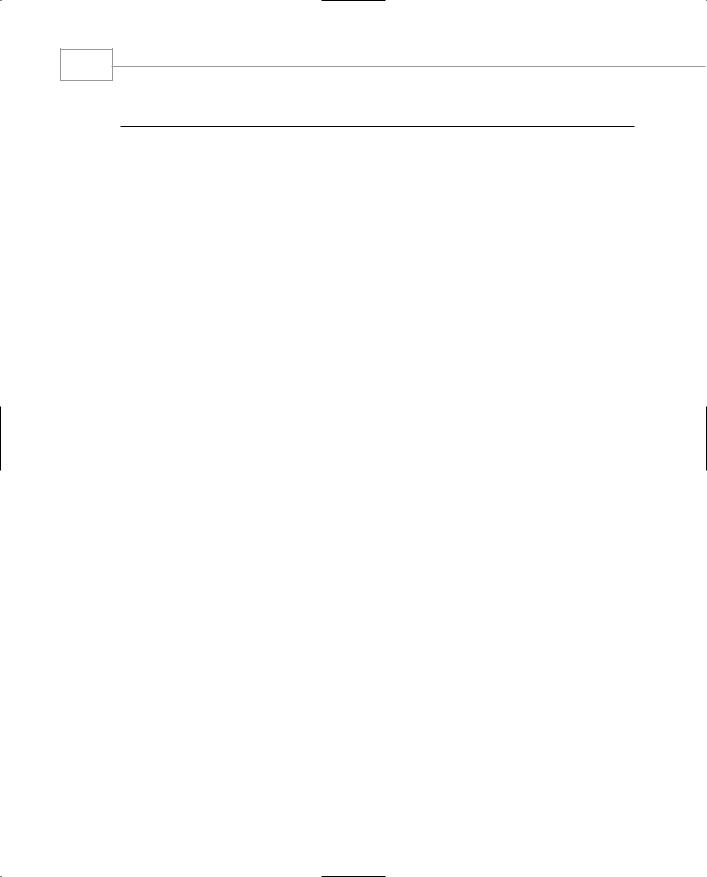
Windows Forms
284
PART III
LISTING 3.3.2 datasetapp.cs: Simple Binding of Multiple Controls
1:namespace Sams
2:{
3:using System;
4:using System.Drawing;
5:using System.Collections;
6:using System.Data;
7:using System.ComponentModel;
8:using System.Windows.Forms;
10://This application shows simple data binding to a
11://programatically created dataset.
12:public class datasetapp : System.Windows.Forms.Form
13:{
14:private System.ComponentModel.Container components;
16://Component declarations
17:private Label lbl_first,lbl_name,lbl_title,lbl_company,lbl_phone;
18:private TextBox FirstName,SurName,Title,Company,Phone;
19:private Button btnNext, btnPrev, btnNew, btnEnd;
20:
21://The dataset used to store the table
22:private DataSet dataset;
23:
24://Button handler to navigate backwards through the table records
25:private void OnPrev(Object sender,EventArgs e)
26:{
27:this.BindingContext[dataset.Tables[“Contacts”]].Position--;
28:}
29:
30://Button handler to navigate forward through the table records
31:private void OnNext(Object sender,EventArgs e)
32:{
33:this.BindingContext[dataset.Tables[“Contacts”]].Position++;
34:}
35:
36://Button handler to create a new row
37:private void OnNew(Object sender, EventArgs e)
38:{
39:NewEntry();
40:}
41:
42://Button handler to exit the application
43:private void OnEnd(Object sender, EventArgs e)
44:{

Data Bound Controls
CHAPTER 3.3
LISTING 3.3.2 Continued
45:Application.Exit();
46:}
47:
48://Method to move to the last record. Used when adding a row.
49:private void MoveToEnd()
50:{
51:this.BindingContext[dataset.Tables[“Contacts”]].Position=
52:dataset.Tables[“Contacts”].Rows.Count-1;
53:}
54:
55://Method to add a new row to the table. Called at initialization
56://and by the “New” button handler.
57:private void NewEntry()
58:{
59:DataRow row = dataset.Tables[“Contacts”].NewRow();
60://set up row data with new entries of your choice
61:row[“First”]=”Blank”;
62:row[“Name”]=””;
63:row[“Company”]=””;
64:row[“Title”]=””;
65:row[“Phone”]=””;
66:dataset.Tables[0].Rows.Add(row);
67:dataset.AcceptChanges();
68:MoveToEnd();
69:}
70:
71://Called at creation to initialize the
72://dataset and create an empty table
73:private void InitDataSet()
74:{
75:dataset = new DataSet(“ContactData”);
77: DataTable t=new DataTable(“Contacts”); 78:
79:t.Columns.Add(“First”,typeof(System.String));
80:t.Columns.Add(“Name”,typeof(System.String));
81:t.Columns.Add(“Company”,typeof(System.String));
82:t.Columns.Add(“Title”,typeof(System.String));
83:t.Columns.Add(“Phone”,typeof(System.String));
85: |
t.MinimumCapacity=100; |
86: |
|
87:dataset.Tables.Add(t);
88:}
285
3.3
OUNDB ATAD
ONTROLSC
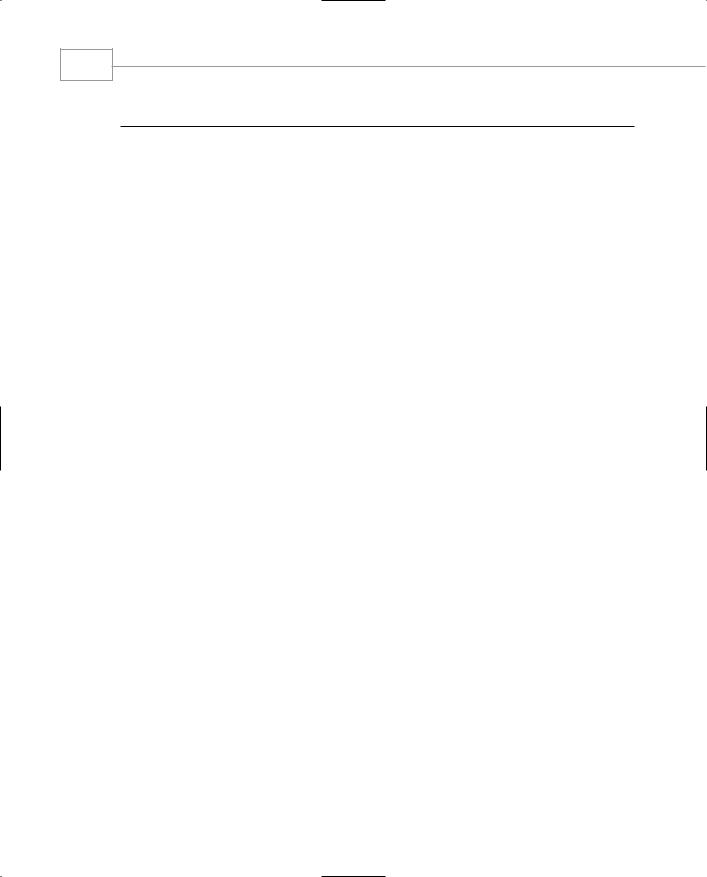
Windows Forms
286
PART III
LISTING 3.3.2 Continued
89:
90://Called at initialization to do simple binding of the edit
91://controls on the form to the dataset’s “Contacts” table entries
92:private void BindControls()
93:{
94:FirstName.DataBindings.Add(“Text”,dataset.Tables[“Contacts”],
”First”);
95:SurName.DataBindings.Add(“Text”,dataset.Tables[“Contacts”],
”Name”);
96:Title.DataBindings.Add(“Text”,dataset.Tables[“Contacts”],
”Title”);
97:Company.DataBindings.Add(“Text”,dataset.Tables[“Contacts”],
”Company”);
98:Phone.DataBindings.Add(“Text”,dataset.Tables[“Contacts”],
”Phone”);
99:}
100:
101://Constructor. Positions the form controls,
102://Ininitializes the dataset, binds the controls and
103://wires up the handlers.
104:public datasetapp()
105:{
106:this.components = new System.ComponentModel.Container ();
107:this.Text = “datasetapp”;
108:this.AutoScaleBaseSize = new System.Drawing.Size (5, 13);
109:this.ClientSize = new System.Drawing.Size (250, 200);
110:this.FormBorderStyle = FormBorderStyle.Fixed3D;
111:
112:lbl_first = new Label();
113:lbl_first.Text=”First name”;
114:lbl_first.Location = new Point(5,5);
115:lbl_first.Size = new Size(120,28);
116:lbl_first.Anchor = AnchorStyles.Left | AnchorStyles.Right;
117:Controls.Add(lbl_first);
118:
119:FirstName = new TextBox();
120:FirstName.Location = new Point(125,5);
121:FirstName.Size = new Size(120,28);
122:FirstName.Anchor = AnchorStyles.Left | AnchorStyles.Right;
123:Controls.Add(FirstName);
124:
125:lbl_name = new Label();
126:lbl_name.Text=”Surname”;
127:lbl_name.Location = new Point(5,35);

Data Bound Controls
CHAPTER 3.3
LISTING 3.3.2 Continued
128:lbl_name.Size = new Size(120,28);
129:lbl_name.Anchor = AnchorStyles.Left|AnchorStyles.Right;
130:Controls.Add(lbl_name);
131:
132:SurName = new TextBox();
133:SurName.Location = new Point(125,35);
134:SurName.Size = new Size(120,28);
135:SurName.Anchor = AnchorStyles.Left | AnchorStyles.Right;
136:Controls.Add(SurName);
137:
138:lbl_company = new Label();
139:lbl_company.Text=”Company”;
140:lbl_company.Location = new Point(5,65);
141:lbl_company.Size = new Size(120,28);
142:Controls.Add(lbl_company);
143:
144:Company = new TextBox();
145:Company.Location = new Point(125,65);
146:Company.Size = new Size(120,28);
147:Controls.Add(Company);
148:
149:lbl_title = new Label();
150:lbl_title.Text=”Title”;
151:lbl_title.Location = new Point(5,95);
152:lbl_title.Size = new Size(120,28);
153:Controls.Add(lbl_title);
154:
155:Title = new TextBox();
156:Title.Location = new Point(125,95);
157:Title.Size = new Size(120,28);
158:Controls.Add(Title);
159:
160:lbl_phone = new Label();
161:lbl_phone.Text=”Telephone”;
162:lbl_phone.Location = new Point(5,125);
163:lbl_phone.Size = new Size(120,28);
164:Controls.Add(lbl_phone);
165:
166:Phone = new TextBox();
167:Phone.Location = new Point(125,125);
168:Phone.Size = new Size(120,28);
169:Controls.Add(Phone);
170:
171: btnNew = new Button();
287
3.3
OUNDB ATAD
ONTROLSC
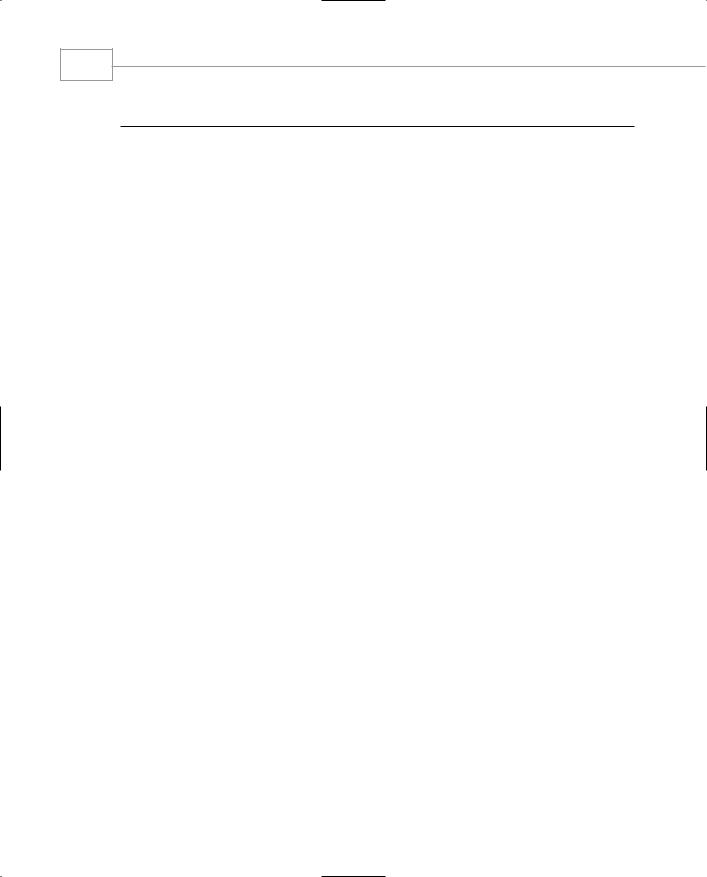
Windows Forms
288
PART III
LISTING 3.3.2 Continued
172:btnNew.Location = new Point(5,155);
173:btnNew.Size = new Size(70,28);
174:btnNew.Text=”New”;
175:btnNew.Click+=new EventHandler(OnNew);
176:Controls.Add(btnNew);
177:
178:btnPrev = new Button();
179:btnPrev.Location = new Point(80,155);
180:btnPrev.Size = new Size(35,28);
181:btnPrev.Text=”<<”;
182:btnPrev.Click += new EventHandler(OnPrev);
183:Controls.Add(btnPrev);
184:
185:btnEnd = new Button();
186:btnEnd.Location = new Point(120,155);
187:btnEnd.Size = new Size(70,28);
188:btnEnd.Text=”End”;
189:btnEnd.Click += new EventHandler(OnEnd);
190:Controls.Add(btnEnd);
191:
192:btnNext = new Button();
193:btnNext.Location = new Point(200,155);
194:btnNext.Size = new Size(35,28);
195:btnNext.Text=”>>”;
196:btnNext.Click += new EventHandler(OnNext);
197:Controls.Add(btnNext);
198: |
|
199: |
InitDataSet(); |
200: |
|
201: |
NewEntry(); |
202: |
|
203: |
BindControls(); |
204: |
|
205: |
} |
206: |
|
207://Cleans up the Form
208:public override void Dispose()
209:{
210:base.Dispose();
211:components.Dispose();
212:}
213:
214://Main method to instantiate and run the application.
215:static void Main()