
- •Contents
- •What Is C#?
- •C# Versus Other Programming Languages
- •Preparing to Program
- •The Program Development Cycle
- •Your First C# Program
- •Types of C# Programs
- •Summary
- •Workshop
- •C# Applications
- •Basic Parts of a C# Application
- •Structure of a C# Application
- •Analysis of Listing 2.1
- •Object-Oriented Programming (OOP)
- •Displaying Basic Information
- •Summary
- •Workshop
- •Variables
- •Using Variables
- •Understanding Your Computer’s Memory
- •C# Data Types
- •Numeric Variable Types
- •Literals Versus Variables
- •Constants
- •Reference Types
- •Summary
- •Workshop
- •Types of Operators
- •Punctuators
- •The Basic Assignment Operator
- •Mathematical/Arithmetic Operators
- •Relational Operators
- •Logical Bitwise Operators
- •Type Operators
- •The sizeof Operator
- •The Conditional Operator
- •Understanding Operator Precedence
- •Converting Data Types
- •Understanding Operator Promotion
- •For Those Brave Enough
- •Summary
- •Workshop
- •Controlling Program Flow
- •Using Selection Statements
- •Using Iteration Statements
- •Using goto
- •Nesting Flow
- •Summary
- •Workshop
- •Introduction
- •Abstraction and Encapsulation
- •An Interactive Hello World! Program
- •Basic Elements of Hello.cs
- •A Few Fundamental Observations
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Essential Elements of SimpleCalculator.cs
- •A Closer Look at SimpleCalculator.cs
- •Simplifying Your Code with Methods
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Lexical Structure
- •Some Thoughts on Elevator Simulations
- •Concepts, Goals and Solutions in an Elevator Simulation Program: Collecting Valuable Statistics for Evaluating an Elevator System
- •A Deeper Analysis of SimpleElevatorSimulation.cs
- •Class Relationships and UML
- •Summary
- •Review Questions
- •Programming Exercises
- •The Hello Windows Forms Application
- •Creating and Using an Event Handler
- •Defining the Border Style of the Form
- •Adding a Menu
- •Adding a Menu Shortcut
- •Handling Events from Menus
- •Dialogs
- •Creating Dialogs
- •Using Controls
- •Data Binding Strategies
- •Data Binding Sources
- •Simple Binding
- •Simple Binding to a DataSet
- •Complex Binding of Controls to Data
- •Binding Controls to Databases Using ADO.NET
- •Creating a Database Viewer with Visual Studio and ADO.NET
- •Resources in .NET
- •Localization Nuts and Bolts
- •.NET Resource Management Classes
- •Creating Text Resources
- •Using Visual Studio.NET for Internationalization
- •Image Resources
- •Using Image Lists
- •Programmatic Access to Resources
- •Reading and Writing RESX XML Files
- •The Basic Principles of GDI+
- •The Graphics Object
- •Graphics Coordinates
- •Drawing Lines and Simple Shapes
- •Using Gradient Pens and Brushes
- •Textured Pens and Brushes
- •Tidying up Your Lines with Endcaps
- •Curves and Paths
- •The GraphicsPath Object
- •Clipping with Paths and Regions
- •Transformations
- •Alpha Blending
- •Alpha Blending of Images
- •Other Color Space Manipulations
- •Using the Properties and Property Attributes
- •Demonstration Application: FormPaint.exe
- •Why Use Web Services?
- •Implementing Your First Web Service
- •Testing the Web Service
- •Implementing the Web Service Client
- •Understanding How Web Services Work
- •Summary
- •Workshop
- •How Do Web References Work?
- •What Is UDDI?
- •Summary
- •Workshop
- •Passing Parameters and Web Services
- •Accessing Data with Web Services
- •Summary
- •Workshop
- •Managing State in Web Services
- •Dealing with Slow Services
- •Workshop
- •Creating New Threads
- •Synchronization
- •Summary
- •The String Class
- •The StringBuilder Class
- •String Formatting
- •Regular Expressions
- •Summary
- •Discovering Program Information
- •Dynamically Activating Code
- •Reflection.Emit
- •Summary
- •Simple Debugging
- •Conditional Debugging
- •Runtime Tracing
- •Making Assertions
- •Summary
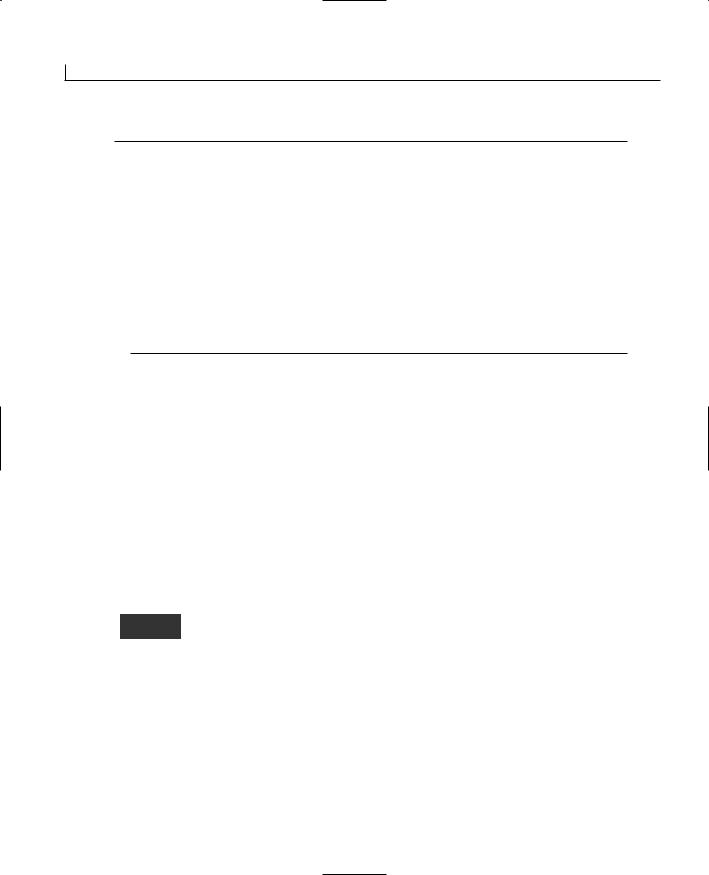
106 |
Day 4 |
LISTING 4.7 continued
41:
42:NewVal = ValZero | ValZero;
43:System.Console.WriteLine(“\nThe OR Operator: \n 0 | 0 = {0}”,
NewVal);
45:NewVal = ValZero | ValOne;
46:System.Console.WriteLine(“ 0 | 1 = {0}”, NewVal);
48:NewVal = ValOne | ValZero;
49:System.Console.WriteLine(“ 1 | 0 = {0}”, NewVal);
51:NewVal = ValOne | ValOne;
52:System.Console.WriteLine(“ 1 | 1 = {0}”, NewVal);
53:}
54:}
OUTPUT |
The |
NOT Operator: |
|
0 |
^ 0 = 0 |
||
|
0 |
^ 1 = 1 |
|
|
1 |
^ 0 = 1 |
|
|
1 |
^ 1 = 0 |
|
|
The |
AND Operator: |
|
|
0 |
& 0 = 0 |
|
|
0 |
& 1 = 0 |
|
|
1 |
& 0 = 0 |
|
|
1 |
& 1 = 1 |
|
|
The |
OR Operator: |
|
|
0 |
| 0 |
= 0 |
|
0 |
| 1 |
= 1 |
|
1 |
| 0 |
= 1 |
|
1 |
| 1 |
= 1 |
Listing 4.7 is a long listing; however, it summarizes the logical bitwise operators. Lines 8 and 9 define two variables and assign the values 1 and 0 to them. These
two variables are then used repeatedly with the bitwise operators. A bitwise operation is done, and then the result is written to the console. You should review the output and see that the results are exactly as described in the earlier sections.
Summary
Today’s lesson presents a lot of information regarding operators and their use. You have learned about the types of operators, including arithmetic, multiplicative, relational, logical, and conditional. You also learned the order in which operators are evaluated

Working with Operators |
107 |
(operator precedence). Finally, today’s lesson ended with an explanation of bitwise operations and the bitwise operators.
Q&A
Q How important is it to understand operators and operator precedence?
AYou will use the operators in almost every application you create. Operator precedence is critical to understand. As you saw in today’s lesson, if you don’t understand operator precedence, you might end up with results different from what you expect.
QToday’s lesson covered the binary number system briefly. Is it important to understand this number system? Also, what other number systems are i mportant?
AAlthough it is not critical to understand binary, it is important. With computers today, information is stored in a binary format. Whether it is a positive versus negative charge, a bump versus an impression, or some other representation, all data is ultimately stored in binary. Knowing how the binary system works will
make it easier for you to understand these actual storage values. |
4 |
|
In addition to binary, many computer programmers also work with octal and |
||
|
||
hexadecimal. Octal is a base 8 number system and hexadecimal is a base 16 num- |
|
|
ber system. Appendix D, “Understanding Number Systems,” covers this in more |
|
|
detail. |
|
Workshop
The Workshop provides quiz questions to help you solidify your understanding of the material covered and exercises to provide you with experience in using what you’ve learned. Try to understand the quiz and exercise answers before continuing to the next day’s lesson. Answers are provided in Appendix A, “Answers.”
Quiz
The following quiz questions will help verify your understanding of today’s lessons. 1. What character is used for multiplication?
2. What is the result of 10 % 3?
3. What is the result of 10 + 3 * 2?
4. What are the conditional operators?
5. What C# keyword can be used to change the flow of a program?
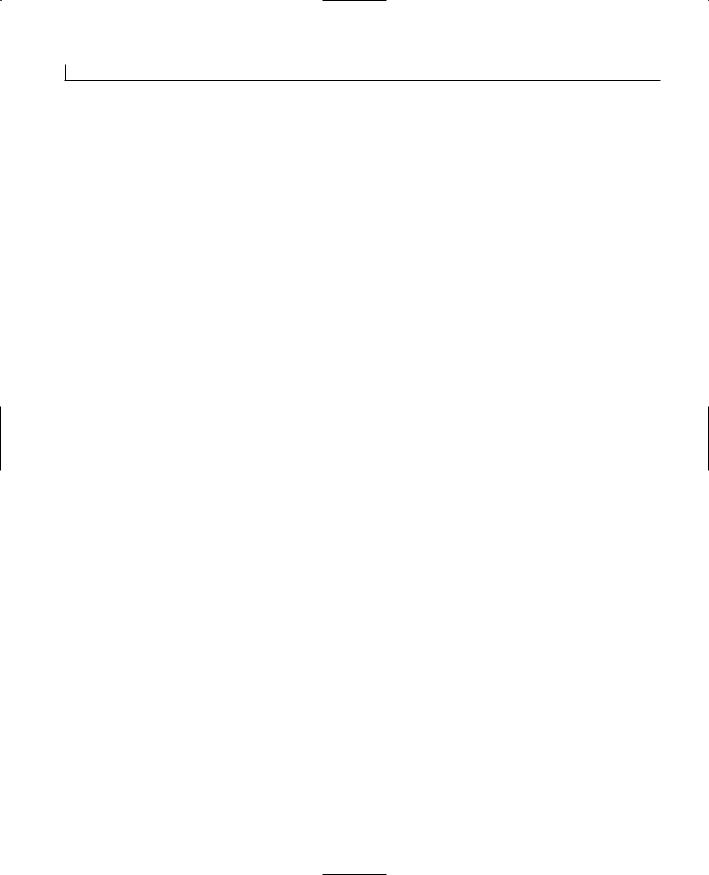
108 |
Day 4 |
6.What is the difference between a unary operator and a binary operator?
7.What is the difference between an explicit data type conversion versus an implicit conversion?
8.Is it possible to convert from a long to an integer?
9.What are the possible results of a conditional operation?
10.What do the shift operators do?
Exercises
Please note that answers will not be provided for all exercises. The exercises will help you apply what you have learned in today’s lessons.
1.What is the result of the following operation? 2 + 6 * 3 + 5 – 2 *4
2.What is the result of the following operation? 4 * (8 – 3 * 2) * (0 + 1) / 2
3Write a program that checks to see whether a variable is greater than 65. If the value is greater than 65, print the statement “The value is greater than 65!”.
4.Write a program that checks to see whether a character contains the value of ‘t’ or ‘T’.
5.Write the line of code to convert a long called MyLong to a short called MyShort.
6.BUG BUSTER: The following program has a problem. Enter it in your editor and compile it. Which lines generate error messages? What is the error?
1:class exercise
2:{
3:static void Main()
4:{
5:int value = 1;
6:
7:if ( value > 100 );
8:{
9:System.Console.WriteLine(“Number is greater than 100”);
10:}
11:}
12:}
7.Write the line of code to convert an integer, IntVal, to a short, ShortVal.
8.Write the line of code to convert a decimal, DecVal, to a long, LongVal.
9.Write the line of code to convert an integer, ch, to a character, charVal.
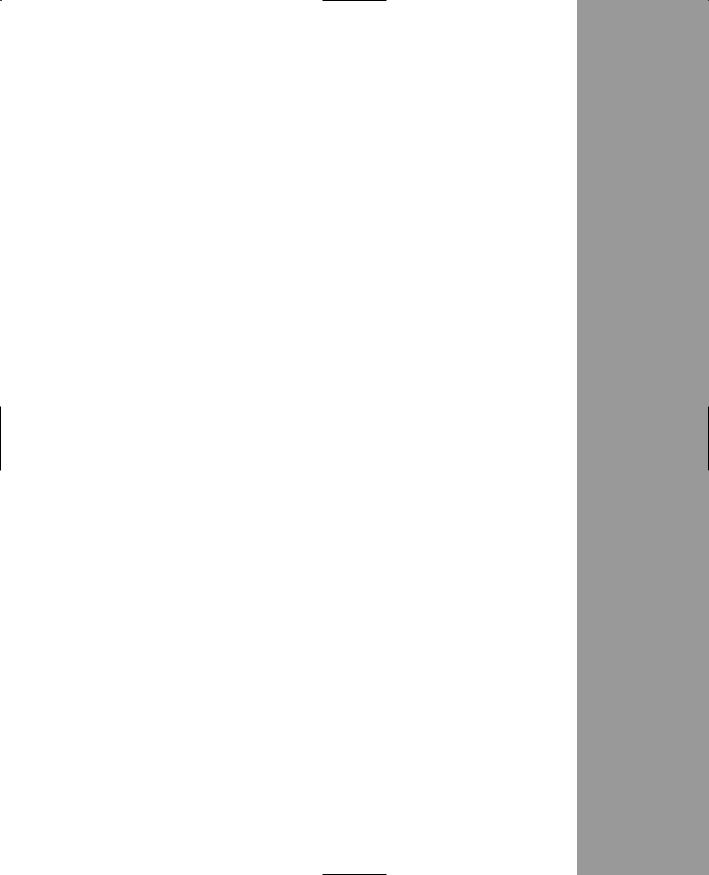
WEEK 1
DAY 5
Control Statements
You’ve learned a lot in the previous four days. This includes knowing how to store information, knowing how to do operations, and even knowing how to avoid executing certain commands by using the if statement. You have learned a little about controlling the flow of a program using the if statement; however, there are often times where you need to be able to control the flow of a program even more. Today you
•See the other commands to use for program flow control
•Explore how to do even more with the if command
•Learn to switch between multiple options
•Investigate how to repeat a block of statements multiple times
•Discover how to abruptly stop the repeating of code
Controlling Program Flow
By controlling the flow of a program, you are able to create functionality that results in something useful. As you continue to program, you will want to