
- •Contents
- •What Is C#?
- •C# Versus Other Programming Languages
- •Preparing to Program
- •The Program Development Cycle
- •Your First C# Program
- •Types of C# Programs
- •Summary
- •Workshop
- •C# Applications
- •Basic Parts of a C# Application
- •Structure of a C# Application
- •Analysis of Listing 2.1
- •Object-Oriented Programming (OOP)
- •Displaying Basic Information
- •Summary
- •Workshop
- •Variables
- •Using Variables
- •Understanding Your Computer’s Memory
- •C# Data Types
- •Numeric Variable Types
- •Literals Versus Variables
- •Constants
- •Reference Types
- •Summary
- •Workshop
- •Types of Operators
- •Punctuators
- •The Basic Assignment Operator
- •Mathematical/Arithmetic Operators
- •Relational Operators
- •Logical Bitwise Operators
- •Type Operators
- •The sizeof Operator
- •The Conditional Operator
- •Understanding Operator Precedence
- •Converting Data Types
- •Understanding Operator Promotion
- •For Those Brave Enough
- •Summary
- •Workshop
- •Controlling Program Flow
- •Using Selection Statements
- •Using Iteration Statements
- •Using goto
- •Nesting Flow
- •Summary
- •Workshop
- •Introduction
- •Abstraction and Encapsulation
- •An Interactive Hello World! Program
- •Basic Elements of Hello.cs
- •A Few Fundamental Observations
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Essential Elements of SimpleCalculator.cs
- •A Closer Look at SimpleCalculator.cs
- •Simplifying Your Code with Methods
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Lexical Structure
- •Some Thoughts on Elevator Simulations
- •Concepts, Goals and Solutions in an Elevator Simulation Program: Collecting Valuable Statistics for Evaluating an Elevator System
- •A Deeper Analysis of SimpleElevatorSimulation.cs
- •Class Relationships and UML
- •Summary
- •Review Questions
- •Programming Exercises
- •The Hello Windows Forms Application
- •Creating and Using an Event Handler
- •Defining the Border Style of the Form
- •Adding a Menu
- •Adding a Menu Shortcut
- •Handling Events from Menus
- •Dialogs
- •Creating Dialogs
- •Using Controls
- •Data Binding Strategies
- •Data Binding Sources
- •Simple Binding
- •Simple Binding to a DataSet
- •Complex Binding of Controls to Data
- •Binding Controls to Databases Using ADO.NET
- •Creating a Database Viewer with Visual Studio and ADO.NET
- •Resources in .NET
- •Localization Nuts and Bolts
- •.NET Resource Management Classes
- •Creating Text Resources
- •Using Visual Studio.NET for Internationalization
- •Image Resources
- •Using Image Lists
- •Programmatic Access to Resources
- •Reading and Writing RESX XML Files
- •The Basic Principles of GDI+
- •The Graphics Object
- •Graphics Coordinates
- •Drawing Lines and Simple Shapes
- •Using Gradient Pens and Brushes
- •Textured Pens and Brushes
- •Tidying up Your Lines with Endcaps
- •Curves and Paths
- •The GraphicsPath Object
- •Clipping with Paths and Regions
- •Transformations
- •Alpha Blending
- •Alpha Blending of Images
- •Other Color Space Manipulations
- •Using the Properties and Property Attributes
- •Demonstration Application: FormPaint.exe
- •Why Use Web Services?
- •Implementing Your First Web Service
- •Testing the Web Service
- •Implementing the Web Service Client
- •Understanding How Web Services Work
- •Summary
- •Workshop
- •How Do Web References Work?
- •What Is UDDI?
- •Summary
- •Workshop
- •Passing Parameters and Web Services
- •Accessing Data with Web Services
- •Summary
- •Workshop
- •Managing State in Web Services
- •Dealing with Slow Services
- •Workshop
- •Creating New Threads
- •Synchronization
- •Summary
- •The String Class
- •The StringBuilder Class
- •String Formatting
- •Regular Expressions
- •Summary
- •Discovering Program Information
- •Dynamically Activating Code
- •Reflection.Emit
- •Summary
- •Simple Debugging
- •Conditional Debugging
- •Runtime Tracing
- •Making Assertions
- •Summary
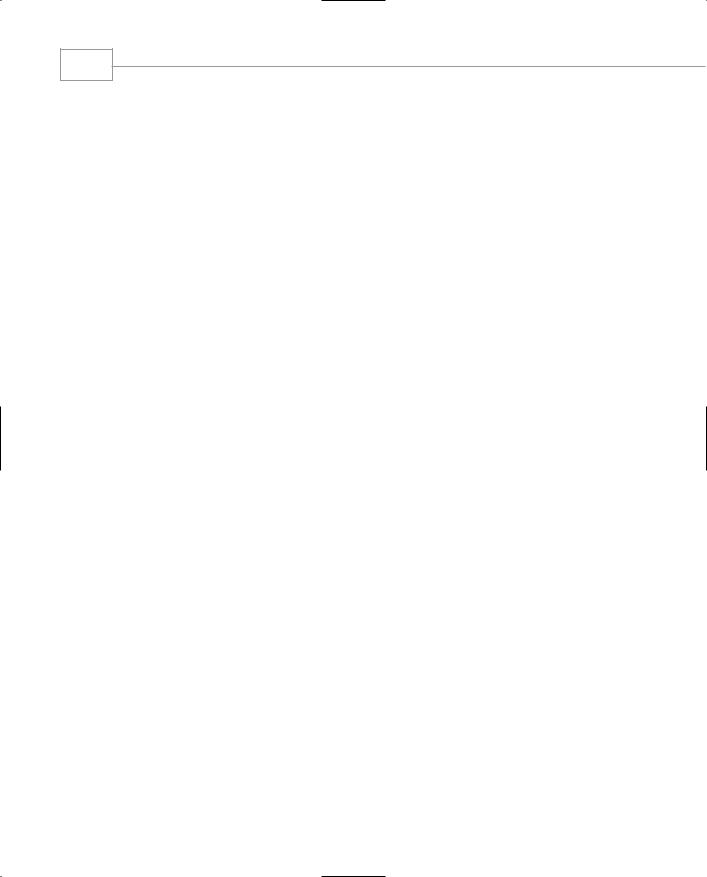
Windows Forms
278
PART III
In the previous chapter, we looked at some of the more common user interface elements and how to place and interact with them. The user interfaces presented in Windows Forms are not so different from the familiar MFC ones with which we are familiar. However, the MFC controls can require a lot of programming to make them useful. Some of the Windows Forms elements, such as the TreeView and ListView controls, are very complex and look as if they require a lot of coding to populate and use. This is not always the case, especially when controls are tied to database tables through data binding.
Data Binding Strategies
Data is generally held in tables that have one or more rows and one or more columns. Data Binding is the technique of tying user interface controls directly to individual elements, whole rows, whole columns, or entire tables for the purpose of viewing, manipulating, or editing them.
There are two types of data binding. Simple Binding ties a simple data viewer such as a text box or a numeric edit box to a single data item in a data table. Complex Binding presents a view of a whole table or many tables.
Data Binding Sources
Suitable sources for data binding include any object that exposes the IList interface. Most of the .NET collections implement this interface. Data objects provided by ADO.NET also expose Ilist, and you can also write your own objects that expose data through this interface.
The IList Interface
The Ilist interface has only a few methods. They are as follows:
•Add adds an item to the list.
•Clear removes all items from a list.
•Contains searches a list for a certain value.
•IndexOf determines the index of a particular value within the list.
•Insert places a value into the list before a given index. This moves all following indexes
up one and makes the list longer.
•Remove takes an item out of the list.
•RemoveAt removes one item from a specific index.
Accessing items within the list is done by index. For example, Item x = MyList[n] items are assumed to be objects so any data can be stored in a list if it’s a boxed value type or if it’s a
.NET object.
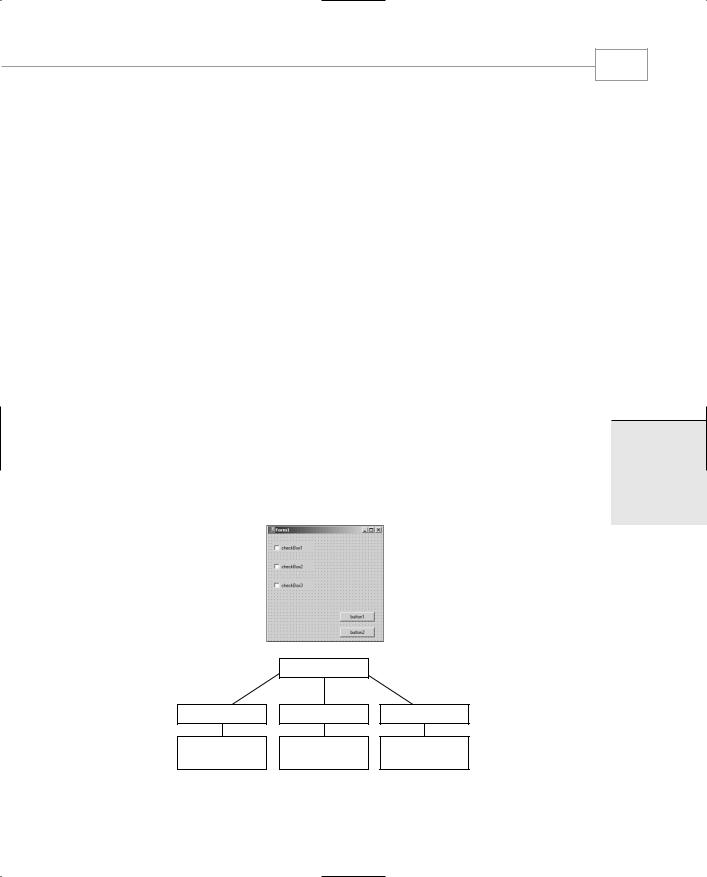
Data Bound Controls
CHAPTER 3.3
Some .NET Objects That Implement IList
There are a lot of classes in the framework that implement IList. Some of them are just for accessing data. The Array, ArrayList, and StringCollection classes are very commonly used. More complex classes include DataSetView and DataView, which work in conjunction with ADO to provide data table access.
The less mundane classes that support the interface are used extensively by the .NET developer tools for looking at the metadata associated with classes. These include the
CodeClassCollection and the CodeStatementCollection classes and other objects that use the code Document Object Model or DOM.
Simple Binding
As previously mentioned, simple binding is the business of taking a simple chunk of data and tying it to a user control. When the data changes, the control reflects that change. When the control’s display is edited, the data changes if write access is enabled.
Each Windows Forms control maintains a BindingContext object that, in turn, has a collection of CurrencyManager objects. By the way, the CurrencyManager has nothing to do with financial exchanges. It simply maintains a current position within a particular data object for you. Child controls, such as the GroupBox, can also have their own independent BindingContexts and CurrencyManagers.
The illustration in Figure 3.3.1 shows the relationships between Form, Control, BindingContext and CurrencyManager.
|
BindingContext |
|
CurrencyManager |
CurrencyManager |
CurrencyManager |
System.Collection |
System.Array |
ADO.NET data |
based objects. |
based objects. |
objects |
FIGURE 3.3.1
279
3.3
OUNDB ATAD
ONTROLSC
The relationship between form, BindingManager, and CurrencyManager.
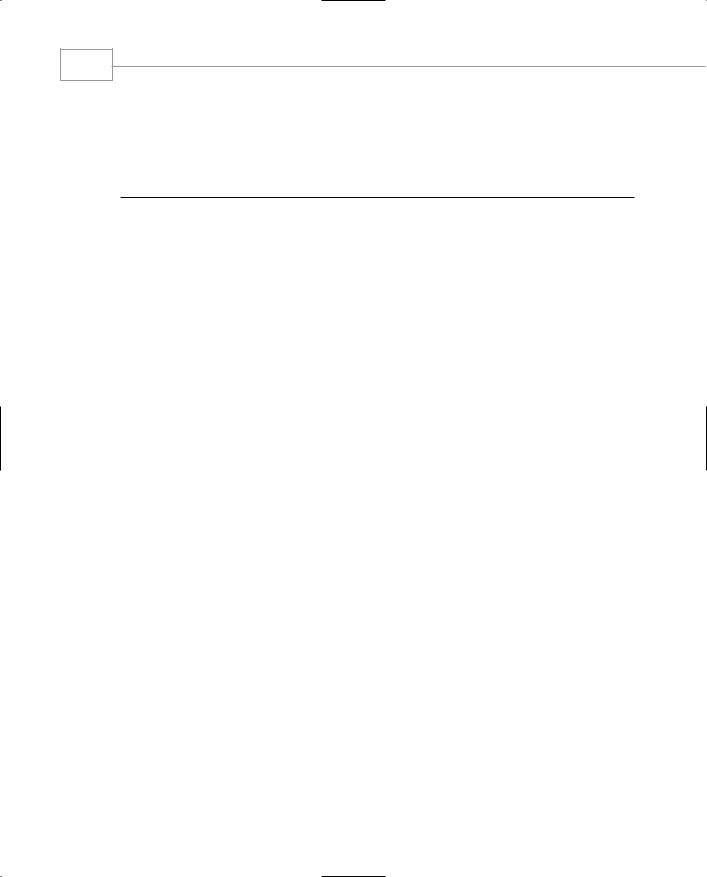
Windows Forms
280
PART III
Let’s take a look at using the BindingManager and CurrencyManager with a simple application that binds a text box’s Text property to a collection of strings. Listing 3.3.1 shows the DataBound application source code.
LISTING 3.3.1 databound.cs: Simple Binding of Controls to Data
1:namespace Sams
2:{
3:using System;
4:using System.Drawing;
5:using System.Collections;
6:using System.Collections.Specialized;
7:using System.ComponentModel;
8:using System.Windows.Forms;
9:
10:/// <summary>
11:/// Summary description for databound.
12:/// </summary>
13:public class databound : System.Windows.Forms.Form
14:{
15:private Button RightButton;
16:private Button LeftButton;
17:private TextBox textBox2;
18:private TextBox textBox1;
19:private Button DoneButton;
20:
21: private StringCollection sa; 22:
23:public databound()
24:{
25:this.textBox2 = new TextBox();
26:this.RightButton = new Button();
27:this.textBox1 = new TextBox();
28:this.DoneButton = new Button();
29:this.LeftButton = new Button();
30:this.SuspendLayout();
31://
32:// textBox2
33://
34:this.textBox2.Location = new Point(184, 16);
35:this.textBox2.Name = “textBox2”;
36:this.textBox2.TabIndex = 2;
37:this.textBox2.Text = “textBox2”;
38://
39:// RightButton
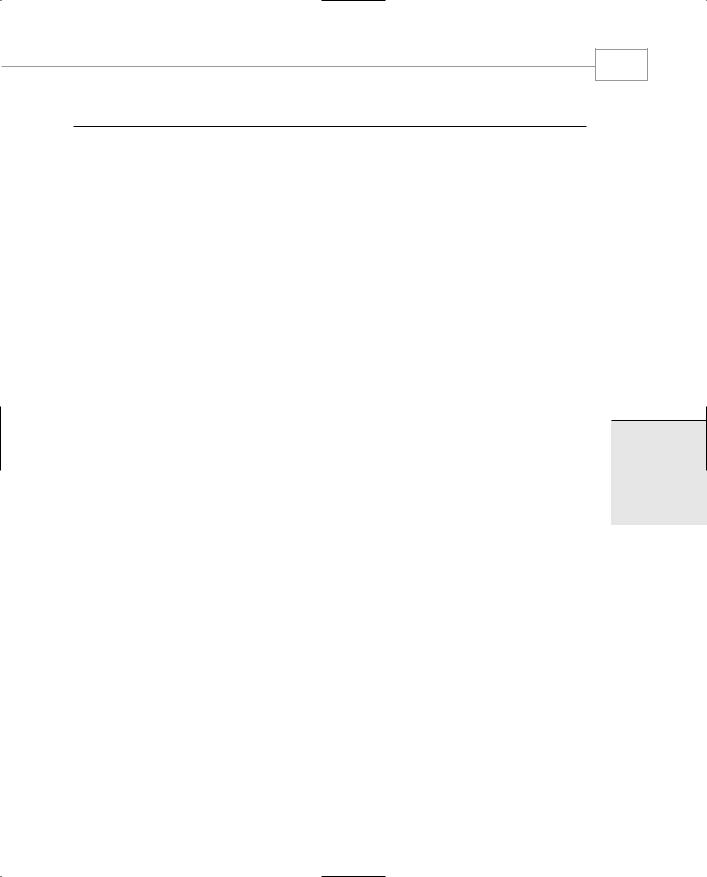
Data Bound Controls
CHAPTER 3.3
LISTING 3.3.1 Continued
40://
41:this.RightButton.Location = new Point(192, 64);
42:this.RightButton.Name = “RightButton”;
43:this.RightButton.TabIndex = 4;
44:this.RightButton.Text = “>>”;
45:this.RightButton.Click += new EventHandler(this.RightButton_Click);
46://
47:// textBox1
48://
49:this.textBox1.Location = new Point(8, 16);
50:this.textBox1.Name = “textBox1”;
51:this.textBox1.TabIndex = 1;
52:this.textBox1.Text = “textBox1”;
53://
54:// DoneButton
55://
56:this.DoneButton.Location = new Point(104, 64);
57:this.DoneButton.Name = “DoneButton”;
58:this.DoneButton.TabIndex = 0;
59:this.DoneButton.Text = “Done”;
60:this.DoneButton.Click += new EventHandler(this.DoneButton_Click);
61://
62:// LeftButton
63://
64:this.LeftButton.Location = new Point(16, 64);
65:this.LeftButton.Name = “LeftButton”;
66:this.LeftButton.TabIndex = 3;
67:this.LeftButton.Text = “<<”;
68:this.LeftButton.Click += new EventHandler(this.LeftButton_Click);
69://
70:// databound
71://
72:this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
73:this.ClientSize = new System.Drawing.Size(294, 111);
74:this.ControlBox = false;
75:this.Controls.AddRange(new Control[] {
76: this.RightButton, 77: this.LeftButton, 78: this.textBox2, 79: this.textBox1,
80: this.DoneButton});
81:this.FormBorderStyle = FormBorderStyle.FixedDialog;
82:this.Name = “databound”;
83:this.ShowInTaskbar = false;
281
3.3
OUNDB ATAD
ONTROLSC
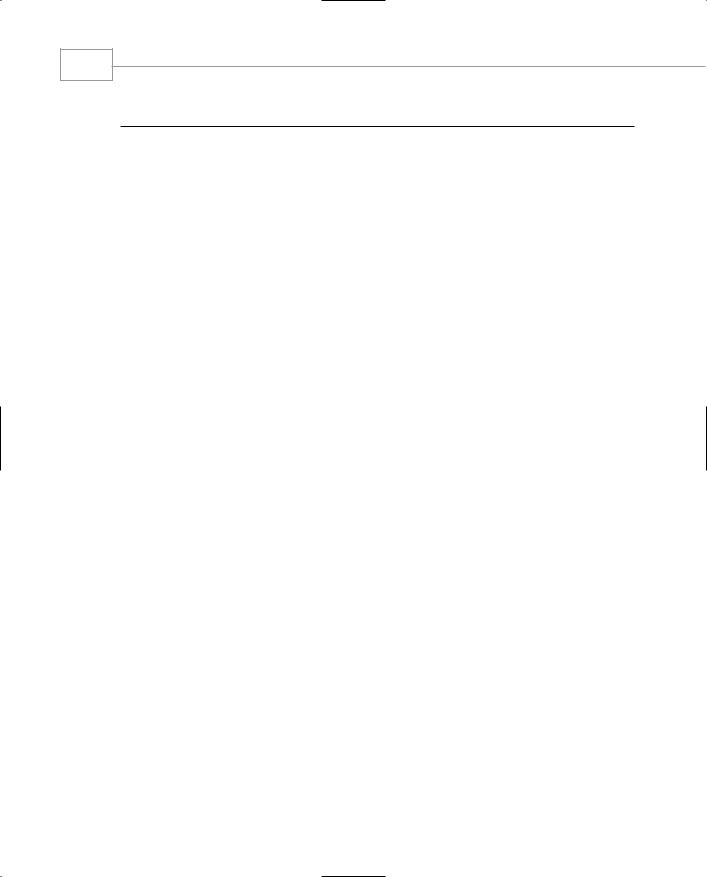
Windows Forms
282
PART III
LISTING 3.3.1 Continued
84:this.Text = “databound”;
85:this.ResumeLayout(false);
87://This is the setup code for the simple binding example
88://We have bound two text controls to the same StringCollection
90:// This is setting up the “database” (an IList thing)
91:sa=new StringCollection();
92:sa.Add(“Hello databinding”);
93:sa.Add(“Sams publishing”);
94:sa.Add(“C# example”);
95:sa.Add(“By Bob Powell”);
96:
97://This binds the controls to that database.
98:this.textBox1.DataBindings.Add(“Text”,sa,””);
99:this.textBox2.DataBindings.Add(“Text”,sa,””);
100://See the button handlers below for details
101://on how to move through the data.
102:}
103:
104:/// <summary>
105:/// Clean up any resources being used.
106:/// </summary>
107:public override void Dispose()
108:{
109:base.Dispose();
110:}
111:
112:
113://Very simply increments the Position property of the CurrencyManager
114:protected void RightButton_Click (object sender, System.EventArgs e)
115:{
116://Note how updating one position affects all
117://controls bound to this data
118:this.BindingContext[sa].Position++;
119:}
120:
121://Very simply decrements the Position property of the CurrencyManager
122:protected void LeftButton_Click (object sender, System.EventArgs e)
123:{
124://Note how updating one position affects all
125://controls bound to this data
126:this.BindingContext[sa].Position--;
127:}