
Microsoft ASP .NET Professional Projects - Premier Press
.pdf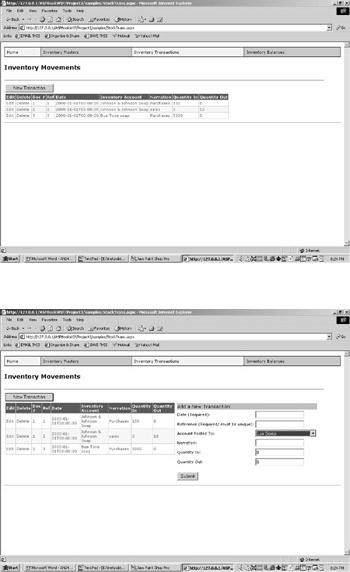
Inventory Transactions
Inventory Transaction maintenance involves adding, modifying, and deleting transactions. The following objects are involved in this Sub system:
1.The Inventory Transactions web form (StockTrans.aspx) and the CodeBehind form (StockTrans.vb)
2.The stored procedure (p_stock_trans)
The Inventory Transactions Form
The Inventory Transactions web form enables users to add and modify inventory transaction records. The add functionality is provided by TextBox controls, residing on a panel that is made visible when the Add button is clicked. A DataGrid implements the modify functionality. This form is quite similar to the stock_masters form that we built in Chapter 24, "Inventory Masters." The DataGrid and the Add portion of the form are set up as described in that chapter.
Figure 25.1 shows what the form looks like.
Figure 25.1: The Inventory Transactions form.
Figure 25.2 shows the Inventory Transactions Form in Add mode. Figure 25.3 shows the Inventory Transactions Form in Edit mode
Figure 25.2: The Inventory Transactions form in Add mode.
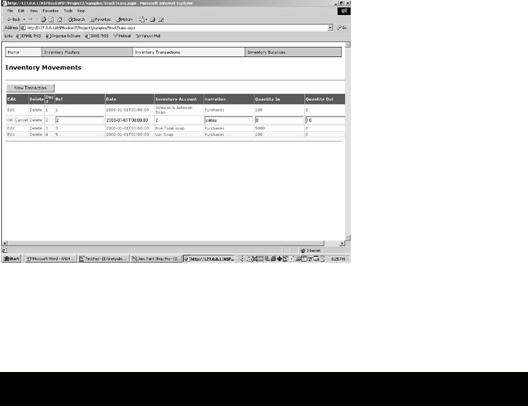
Figure 25.3: The Inventory Transactions form in Edit mode.
The ReBind Function
The ReBind function binds the DataGrid to a SQL query, first in the Page_load event, and then whenever the data changes and the Grid needs to be refreshed. Sub ReBind shows the script of the function.
Sub ReBind
Sub ReBind()
Dim t As New NameSpaceHersh.SQLService
Dim ds As DataSet
'DataSetCommand
SQL = "select * from tr_header h, stock_detail s, stock_master m"
SQL = SQL + " where h.doc_no = s.doc_no"
SQL = SQL + " AND s.code_value = m.code_value"
ds = t.Populate(ConnStr, SQL)
Grid1.DataSource=ds.Tables("vTable").DefaultView
Grid1.DataBind()
'populate inventory account(add mode) selection drop down list
SQL = "Select * from stock_master"
ds = t.Populate(ConnStr, SQL)
acode_value.DataSource=ds.Tables("vTable").DefaultView
acode_value.DataBind()
addshow.visible = true

End Sub
In this function, I call the web service method Populate twice, each time passing to it as parameters the connection string and the SQL query. A DataSet containing the result set is returned from the function, which I use to bind the DataGrid and the DropDownList, respectively.
The Add Mode
When the addshow button is clicked, the add_show Sub is fired. This Sub simply sets the visible property of the panel AddPanel to true. This, in turn, makes all the controls residing on this panel visible.
Sub add_show
Sub add_show(Source As Object, E As EventArgs)
AddPanel.visible = true
End Sub
The input controls for the Insert mode have been marked in the web form within the HTML comment blocks Insert Logic Starts and Insert Logic Ends. Each control has an associated id property, which will be used to refer to the control. There is a RequiredFieldValidator attached to the Date and ref fields. The stored procedure p_stock_trans will also check for the uniqueness of the ref field. If it is not unique, the procedure will return an error condition. The add_click button is fired when the user clicks on the SubmitDetailsBtn button. This method builds a SQL execute query and passes it on to the RunSql function, which in turn executes it. The script for the add_click method is as follows:
Sub add_click
Sub add_click(Source As Object, E As EventArgs)
Dim sql As string
sql = "Execute p_stock_trans @date = '"
sql = sql+ adate.text+"',@ref= '"+aref.text+"', @qty_in ="
sql = sql+ aqty_in.text+",@qty_out = "+aqty_out.text+" ,"
sql = sql+ "@id = 'RPT', @doc_no = NULL"+", @narr= '"
sql = sql+ anarr.text+"', @code_value = "+acode_value.SelectedItem.value
RunSql(sql)
rebind()

hidePanel()
End Sub
The hidePanel function simply hides the panel (by setting its visible property to False) and sets the value property of all the TextBox controls to spaces.
The Update Mode
The DataGrid operates in the Edit mode when the edit link is clicked. The user makes the appropriate changes and clicks on the Ok link. This fires off the Grid1_Update function. The value property for all the TextBox controls is extracted, and a SQL procedure call string is built. This string is passed onto the RunSQL function, which makes the actual procedure call.
Sub Grid1_Update
Sub Grid1_Update(sender As Object, e As DataGridCommandEventArgs)
Dim sql As string
Dim vdate As String
Dim ref As String
Dim code_value As String
Dim qty_in As String
Dim qty_out As String
Dim id As String
Dim narr As String
Dim myTextBox As TextBox
'This is the key value:
'Retrieved from the DataKey, since it's a read only field
Dim doc_no As string = Grid1.DataKeys.Item(E.Item.ItemIndex).ToString
myTextBox = E.Item.FindControl("edit_date")
vdate = mytextbox.text
myTextBox = E.Item.FindControl("edit_ref")
ref = trim(mytextbox.text)
myTextBox = E.Item.FindControl("edit_qty_in")
qty_in = trim(mytextbox.text)
myTextBox = E.Item.FindControl("edit_qty_out")
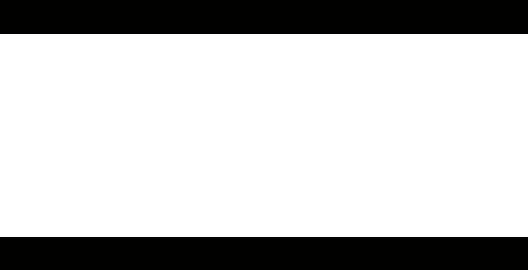
qty_out = trim(mytextbox.text)
myTextBox = E.Item.FindControl("edit_narr")
narr = trim(mytextbox.text)
myTextBox = E.Item.FindControl("edit_code_value")
code_value = trim(mytextbox.text)
'Now execute stored procedure
sql = "Execute p_stock_trans @date = '"
sql = sql + vdate + "' ,@ref= '" + ref + "', @qty_in ="
sql = sql + qty_in + ",@qty_out = "+ qty_out +" , "
sql = sql + "@id = 'STK', @doc_no = "+doc_no+", @narr= '"+narr+ "',"
sql = sql + "@code_value=" + code_value
'response.write(sql)
RunSql(sql)
rebind()
End Sub
Function RunSql
This is a generic function, which executes a SQL Action query against the database. The SQL query is passed to this function as a parameter. The Grid1_update Sub, the Add_click Sub, and the Grid1_delete Sub call this function to update, add, or delete a record. This function in turn calls the RunSql function of the web service and passes to it as parameters the connection string as well as the SQL action query/procedure call. If the procedure/query was executed successfully, the string "Success" is returned from the function. Otherwise, the appropriate error string is returned, which is displayed in the browser.
Sub RunSQL
Sub RunSql(vsql as string)
Dim t As New NameSpaceHersh.SQLService
Dim s As string
s = t.RunSQL(ConnStr,vSQL)
Grid1.EditItemIndex = -1
Rebind

if s <> "Success" then
Message.Text = s
Message.Style("color") = "red"
End if
End Sub
The Delete Mode
I have created a ButtonColumn having a CommandName of Delete as follows:
<asp:ButtonColumn Text = "Delete" CommandName = "Delete" HeaderText = "Delete"/>.
The OnDeleteCommand of the DataGrid fires the Grid1_delete function whenever the user clicks on the delete hyperlink. The Grid1_delete function sends a SQL delete query to the RunSql function, which deletes all tr_header and transactions records having a document number equal to the clicked doc_no.
Grid1_delete
Sub Grid1_delete(sender As Object, e As DataGridCommandEventArgs)
Dim doc_no As string = Grid1.DataKeys.Item(E.Item.ItemIndex).ToString
Dim sql As string
sql = " Delete from stock_detail where doc_no = " + cstr(doc_no)
sql = sql + " Delete from tr_header where doc_no = " + cstr(doc_no)
RunSql(sql)
rebind()
End Sub
Here is the complete code listing of StockTrans.aspx
StockTrans.aspx
<%@Page Language="VB" Inherits="BaseClass" Src="StockTrans.vb" %>
<%@ Import Namespace="System.Data" %>
<%@ Import Namespace="System.Data" %>
<%@ Import Namespace="System.Data.OleDb" %>
<%@ Register TagPrefix="Hersh" TagName="nav" Src="nav.ascx" %> <html>
<script language="VB" runat="server">
Sub Grid1_Update(sender As Object, e As DataGridCommandEventArgs) Dim sql As string
Dim vdate As String
Dim ref As String
Dim code_value As String
Dim qty_in As String
Dim qty_out As String
Dim id As String
Dim narr As String
Dim myTextBox As TextBox 'This is the key value:
'Retrieved from the DataKey, since it's a read only field
Dim doc_no As string = Grid1.DataKeys.Item(E.Item.ItemIndex).ToString myTextBox = E.Item.FindControl("edit_date")
vdate = mytextbox.text
myTextBox = E.Item.FindControl("edit_ref") ref = trim(mytextbox.text)
myTextBox = E.Item.FindControl("edit_qty_in") qty_in = trim(mytextbox.text)
myTextBox = E.Item.FindControl("edit_qty_out") qty_out = trim(mytextbox.text)
myTextBox = E.Item.FindControl("edit_narr") narr = trim(mytextbox.text)
myTextBox = E.Item.FindControl("edit_code_value") code_value = trim(mytextbox.text)
'Now execute stored procedure
sql = "Execute p_stock_trans @date = '"
sql = sql + vdate + "' ,@ref= '" + ref + "', @qty_in =" sql = sql + qty_in + ",@qty_out = "+ qty_out +" , "
sql = sql + "@id = 'STK', @doc_no = "+doc_no+", @narr= '"+narr+ "'," sql = sql + "@code_value=" + code_value
'response.write(sql)
RunSql(sql)
rebind() End Sub
Sub add_click(Source As Object, E As EventArgs)
Dim sql As string
sql = "Execute p_stock_trans @date = '"
sql = sql+ adate.text+"',@ref= '"+aref.text+"', @qty_in =" sql = sql+ aqty_in.text+",@qty_out = "+aqty_out.text+" ," sql = sql+ "@id = 'RPT', @doc_no = NULL"+", @narr= '"
sql = sql+ anarr.text+"', @code_value = "+acode_value.SelectedItem.value RunSql(sql)
rebind()
hidePanel() End Sub
Sub add_show(Source As Object, E As EventArgs)
AddPanel.visible = true
End Sub
Sub Grid1_delete(sender As Object, e As DataGridCommandEventArgs) Dim doc_no As string = Grid1.DataKeys.Item(E.Item.ItemIndex).ToString Dim sql As string
sql = " Delete from stock_detail where doc_no = " + cstr(doc_no) sql = sql + " Delete from tr_header where doc_no = " + cstr(doc_no) RunSql(sql)
rebind() End Sub
</script>
<head>
<style>
a { color:black; text-decoration:none;}
a:hover {color:red; text-decoration:underline;}
</style>
</head>
<body style="font: 10pt verdana; background-color:ivory"> <form runat="server">
<asp:ValidationSummary runat=server headertext="There were errors on the page:" /> <!------ Navigation Start------------>
<Hersh:nav id="menu" runat = server vGridlines = Both
vBorderColor = "Black" vCellPadding = 7 />
<!------ Navigation Ends------------>
<h3><font face="Verdana"> Inventory Movements <asp:Label id="title" runat="server"/> </font></h3> <table width="95%">
<tr><td>
<asp:Button id="Addshow" visible = "false" text="New Tranaction" onclick="add_show" runat="server" />
</td></tr>
<hr>
<tr>
<td valign="top">
<asp:DataGrid id="Grid1" runat="server"
AutoGenerateColumns="false"
BackColor="White"
BorderWidth="1px" BorderStyle="Solid" BorderColor="Tan"
CellPadding="2" CellSpacing="0"
Font-Name="Verdana" Font-Size="8pt"
OnEditCommand="Grid1_Edit"
OnCancelCommand="Grid1_Cancel"
OnUpdateCommand="Grid1_Update"
OnDeleteCommand = "Grid1_delete"
DataKeyField="doc_no">
<Columns>
<asp:EditCommandColumn
EditText="Edit"
CancelText="Cancel"
UpdateText="OK"
ItemStyle-Wrap="false"
HeaderText="Edit"
HeaderStyle-Wrap="false"/>
<asp:ButtonColumn Text="Delete" CommandName="Delete" HeaderText="Delete"/>
<asp:BoundColumn HeaderText="Doc #" ReadOnly="true" DataField="doc_no"/>
<asp:TemplateColumn HeaderText="Ref" > <ItemTemplate>
<asp:Label Text='<%# Container.DataItem("ref") %>' runat="server"/>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="edit_ref" Text='<%# Container.DataItem("ref") %>' runat="server"/>