
Microsoft ASP .NET Professional Projects - Premier Press
.pdf
sql = "UPDATE Masters Set Opening = 90 WHERE code_display = 'test'"
RunSQL(sql)
rebind
Message.Text = "...Updated all test records: Set closing balance = 90...! "
End Sub
Sub ReBind()
Dim t As NameSpaceHersh.SqlService = New
NameSpaceHersh.SqlService()
Dim vsql as string
Dim ds as DataSet
vSQL = "select * from Masters"
ds = t.Populate(vcn, vSql)
DataGrid1.DataSource=ds.Tables("vTable").DefaultView
DataGrid1.DataBind()
End Sub
Function RunSQL (vSQL as String)
Dim t As NameSpaceHersh.SqlService = New
NameSpaceHersh.SqlService()
t.RunSQL(vcn,vSQL)
End Function
</script>
<body>
<body style="font: 10pt verdana; background-color:beige">
<form runat=server>
<h4> ASP .NET Web Services </h4>
<asp:button text="Refresh" Onclick="Show_Click" runat=server/>
<asp:button text="Insert" Onclick="Insert_Click" runat=server/>
<asp:button text="Update" Onclick="Update_Click" runat=server/>
<asp:button text="Delete" Onclick="Delete_Click" runat=server/>
<asp:label id="Message" runat=server/>
<asp:DataGrid id="DataGrid1" runat="server" />
</form>
</body>
</html>
In the Page_load event, I specify the connection string as:
vcn= "Provider=SQLOLEDB; Data Source=(local); _
Initial Catalog=ASPNET;User ID=sa;"
The Sub ReBind calls the Populate function of the web service and passes it the query "select * from Masters" as well as the connection string. The Populate function returns a DataSet, the default view of which is bound to a DataGrid as follows:
Sub ReBind()
Dim t As NameSpaceHersh.SqlService = New
NameSpaceHersh.SqlService()
Dim vsql as string
Dim ds as DataSet
vSQL = "select * from Masters"
ds = t.Populate(vcn, vSql)
DataGrid1.DataSource=ds.Tables("vTable").DefaultView
DataGrid1.DataBind()
End Sub
Note that this is a pretty nifty way of calling queries that return data. You can pass any SQL query (the query may be a join on multiple tables) and get a DataSet back, which can then be manipulated in any way. I can also connect to any database as I am passing the database connection string to the function.
I have three buttons and associated click events which pass an insert, an update, and a delete statement respectively to the RunSQL function on the form, which in turn calls the RunSQL function of the web service. Here are the three functions:
Sub Insert_click(Sender As Object, E As EventArgs)
sql = "Insert into Masters(code_display,code_category,type)"
sql = sql + "Values ('test',701,'E')"
RunSQL(sql)
rebind
Message.Text = " ..Inserted test record... "
End Sub
Sub Delete_click(Sender As Object, E As EventArgs)
sql = "delete from masters where code_display = 'test'"
RunSQL(sql)
rebind
Message.Text = "...Deleted all test records..."
End Sub
Sub Update_Click(Sender As Object, E As EventArgs)
sql = "UPDATE Masters Set Opening = 90 WHERE code_display = 'test'"
RunSQL(sql)
rebind
Message.Text = "...Updated all test records: Set closing balance = 90...!
"
End Sub
The local RunSQL function calls the RunSQL function of the web service and passes it the SQL string and the connection string. The web service function then executes the action query.
Function RunSQL (vSQL as String)
Dim t As NameSpaceHersh.SqlService = New
NameSpaceHersh.SqlService()
t.RunSQL(vcn,vSQL)
End Function

Web services is an important element of ASP.NET. The techniques developed in this chapter show how the process of database interaction can be abstracted and encapsulated as a web service. In the subsequent chapters of this project (Chapters 19 through 22), I will explain how the techniques developed in this chapter can be applied to an accounting application.
Chapter 19: Designing a Navigation System
Overview
In Chapter 6, you designed an XML-based site navigation system. You developed a user control which when placed on a Web page generated the navigation links. Figure 19.1 shows what the navigation links look like.
Figure 19.1: Navigation links.
The navigation links were stored in an XML file. I will briefly review the user control here because I am going to use it on every form of the project.
Navigation Links
The navigation links of this project are stored in the file nav.xml.
Nav.xml
<Siteinfo>
<site>
<sitename>Home</sitename>
<siteurl>default.aspx</siteurl>
</site>
<site>
<sitename>Masters</sitename>
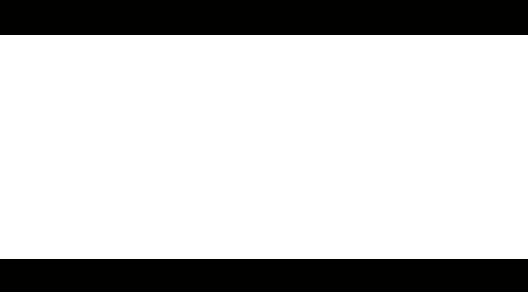
<siteurl>masters3.aspx</siteurl>
</site>
<site>
<sitename>Transactions</sitename>
<siteurl>selection.aspx</siteurl>
</site>
<site>
<sitename>Trial Balance</sitename>
<siteurl>Trialbalance.aspx</siteurl>
</site>
</Siteinfo>
Each link to be displayed is enclosed within the site node, which has two elements: the site name and the site URL. My user control will display each of these URLs at the top of each page.
The User Control
The user control is described in Chapter 6. To reiterate, you can assign it the GridLines, BorderColor, and CellPadding properties. It reads the XML file and binds a DataList to it. The DataList displays the links that you see on each page. Here is the code:
nav.ascx
<%@ Import Namespace="System.Data" %>
<%@ Import Namespace="System.IO" %>
<%@ Import Namespace="System.Drawing" %>
<script language="VB" runat="server">
'Public Variable for each exposed Property
PUBLIC vGridLines As GridLines
PUBLIC vBorderColor as String
PUBLIC vCellPadding As Integer
Sub Page_Load(Source As Object, E As EventArgs)
Dim ds As New DataSet
Dim fs As filestream
Dim xmLStream As StreamReader
fs = New filestream(Server.MapPath("nav.xml"), FileMode.Open, FileAccess.Read) xmlStream = new StreamReader(fs)
ds.ReadXML(XmlStream)
fs.Close() dlist.DataSource=ds.Tables("site").DefaultView dlist.DataBind()
dlist.GridLines = vGridLines dlist.BorderColor=System.Drawing.Color.FromName(vBorderColor) dlist.CellPadding=vCellPadding
End Sub </script>
<asp:DataList runat=server id="dlist" RepeatDirection="horizontal" RepeatMode="Table" Width="100%"
BorderWidth="1"
Font-Name="Verdana"
Font-Size="8pt"
HeaderStyle-BackColor="#aaaadd"
SelectedItemStyle-BackColor="yellow"
ItemStyle-BackColor="antiquewhite"
AlternatingItemStyle-BackColor="tan"
>
<ItemTemplate>

<asp:HyperLink runat="server"
Text='<%# Container.DataItem("sitename") %>'
NavigateUrl= '<%# Container.DataItem("siteurl") %>' />
</ItemTemplate>
</asp:DataList>
Using the Control
Each Web page must register the control by using the following declaration at the top of each page:
<%@ Register TagPrefix="Hersh" TagName="nav" Src="nav.ascx" %>
Within the page, the control is invoked as follows:
<!------ |
Navigation Start------------ |
> |
<Hersh:nav id="menu" runat = server
vGridlines = Both
vBorderColor = "Black"
vCellPadding = 7 />
<!------ |
Navigation Ends |
-------------> |
Chapter 20: Incorporating Web Services in the Chart of Accounts Form
Overview
The Personal Finance Manager built in Project 1 of this book used the Masters.aspx web form (together with its Code Behind form, Masters.vb) to interact with the masters database table. This web form was developed in chapter 15 (Chart of Accounts). In this chapter, I will modify this web form so that it can use the SQLService web service. I will need to change two methods residing in the Code Behind form Masters.vb in order to use the web service. These methods are the ReBind and the RunSql methods, both of which interact with the database.
The ReBind() method was used to bind a DataGrid and a DropDownList control to a database table. The following is the code snippet of the ReBind function, which I am going to change:
The Original ReBind Method
Sub ReBind()
SQL = "select m.*, g.code_display as category "
SQL = SQL + "from masters m, groups g "
SQL = SQL + " where m.code_category = g.code_value"
myCommand = New OleDbDataAdapter(SQL, myConnection)
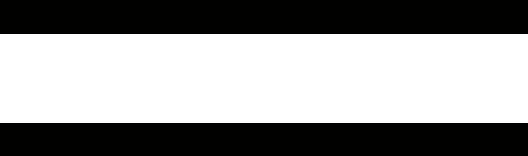
myCommand.Fill(ds, "masters") 'Binding a Grid
Grid1.DataSource=ds.Tables("masters").DefaultView
Grid1.DataBind()
SQL = "Select * from groups order by code_display" myCommand = New OleDbDataAdapter(SQL, myConnection) myCommand.Fill(ds, "groups")
'populate drop down list acode_category.DataSource=ds.Tables("groups").DefaultView acode_category.DataBind()
hidePanel() End Sub
To use the web service, I have changed this to what is shown in the following code snippet:
The Mmodified ReBind Method that Uses the Web Services
Sub ReBind()
Dim t As New NameSpaceHersh.SQLService
Dim ds As DataSet
' Bind Grid
SQL = "select m.*, g.code_display as category "
SQL = SQL + "from masters m, groups g "
SQL = SQL + " where m.code_category = g.code_value" ds = t.Populate(ConnStr, SQL) Grid1.DataSource=ds.Tables("vTable").DefaultView Grid1.DataBind()
'populate drop down list
SQL = "Select * from groups order by code_display" ds = t.Populate(ConnStr, SQL)
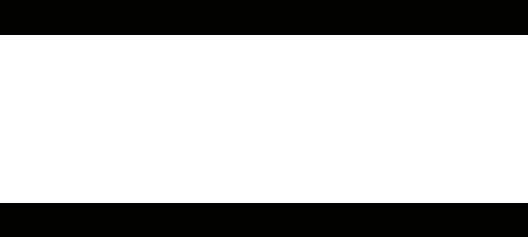
acode_category.DataSource=ds.Tables("vTable").DefaultView
acode_category.DataBind()
hidePanel()
End Sub
In the modified ReBind method, I call the web service method called Populate twice, each time passing it a connection string and a SQL query. A DataSet containing the result set is returned from the function, which I use to bind the DataGrid and DropDownList control respectively.
The second function that I need to modify is the RunSql function. This function is used to add, delete, or update a row. The appropriate event handlers pass to this function a SQL action query or a stored procedure name with the appropriate parameters. The original code snippet is listed below:
The Original RunSql Method
Sub RunSql(sql as string)
try
Dim mycommand2 As New OleDbCommand(sql,myConnection)
myConnection.Open()
myCommand2.ExecuteNonQuery()
myConnection.Close()
'turn off editing
Grid1.EditItemIndex = -1
Catch ex As OleDbException
' SQL error
Dim errItem As OleDbError
Dim errString As String
For Each errItem In ex.Errors
errString += ex.Message + "<br/>"
Next
Message.Text = "SQL Error.Details follow:<br/><br/>" & errString
Message.Style("color") = "red"
Catch myException as Exception
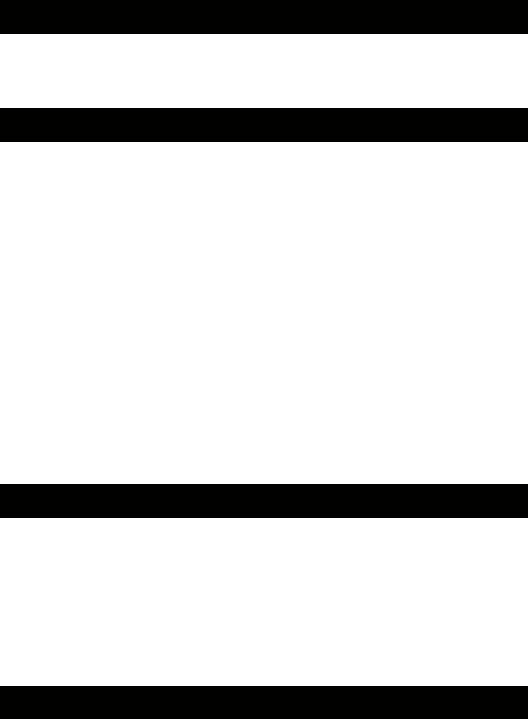
Response.Write("Exception: " + myException.ToString())
Message.Style("color") = "red"
End try
rebind
response.write(sql)
End Sub
The following code extract shows the modified version of this function:
Modified Version of the RunSql Method
Sub RunSql(vSQL as string)
Dim t As New NameSpaceHersh.SQLService
Dim s As string
s = t.RunSQL(ConnStr,vSQL)
Grid1.EditItemIndex = -1
Rebind
if s <> "Success" then
Message.Text = s
Message.Style("color") = "red"
End if
End Sub
This function calls the RunSql function of the web service and passes it the connection string as well as the SQL action query/procedure call as parameters. If the procedure/query was executed successfully, the string Success is returned from the function. Otherwise, the appropriate error string is returned, which is displayed in the browser.
I include the complete listing of the Code Behind file, Masters.vb, after modifying the two methods as discussed above. There is no change made to the web form Masters.aspx.
Masters3.vb
Option Strict Off
Imports System
Imports System.Collections
Imports System.Text
Imports System.Data
Imports System.Data.OleDb
Imports System.Web.UI
Imports System.Web.UI.WebControls
Public Class BaseClass
Inherits System.Web.UI.Page
Protected Grid1 as DataGrid
Protected Message as label
Protected acode_category as dropdownlist
Protected AddPanel as Panel
Dim ConnStr As String
Dim SQL As String
Sub Page_Load(Source As Object, E As EventArgs) ConnStr = "Provider=SQLOLEDB; Data Source=(local); " ConnStr = ConnStr+" Initial Catalog=ASPNET;User ID=sa;" if NOT (isPostBack)
rebind end if
End Sub
Sub ReBind()
Dim t As New NameSpaceHersh.SQLService
Dim ds As DataSet
' Bind Grid
SQL = "select m.*, g.code_display as category " SQL = SQL + "from masters m, groups g "
SQL = SQL + " where m.code_category = g.code_value"