
Microsoft ASP .NET Professional Projects - Premier Press
.pdfend try End Sub
Sub hidePanel()
if AddPanel.visible = true then AddPanel.visible = false 'reset values
adate.text = "" aref.text = "" adr_amount.text = "" acr_amount.text = "" anarr.text = ""
end if End Sub
Sub UpdateSelection(vselection)
sql = "delete from tblSelection "
sql = sql + " insert into tblSelection(selection)" sql = sql + " values('" + vselection + "')" runSql(sql)
End Sub
End Class
Chapter 17: The Trial Balance Report
Overview
The Trial Balance is the main accounting report and is the basis for all other financial reports like the balance sheet, the profit and loss, and the cash flow statement. This report presents the closing balance of all master accounts in a tabular layout with the debit accounts on one side and the credit accounts on the other. The Acid test to show that all our transaction entries are correct is ensuring that the Trial Balance's debits and credits match.
Figure 17.1 shows what the Trial Balance web form looks like.

Figure 17.1: The Trial Balance.
The Trial Balance web form is comprised of two DataGrids. The first gets the detail rows of the Trial Balance and is bound to the following query:
SELECT code_display, closing,
dr_amount = CASE type
WHEN 'A' THEN closing
WHEN 'E' THEN closing
ELSE 0
END,
cr_amount = CASE type
WHEN 'I' THEN closing
WHEN 'L' THEN closing
ELSE 0
END
FROM Masters
I need to show debits and credits in two separate columns; hence, using a case statement I separate the debits and credits. Remember that assets and expenses are of type debit and liabilities and income are of type credit. The second grid displays the grand total of all debits and credits. This is bound to the following SQL query:
SELECT 'Total' as nothing,
(Select sum(closing) From masters where type in('A','E')) as dr_total ,
(Select sum(closing) From masters where type in('I','L')) as cr_total
Here is the complete code listing for the TrialBalance.aspx web form.
TrialBalance.aspx
<%@ Import Namespace="System.Data" %>
<%@ Import Namespace="System.Data.OleDb" %>
<html>
<script language="VB" runat="server">
Dim myConnection As OleDbConnection
Dim myCommand As OleDbDataAdapter
Dim ds As New DataSet
Dim ConnStr As String
Dim SQL As String
Sub Page_Load(Source As Object, E As EventArgs)
ConnStr = "Provider=SQLOLEDB; Data Source=(local); "
ConnStr = ConnStr+" Initial Catalog=ASPNET;User ID=sa;"
myConnection = New OleDbConnection(ConnStr) |
|
|||
if NOT (isPostBack) |
|
|
|
|
rebind |
|
|
|
|
end if |
|
|
|
|
End Sub |
|
|
|
|
Sub ReBind() |
|
|
|
|
'DataSetCommand |
|
|
|
|
sql = "SELECT code_display, closing, " |
|
|
||
sql = sql + " dr_amount = CASE type |
WHEN 'A' THEN " |
|||
sql = sql + " closing WHEN 'E' THEN closing |
ELSE |
0 END, " |
||
sql = sql + " |
cr_amount = CASE type |
WHEN 'I' |
" |
|
sql = sql + " |
THEN closing WHEN 'L' |
THEN closing |
ELSE 0 END " |
sql = sql + " From Masters"
myCommand = New OleDbDataAdapter(SQL, myConnection) 'use Fill method of DataSetCommand to populate dataset myCommand.Fill(ds, "Masters")
'Binding a Grid
Grid1.DataSource=ds.Tables("Masters").DefaultView
Grid1.DataBind()
'totals
sql = "SELECT 'Total' as nothing ,"
sql = sql + " (Select sum(closing) From masters "
sql = sql + " where type in('A','E')) as dr_total , " sql = sql + " (Select sum(closing) From masters " sql = sql + " where type in('I','L')) as cr_total "
myCommand = New OleDbDataAdapter(SQL, myConnection) myCommand.Fill(ds, "totals")
'Binding a Grid
Grid2.DataSource=ds.Tables("totals").DefaultView
Grid2.DataBind()
End Sub </script> <body>
<h3><font face="Verdana">Trial Balance </font></h3> <form runat=server>
<asp:HyperLink runat="server" Text="Masters" NavigateUrl="masters3.aspx"></asp:HyperLink>  <asp:HyperLink runat="server" Text="Transactions" NavigateUrl="selection.aspx"></asp:HyperLink>  <asp:HyperLink runat="server" Text="Home"
NavigateUrl="default.aspx"></asp:HyperLink> <br> <br>
<asp:DataGrid id="Grid1" runat="server" AutoGenerateColumns="false" BackColor="White"
BorderWidth="1px" BorderStyle="Solid" BorderColor="Tan"
CellPadding="2" CellSpacing="0"
Font-Name="Verdana" Font-Size="8pt"> <Columns>
<asp:BoundColumn HeaderText="Account" DataField="code_display"> <HeaderStyle Width="150px">
</HeaderStyle>
</asp:BoundColumn>
<asp:BoundColumn HeaderText="Debit Amount" DataField="dr_amount" > <HeaderStyle Width="150px">
</HeaderStyle>
</asp:BoundColumn>
<asp:BoundColumn HeaderText="Credit Amount" DataField="cr_amount" > <HeaderStyle Width="150px">
</HeaderStyle>
</asp:BoundColumn>
</Columns>
<HeaderStyle BackColor="teal" ForeColor="white" Font-Bold="true"> </HeaderStyle>
<ItemStyle ForeColor="DarkSlateBlue"> </ItemStyle>
<AlternatingItemStyle BackColor="Beige"> </AlternatingItemStyle>
</asp:DataGrid>
<!----Totals //------>
<asp:DataGrid id="Grid2" runat="server" AutoGenerateColumns="false" BackColor="White"
BorderWidth="1px" BorderStyle="Solid" BorderColor="Tan"
CellPadding="2" CellSpacing="0"
Font-Name="Verdana" Font-Size="8pt"> <Columns>
<asp:BoundColumn HeaderText="" DataField="nothing"> <HeaderStyle Width="150px">
</HeaderStyle>
</asp:BoundColumn>
</Columns>
<Columns>
<asp:BoundColumn HeaderText="" DataField="dr_total">
<HeaderStyle Width="150px">
</HeaderStyle>
</asp:BoundColumn>
<asp:BoundColumn HeaderText="" DataField="cr_total" >
<HeaderStyle Width="150px">
</HeaderStyle>
</asp:BoundColumn>
</Columns>
<ItemStyle BackColor="teal" foreColor="white" Font-Bold="true">
</ItemStyle>
</asp:DataGrid>
</form>
</body>
</html>
Project 1 Summary
In this Project, I took a traditional client/server application and revamped it for the Web. Applications that work off the Web offer significant advantages over their client/server brethren. This accounting application is totally wireless. You don't need to lay out network cables to connect it to the database. You will notice that I have relegated most of the script processing to stored procedures and triggers. This has enabled me to have a very thin web form.
In Project 2 of this book, I will incorporate web services in this application. I can then harness the full power of the Web as well as that of a client/server product.
Project 2: Web Services
Chapter List
§Chapter 18: Creating a Generic Database Web Service
§Chapter 19: Designing a Navigation System
§Chapter 20: Incorporating Web Services in the Chart of Accounts Form
§Chapter 21: Incorporating Web Services in the Transactions Form
§Chapter 22: Incorporating Web Services in the Trial Balance
Project 2 Overview
The Personal Finance Manager described in the preceding project showed how we can Web-enable an accounting application and make it accessible through the Internet. The consequences of this approach are far-reaching. Consider the case of a car manufacturer who has a large number of ancillary companies manufacturing components, such as air filters, wind screens, tires, and so on. These ancillary companies are spread all over the world. This car manufacturer uses "Just in Time" manufacturing techniques and the ancillary companies only produce (and ship) as much as can be consumed by the manufacturer in a given period. This removes the necessity of holding inventory both by the supplier and the car manufacturer. Say an overseas company manufactures air filters for the car manufacturer. It will need day-to-day information on the air filter requirements of the car manufacturer and will manufacture them accordingly. To do this, the overseas company needs access to the daily production requirements of the car manufacturer, who, in turn, requires access to the production and inventory records of the supplier. Do we need to set up a satellite link between the companies to enable their databases to communicate?
A retail chain has store locations all over the U.S. Each time a customer makes a purchase at the store, the item sold must be removed from inventory and the dollar amount of the purchase incorporated in the financial books as a sale. Suppose all the constituent stores of the chain read and write to one central database. Do we set up network infrastructure between all the stores for the communication to take place? And, at what cost?
In both the preceding scenarios, we need to set up lines of communication between databases located in distant locations. Traditionally, we would have to set up network communication infrastructure to make this happen. However, using web services, we can link them using the Internet.
The basic requirement of an application that interacts with a database is to select, insert, update, and delete records from the database. Using web services, we can encapsulate the database interaction logic as a series of methods that are called over the Internet. When we have the ability to interact with the database over the Web, we don't require expensive satellite or "wire" links between applications and databases.
The Personal Finance Manager developed in the previous project was tightly coupled to the ASP.NET technology and worked in the browser. Tools such as Visual Basic.NET and C# enable us to build applications that are user-friendly and have a rich user interface. For example, users have grown accustomed to seeing toolbars, MDI (multiple document interface), and other graphical niceties, which a browser is not able to provide. Won't it be nice to build applications using one of these tools and still be able to communicate over the Internet? We would then be able to build applications with a rich graphical user interface and not have to worry about setting up elaborate network systems for communication. Web services enables us to do just that. As I will show you in this project, we can build generic database access services which can then be us ed with an application built in Visual Basic.NET, C#, or ASP.NET. I will show you how to use this web service with the Personal Finance Manager using ASP.NET. You can develop a feature-rich application (with all the associated graphical niceties) in Visual Basic.NET or C# and still use this web service.
Chapter 18: Creating a Generic Database Web
Service
Overview
In Chapter 8, I showed you how to create a generic database business object for communicating with a database. This object had useful functions for executing action
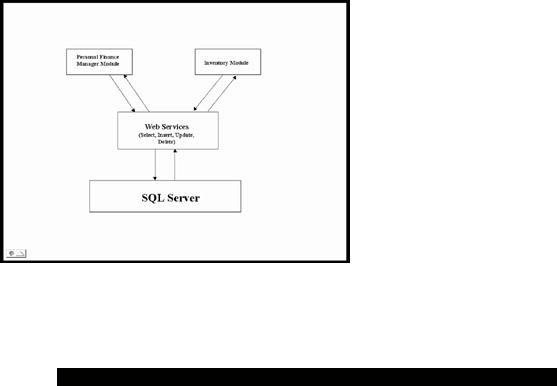
queries, such as insert, update, and delete as well as a generic routine for returning a DataSet, based on any user-supplied SQL query. This DataSet then could be used to bind an ASP.NET server control. I will now show you how to convert this business object into a web service. Various accounting modules can then use the functionality provided by the service. In Figure 18.1, I show how the two projects developed in this book (the Personal Finance Manager module and the Inventory module, which will be developed in Part IV) interact with the web service. The supporting example files for this example are located in the folder samples\SqlService of this chapter on the book's Web site at www.premierpressbooks.com/downloads.asp.
Figure 18.1: Interacting with the web service.
I will now walk you through the process of creating the web service. This comprises a number of steps, as follows:
1.Create the web service asmx file: Use NotePad to create a file called
SQLService.asmx, which has the following script:
SQLService.asmx
<%@ WebService Language="VB" Class="SQLService"%> Imports System
Imports System.Web.Services Imports System.Data
Imports System.Data.OleDb Imports System.Text
Public Class SQLService: Inherits WebService
<WebMethod()> Public Function TestFunction (vInput as Boolean) As String
If (vInput = TRUE) Then TestFunction = "It is the truth..."
Else
TestFunction = "False!False!False" End if
End Function
<WebMethod()> Public Function add( a as integer, b as integer) as string add = cstr(a+b)
End function
<WebMethod()> Public Function Populate(ConnStr as string, SQL as string) As DataSet
Dim dv As DataView Dim i As integer
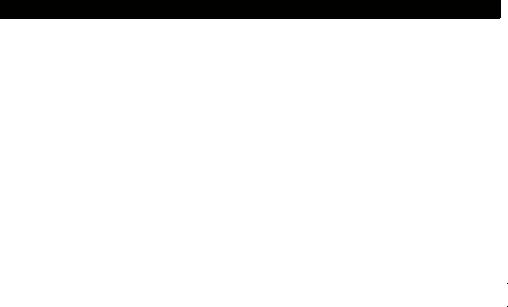
Dim myConnection As OleDbConnection Dim myCommand As OleDbDataAdapter Dim ds As New DataSet
myConnection = New OleDbConnection(ConnStr) myCommand = New OleDbDataAdapter(SQL, myConnection) myCommand.Fill(ds, "vTable")
'Populate = ds.Tables("vTable").DefaultView Populate = ds
End Function
<WebMethod()>PUBLIC Function RunSql ( ConnStr as string, vsql as string) as String
Dim Message As string try
message = "Success"
Dim myConnection As OleDbConnection myConnection = New OleDbConnection(ConnStr)
Dim mycommand As New OleDbCommand(vsql,myConnection) myConnection.Open()
myCommand.ExecuteNonQuery()
myConnection.Close()
Catch ex As OleDbException Dim errItem As OleDbError Dim errString As String
For Each errItem In ex.Errors errString += ex.Message + " "
Next
Message = "SQL Error.Details follow:<br/><br/>" & errString Catch myException as Exception
message = "Exception: " + myException.ToString() End try
RunSql = message End Function
End Class
This is exactly the same code that I developed in Chapter 8, hence I am not discussing it in detail here. The differences are that this file has an .asmx extension, the class inherits from WebService, a WebService attribute is added to the form header, the System.Web.Services namespace is imported and each function is prefixed with a <WebMethod()> tag. The WebMethod tag indicates to the ASP.NET run time that the method in question can be called over the Web.
2.Create the WSDL file: Open SQLService.asmx so that it goes through IIS (such as http://localhost/your virtual directory/SQLService.asmx). Click on Show WSDL. Save the resultant file as SQLService.wsdl. Note that you can do the same thing by browsing to http://localhost/your virtual directory/SQLService.asmx?wsdl.
3.Create and compile the proxy: Run msqlProxy.bat, which will create the
proxy SQLService.vb in the local folder and compile the DLL SQLService.dll to the bin folder.
msqlProxy.bat
|
|
|
REM |
------------ ------------Make Proxy |
wsdl.exe /l:VB /n:NameSpaceHersh /out:SqlService.vb SqlService.wsdl
REM |
------------Compile Proxy------------ |
Rem Remember to change outdir variable to point to your bin folder
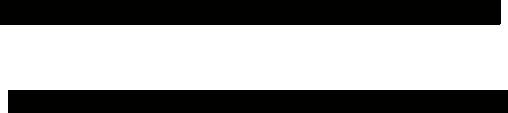
set outdir=g:\AspNetSamples\bin\SQLService.dll
set assemblies=System.dll,System.Web.dll,System.Data.dll,System.Web.Service s.dll,System.Xml.dll
vbc /t:library /out:%outdir% /r:%assemblies% SQLService.vb
pause
4.Test the service: The form that I have written to test the service is SQLService.aspx, which has the following code:
SQLService.aspx
<%@ Import Namespace="System.Data" %>
<%@ Import Namespace="System.Data.OleDb" %> <%@ Import Namespace="NameSpaceHersh" %>
<html>
<script language="VB" runat="server"> Dim SQL as string, vcn as string
Sub Page_Load(Source As Object, E As EventArgs)
vcn= "Provider=SQLOLEDB; Data Source=(local); Initial Catalog=ASPNET;User ID=sa;"
if NOT (isPostBack) rebind
end if End Sub
Sub Show_Click(Sender As Object, E As EventArgs)
Message.Text = "Masters Table Displayed... "
ReBind
End Sub
Sub Insert_click(Sender As Object, E As EventArgs)
sql = "Insert into Masters(code_display,code_category,type)" sql = sql + "Values ('test',701,'E')"
RunSQL(sql) rebind
Message.Text = " ..Inserted test record... "
End Sub
Sub Delete_click(Sender As Object, E As EventArgs) sql = "delete from masters where code_display = 'test'" RunSQL(sql)
rebind
Message.Text = "...Deleted all test records..."
End Sub
Sub Update_Click(Sender As Object, E As EventArgs)