
AhmadLang / Java, How To Program, 2004
.pdf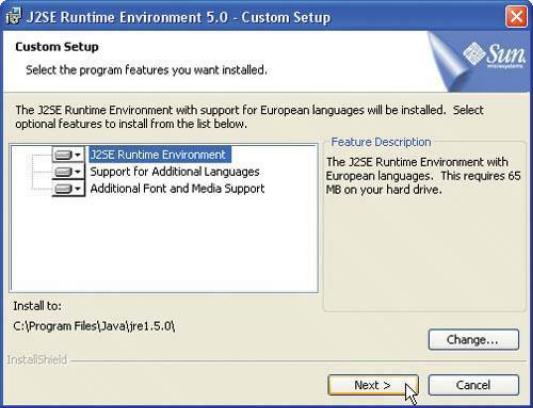
6.Selecting browsers. As part of the installation, you have the option of making the Java plug-in the default Java runtime environment for Java programs called applets that run in Web browsers (Fig. 11). After you select the desired browsers click the Next > button.
Figure 11. Selecting browsers.
(This item is displayed on page lxiv in the print version)

[Page lxiv]
7.Finishing the installation. The program will now install the JRE. Click the Finish button to complete the installation process (Fig. 12).
Figure 12. Completing the installation.

The PATH environment variable on your computer designates which directories the computer searches when looking for applications, such as the applications that enable you to compile and run your Java applications (called javac.exe and java.exe, respectively). You will now learn how to set the PATH environment variable on your computer.

[Page lxiv (continued)]
Setting the PATH Variable
The last step before you can use the JDK is to set the PATH environment variable to indicate where the JDK's tools are installed.
1.Opening the System Properties dialog. Right click on the My Computer icon on your desktop and select Properties from the menu. The System Properties dialog (Fig. 13) appears. [Note: Your System Properties dialog may appear different than the one shown in Fig. 13, depending on your version of Microsoft Windows. This particular dialog is from a computer running Microsoft Windows XP. Your dialog might include different information.]
[Page lxv]
Figure 13. System Properties dialog.
2.Opening the Environment Variables dialog. Select the Advanced tab at the top of the System Properties dialog (Fig. 14). Click the Environment Variables button to display the Environment Variables dialog (Fig. 15).
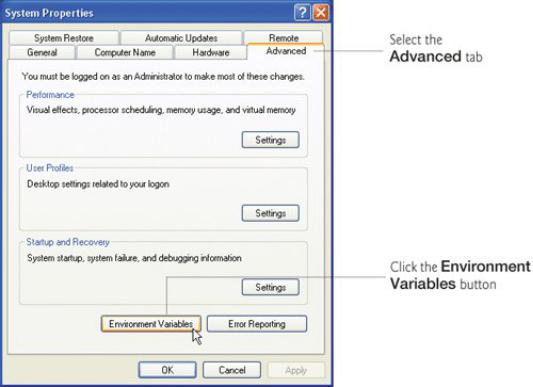
Figure 14. Advanced tab of System Properties dialog.
[View full size image]
Figure 15. Environment Variables dialog.
(This item is displayed on page lxvi in the print version)

[Page lxvi]
3.Editing the PATH variable. Scroll down inside the System variables box to select the PATH variable. Click the Edit button. This will cause the Edit System Variable dialog to appear (Fig. 16).
Figure 16. Edit System Variable dialog.
4.Changing the contents of the PATH variable. Place the cursor inside the Variable Value field. Use the left-arrow key to move the cursor to the beginning of the list. At the beginning of the list, type the name of the directory in which you placed the JDK followed by \bin; (Fig. 17). If you chose the default installation directory in Step 4 of Installing the J2SE Development Kit (JDK), you will add C:\Program Files\Java\jdk1.5.0\bin; to the PATH variable. Click the OK button to complete the modification of the PATH variable. [Note: The default installation directory is named jdk1.5.0, even though the JDK is now version 5.0.]
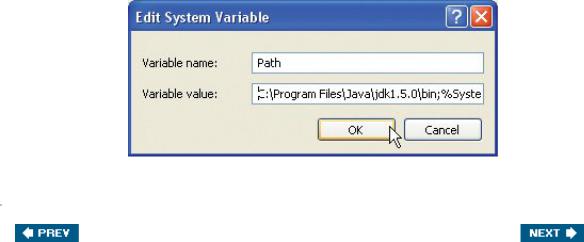
Figure 17. Editing the PATH variable.
You are now ready to being your Java studies with Java How to Program. We hope you enjoy the book! You can reach us easily at deitel@deitel.com. We will respond promptly.

[Page 1]
Chapter 1. Introduction to Computers, the Internet and the World Wide Web
Our life is frittered away by detail....Simplify, simplify.
Henry David Thoreau
The chief merit of language is clearness.
Galen
My object all sublime I shall achieve in time.
W. S. Gilbert
He had a wonderful talent for packing thought close, and rendering it portable.
Thomas B. Macaulay
"Egad, I think the interpreter is the hardest to be understood of the two!"
Richard Brinsley Sheridan
Man is still the most extraordinary computer of all.
John F. Kennedy
OBJECTIVES
In this chapter you will learn:
Basic hardware and software concepts.
Basic object technology concepts, such as classes, objects, attributes, behaviors, encapsulation, inheritance and polymorphism.
The different types of programming languages.
Which programming languages are most widely used.
A typical Java development environment.
Java's role in developing distributed client/server applications for the Internet and the Web.
The history of the industry-standard object-oriented design language, the UML.
The history of the Internet and the World Wide Web.
To test-drive Java applications.
[Page 2]
Outline

1.1 Introduction
1.2 What Is a Computer?
1.3 Computer Organization
1.4 Early Operating Systems
1.5 Personal, Distributed and Client/Server Computing
1.6 The Internet and the World Wide Web
1.7 Machine Languages, Assembly Languages and High-Level Languages
1.8 History of C and C++
1.9 History of Java
1.10 Java Class Libraries
1.11 FORTRAN, COBOL, Pascal and Ada
1.12 BASIC, Visual Basic, Visual C++, C# and .NET
1.13 Typical Java Development Environment
1.14 Notes about Java and Java How to Program, Sixth Edition
1.15 Test-Driving a Java Application
1.16 Software Engineering Case Study: Introduction to Object Technology and the UML (Required)
1.17 Wrap-Up
1.18 Web Resources
Summary
Terminology
Self-Review Exercises
Answers to Self-Review Exercises
Exercises

[Page 2 (continued)]
1.1. Introduction
Welcome to Java! We have worked hard to create what we hope you will find to be an informative, entertaining and challenging learning experience. Java is a powerful computer programming language that is fun for novices and appropriate for experienced programmers to use in building substantial information systems. Java How to Program, Sixth Edition, is an effective learning tool for each of these audiences.
The core of the book emphasizes achieving program clarity through the proven techniques of objectoriented programming. Nonprogrammers will learn programming the right way from the beginning. The presentation is clear, straightforward and abundantly illustrated. It includes hundreds of working Java programs and shows the outputs produced when those programs are run on a computer. We teach Java features in the context of complete working Java programswe call this the live-code approach. The example programs are included on the CD that accompanies this book, or you may download them from www.deitel.com or www.prenhall.com/deitel.
The early chapters introduce the fundamentals of computers, computer programming and the Java computer programming language, providing a solid foundation for the deeper treatment of Java in the later chapters. Experienced programmers tend to read the early chapters quickly and find the treatment of Java in the later chapters rigorous and challenging.
Most people are familiar with the exciting tasks computers perform. Using this text-book, you will learn how to command computers to perform those tasks. It is software (i.e., the instructions you write to command computers to perform actions and make decisions) that controls computers (often referred to as hardware). Java, developed by Sun Microsystems, is one of today's most popular software development languages.
[Page 3]
This book is based on Sun's Java 2 Platform, Standard Edition (J2SE). Sun provides an implementation of this platform, called the J2SE Development Kit (JDK), that includes the minimum set of tools you need to write software in Java. We used JDK version 5.0 to implement and test the programs in this book. When Sun makes the JDK available to publishers, it will be wrapped with the textbook on the accompanying CD. Sun updates the JDK on a regular basis to fix bugs. To download the most recent version of the JDK, visit java.sun.com/j2se.
Computer use is increasing in almost every field of endeavor. Computing costs have been decreasing dramatically due to rapid developments in both hardware and software technologies. Computers that might have filled large rooms and cost millions of dollars two decades ago can now be inscribed on silicon chips smaller than a fingernail, costing perhaps a few dollars each. Fortunately, silicon is one of the most abundant materials on earthit is an ingredient in common sand. Silicon chip technology has made computing so economical that hundreds of millions of general-purpose computers are in use worldwide, helping people in business, industry and government, and in their personal lives. The number could easily double in the next few years.
Over the years, many programmers learned the programming methodology called structured programming. You will learn structured programming and an exciting newer methodology, objectoriented programming. Why do we teach both? Object orientation is the key programming methodology used by programmers today. You will create and work with many software objects in this text. But you will discover that their internal structure is often built using structured-programming techniques. Also, the logic of manipulating objects is occasionally expressed with structured programming.
Java has become the language of choice for implementing Internet-based applications and software for devices that communicate over a network. Before long the stereo and other devices in your home will be networked together by Java technology. Don't be surprised when your wireless devices, like cell phones, pagers and personal digital assistants (PDAs), begin to communicate over the so-called wireless Internet via the kind of Java-based networking applications that you will learn in this book and its companion, Advanced Java 2 Platform How to Program. According to Sun's Web site (www.sun.com), in 2003, over 267 million cell phones equipped with Java technology were shipped! Java has evolved rapidly into the large-scale applications arena. It is no longer used simply to make World Wide Web pages come aliveit has become the preferred language for meeting the enterprise-wide programming