
AhmadLang / Java, How To Program, 2004
.pdf
[Page 9 (continued)]
1.10. Java Class Libraries
Java programs consist of pieces called classes. Classes include pieces called methods that perform tasks and return information when they complete them. Programmers can create each piece they need to form Java programs. However, most Java programmers take advantage of the rich collections of existing classes in the Java class libraries, which are also known as the Java APIs (Application Programming Interfaces). Thus, there are really two aspects to learning the Java "world." The first is the Java language itself, so that you can program your own classes, and the second is the classes in the extensive Java class libraries. Throughout this book, we discuss many library classes. Class libraries are provided primarily by compiler vendors, but many are supplied by independent software vendors (ISVs).
[Page 10]
Software Engineering Observation 1.1
Use a building-block approach to create programs. Avoid reinventing the wheeluse existing pieces wherever possible. Called software reuse, this practice is central to object-oriented programming.
We include many tips such as these Software Engineering Observations throughout the book to explain concepts that affect and improve the overall architecture and quality of software systems. We also highlight other kinds of tips, including Good Programming Practices (to help you write programs that are clearer, more understandable, more maintainable and easier to test and debugor remove programming errors), Common Programming Errors (problems to watch out for and avoid), Performance Tips (techniques for writing programs that run faster and use less memory), Portability Tips (techniques to help you write programs that can run, with little or no modification, on a variety of computersthese tips also include general observations about how Java achieves its high degree of portability), Error-Prevention Tips (techniques for removing bugs from your programs and, more important, techniques for writing bug-free programs in the first place) and Look and Feel Observations (techniques to help you design the "look" and "feel" of your applications' user interfaces for appearance and ease of use). Many of these are only guidelines. You will, no doubt, develop your own preferred programming style.
Software Engineering Observation 1.2
When programming in Java, you will typically use the following building blocks: Classes and methods from class libraries, classes and methods you create yourself and classes and methods that others create and make available to you.
The advantage of creating your own classes and methods is that you know exactly how they work and you can examine the Java code. The disadvantage is the time-consuming and potentially complex effort that is required.
Performance Tip 1.1
Using Java API classes and methods instead of writing your own
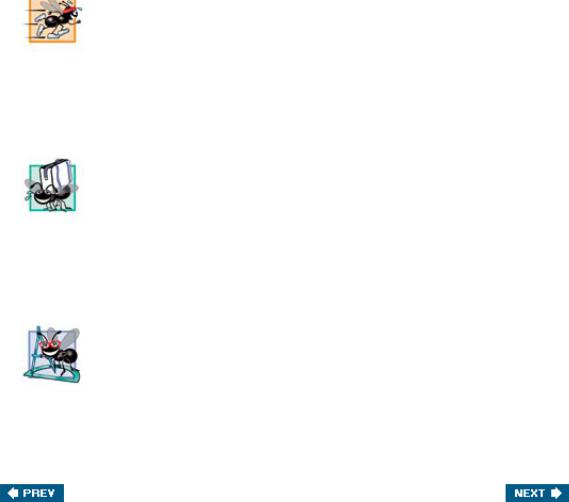
versions can improve program performance, because they are carefully written to perform efficiently. This technique also shortens program development time.
Portability Tip 1.1
Using classes and methods from the Java API instead of writing your own improves program portability, because they are included in every Java implementation.
Software Engineering Observation 1.3
Extensive class libraries of reusable software components are available over the Internet and the Web, many at no charge.
To download the Java API documentation, go to the Sun Java site java.sun.com/j2se/5.0/download.jsp.

[Page 11]
1.11. FORTRAN, COBOL, Pascal and Ada
Hundreds of high-level languages have been developed, but only a few have achieved broad acceptance. FORTRAN (FORmula TRANslator) was developed by IBM Corporation in the mid-1950s to be used for scientific and engineering applications that require complex mathematical computations. FORTRAN is still widely used, especially in engineering applications.
COBOL (COmmon Business Oriented Language) was developed in the late 1950s by computer manufacturers, the U.S. government and industrial computer users. COBOL is used for commercial applications that require precise and efficient manipulation of large amounts of data. Much business software is still programmed in COBOL.
During the 1960s, many large software-development efforts encountered severe difficulties. Software deliveries were typically late, costs greatly exceeded budgets and the finished products were unreliable. People began to realize that software development was a far more complex activity than they had imagined. Research in the 1960s resulted in the evolution of structured programminga disciplined approach to writing programs that are clearer, easier to test and debug and easier to modify than large programs produced with previous techniques.
One of the more tangible results of this research was the development of the Pascal programming language by Professor Niklaus Wirth in 1971. Named after the seventeenth-century mathematician and philosopher Blaise Pascal, it was designed for teaching structured programming in academic environments and rapidly became the preferred programming language in most colleges. Pascal lacks many features needed to make it useful in commercial, industrial and government applications, so it has not been widely accepted in these environments.
The Ada programming language was developed under the sponsorship of the U.S. Department of Defense (DOD) during the 1970s and early 1980s. Hundreds of separate languages were being used to produce the DOD's massive command-and-control software systems. The DOD wanted a single language that would fill most of its needs. The Ada language was named after Lady Ada Lovelace, daughter of the poet Lord Byron. Lady Lovelace is credited with writing the world's first computer program in the early 1800s (for the Analytical Engine mechanical computing device designed by Charles Babbage). One important capability of Ada, called multitasking, allows programmers to specify that many activities are to occur in parallel. Java, through a technique called multithreading, also enables programmers to write programs with parallel activities.

[Page 11 (continued)]
1.12. BASIC, Visual Basic, Visual C++, C# and .NET
The BASIC (Beginner's All-Purpose Symbolic Instruction Code) programming language was developed in the mid-1960s at Dartmouth College as a means of writing simple programs. BASIC's primary purpose was to familiarize novices with programming techniques.
Microsoft's Visual Basic language was introduced in the early 1990s to simplify the development of Microsoft Windows applications and is one of the most popular programming languages in the world.
Microsoft's latest development tools are part of its corporate-wide strategy for integrating the Internet and the Web into computer applications. This strategy is implemented in Microsoft's .NET platform, which provides developers with the capabilities they need to create and run computer applications that can execute on computers distributed across the Internet. Microsoft's three primary programming languages are Visual Basic .NET (based on the original BASIC), Visual C++ .NET (based on C++) and C# (a new language based on C++ and Java that was developed expressly for the .NET platform). Developers using .NET can write software components in the language they are most familiar with and then form applications by combining those components with components written in any .NET language.
[Page 12]

[Page 12 (continued)]
1.13. Typical Java Development Environment
We now explain the commonly used steps in creating and executing a Java application using a Java development environment (illustrated in Fig. 1.1).
Figure 1.1. Typical Java development environment.
(This item is displayed on page 13 in the print version)
[View full size image]

Java programs normally go through five phasesedit, compile, load, verify and execute. We discuss these phases in the context of the J2SE Development Kit (JDK) version 5.0 from Sun Microsystems, Inc., which will be wrapped with the book as an accompanying CD once Sun releases the final version of the JDK 5.0. If the CD for your book does not have the JDK on it, you can download both the JDK and its documentation now from java.sun.com/j2se/5.0/download.jsp. For help with the download, visit

servlet.java.sun.com/help/download. Carefully follow the installation instructions for the JDK provided on the CD (or at java.sun.com/j2se/5.0/install.html) to ensure that you set up your computer properly to compile and execute Java programs. Complete installation instructions can also be found on Sun's Java Web site at
java.sun.com/learning/new2java/index.html
[Note: This Web site provides installation instructions for Windows, UNIX/Linux and Mac OS X. If you are not using one of these operating systems, refer to the manuals for your system's Java environment or ask your instructor how to accomplish these tasks based on your computer's operating system. In addition, please keep in mind that Web links occasionally break as companies evolve their Web sites. If you encounter a problem with this link or any other links referenced in this book, please check our Web site (www.deitel.com) for errata and please notify us by e-mail at deitel@deitel.com. We will respond promptly.]
Phase 1: Creating a Program
Phase 1 consists of editing a file with an editor program (normally known simply as an editor). You type a Java program (typically referred to as source code) using the editor, make any necessary corrections and save the program on a secondary storage device, such as your hard drive. Java sourcecode file names end with the .java extension, which indicates that a file contains Java source code. We assume that the reader knows how to edit a file.
Two editors widely used on UNIX/Linux systems are vi and emacs. On Windows, a simple editing program like Windows Notepad will suffice. Many freeware and shareware editors are also available for download from the Internet on sites like www.download.com.
For organizations that develop substantial information systems, integrated development environments (IDEs) are available from many major software suppliers, including Sun Microsystems. IDEs provide many tools that support the software development process, including editors for writing and editing programs and debuggers for locating logic errors in programs.
[Page 14]
Several popular IDEs are NetBeans (www.netbeans.org), jEdit (www.jedit.org), Eclipse (www.eclipse.org), JBuilder (www.borland.com), JCreator (www.jcreator.com), BlueJ (www.blueJ.org) and jGRASP (www.jgrasp.org). Sun Microsystems has the Sun Java Studio (wwws.sun.com/software/sundev/jde/), which is an enhanced version of Net-Beans. [Note: NetBeans v. 3.6, jEdit v. 4.1, jGRASP v. 1.7 and BlueJ v. 1.3.5 are included on the CD that accompanies this book. These IDEs are designed to execute on most major platforms. Our example programs should operate properly with any Java integrated development environment that supports the JDK 5.0. We also provide free Dive Into™ guides for various IDEs on our Web site at www.deitel.com/books/jHTP6/index.html.]
Phase 2: Compiling a Java Program into Bytecodes
In Phase 2, the programmer uses the command javac (the Java compiler) to compile a program. For example, to compile a program called Welcome.java, you would type
javac Welcome.java
in the command window of your system (i.e., the MS-DOS prompt in Windows 95/98/ ME, the Command Prompt in Windows NT/2000/XP, the shell prompt in UNIX/Linux or the Terminal application in Mac OS X). If the program compiles, the compiler produces a .class file called Welcome.class that contains the compiled version of the program.
The Java compiler translates the Java source code into bytecodes that represent the tasks to be performed during the execution phase (Phase 5). Bytecodes are executed by the Java Virtual Machine (JVM)a part of the JDK and the foundation of the Java platform. A virtual machine(VM) is a software application that simulates a computer, but hides the underlying operating system and hardware from the programs that interact with the VM. If the same VM is implemented on many computer platforms, applications that it executes can be used on all those platforms. The JVM is one of the most widely used virtual machines.

Unlike machine language, which is dependent on specific computer hardware, byte-codes are platformindependent instructionsthey are not dependent on a particular hardware platform. So Java's bytecodes are portablethat is, the same bytecodes can execute on any platform containing a JVM that understands the version of Java in which the bytecodes were compiled. The JVM is invoked by the java command. For example, to execute a Java application called Welcome, you would type the command
java Welcome
in a command window to invoke the JVM, which would then initiate the steps necessary to execute the application. This begins Phase 3.
Phase 3: Loading a Program into Memory
In Phase 3, the program must be placed in memory before it can executea process known as loading. The class loader takes the .class files containing the program's bytecodes and transfers them to primary memory. The class loader also loads any of the .class files provided by Java that your program uses. The .class files can be loaded from a disk on your system or over a network (e.g., your local college or company network, or the Internet).
Phase 4: Bytecode Verification
In Phase 4, as the classes are loaded, the bytecode verifier examines their bytecodes to ensure that they are valid and do not violate Java's security restrictions. Java enforces strong security, to make sure that Java programs arriving over the network do not damage your files or your system (as computer viruses and worms might).
[Page 15]
Phase 5: Execution
In Phase 5, the JVM executes the program's bytecodes, thus performing the actions specified by the program. In early Java versions, the JVM was simply an interpreter for Java bytecodes. This caused most Java programs to execute slowly because the JVM would interpret and execute one bytecode at a time. Today's JVMs typically execute bytecodes using a combination of interpretation and so-called just-in- time (JIT) compilation. In this process, The JVM analyzes the bytecodes as they are interpreted, searching for hot spotsparts of the bytecodes that execute frequently. For these parts, a just-in-time (JIT) compilerknown as the Java HotSpot compilertranslates the bytecodes into the underlying computer's machine language. When the JVM encounters these compiled parts again, the faster machinelanguage code executes. Thus Java programs actually go through two compilation phasesone in which source code is translated into bytecodes (for portability across JVMs on different computer platforms) and a second in which, during execution, the bytecodes are translated into machine language for the actual computer on which the program executes.
Problems That May Occur at Execution Time
Programs might not work on the first try. Each of the preceding phases can fail because of various errors that we will discuss throughout this book. For example, an executing program might attempt to divide by zero (an illegal operation for whole-number arithmetic in Java). This would cause the Java program to display an error message. If this occurs, you would have to return to the edit phase, make the necessary corrections and proceed through the remaining phases again to determine that the corrections fix the problem(s). [Note: Most programs in Java input or output data. When we say that a program displays a message, we normally mean that it displays that message on your computer's screen. Messages and other data may be output to other devices, such as disks and hardcopy printers, or even to a network for transmission to other computers.]
Common Programming Error 1.1

Errors like division by zero occur as a program runs, so they are called runtime errors or execution-time errors. Fatal runtime errors cause programs to terminate immediately without having successfully performed their jobs. Nonfatal runtime errors allow programs to run to completion, often producing incorrect results.

[Page 15 (continued)]
1.14. Notes about Java and Java How to Program, Sixth Edition
Java is a powerful programming language. Experienced programmers sometimes take pride in creating weird, contorted, convoluted usage of a language. This is a poor programming practice. It makes programs more difficult to read, more likely to behave strangely, more difficult to test and debug, and more difficult to adapt to changing requirements. This book stresses clarity. The following is our first "good programming practice" tip.
Good Programming Practice 1.1
Write your Java programs in a simple and straightforward manner. This is sometimes referred to as KIS ("keep it simple"). Do not "stretch" the language by trying bizarre usages.
[Page 16]
You have heard that Java is a portable language and that programs written in Java can run on many different computers. For programming in general, portability is an elusive goal. The ANSI C standard document (located at www.ansi.org), which describes the C programming language, contains a lengthy list of portability issues. In fact, whole books have been written to discuss portability, such as Rex Jaeschke's Portability and the C Language.
Portability Tip 1.2
Although it is easier to write portable programs in Java than in other programming languages, differences between compilers, JVMs and computers can make portability difficult to achieve. Simply writing programs in Java does not guarantee portability.
Error-Prevention Tip 1.1
Always test your Java programs on all systems on which you intend to run them, to ensure that they will work correctly for their intended audiences.
We have audited our presentation against Sun's current Java documentation for completeness and accuracy. However, Java is a rich language, and no textbook can cover every topic. A Web-based version of the Java API documentation can be found at java.sun.com/j2se/5.0/docs/api/index.html or you can download this documentation to your own computer from java.sun.com/j2se/5.0/download.html. For additional technical details on Java, visit java.sun.com/reference/docs/index.html. This site provides detailed information about many aspects of Java development, including all three Java platforms.