
- •Cover Page
- •Java Programming: Contents
- •Java Programming: Preface to the Third Edition
- •Java Programming: Preface to Previous Edition
- •Java Programing: Chapter 1
- •Java Programing: Section 1.1
- •Java Programing: Section 1.2
- •Java Programing: Section 1.3
- •Java Programing: Section 1.4
- •Java Programing: Section 1.5
- •Java Programing: Section 1.6
- •Java Programing: Section 1.7
- •Java Programing: Chapter 1 Quiz
- •Java Programing: Chapter 2 Index
- •Java Programing: Section 2.1
- •Java Programing: Section 2.2
- •Java Programing: Section 2.3
- •Java Programing: Section 2.4
- •Java Programing: Section 2.5
- •Java Programing: Chapter 2 Exercises
- •Java Programing: Chapter 2 Quiz
- •Java Programing: Chapter 3 Index
- •Java Programing: Section 3.1
- •Java Programing: Section 3.2
- •Java Programing: Section 3.3
- •Java Programing: Section 3.4
- •Java Programing: Section 3.5
- •Java Programing: Section 3.6
- •Java Programing: Section 3.7
- •Java Programing: Chapter 3 Exercises
- •Java Programing: Chapter 3 Quiz
- •Java Programing: Chapter 4 Index
- •Java Programing: Section 4.1
- •Java Programing: Section 4.2
- •Java Programing: Section 4.3
- •Java Programing: Section 4.4
- •Java Programing: Section 4.5
- •Java Programing: Section 4.6
- •Java Programing: Section 4.7
- •Java Programing: Chapter 4 Exercises
- •Java Programing: Chapter 4 Quiz
- •Java Programing: Chapter 5 Index
- •Java Programing: Section 5.1
- •Java Programing: Section 5.2
- •Java Programing: Section 5.3
- •Java Programing: Section 5.4
- •Java Programing: Section 5.5
- •Java Programing: Chapter 5 Exercises
- •Java Programing: Chapter 5 Quiz
- •Java Programing: Chapter 6 Index
- •Java Programing: Section 6.1
- •Java Programing: Section 6.2
- •Java Programing: Section 6.3
- •Java Programing: Section 6.4
- •Java Programing: Section 6.5
- •Java Programing: Section 6.6
- •Java Programing: Section 6.7
- •Java Programing: Chapter 6 Exercises
- •Java Programing: Chapter 6 Quiz
- •Java Programing: Chapter 7 Index
- •Java Programing: Section 7.1
- •Java Programing: Section 7.2
- •Java Programing: Section 7.3
- •Java Programing: Section 7.4
- •Java Programing: Section 7.5
- •Java Programing: Section 7.6
- •Java Programing: Section 7.7
- •Java Programing: Section 7.8
- •Java Programing: Chapter 7 Exercises
- •Java Programing: Chapter 7 Quiz
- •Java Programing: Chapter 8 Index
- •Java Programing: Section 8.1
- •Java Programing: Section 8.2
- •Java Programing: Section 8.3
- •Java Programing: Section 8.4
- •Java Programing: Section 8.5
- •Java Programing: Chapter 8 Exercises
- •Java Programing: Chapter 8 Quiz
- •Java Programing: Chapter 9 Index
- •Java Programing: Section 9.1
- •Java Programing: Section 9.2
- •Java Programing: Section 9.3
- •Java Programing: Section 9.4
- •Java Programing: Chapter 9 Exercises
- •Java Programing: Chapter 9 Quiz
- •Java Programing: Chapter 10 Index
- •Java Programing: Section 10.1
- •Java Programing: Section 10.2
- •Java Programing: Section 10.3
- •Java Programing: Section 10.4
- •Java Programing: Section 10.5
- •Java Programing: Chapter 10 Exercises
- •Java Programing: Chapter 10 Quiz
- •Java Programing: Chapter 11 Index
- •Java Programing: Section 11.1
- •Java Programing: Section 11.2
- •Java Programing: Section 11.3
- •Java Programing: Section 11.4
- •Java Programing: Section 11.5
- •Java Programming, Chapter 11 Exercises
- •Java Programming, Chapter 11 Quiz
- •Java Programing: Appendix 1 Index
- •Java Programing: Appendix 1, Section 1
- •Java Programing: Appendix 1, Section 2
- •Java Programing: Appendix 1, Section 3
- •Some Notes on Java Programming Environments
Cover Page
Introduction to Programming Using Java Version 3.0, Summer 2000
Author: David J. Eck
Department of Mathematics and Computer Science
Hobart and William Smith Colleges
Geneva, New York 14456
Email: eck@hws.edu
WWW: http://math.hws.edu/eck/
May 24, 2000
This PDF file or printout contains parts of a free textbook that covers introductory programming with Java.
The entire text is available on the World-Wide Web, for use on-line and for downloading,
at this Web address:
http://math.hws.edu/javanotes/
The PDF file and printouts that are made from it do not show the Java applets that are embedded throughout the text. In most places where an applet should appear, you will see a message such as "Sorry, but your Web browser does not support Java." Also not included are Java source code examples from Appendix 3 of the text and solutions to the quizzes and programming exercises. The real version of the textbook is on-line, to be read with a Web browser.
Permission is hereby granted to duplicate and distribute all or part of the following material,
provided that the material is unmodified and that it is accompanied by a copy of this cover page.
http://math.hws.edu/eck/cs124/pdf-front-page.html [5/24/2000 8:32:13 AM]

Java Programming, Main Index
Introduction to Programming Using Java
Version 3.0, Summer 2000
Author: David J. Eck (eck@hws.edu)
WELCOME TO Introduction to Programming Using Java, an on-line textbook on introductory
programming, which uses Java as the language of instruction. This text has more than enough material for a one-semester course, and it also suitable for individuals who want to learn programming on their own. This is the third edition of the text. It covers more material and has more examples than the second edition. It also adds end-of-chapter quizzes and solved programming exercises. Previous editions have been used in a course, Computer Science 124: Introductory Programming, at Hobart and William Smith Colleges. (The
title of the previous editions included a reference to this course.) This textbook covers Java 1.1. Most of the applets that are contained in the text require Java 1.1 or higher.
Links for downloading copies of this text can be found at the bottom of this page. To learn more about this on-line text, including usage restrictions and links to previous editions, please read its preface.
Short Table of Contents:
●Full Table of Contents
●Preface
●Preface to the Second Edition
●Chapter 1: Overview: The Mental Landscape
●Chapter 2: Programming in the Small I: Names and Things
●Chapter 3: Programming in the Small II: Control
●Chapter 4: Programming in the Large I: Subroutines
●Chapter 5: Programming in the Large II: Objects and Classes
●Chapter 6: Applets, HTML, and GUI's
●Chapter 7: Components and Events
●Chapter 8: Arrays
●Chapter 9: Correctness and Robustness
●Chapter 10: Advanced Input/Output
●Chapter 11: Linked Data Structures and Recursion
●Appendix 1: From Java to C++
●Appendix 2: Some Notes on Java Programming Environments
●Appendix 3: Source code for all examples in the text
http://math.hws.edu/javanotes/ (1 of 2) [5/24/2000 8:32:47 AM]
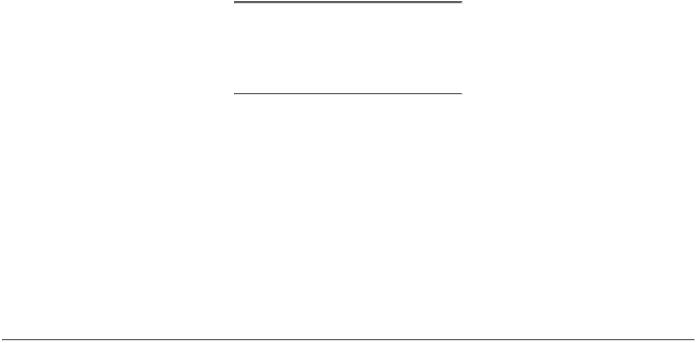
Java Programming, Main Index
● News and Errata
This is a free textbook. The latest edition is always available, at no charge, for downloading and for on-line use at the Web address http://math.hws.edu/javanotes/. This edition, the third, is also permanently
archived at the address http://math.hws.edu/eck/cs124/javanotes3/.
Downloading Links
Use one of the following direct links to download a compressed archive of this entire textbook. You can use this material on your own computer. You can also re-post it in unmodified form on any Web server. See the preface for more information on usage restrictions and for full downloading instructions. A PDF file
that can be used for printing the textbook is also available there.
●http://math.hws.edu/eck/cs124/downloads/javanotes3.zip (1.6 MB), for Windows.
●http://math.hws.edu/eck/cs124/downloads/javanotes3.sit.hqx (2.1 MB), for Macintosh.
●http://math.hws.edu/eck/cs124/downloads/javanotes3.tar.Z (1.8 MB), for Linux/UNIX.
David Eck (eck@hws.edu), May 2000
http://math.hws.edu/javanotes/ (2 of 2) [5/24/2000 8:32:47 AM]
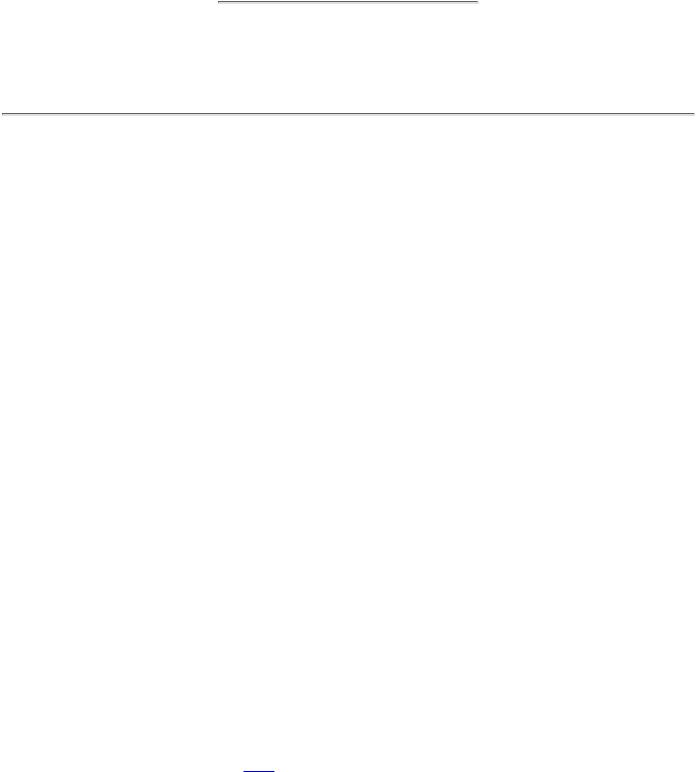
Java Programming: Contents
Introduction to Programming Using Java, Third Edition
Table of Contents
THIS IS THE FULL TABLE OF CONTENTS for an on-line introductory programming textbook that uses Java as the language of instruction. For more information about the text, please see its front page. The text is available on-line at http://math.hws.edu/javanotes/.
Preface
Preface to the Second Edition
Chapter 1: Overview: The Mental Landscape
●Section 1: The Fetch-and-Execute Cycle: Machine Language
●Section 2: Asynchronous Events: Polling Loops and Interrupts
●Section 3: The Java Virtual Machine
●Section 4: Fundamental Building Blocks of Programs
●Section 5: Objects and Object-oriented Programming
●Section 6: The Modern User Interface
●Section 7: The Internet and World-Wide Web
●Quiz on this Chapter
Chapter 2: Programming in the Small I: Names and Things
●Section 1: The Basic Java Application
●Section 2: Variables and the Primitive Types
●Section 3: Strings, Objects, and Subroutines
●Section 4: Text Input and Output
●Section 5: Details of Expressions
●Programming Exercises
●Quiz on this Chapter
Chapter 3: Programming in the Small II: Control
●Section 1: Blocks, Loops, and Branches
●Section 2: Algorithm Development
●Section 3: The while and do..while Statements
●Section 4: The for Statement
●Section 5: The if Statement
http://math.hws.edu/javanotes/contents.html (1 of 4) [5/24/2000 8:32:55 AM]
Java Programming: Contents
●Section 6: The switch Statement
●Section 7: Introduction to Applets and Graphics
●Programming Exercises
●Quiz on this Chapter
Chapter 4: Programming in the Large I: Subroutines
●Section 1: Black Boxes
●Section 2: Static Subroutines and Static Variables
●Section 3: Parameters
●Section 4: Return Values
●Section 5: Toolboxes, API's, and Packages
●Section 6: More on Program Design
●Section 7: The Truth about Declarations
●Programming Exercises
●Quiz on this Chapter
Chapter 5: Programming in the Large II: Objects and Classes
●Section 1: Objects, Instance Variables, and Instance Methods
●Section 2: Constructors and Object Initialization
●Section 3: Programming with Objects
●Section 4: Inheritance, Polymorphism, and Abstract Classes
●Section 5: More Details of Classes
●Programming Exercises
●Quiz on this Chapter
Chapter 6: Applets, HTML, and GUI's
●Section 1: The Basic Java Applet
●Section 2: HTML Basics and the Web
●Section 3: Graphics and the Paint Method
●Section 4: Mouse Events
●Section 5: Keyboard Events
●Section 6: Introduction to Layouts and Components
●Section 7: Looking Back: The Java 1.0 Event Model
●Programming Exercises
●Quiz on this Chapter
Chapter 7: Advanced GUI Programming
●Section 1: More about Graphics
●Section 2: More about Layouts and Components
http://math.hws.edu/javanotes/contents.html (2 of 4) [5/24/2000 8:32:55 AM]
Java Programming: Contents
●Section 3: Standard Components and Their Events
●Section 4: Programming with Components
●Section 5: Threads, Synchronization, and Animation
●Section 6: Nested Classes and Adapter Classes
●Section 7: Frames and Dialogs
●Section 8: Looking Forward: Swing and Java 2.0
●Programming Exercises
●Quiz on this Chapter
Chapter 8: Arrays
●Section 1: Creating and Using Arrays
●Section 2: Programming with Arrays
●Section 3: Vectors and Dynamic Arrays
●Section 4: Searching and Sorting
●Section 5: Multi-Dimensional Arrays
●Programming Exercises
●Quiz on this Chapter
Chapter 9: Correctness and Robustness
●Section 1: Introduction to Correctness and Robustness
●Section 2: Writing Correct Programs
●Section 3: Exceptions and the try...catch Statement
●Section 4: Programming with Exceptions
●Programming Exercises
●Quiz on this Chapter
Chapter 10: Advanced Input/Output
●Section 1: Streams, Readers, and Writers
●Section 2: Files
●Section 3: Programming with Files
●Section 4: Networking
●Section 5: Programming Networked Applications
●Programming Exercises
●Quiz on this Chapter
Chapter 11: Linked Data Structures and Recursion
●Section 1: Recursion
●Section 2: Linking Objects
●Section 3: Stacks and Queues
http://math.hws.edu/javanotes/contents.html (3 of 4) [5/24/2000 8:32:55 AM]

Java Programming: Contents
●Section 4: Binary Trees
●Section 5: A Simple Recursive-descent Parser
●Programming Exercises
●Quiz on this Chapter
Appendix 1: From Java to C++
●Section 1: C++ Programming Fundamentals
●Section 2: Pointers and Arrays in C++
●Section 3: Classes and Objects in C++
Appendix 2: Some Notes on Java Programming Environments
Appendix 3: Source code for all examples in the text
News and Errata
David J. Eck (eck@hws.edu), May 2000
http://math.hws.edu/javanotes/contents.html (4 of 4) [5/24/2000 8:32:55 AM]